Python storing & accessing information via txt file
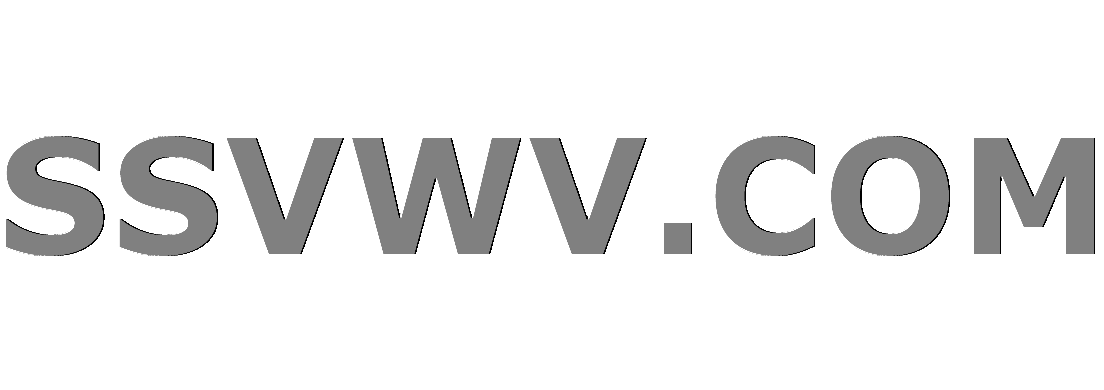
Multi tool use
I'm writing a gambling/dice game program for a course I'm taking, each week new requirements are added, and we have to implement the new code w/o radically changing the code we have written thus far. The part I'm having trouble with is: right now, if the user quits (by entering "0" as their "bet"), the contents of their "bank" is saved to a text file, so they can resume play later with the same amount of "winnings". If the user goes bankrupt (game over), the next time they run the program their bank is reset to the default amount ($500). When the program starts, the code is supposed to check to see if a file holding previous play data (bank.txt) exists; if the file exists, the number in bank.txt is the user's "bank"; if the file doesn't exist, the program creates it and adds $500 to the user's bank. By tweaking things here and there I've gotten parts of the code to work (i.e., quit with $800 in bank, restart game and it's still there), but I've been at it so long (and am so frustrated) I just can't figure out what I'm doing wrong here.
> `import os
import random
def main():
intro()
bank = check_bank()
show_bank(bank)
bet = bet_validation(bank)`
That's the beginning of the program, obviously, the part I can't seem to get right is below... If I need to post more of the code, that's not a problem, it's just that everything else seems to work right (except for the following)
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
if float(bank_file.readline()) > 0.0:
bank = float(bank_file.readline())
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
Any and all help/suggestions/tips would be greatly appreciated. When I start the program, I get a "ValueError" exception from the "check_bank()" function. I'd be happy to provide the rest of my code, if necessary, just included this bit because it seems to be where I'm having the problem. If you can help, please let me know what I'm doing wrong, and how to correct it! Thanks in advance guys!
python python-3.x
add a comment |
I'm writing a gambling/dice game program for a course I'm taking, each week new requirements are added, and we have to implement the new code w/o radically changing the code we have written thus far. The part I'm having trouble with is: right now, if the user quits (by entering "0" as their "bet"), the contents of their "bank" is saved to a text file, so they can resume play later with the same amount of "winnings". If the user goes bankrupt (game over), the next time they run the program their bank is reset to the default amount ($500). When the program starts, the code is supposed to check to see if a file holding previous play data (bank.txt) exists; if the file exists, the number in bank.txt is the user's "bank"; if the file doesn't exist, the program creates it and adds $500 to the user's bank. By tweaking things here and there I've gotten parts of the code to work (i.e., quit with $800 in bank, restart game and it's still there), but I've been at it so long (and am so frustrated) I just can't figure out what I'm doing wrong here.
> `import os
import random
def main():
intro()
bank = check_bank()
show_bank(bank)
bet = bet_validation(bank)`
That's the beginning of the program, obviously, the part I can't seem to get right is below... If I need to post more of the code, that's not a problem, it's just that everything else seems to work right (except for the following)
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
if float(bank_file.readline()) > 0.0:
bank = float(bank_file.readline())
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
Any and all help/suggestions/tips would be greatly appreciated. When I start the program, I get a "ValueError" exception from the "check_bank()" function. I'd be happy to provide the rest of my code, if necessary, just included this bit because it seems to be where I'm having the problem. If you can help, please let me know what I'm doing wrong, and how to correct it! Thanks in advance guys!
python python-3.x
Can you replaceexcept ValueError
toexcept ValueError as e
and thenprint(e)
? Or just show a stack trace? Also you mixed up yourelse
branch.
