JavaFX: table columns fit width with background load
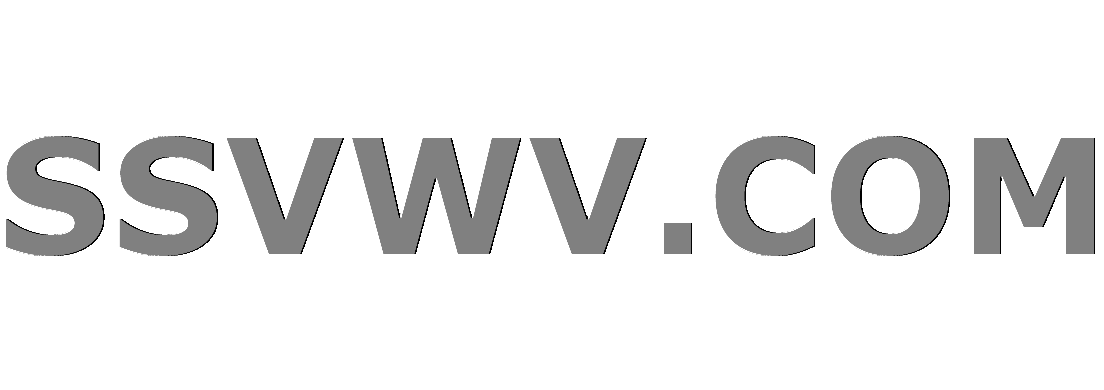
Multi tool use
up vote
1
down vote
favorite
I hava a JavaFX table with two columns. Depending on whether the code to populate it is written before or after showing the scene, the columns fit their content or not.
Before (expected result):
After (actual result):
Why is that? Is there any way I can tell the table to do the same adjusting when I'll be loading the data asynchronously (by request), after the table was constructed and displayed?
Thanks in advance
Complete working example:
import javafx.application.Application;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Example extends Application
@Override
public void start(Stage primaryStage) throws Exception
primaryStage.setTitle("Test");
TableView<Person> table = new TableView<>();
ObservableList<Person> people = FXCollections.observableArrayList(
new Person("Very very very very long name", 100),
new Person("Not much shorter name", 44));
TableColumn nameColumn = new TableColumn("First Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<Person, String>("name"));
table.getColumns().add(nameColumn);
TableColumn ageColumn = new TableColumn("Age");
ageColumn.setCellValueFactory(new PropertyValueFactory<Person, Integer>("age"));
table.getColumns().add(ageColumn);
// table.setItems(people); /* Here it works */
BorderPane root = new BorderPane();
root.setCenter(table);
primaryStage.setScene(new Scene(root, 700, 400));
primaryStage.show();
table.setItems(people); /* Here it does not */
public static void main(String args)
launch(args);
public static class Person
private SimpleStringProperty name = new SimpleStringProperty();
private SimpleIntegerProperty age = new SimpleIntegerProperty();
public Person(String name, int age)
super();
this.name.set(name);
this.age.set(age);
public String getName()
return name.get();
public Integer getAge()
return age.get();
java javafx javafx-8 desktop-application
|
show 1 more comment
up vote
1
down vote
favorite
I hava a JavaFX table with two columns. Depending on whether the code to populate it is written before or after showing the scene, the columns fit their content or not.
Before (expected result):
After (actual result):
Why is that? Is there any way I can tell the table to do the same adjusting when I'll be loading the data asynchronously (by request), after the table was constructed and displayed?
Thanks in advance
Complete working example:
import javafx.application.Application;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Example extends Application
@Override
public void start(Stage primaryStage) throws Exception
primaryStage.setTitle("Test");
TableView<Person> table = new TableView<>();
ObservableList<Person> people = FXCollections.observableArrayList(
new Person("Very very very very long name", 100),
new Person("Not much shorter name", 44));
TableColumn nameColumn = new TableColumn("First Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<Person, String>("name"));
table.getColumns().add(nameColumn);
TableColumn ageColumn = new TableColumn("Age");
ageColumn.setCellValueFactory(new PropertyValueFactory<Person, Integer>("age"));
table.getColumns().add(ageColumn);
// table.setItems(people); /* Here it works */
BorderPane root = new BorderPane();
root.setCenter(table);
primaryStage.setScene(new Scene(root, 700, 400));
primaryStage.show();
table.setItems(people); /* Here it does not */
public static void main(String args)
launch(args);
public static class Person
private SimpleStringProperty name = new SimpleStringProperty();
private SimpleIntegerProperty age = new SimpleIntegerProperty();
public Person(String name, int age)
super();
this.name.set(name);
this.age.set(age);
public String getName()
return name.get();
public Integer getAge()
return age.get();
java javafx javafx-8 desktop-application
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11
|
show 1 more comment
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I hava a JavaFX table with two columns. Depending on whether the code to populate it is written before or after showing the scene, the columns fit their content or not.
