how to make switch case with characters work? [duplicate]
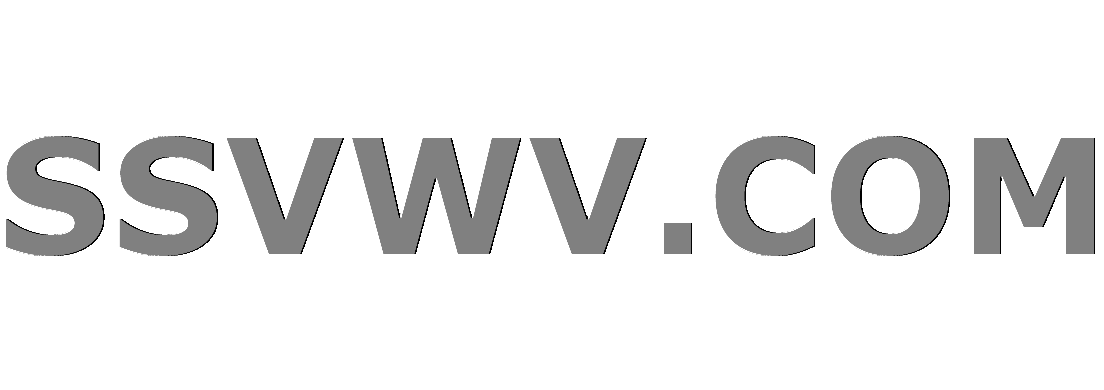
Multi tool use
up vote
0
down vote
favorite
This question already has an answer here:
scanf() leaves the new line char in the buffer
3 answers
I'm currently working on an assignment for my class. In C, I have to make a program that works with the roster of a soccer team, where you can update, replace, compare players etc. However, right now I cannot make any of the options in my menu work. This will probably be better understood with my code:
#include <stdio.h>
int main(void)
int i, jersey, rating, newJersey, newRating, playerJerseyNumber[5], playerRating[5];
char choice;
for (i = 0; i < 5; i++)
printf("Enter player %d's jersey number: n", (i + 1));
scanf("%d", &playerJerseyNumber[i]);
printf("Enter player %d's rating: nn", (i + 1));
scanf("%d", &playerRating[i]);
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
printf("nnMENU nu - Update player rating na - Output players above a rating nr - Replace player no - Output roster nq - Quitnn");
printf("Choose an option: n");
scanf("%c", &choice);
switch (choice)
case 'u':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new rating for player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerRating[i] = newRating;
break;
case 'a':
printf("Enter a rating: n");
scanf("%d", &rating);
printf("n ABOVE %dn", rating);
for (i = 0; i < 5; i++)
if (playerRating[i] > rating)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
case 'r':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new jersey number: n");
scanf("%d", &newJersey);
printf("Enter a rating for the new player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerJerseyNumber[i] = newJersey;
playerRating[i] = newRating;
break;
case 'o':
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
default:
printf("didnt work");
break;
return 0;
now, the first part of my code works correctly. however, if i try to use any of the options in the menu, they do not work. it automatically goes to the default case and prints "didn't work".
right now, i am testing with
84 7
23 4
4 5
30 2
66 9
u
4
6
o
q
which does not work, even though it should update jersey #4 to a rating of 6.
Any ideas on why this isn't working? thanks.
c arrays switch-statement
marked as duplicate by Lundin
StackExchange.ready(function()
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function()
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function()
$hover.showInfoMessage('',
messageElement: $msg.clone().show(),
transient: false,
position: my: 'bottom left', at: 'top center', offsetTop: -7 ,
dismissable: false,
relativeToBody: true
);
,
function()
StackExchange.helpers.removeMessages();
);
);
);
Nov 12 at 10:30
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
|
show 3 more comments
up vote
0
down vote
favorite
This question already has an answer here:
scanf() leaves the new line char in the buffer
3 answers
I'm currently working on an assignment for my class. In C, I have to make a program that works with the roster of a soccer team, where you can update, replace, compare players etc. However, right now I cannot make any of the options in my menu work. This will probably be better understood with my code:
#include <stdio.h>
int main(void)
int i, jersey, rating, newJersey, newRating, playerJerseyNumber[5], playerRating[5];
char choice;
for (i = 0; i < 5; i++)
printf("Enter player %d's jersey number: n", (i + 1));
scanf("%d", &playerJerseyNumber[i]);
printf("Enter player %d's rating: nn", (i + 1));
scanf("%d", &playerRating[i]);
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
printf("nnMENU nu - Update player rating na - Output players above a rating nr - Replace player no - Output roster nq - Quitnn");
printf("Choose an option: n");
scanf("%c", &choice);
switch (choice)
case 'u':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new rating for player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerRating[i] = newRating;
break;
case 'a':
printf("Enter a rating: n");
scanf("%d", &rating);
printf("n ABOVE %dn", rating);
for (i = 0; i < 5; i++)
if (playerRating[i] > rating)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
case 'r':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new jersey number: n");
scanf("%d", &newJersey);
printf("Enter a rating for the new player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerJerseyNumber[i] = newJersey;
playerRating[i] = newRating;
break;
case 'o':
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
default:
printf("didnt work");
break;
return 0;
now, the first part of my code works correctly. however, if i try to use any of the options in the menu, they do not work. it automatically goes to the default case and prints "didn't work".
right now, i am testing with
84 7
23 4
4 5
30 2
66 9
u
4
6
o
q
which does not work, even though it should update jersey #4 to a rating of 6.
