c++ sorting vector pairs quickly as part of a genetic learning algorithm
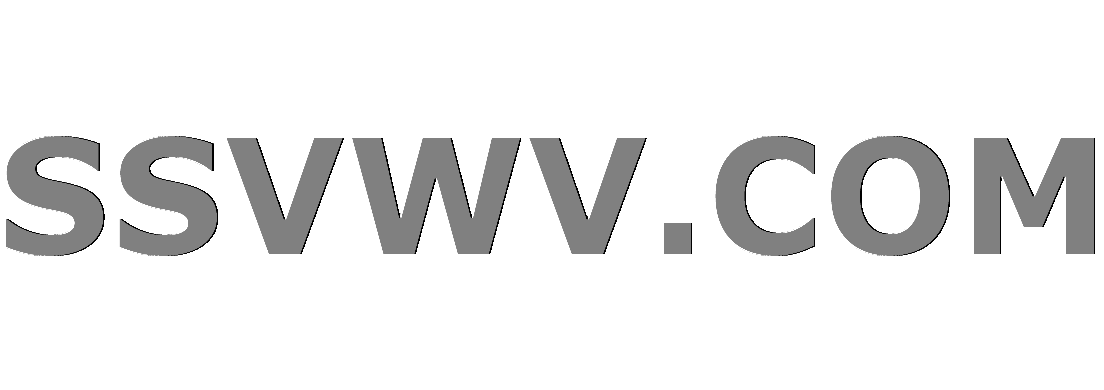
Multi tool use
I have a very interesting problem, I've started developing a genetic learning algorithm and have succeeded on doing so. its a simple GA designed to find a phrase by randomly selecting characters to store into strings and using the standard selection and mutation methods to progress until the it has the final answer, and sometimes this works perfectly.
However, sometimes there's one character incorrect.
I think this is due to the sorting algorithm being slow.
this is what i have so far
This is the loop code
while (!word.Get_found())
generation++;
word.Calculate_fitness();
word.Selection(); //selection
word.Crossover(); //crossover
system("cls");
std::cout << "Generation: " << generation << " Highest fitness: " << word.get_fittest() << " with string: " << word.get_item() << "n";
This is the code for the fitness function
void Guess_word::Calculate_fitness()// calculates fittness based on guess
word against matching string;
for (int i = 0; i < population.size(); i++)
population.at(i).second = 0;
for (int j = 0; j < population.at(i).first.size(); j++)
if (population.at(i).first.at(j) == Phrase.at(j))
population.at(i).second += 1;//calculate fitness
if (population.at(i).second == Phrase.size() && population.at(i).first == Phrase)
found = true;
And this is the selection function
void Guess_word::Selection()//determine highest fitness of population and make them parents
//i hate stable sort....
//it indicates to sort in pairs and keep them together
std::sort(population.begin(), population.end(), (auto &a, auto &b) return a.second > b.second; );
//select two random parent from mating pool
parents.clear();
parents.push_back(population.at(0));
parents.push_back(population.at(1));
The population entities are in vector pairs with strings and ints representing the guess and fitness respectively.
after debugging the code i found that the population does indeed contain the correct guess but with the wrong fitness, i think the sorting algorithm moves the ints faster than the paired strings. meaning that during the fitness function it selects an item as the answer that is one character incorrect however with the correct fitness moved from another vector entity.
I've tried using stable sort and moving the algorithm around to see if timing is a problem. however, no dice.
is there a way to either make the program wait for the sort to complete (which is inefficient in terms of time) or a way to either make the sort faster or implement a faster custom sorting algorithm which would be much more efficient especially on older hardware.
Any suggestions would be greatly appreciated!
c++ algorithm artificial-intelligence
|
show 6 more comments
I have a very interesting problem, I've started developing a genetic learning algorithm and have succeeded on doing so. its a simple GA designed to find a phrase by randomly selecting characters to store into strings and using the standard selection and mutation methods to progress until the it has the final answer, and sometimes this works perfectly.
However, sometimes there's one character incorrect.
