Image resizing, IndexError: list assignment index out of range
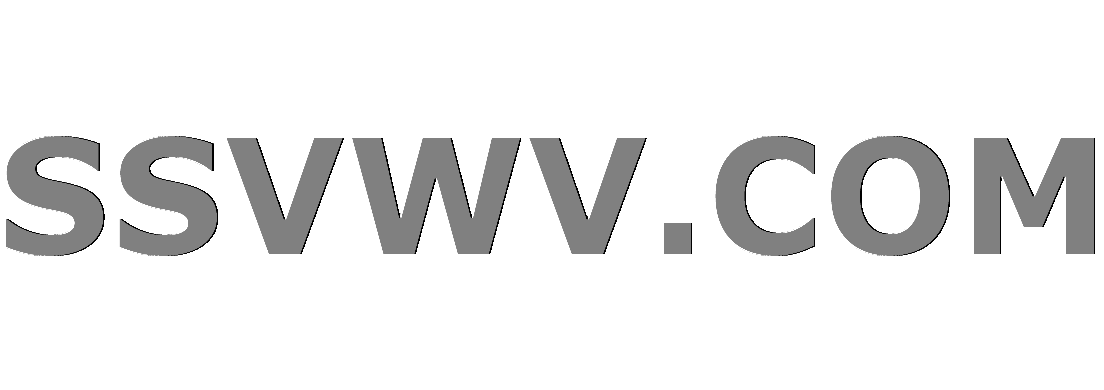
Multi tool use
I have the assignment to scale/ resize an image without the use functions from external libraries and need to create the algorithm myself, have searched, but the code that I have found on different forums have not worked, but I have come up with a code that I would believe would work, but I get the index error on a side note I also need to make forward and backward mapping in relation to scaling the image.
My code can be seen below
import cv2 as cv
img = cv.imread('Scale.jpg', 1)
cv.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2) :
retval = [w2,h2]
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
y_ratio = (int)((h1<<16)/h2) +1
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range (h2):
i += 1
for j in range(w2):
j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
return retval;
dst = resizePixels(img,h,w,300,300)
#cv.imshow('Resize',dst)
cv.waitKey(0)
EDIT: This is the Traceback I receive
Traceback (most recent call last):
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 27, in
<module>
dst = resizePixels(img,h,w,300,300)
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 23, in
resizePixels
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
IndexError: list assignment index out of range
The picture I use to scale
python-3.x opencv image-processing image-resizing index-error
add a comment |
I have the assignment to scale/ resize an image without the use functions from external libraries and need to create the algorithm myself, have searched, but the code that I have found on different forums have not worked, but I have come up with a code that I would believe would work, but I get the index error on a side note I also need to make forward and backward mapping in relation to scaling the image.
My code can be seen below
import cv2 as cv
img = cv.imread('Scale.jpg', 1)
cv.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2) :
retval = [w2,h2]
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
y_ratio = (int)((h1<<16)/h2) +1
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range (h2):
i += 1
for j in range(w2):
j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
return retval;
dst = resizePixels(img,h,w,300,300)
#cv.imshow('Resize',dst)
cv.waitKey(0)
EDIT: This is the Traceback I receive
Traceback (most recent call last):
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 27, in
<module>
dst = resizePixels(img,h,w,300,300)
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 23, in
resizePixels
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
IndexError: list assignment index out of range
The picture I use to scale
python-3.x opencv image-processing image-resizing index-error
add a comment |
I have the assignment to scale/ resize an image without the use functions from external libraries and need to create the algorithm myself, have searched, but the code that I have found on different forums have not worked, but I have come up with a code that I would believe would work, but I get the index error on a side note I also need to make forward and backward mapping in relation to scaling the image.
My code can be seen below
import cv2 as cv
img = cv.imread('Scale.jpg', 1)
cv.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2) :
retval = [w2,h2]
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
y_ratio = (int)((h1<<16)/h2) +1
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range (h2):
i += 1
for j in range(w2):
j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
return retval;
dst = resizePixels(img,h,w,300,300)
#cv.imshow('Resize',dst)
cv.waitKey(0)
EDIT: This is the Traceback I receive
Traceback (most recent call last):
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 27, in
<module>
dst = resizePixels(img,h,w,300,300)
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 23, in
resizePixels
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
IndexError: list assignment index out of range
The picture I use to scale
python-3.x opencv image-processing image-resizing index-error
I have the assignment to scale/ resize an image without the use functions from external libraries and need to create the algorithm myself, have searched, but the code that I have found on different forums have not worked, but I have come up with a code that I would believe would work, but I get the index error on a side note I also need to make forward and backward mapping in relation to scaling the image.