Any ideas on why this isn't working? thanks.
c arrays switch-statement
marked as duplicate by Lundin
StackExchange.ready(function()
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function()
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function()
$hover.showInfoMessage('',
messageElement: $msg.clone().show(),
transient: false,
position: my: 'bottom left', at: 'top center', offsetTop: -7 ,
dismissable: false,
relativeToBody: true
);
,
function()
StackExchange.helpers.removeMessages();
);
);
);
Nov 12 at 10:30
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
2
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Please see scanf() leaves the newline char in the buffer because you usescanf("%c", &choice);
as the next input afterscanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
You don't even need a debugger. Just printing the value ofchoice
would lead you toward an answer.
– John3136
Nov 11 at 22:51
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
@Swordfish ...perhaps thedefault: printf("didnt work");
– Weather Vane
Nov 11 at 22:54
|
show 3 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This question already has an answer here:
scanf() leaves the new line char in the buffer
3 answers
I'm currently working on an assignment for my class. In C, I have to make a program that works with the roster of a soccer team, where you can update, replace, compare players etc. However, right now I cannot make any of the options in my menu work. This will probably be better understood with my code:
#include <stdio.h>
int main(void)
int i, jersey, rating, newJersey, newRating, playerJerseyNumber[5], playerRating[5];
char choice;
for (i = 0; i < 5; i++)
printf("Enter player %d's jersey number: n", (i + 1));
scanf("%d", &playerJerseyNumber[i]);
printf("Enter player %d's rating: nn", (i + 1));
scanf("%d", &playerRating[i]);
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
printf("nnMENU nu - Update player rating na - Output players above a rating nr - Replace player no - Output roster nq - Quitnn");
printf("Choose an option: n");
scanf("%c", &choice);
switch (choice)
case 'u':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new rating for player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerRating[i] = newRating;
break;
case 'a':
printf("Enter a rating: n");
scanf("%d", &rating);
printf("n ABOVE %dn", rating);
for (i = 0; i < 5; i++)
if (playerRating[i] > rating)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
case 'r':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new jersey number: n");
scanf("%d", &newJersey);
printf("Enter a rating for the new player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerJerseyNumber[i] = newJersey;
playerRating[i] = newRating;
break;
case 'o':
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
default:
printf("didnt work");
break;
return 0;
now, the first part of my code works correctly. however, if i try to use any of the options in the menu, they do not work. it automatically goes to the default case and prints "didn't work".
right now, i am testing with
84 7
23 4
4 5
30 2
66 9
u
4
6
o
q
which does not work, even though it should update jersey #4 to a rating of 6.
Any ideas on why this isn't working? thanks.
c arrays switch-statement
This question already has an answer here:
scanf() leaves the new line char in the buffer
3 answers
I'm currently working on an assignment for my class. In C, I have to make a program that works with the roster of a soccer team, where you can update, replace, compare players etc. However, right now I cannot make any of the options in my menu work. This will probably be better understood with my code:
#include <stdio.h>
int main(void)
int i, jersey, rating, newJersey, newRating, playerJerseyNumber[5], playerRating[5];
char choice;
for (i = 0; i < 5; i++)
printf("Enter player %d's jersey number: n", (i + 1));
scanf("%d", &playerJerseyNumber[i]);
printf("Enter player %d's rating: nn", (i + 1));
scanf("%d", &playerRating[i]);
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
printf("nnMENU nu - Update player rating na - Output players above a rating nr - Replace player no - Output roster nq - Quitnn");
printf("Choose an option: n");
scanf("%c", &choice);
switch (choice)
case 'u':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new rating for player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerRating[i] = newRating;
break;
case 'a':
printf("Enter a rating: n");
scanf("%d", &rating);
printf("n ABOVE %dn", rating);
for (i = 0; i < 5; i++)
if (playerRating[i] > rating)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
case 'r':
printf("Enter a jersey number: n");
scanf("%d", &jersey);
printf("Enter a new jersey number: n");
scanf("%d", &newJersey);
printf("Enter a rating for the new player: n");
scanf("%d", &newRating);
for (i = 0; i < 5; i++)
if (jersey == playerJerseyNumber[i])
playerJerseyNumber[i] = newJersey;
playerRating[i] = newRating;
break;
case 'o':
printf("ROSTERn");
for (i = 0; i < 5; i++)
printf("Player %d -- Jersey number: %d, Rating: %dn", (i + 1), playerJerseyNumber[i], playerRating[i]);
break;
default:
printf("didnt work");
break;
return 0;
now, the first part of my code works correctly. however, if i try to use any of the options in the menu, they do not work. it automatically goes to the default case and prints "didn't work".
right now, i am testing with
84 7
23 4
4 5
30 2
66 9
u
4
6
o
q
which does not work, even though it should update jersey #4 to a rating of 6.