I think this is due to the sorting algorithm being slow.
this is what i have so far
This is the loop code
while (!word.Get_found())
generation++;
word.Calculate_fitness();
word.Selection(); //selection
word.Crossover(); //crossover
system("cls");
std::cout << "Generation: " << generation << " Highest fitness: " << word.get_fittest() << " with string: " << word.get_item() << "n";
This is the code for the fitness function
void Guess_word::Calculate_fitness()// calculates fittness based on guess
word against matching string;
for (int i = 0; i < population.size(); i++)
population.at(i).second = 0;
for (int j = 0; j < population.at(i).first.size(); j++)
if (population.at(i).first.at(j) == Phrase.at(j))
population.at(i).second += 1;//calculate fitness
if (population.at(i).second == Phrase.size() && population.at(i).first == Phrase)
found = true;
And this is the selection function
void Guess_word::Selection()//determine highest fitness of population and make them parents
//i hate stable sort....
//it indicates to sort in pairs and keep them together
std::sort(population.begin(), population.end(), (auto &a, auto &b) return a.second > b.second; );
//select two random parent from mating pool
parents.clear();
parents.push_back(population.at(0));
parents.push_back(population.at(1));
The population entities are in vector pairs with strings and ints representing the guess and fitness respectively.
after debugging the code i found that the population does indeed contain the correct guess but with the wrong fitness, i think the sorting algorithm moves the ints faster than the paired strings. meaning that during the fitness function it selects an item as the answer that is one character incorrect however with the correct fitness moved from another vector entity.
I've tried using stable sort and moving the algorithm around to see if timing is a problem. however, no dice.
is there a way to either make the program wait for the sort to complete (which is inefficient in terms of time) or a way to either make the sort faster or implement a faster custom sorting algorithm which would be much more efficient especially on older hardware.
Any suggestions would be greatly appreciated!
c++ algorithm artificial-intelligence
This doesn't address the question, but in that loop inCalculate_fitness()
,i
will always be greater than or equal to 0 and less thanpopulation.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless becausei
will always be in bounds.population[i]
works just fine.
– Pete Becker
Nov 13 '18 at 15:55
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
2
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
1
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop callsword.Selection()
.word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in thewhile
loop goes on, callingword.Crossover()
. Everything here just goes one step after another;word.Selection()
won't return before the sort has been done, and thewhile
loop won't do anything untilword.Selection()
returns.
– Pete Becker
Nov 13 '18 at 16:52
1
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug instd::sort
– Alan Birtles
Nov 13 '18 at 17:08
|
show 6 more comments
I have a very interesting problem, I've started developing a genetic learning algorithm and have succeeded on doing so. its a simple GA designed to find a phrase by randomly selecting characters to store into strings and using the standard selection and mutation methods to progress until the it has the final answer, and sometimes this works perfectly.
However, sometimes there's one character incorrect.
I think this is due to the sorting algorithm being slow.
this is what i have so far
This is the loop code
while (!word.Get_found())
generation++;
word.Calculate_fitness();
word.Selection(); //selection
word.Crossover(); //crossover
system("cls");
std::cout << "Generation: " << generation << " Highest fitness: " << word.get_fittest() << " with string: " << word.get_item() << "n";
This is the code for the fitness function
void Guess_word::Calculate_fitness()// calculates fittness based on guess
word against matching string;
for (int i = 0; i < population.size(); i++)
population.at(i).second = 0;
for (int j = 0; j < population.at(i).first.size(); j++)
if (population.at(i).first.at(j) == Phrase.at(j))
population.at(i).second += 1;//calculate fitness
if (population.at(i).second == Phrase.size() && population.at(i).first == Phrase)
found = true;
And this is the selection function
void Guess_word::Selection()//determine highest fitness of population and make them parents
//i hate stable sort....