– sashaaero
Nov 13 '18 at 2:23
1
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Causefloat('500.0')
will work in 100% of cases.
– sashaaero
Nov 13 '18 at 2:51
add a comment |
I'm writing a gambling/dice game program for a course I'm taking, each week new requirements are added, and we have to implement the new code w/o radically changing the code we have written thus far. The part I'm having trouble with is: right now, if the user quits (by entering "0" as their "bet"), the contents of their "bank" is saved to a text file, so they can resume play later with the same amount of "winnings". If the user goes bankrupt (game over), the next time they run the program their bank is reset to the default amount ($500). When the program starts, the code is supposed to check to see if a file holding previous play data (bank.txt) exists; if the file exists, the number in bank.txt is the user's "bank"; if the file doesn't exist, the program creates it and adds $500 to the user's bank. By tweaking things here and there I've gotten parts of the code to work (i.e., quit with $800 in bank, restart game and it's still there), but I've been at it so long (and am so frustrated) I just can't figure out what I'm doing wrong here.
> `import os
import random
def main():
intro()
bank = check_bank()
show_bank(bank)
bet = bet_validation(bank)`
That's the beginning of the program, obviously, the part I can't seem to get right is below... If I need to post more of the code, that's not a problem, it's just that everything else seems to work right (except for the following)
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
if float(bank_file.readline()) > 0.0:
bank = float(bank_file.readline())
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
Any and all help/suggestions/tips would be greatly appreciated. When I start the program, I get a "ValueError" exception from the "check_bank()" function. I'd be happy to provide the rest of my code, if necessary, just included this bit because it seems to be where I'm having the problem. If you can help, please let me know what I'm doing wrong, and how to correct it! Thanks in advance guys!
python python-3.x
I'm writing a gambling/dice game program for a course I'm taking, each week new requirements are added, and we have to implement the new code w/o radically changing the code we have written thus far. The part I'm having trouble with is: right now, if the user quits (by entering "0" as their "bet"), the contents of their "bank" is saved to a text file, so they can resume play later with the same amount of "winnings". If the user goes bankrupt (game over), the next time they run the program their bank is reset to the default amount ($500). When the program starts, the code is supposed to check to see if a file holding previous play data (bank.txt) exists; if the file exists, the number in bank.txt is the user's "bank"; if the file doesn't exist, the program creates it and adds $500 to the user's bank. By tweaking things here and there I've gotten parts of the code to work (i.e., quit with $800 in bank, restart game and it's still there), but I've been at it so long (and am so frustrated) I just can't figure out what I'm doing wrong here.
> `import os
import random
def main():
intro()
bank = check_bank()
show_bank(bank)
bet = bet_validation(bank)`
That's the beginning of the program, obviously, the part I can't seem to get right is below... If I need to post more of the code, that's not a problem, it's just that everything else seems to work right (except for the following)
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
if float(bank_file.readline()) > 0.0:
bank = float(bank_file.readline())
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
Any and all help/suggestions/tips would be greatly appreciated. When I start the program, I get a "ValueError" exception from the "check_bank()" function. I'd be happy to provide the rest of my code, if necessary, just included this bit because it seems to be where I'm having the problem. If you can help, please let me know what I'm doing wrong, and how to correct it! Thanks in advance guys!
python python-3.x
python python-3.x
edited Nov 13 '18 at 2:47


Peanut Butter Vibes
1429
1429
asked Nov 13 '18 at 2:17


deHartdeHart
358
358
Can you replaceexcept ValueError
toexcept ValueError as e
and thenprint(e)
? Or just show a stack trace? Also you mixed up yourelse
branch.
– sashaaero
Nov 13 '18 at 2:23
1
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Causefloat('500.0')
will work in 100% of cases.
– sashaaero
Nov 13 '18 at 2:51
add a comment |
Can you replaceexcept ValueError
toexcept ValueError as e
and thenprint(e)
? Or just show a stack trace? Also you mixed up yourelse
branch.
