How to fetch and store [[PromiseValue]] into local variable?
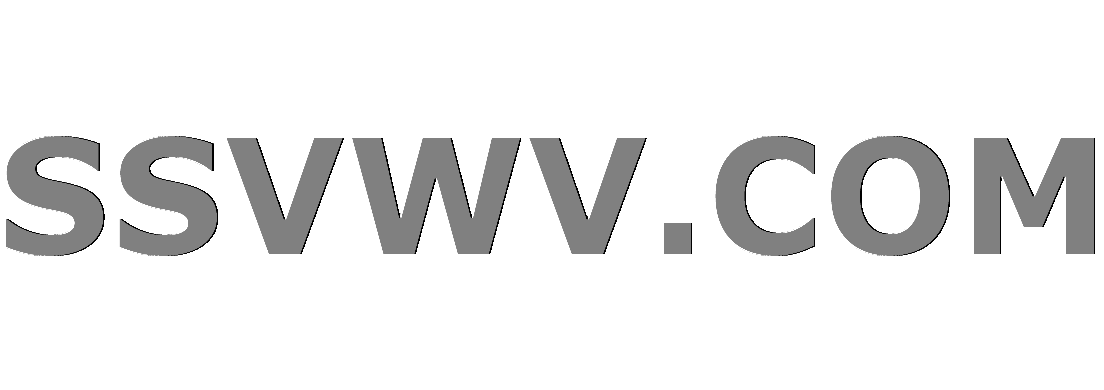
Multi tool use
There are two functions; function getThumbnail(url)
and function getVimeoData()
.
I'm using fetch()
to handle the HTTP
calls for retrieving the url
of thumbnail of a video in Vimeo. oEmbed is used to fetch JSON data of a video, which contains thumbnail_url
.
The functions goes as follow:
async function getVimeoThumb(videoUrl)
return await fetch(videoUrl)
.then(response =>
if (response.status !== 200)
console.log('Error occurred while fetching thumbnail.');
return null;
return response.json();
)
.then(data => return data['thumbnail_url'])
.catch(err => console.log(err.reason));
The above function returns Promise with a value [[PromiseValue]]: "thumbnail_url"
. The returned Promise value is fetched in following function as:
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => console.log(result))
...
The above function returns console log as thumbnail_url
.
Hence, my question is that is there any workaround to store the fetched value thumbnail_url
in my local variable newUrl
?
javascript fetch es6-promise vimeo-api
add a comment |
There are two functions; function getThumbnail(url)
and function getVimeoData()
.
I'm using fetch()
to handle the HTTP
calls for retrieving the url
of thumbnail of a video in Vimeo. oEmbed is used to fetch JSON data of a video, which contains thumbnail_url
.
The functions goes as follow:
async function getVimeoThumb(videoUrl)
return await fetch(videoUrl)
.then(response =>
if (response.status !== 200)
console.log('Error occurred while fetching thumbnail.');
return null;
return response.json();
)
.then(data => return data['thumbnail_url'])
.catch(err => console.log(err.reason));
The above function returns Promise with a value [[PromiseValue]]: "thumbnail_url"
. The returned Promise value is fetched in following function as:
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => console.log(result))
...
The above function returns console log as thumbnail_url
.
Hence, my question is that is there any workaround to store the fetched value thumbnail_url
in my local variable newUrl
?
javascript fetch es6-promise vimeo-api
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27
add a comment |
There are two functions; function getThumbnail(url)
and function getVimeoData()
.
I'm using fetch()
to handle the HTTP
calls for retrieving the url
of thumbnail of a video in Vimeo. oEmbed is used to fetch JSON data of a video, which contains thumbnail_url
.
The functions goes as follow:
async function getVimeoThumb(videoUrl)
return await fetch(videoUrl)
.then(response =>
if (response.status !== 200)
console.log('Error occurred while fetching thumbnail.');
return null;
return response.json();
)
.then(data => return data['thumbnail_url'])
.catch(err => console.log(err.reason));
The above function returns Promise with a value [[PromiseValue]]: "thumbnail_url"
. The returned Promise value is fetched in following function as:
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => console.log(result))
...
The above function returns console log as thumbnail_url
.
Hence, my question is that is there any workaround to store the fetched value thumbnail_url
in my local variable newUrl
?
javascript fetch es6-promise vimeo-api
There are two functions; function getThumbnail(url)
and function getVimeoData()
.
I'm using fetch()
to handle the HTTP
calls for retrieving the url
of thumbnail of a video in Vimeo. oEmbed is used to fetch JSON data of a video, which contains thumbnail_url
.
The functions goes as follow:
async function getVimeoThumb(videoUrl)
return await fetch(videoUrl)
.then(response =>
if (response.status !== 200)
console.log('Error occurred while fetching thumbnail.');
return null;
return response.json();
)
.then(data => return data['thumbnail_url'])
.catch(err => console.log(err.reason));
The above function returns Promise with a value [[PromiseValue]]: "thumbnail_url"
. The returned Promise value is fetched in following function as:
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => console.log(result))
...