My code can be seen below
import cv2 as cv
img = cv.imread('Scale.jpg', 1)
cv.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2) :
retval = [w2,h2]
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
y_ratio = (int)((h1<<16)/h2) +1
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range (h2):
i += 1
for j in range(w2):
j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
return retval;
dst = resizePixels(img,h,w,300,300)
#cv.imshow('Resize',dst)
cv.waitKey(0)
EDIT: This is the Traceback I receive
Traceback (most recent call last):
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 27, in
<module>
dst = resizePixels(img,h,w,300,300)
File "C:/Users/Asus/PycharmProjects/Scaling/Scaling.py", line 23, in
resizePixels
retval[(i*w2)+j] = pixels[(y2*w1)+x2]
IndexError: list assignment index out of range
The picture I use to scale
python-3.x opencv image-processing image-resizing index-error
python-3.x opencv image-processing image-resizing index-error
edited Nov 13 '18 at 9:16
Frederik Jensen
asked Nov 13 '18 at 8:03


Frederik JensenFrederik Jensen
85
85
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I make some little changes in your code and it works. Read my comment in the code for more detail.
import cv2
import numpy as np
img = cv2.imread('1.png', 0)
cv2.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2):
retval =
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
print(x_ratio)
y_ratio = (int)((h1<<16)/h2) +1
print(y_ratio)
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range(h2):
# no need to have this: i += 1
for j in range(w2):
# no need to have this too: j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
#add pixel values from original image to an array
retval.append(pixels[y2,x2])
return retval;
ret = resizePixels(img,w,h,300,300)
# reshape the array to get the resize image
dst = np.reshape(ret,(300,300))
cv2.imshow('Resize',dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53276369%2fimage-resizing-indexerror-list-assignment-index-out-of-range%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I make some little changes in your code and it works. Read my comment in the code for more detail.
import cv2
import numpy as np
img = cv2.imread('1.png', 0)
cv2.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2):
retval =
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
print(x_ratio)
y_ratio = (int)((h1<<16)/h2) +1
print(y_ratio)
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range(h2):
# no need to have this: i += 1
for j in range(w2):
# no need to have this too: j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
#add pixel values from original image to an array
retval.append(pixels[y2,x2])
return retval;
ret = resizePixels(img,w,h,300,300)
# reshape the array to get the resize image
dst = np.reshape(ret,(300,300))
cv2.imshow('Resize',dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
add a comment |
I make some little changes in your code and it works. Read my comment in the code for more detail.
import cv2
import numpy as np
img = cv2.imread('1.png', 0)
cv2.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2):
retval =
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
print(x_ratio)
y_ratio = (int)((h1<<16)/h2) +1
print(y_ratio)
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range(h2):
# no need to have this: i += 1
for j in range(w2):
# no need to have this too: j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
#add pixel values from original image to an array
retval.append(pixels[y2,x2])
return retval;
ret = resizePixels(img,w,h,300,300)
# reshape the array to get the resize image
dst = np.reshape(ret,(300,300))
cv2.imshow('Resize',dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
add a comment |
I make some little changes in your code and it works. Read my comment in the code for more detail.
import cv2
import numpy as np
img = cv2.imread('1.png', 0)
cv2.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2):
retval =
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
print(x_ratio)
y_ratio = (int)((h1<<16)/h2) +1
print(y_ratio)
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range(h2):
# no need to have this: i += 1
for j in range(w2):
# no need to have this too: j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
#add pixel values from original image to an array
retval.append(pixels[y2,x2])
return retval;
ret = resizePixels(img,w,h,300,300)
# reshape the array to get the resize image
dst = np.reshape(ret,(300,300))
cv2.imshow('Resize',dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
I make some little changes in your code and it works. Read my comment in the code for more detail.
import cv2
import numpy as np
img = cv2.imread('1.png', 0)
cv2.imshow('unscaled', img)
h,w = img.shape[:2]
print(h)
print(w)
def resizePixels(pixels,w1,h1,w2,h2):
retval =
# EDIT: added +1 to remedy an early rounding problem
x_ratio = (int)((w1<<16)/w2) +1
print(x_ratio)
y_ratio = (int)((h1<<16)/h2) +1
print(y_ratio)
#int x_ratio = (int)((w1<<16)/w2)
#int y_ratio = (int)((h1<<16)/h2)
#two = int(x2,y2)
for i in range(h2):
# no need to have this: i += 1
for j in range(w2):
# no need to have this too: j += 1
x2 = ((j*x_ratio)>>16)
y2 = ((i*y_ratio)>>16)
#add pixel values from original image to an array
retval.append(pixels[y2,x2])
return retval;
ret = resizePixels(img,w,h,300,300)
# reshape the array to get the resize image
dst = np.reshape(ret,(300,300))
cv2.imshow('Resize',dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
answered Nov 14 '18 at 7:55
Ha BomHa Bom
7352418
7352418
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53276369%2fimage-resizing-indexerror-list-assignment-index-out-of-range%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s OYRQ9kRln0aVP yaT2fLnDK0M8