– sashaaero
Nov 13 '18 at 2:23
1
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Causefloat('500.0')
will work in 100% of cases.
– sashaaero
Nov 13 '18 at 2:51
Can you replace
except ValueError
to except ValueError as e
and then print(e)
? Or just show a stack trace? Also you mixed up your else
branch.– sashaaero
Nov 13 '18 at 2:23
Can you replace
except ValueError
to except ValueError as e
and then print(e)
? Or just show a stack trace? Also you mixed up your else
branch.– sashaaero
Nov 13 '18 at 2:23
1
1
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Cause
float('500.0')
will work in 100% of cases.– sashaaero
Nov 13 '18 at 2:51
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Cause
float('500.0')
will work in 100% of cases.– sashaaero
Nov 13 '18 at 2:51
add a comment |
3 Answers
3
active
oldest
votes
As @deHart mentions, the exception is "Could not convert string to float". The problem is that you are reading the first line to check the text file, and then reading the next (empty) line to set the bank.
Instead, read the line and store to a variable. Then, do the comparison and set the bank from this variable - that should fix the ValueError.
Here is the modified version that worked for me:
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
vault = float(bank_file.readline())
if vault > 0.0:
bank = vault
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
A few notes here:
- This 'fixes' the problem, but you could still throw a ValueError.
- This may be covered elsewhere, but you'll want to update the text file when the bank drops below 0!
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
add a comment |
Finally cracked it! Changed the following code around, and bingo!
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
bank = float(bank_file.readline())
if bank > 0:
return bank
else:
bank = 500.0
return bank
add a comment |
You should not store your values in plain text. I prefer using pickle
import pickle, os
def check_bank():
try:
file_path = './bank.txt'
#ckeck if file exists
if os.path.isfile(file_path):
file_handler = open(file_path,'rb')
bank = pickle.load(file_handler)
return bank
#if the file doesn't exist
else:
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
except IOError:
print("IOError occured")
except EOFError:
#if the file is empty
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272820%2fpython-storing-accessing-information-via-txt-file%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
As @deHart mentions, the exception is "Could not convert string to float". The problem is that you are reading the first line to check the text file, and then reading the next (empty) line to set the bank.
Instead, read the line and store to a variable. Then, do the comparison and set the bank from this variable - that should fix the ValueError.
Here is the modified version that worked for me:
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
vault = float(bank_file.readline())
if vault > 0.0:
bank = vault
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
A few notes here:
- This 'fixes' the problem, but you could still throw a ValueError.
- This may be covered elsewhere, but you'll want to update the text file when the bank drops below 0!
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
add a comment |
As @deHart mentions, the exception is "Could not convert string to float". The problem is that you are reading the first line to check the text file, and then reading the next (empty) line to set the bank.
Instead, read the line and store to a variable. Then, do the comparison and set the bank from this variable - that should fix the ValueError.
Here is the modified version that worked for me:
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
vault = float(bank_file.readline())
if vault > 0.0:
bank = vault
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
A few notes here:
- This 'fixes' the problem, but you could still throw a ValueError.
- This may be covered elsewhere, but you'll want to update the text file when the bank drops below 0!
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
add a comment |
As @deHart mentions, the exception is "Could not convert string to float". The problem is that you are reading the first line to check the text file, and then reading the next (empty) line to set the bank.
Instead, read the line and store to a variable. Then, do the comparison and set the bank from this variable - that should fix the ValueError.
Here is the modified version that worked for me:
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
vault = float(bank_file.readline())
if vault > 0.0:
bank = vault
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
A few notes here:
- This 'fixes' the problem, but you could still throw a ValueError.
- This may be covered elsewhere, but you'll want to update the text file when the bank drops below 0!
As @deHart mentions, the exception is "Could not convert string to float". The problem is that you are reading the first line to check the text file, and then reading the next (empty) line to set the bank.
Instead, read the line and store to a variable. Then, do the comparison and set the bank from this variable - that should fix the ValueError.
Here is the modified version that worked for me:
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
vault = float(bank_file.readline())
if vault > 0.0:
bank = vault
return bank
else:
bank = 500.00
return bank
else:
bank_file = open('bank.txt','w')
bank_file.write(str(500.0))
bank = float(bank_file.readline())
bank_file.close()
return bank
except IOError:
print('IOError in check_bank')
except ValueError:
print('ValueError in check_bank')
except Exception as err:
print(err,'in check_bank')
finally:
bank_file.close()
A few notes here:
- This 'fixes' the problem, but you could still throw a ValueError.