//it indicates to sort in pairs and keep them together
std::sort(population.begin(), population.end(), (auto &a, auto &b) return a.second > b.second; );
//select two random parent from mating pool
parents.clear();
parents.push_back(population.at(0));
parents.push_back(population.at(1));
The population entities are in vector pairs with strings and ints representing the guess and fitness respectively.
after debugging the code i found that the population does indeed contain the correct guess but with the wrong fitness, i think the sorting algorithm moves the ints faster than the paired strings. meaning that during the fitness function it selects an item as the answer that is one character incorrect however with the correct fitness moved from another vector entity.
I've tried using stable sort and moving the algorithm around to see if timing is a problem. however, no dice.
is there a way to either make the program wait for the sort to complete (which is inefficient in terms of time) or a way to either make the sort faster or implement a faster custom sorting algorithm which would be much more efficient especially on older hardware.
Any suggestions would be greatly appreciated!
c++ algorithm artificial-intelligence
I have a very interesting problem, I've started developing a genetic learning algorithm and have succeeded on doing so. its a simple GA designed to find a phrase by randomly selecting characters to store into strings and using the standard selection and mutation methods to progress until the it has the final answer, and sometimes this works perfectly.
However, sometimes there's one character incorrect.
I think this is due to the sorting algorithm being slow.
this is what i have so far
This is the loop code
while (!word.Get_found())
generation++;
word.Calculate_fitness();
word.Selection(); //selection
word.Crossover(); //crossover
system("cls");
std::cout << "Generation: " << generation << " Highest fitness: " << word.get_fittest() << " with string: " << word.get_item() << "n";
This is the code for the fitness function
void Guess_word::Calculate_fitness()// calculates fittness based on guess
word against matching string;
for (int i = 0; i < population.size(); i++)
population.at(i).second = 0;
for (int j = 0; j < population.at(i).first.size(); j++)
if (population.at(i).first.at(j) == Phrase.at(j))
population.at(i).second += 1;//calculate fitness
if (population.at(i).second == Phrase.size() && population.at(i).first == Phrase)
found = true;
And this is the selection function
void Guess_word::Selection()//determine highest fitness of population and make them parents
//i hate stable sort....
//it indicates to sort in pairs and keep them together
std::sort(population.begin(), population.end(), (auto &a, auto &b) return a.second > b.second; );
//select two random parent from mating pool
parents.clear();
parents.push_back(population.at(0));
parents.push_back(population.at(1));
The population entities are in vector pairs with strings and ints representing the guess and fitness respectively.
after debugging the code i found that the population does indeed contain the correct guess but with the wrong fitness, i think the sorting algorithm moves the ints faster than the paired strings. meaning that during the fitness function it selects an item as the answer that is one character incorrect however with the correct fitness moved from another vector entity.
I've tried using stable sort and moving the algorithm around to see if timing is a problem. however, no dice.
is there a way to either make the program wait for the sort to complete (which is inefficient in terms of time) or a way to either make the sort faster or implement a faster custom sorting algorithm which would be much more efficient especially on older hardware.
Any suggestions would be greatly appreciated!
c++ algorithm artificial-intelligence
c++ algorithm artificial-intelligence
asked Nov 13 '18 at 15:50
AlexNotTheLionAlexNotTheLion
85
85
This doesn't address the question, but in that loop inCalculate_fitness()
,i
will always be greater than or equal to 0 and less thanpopulation.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless becausei
will always be in bounds.population[i]
works just fine.
– Pete Becker
Nov 13 '18 at 15:55
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
2
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
1
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop callsword.Selection()
.word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in thewhile
loop goes on, callingword.Crossover()
. Everything here just goes one step after another;word.Selection()
won't return before the sort has been done, and thewhile
loop won't do anything untilword.Selection()
returns.
– Pete Becker
Nov 13 '18 at 16:52
1
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug instd::sort
– Alan Birtles
Nov 13 '18 at 17:08
|
show 6 more comments
This doesn't address the question, but in that loop inCalculate_fitness()
,i
will always be greater than or equal to 0 and less thanpopulation.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless becausei
will always be in bounds.population[i]
works just fine.