Before (expected result):
After (actual result):
Why is that? Is there any way I can tell the table to do the same adjusting when I'll be loading the data asynchronously (by request), after the table was constructed and displayed?
Thanks in advance
Complete working example:
import javafx.application.Application;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Example extends Application
@Override
public void start(Stage primaryStage) throws Exception
primaryStage.setTitle("Test");
TableView<Person> table = new TableView<>();
ObservableList<Person> people = FXCollections.observableArrayList(
new Person("Very very very very long name", 100),
new Person("Not much shorter name", 44));
TableColumn nameColumn = new TableColumn("First Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<Person, String>("name"));
table.getColumns().add(nameColumn);
TableColumn ageColumn = new TableColumn("Age");
ageColumn.setCellValueFactory(new PropertyValueFactory<Person, Integer>("age"));
table.getColumns().add(ageColumn);
// table.setItems(people); /* Here it works */
BorderPane root = new BorderPane();
root.setCenter(table);
primaryStage.setScene(new Scene(root, 700, 400));
primaryStage.show();
table.setItems(people); /* Here it does not */
public static void main(String args)
launch(args);
public static class Person
private SimpleStringProperty name = new SimpleStringProperty();
private SimpleIntegerProperty age = new SimpleIntegerProperty();
public Person(String name, int age)
super();
this.name.set(name);
this.age.set(age);
public String getName()
return name.get();
public Integer getAge()
return age.get();
java javafx javafx-8 desktop-application
I hava a JavaFX table with two columns. Depending on whether the code to populate it is written before or after showing the scene, the columns fit their content or not.
Before (expected result):
After (actual result):
Why is that? Is there any way I can tell the table to do the same adjusting when I'll be loading the data asynchronously (by request), after the table was constructed and displayed?
Thanks in advance
Complete working example:
import javafx.application.Application;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Example extends Application
@Override
public void start(Stage primaryStage) throws Exception
primaryStage.setTitle("Test");
TableView<Person> table = new TableView<>();
ObservableList<Person> people = FXCollections.observableArrayList(
new Person("Very very very very long name", 100),
new Person("Not much shorter name", 44));
TableColumn nameColumn = new TableColumn("First Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<Person, String>("name"));
table.getColumns().add(nameColumn);
TableColumn ageColumn = new TableColumn("Age");
ageColumn.setCellValueFactory(new PropertyValueFactory<Person, Integer>("age"));
table.getColumns().add(ageColumn);
// table.setItems(people); /* Here it works */
BorderPane root = new BorderPane();
root.setCenter(table);
primaryStage.setScene(new Scene(root, 700, 400));
primaryStage.show();
table.setItems(people); /* Here it does not */
public static void main(String args)
launch(args);
public static class Person
private SimpleStringProperty name = new SimpleStringProperty();
private SimpleIntegerProperty age = new SimpleIntegerProperty();
public Person(String name, int age)
super();
this.name.set(name);
this.age.set(age);
public String getName()
return name.get();
public Integer getAge()
return age.get();
java javafx javafx-8 desktop-application
java javafx javafx-8 desktop-application
edited Nov 10 at 15:21
asked Nov 9 at 21:32
user683887
8101818
8101818
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11
|
show 1 more comment
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11
|
show 1 more comment
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53233518%2fjavafx-table-columns-fit-width-with-background-load%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UT IoKHr8S,SUXno5gj,YvZs1QaOhZrWb3lch,3KoG,xFLmoechAqrGmyH02Vlm0PBVjdx,4KdM,klESgXc5
Please post Minimal, Complete, and Verifiable example
– c0der
Nov 10 at 5:22
Examples updated to be complete and verifiable, as requested by @c0der
– user683887
Nov 10 at 14:41
To me complete and verifiable means copy-paste and run.
– c0der
Nov 10 at 14:46
Ok, here you are. Thanks for your time.
– user683887
Nov 10 at 15:21
See stackoverflow.com/questions/39248083/…
– c0der
Nov 10 at 16:11