Any ideas on why this isn't working? thanks.
This question already has an answer here:
scanf() leaves the new line char in the buffer
3 answers
c arrays switch-statement
c arrays switch-statement
edited Nov 11 at 22:51


Swordfish
1
1
asked Nov 11 at 22:35


rmgk
213
213
marked as duplicate by Lundin
StackExchange.ready(function()
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function()
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function()
$hover.showInfoMessage('',
messageElement: $msg.clone().show(),
transient: false,
position: my: 'bottom left', at: 'top center', offsetTop: -7 ,
dismissable: false,
relativeToBody: true
);
,
function()
StackExchange.helpers.removeMessages();
);
);
);
Nov 12 at 10:30
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
marked as duplicate by Lundin
StackExchange.ready(function()
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function()
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function()
$hover.showInfoMessage('',
messageElement: $msg.clone().show(),
transient: false,
position: my: 'bottom left', at: 'top center', offsetTop: -7 ,
dismissable: false,
relativeToBody: true
);
,
function()
StackExchange.helpers.removeMessages();
);
);
);
Nov 12 at 10:30
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
2
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Please see scanf() leaves the newline char in the buffer because you usescanf("%c", &choice);
as the next input afterscanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
You don't even need a debugger. Just printing the value ofchoice
would lead you toward an answer.
– John3136
Nov 11 at 22:51
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
@Swordfish ...perhaps thedefault: printf("didnt work");
– Weather Vane
Nov 11 at 22:54
|
show 3 more comments
2
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Please see scanf() leaves the newline char in the buffer because you usescanf("%c", &choice);
as the next input afterscanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
You don't even need a debugger. Just printing the value ofchoice
would lead you toward an answer.
– John3136
Nov 11 at 22:51
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
@Swordfish ...perhaps thedefault: printf("didnt work");
– Weather Vane
Nov 11 at 22:54
2
2
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Please see scanf() leaves the newline char in the buffer because you use
scanf("%c", &choice);
as the next input after scanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
Please see scanf() leaves the newline char in the buffer because you use
scanf("%c", &choice);
as the next input after scanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
You don't even need a debugger. Just printing the value of
choice
would lead you toward an answer.– John3136
Nov 11 at 22:51
You don't even need a debugger. Just printing the value of
choice
would lead you toward an answer.– John3136
Nov 11 at 22:51
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
@Swordfish ...perhaps the
default: printf("didnt work");
– Weather Vane
Nov 11 at 22:54
@Swordfish ...perhaps the
default: printf("didnt work");
– Weather Vane
Nov 11 at 22:54
|
show 3 more comments
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
use scanf(" %c", &choice)
The blank in the format string tells scanf to skip leading whitespace, and the first non-whitespace character will be read with the %c conversion specifier.
Refer Here
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
use scanf(" %c", &choice)
The blank in the format string tells scanf to skip leading whitespace, and the first non-whitespace character will be read with the %c conversion specifier.
Refer Here
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
add a comment |
up vote
2
down vote
accepted
use scanf(" %c", &choice)
The blank in the format string tells scanf to skip leading whitespace, and the first non-whitespace character will be read with the %c conversion specifier.
Refer Here
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
use scanf(" %c", &choice)
The blank in the format string tells scanf to skip leading whitespace, and the first non-whitespace character will be read with the %c conversion specifier.
Refer Here
use scanf(" %c", &choice)
The blank in the format string tells scanf to skip leading whitespace, and the first non-whitespace character will be read with the %c conversion specifier.
Refer Here
answered Nov 11 at 23:14
harry
1857
1857
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
add a comment |
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
thank you for the simple and correct answer :) it works now
– rmgk
Nov 12 at 0:54
add a comment |
UA,wbvxxTK,Sehq2,bsan0ovJFrK FY6Me3K
2
Have you tried a debugger?
– Matthieu Brucher
Nov 11 at 22:42
Please see scanf() leaves the newline char in the buffer because you use
scanf("%c", &choice);
as the next input afterscanf("%d", &playerRating[i]);
– Weather Vane
Nov 11 at 22:43
You don't even need a debugger. Just printing the value of
choice
would lead you toward an answer.– John3136
Nov 11 at 22:51
"does not work" is a very poor description.
– Swordfish
Nov 11 at 22:53
@Swordfish ...perhaps the
default: printf("didnt work");
– Weather Vane
Nov 11 at 22:54