The above function returns console log as thumbnail_url
.
Hence, my question is that is there any workaround to store the fetched value thumbnail_url
in my local variable newUrl
?
javascript fetch es6-promise vimeo-api
javascript fetch es6-promise vimeo-api
asked Nov 14 '18 at 9:15


Pa-wonPa-won
6517
6517
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27
add a comment |
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27
add a comment |
2 Answers
2
active
oldest
votes
You already have a local variable (newUrl), so where you are logging the result you can save there only
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
1
While this might work in theory, it is not usable in practice. You don't know whennewUrl
is actually defined. The correct answer to this question can be found in the duplicate.
– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
add a comment |
@Nitich is right. But if what you want is use newUrl out side you can try this. newUrl will be in the global scope.
let newUrl;
function getThumbnailUrl()
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
Or simply do
...
getVimeoThumb(url).then(result => newUrl = result; return newUrl; )
...
}
let newUrl = getThumbnailUrl(videoUrl);
I hope this helps.
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296596%2fhow-to-fetch-and-store-promisevalue-into-local-variable%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You already have a local variable (newUrl), so where you are logging the result you can save there only
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
1
While this might work in theory, it is not usable in practice. You don't know whennewUrl
is actually defined. The correct answer to this question can be found in the duplicate.
– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
add a comment |
You already have a local variable (newUrl), so where you are logging the result you can save there only
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
1
While this might work in theory, it is not usable in practice. You don't know whennewUrl
is actually defined. The correct answer to this question can be found in the duplicate.
– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
add a comment |
You already have a local variable (newUrl), so where you are logging the result you can save there only
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
You already have a local variable (newUrl), so where you are logging the result you can save there only
function getThumbnailUrl()
let newUrl;
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
answered Nov 14 '18 at 9:18


Nitish NarangNitish Narang
2,9401815
2,9401815
1
While this might work in theory, it is not usable in practice. You don't know whennewUrl
is actually defined. The correct answer to this question can be found in the duplicate.
– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
add a comment |
1
While this might work in theory, it is not usable in practice. You don't know whennewUrl
is actually defined. The correct answer to this question can be found in the duplicate.
– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
1
1
While this might work in theory, it is not usable in practice. You don't know when
newUrl
is actually defined. The correct answer to this question can be found in the duplicate.– str
Nov 14 '18 at 9:28
While this might work in theory, it is not usable in practice. You don't know when
newUrl
is actually defined. The correct answer to this question can be found in the duplicate.– str
Nov 14 '18 at 9:28
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
I get that @str . Though not clearly stated in OP that async is causing trouble or not getting proper response from async calls rather it was menitioned that results are coming just needed to save to local variable. Still it looks your insight could be correct on this. Thnx
– Nitish Narang
Nov 14 '18 at 10:49
add a comment |
@Nitich is right. But if what you want is use newUrl out side you can try this. newUrl will be in the global scope.
let newUrl;
function getThumbnailUrl()
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
Or simply do
...
getVimeoThumb(url).then(result => newUrl = result; return newUrl; )
...
}
let newUrl = getThumbnailUrl(videoUrl);
I hope this helps.
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
add a comment |
@Nitich is right. But if what you want is use newUrl out side you can try this. newUrl will be in the global scope.
let newUrl;
function getThumbnailUrl()
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
Or simply do
...
getVimeoThumb(url).then(result => newUrl = result; return newUrl; )
...
}
let newUrl = getThumbnailUrl(videoUrl);
I hope this helps.
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
add a comment |
@Nitich is right. But if what you want is use newUrl out side you can try this. newUrl will be in the global scope.
let newUrl;
function getThumbnailUrl()
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
Or simply do
...
getVimeoThumb(url).then(result => newUrl = result; return newUrl; )
...
}
let newUrl = getThumbnailUrl(videoUrl);
I hope this helps.
@Nitich is right. But if what you want is use newUrl out side you can try this. newUrl will be in the global scope.
let newUrl;
function getThumbnailUrl()
...
getVimeoThumb(url).then(result => newUrl = result; console.log(result) )
...
Or simply do
...
getVimeoThumb(url).then(result => newUrl = result; return newUrl; )
...
}
let newUrl = getThumbnailUrl(videoUrl);
I hope this helps.
edited Nov 14 '18 at 10:20
answered Nov 14 '18 at 10:14
Kareem_BincomKareem_Bincom
161
161
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
add a comment |
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
No he is not quite right. Your first example has the same limitations as his. And the second example will return a promise, not the URL.
– str
Nov 14 '18 at 10:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296596%2fhow-to-fetch-and-store-promisevalue-into-local-variable%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bW2JYbN5Ea94ovA,q9FyIg3G1,I5hoURK8pJsm5lF6RXFk722l1TAC6k8v9Ozo3kQH,KNRVe4bd9YqVRbYyxOLWC7DU
Possible duplicate of How do I return the response from an asynchronous call?
– str
Nov 14 '18 at 9:27