– Pete Becker
Nov 13 '18 at 15:55
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
2
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
1
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop callsword.Selection()
.word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in thewhile
loop goes on, callingword.Crossover()
. Everything here just goes one step after another;word.Selection()
won't return before the sort has been done, and thewhile
loop won't do anything untilword.Selection()
returns.
– Pete Becker
Nov 13 '18 at 16:52
1
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug instd::sort
– Alan Birtles
Nov 13 '18 at 17:08
This doesn't address the question, but in that loop in
Calculate_fitness()
, i
will always be greater than or equal to 0 and less than population.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless because i
will always be in bounds. population[i]
works just fine.– Pete Becker
Nov 13 '18 at 15:55
This doesn't address the question, but in that loop in
Calculate_fitness()
, i
will always be greater than or equal to 0 and less than population.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless because i
will always be in bounds. population[i]
works just fine.– Pete Becker
Nov 13 '18 at 15:55
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
2
2
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
1
1
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop calls
word.Selection()
. word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in the while
loop goes on, calling word.Crossover()
. Everything here just goes one step after another; word.Selection()
won't return before the sort has been done, and the while
loop won't do anything until word.Selection()
returns.– Pete Becker
Nov 13 '18 at 16:52
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop calls
word.Selection()
. word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in the while
loop goes on, calling word.Crossover()
. Everything here just goes one step after another; word.Selection()
won't return before the sort has been done, and the while
loop won't do anything until word.Selection()
returns.– Pete Becker
Nov 13 '18 at 16:52
1
1
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug in
std::sort
– Alan Birtles
Nov 13 '18 at 17:08
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug in
std::sort
– Alan Birtles
Nov 13 '18 at 17:08
|
show 6 more comments
1 Answer
1
active
oldest
votes
The issue was simply, the code doing a cross over and storing it back in position 0 of the population making it change randomly just before the final result was displayed
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284692%2fc-sorting-vector-pairs-quickly-as-part-of-a-genetic-learning-algorithm%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The issue was simply, the code doing a cross over and storing it back in position 0 of the population making it change randomly just before the final result was displayed
add a comment |
The issue was simply, the code doing a cross over and storing it back in position 0 of the population making it change randomly just before the final result was displayed
add a comment |
The issue was simply, the code doing a cross over and storing it back in position 0 of the population making it change randomly just before the final result was displayed
The issue was simply, the code doing a cross over and storing it back in position 0 of the population making it change randomly just before the final result was displayed
answered Nov 13 '18 at 20:22
AlexNotTheLionAlexNotTheLion
85
85
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284692%2fc-sorting-vector-pairs-quickly-as-part-of-a-genetic-learning-algorithm%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2tkQI neri,O,0QYTx1gz22zZ,QG4MW84Yn2m,n XTa,VhzVs0 9gG0utB
This doesn't address the question, but in that loop in
Calculate_fitness()
,i
will always be greater than or equal to 0 and less thanpopulation.size()
, because that's what the loop says. All those bounds checks (population.at(i)
) are pointless becausei
will always be in bounds.population[i]
works just fine.– Pete Becker
Nov 13 '18 at 15:55
@PeteBecker ah thank you, I've not used vectors before and wasn't following a great tutorial, i'm changing that now thank you !
– AlexNotTheLion
Nov 13 '18 at 15:59
2
There's nothing in this code that's time-sensitive. Why would slow sorting affect the result?
– Pete Becker
Nov 13 '18 at 15:59
1
@AlexNotTheLion -- re: "it breaks before it can finish sorting" -- not with the code you've shown. The code in the while loop calls
word.Selection()
.word.Selection()
does the sorting, then does some more stuff. When it's finished it returns, and the code in thewhile
loop goes on, callingword.Crossover()
. Everything here just goes one step after another;word.Selection()
won't return before the sort has been done, and thewhile
loop won't do anything untilword.Selection()
returns.– Pete Becker
Nov 13 '18 at 16:52
1
Please provide a Minimal, Complete, and Verifiable example, the problem is more likely to be in your code than a bug in
std::sort
– Alan Birtles
Nov 13 '18 at 17:08