- This may be covered elsewhere, but you'll want to update the text file when the bank drops below 0!
answered Nov 13 '18 at 2:55


Peanut Butter VibesPeanut Butter Vibes
1429
1429
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
add a comment |
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
1
1
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
Good job! You beat me to the answer by approximately 12 minutes! I was having a real hard time. There is code present to keep bank from dipping below 0 (game over once bank hits 0, input validation to keep player from betting more than they have in bank). I really, really appreciate that you took the time to help me out man!
– deHart
Nov 13 '18 at 3:14
add a comment |
Finally cracked it! Changed the following code around, and bingo!
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
bank = float(bank_file.readline())
if bank > 0:
return bank
else:
bank = 500.0
return bank
add a comment |
Finally cracked it! Changed the following code around, and bingo!
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
bank = float(bank_file.readline())
if bank > 0:
return bank
else:
bank = 500.0
return bank
add a comment |
Finally cracked it! Changed the following code around, and bingo!
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
bank = float(bank_file.readline())
if bank > 0:
return bank
else:
bank = 500.0
return bank
Finally cracked it! Changed the following code around, and bingo!
def check_bank():
try:
if os.path.isfile('./bank.txt') == True:
bank_file = open('bank.txt','r')
bank = float(bank_file.readline())
if bank > 0:
return bank
else:
bank = 500.0
return bank
answered Nov 13 '18 at 3:11


deHartdeHart
358
358
add a comment |
add a comment |
You should not store your values in plain text. I prefer using pickle
import pickle, os
def check_bank():
try:
file_path = './bank.txt'
#ckeck if file exists
if os.path.isfile(file_path):
file_handler = open(file_path,'rb')
bank = pickle.load(file_handler)
return bank
#if the file doesn't exist
else:
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
except IOError:
print("IOError occured")
except EOFError:
#if the file is empty
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
add a comment |
You should not store your values in plain text. I prefer using pickle
import pickle, os
def check_bank():
try:
file_path = './bank.txt'
#ckeck if file exists
if os.path.isfile(file_path):
file_handler = open(file_path,'rb')
bank = pickle.load(file_handler)
return bank
#if the file doesn't exist
else:
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
except IOError:
print("IOError occured")
except EOFError:
#if the file is empty
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
add a comment |
You should not store your values in plain text. I prefer using pickle
import pickle, os
def check_bank():
try:
file_path = './bank.txt'
#ckeck if file exists
if os.path.isfile(file_path):
file_handler = open(file_path,'rb')
bank = pickle.load(file_handler)
return bank
#if the file doesn't exist
else:
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
except IOError:
print("IOError occured")
except EOFError:
#if the file is empty
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
You should not store your values in plain text. I prefer using pickle
import pickle, os
def check_bank():
try:
file_path = './bank.txt'
#ckeck if file exists
if os.path.isfile(file_path):
file_handler = open(file_path,'rb')
bank = pickle.load(file_handler)
return bank
#if the file doesn't exist
else:
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
except IOError:
print("IOError occured")
except EOFError:
#if the file is empty
file_handler = open(file_path, 'wb')
pickle.dump(500, file_handler)
file_handler.close()
return 500
answered Nov 13 '18 at 3:11


DoniDoni
13
13
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
add a comment |
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
Still being rather new, this answer is a bit beyond me... But I am intrigued. I will definitely be looking into what you've shown me here, and hopefully learn all I can from it.
– deHart
Nov 13 '18 at 3:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272820%2fpython-storing-accessing-information-via-txt-file%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
O,RQ,vG9OaeRq,K70RN0C
Can you replace
except ValueError
toexcept ValueError as e
and thenprint(e)
? Or just show a stack trace? Also you mixed up yourelse
branch.– sashaaero
Nov 13 '18 at 2:23
1
"Could not convert string to float"... The "string" in question is "500.0"
– deHart
Nov 13 '18 at 2:47
try to save that string into object and print it also to be sure that it doesn't contain wrong characters. Cause
float('500.0')
will work in 100% of cases.– sashaaero
Nov 13 '18 at 2:51