Python: E1136:Value 'self.exchange.get_portfolio' is unsubscriptable
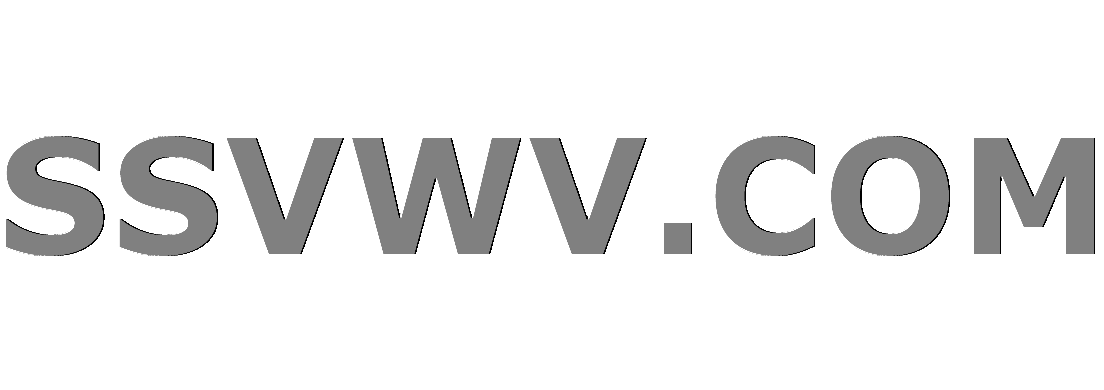
Multi tool use
up vote
0
down vote
favorite
def get_portfolio(self):
contracts = settings.CONTRACTS
portfolio =
for symbol in contracts:
position = self.bitmex.position(symbol=symbol)
instrument = self.bitmex.instrument(symbol=symbol)
if instrument['isQuanto']:
future_type = "Quanto"
elif instrument['isInverse']:
future_type = "Inverse"
elif not instrument['isQuanto'] and not instrument['isInverse']:
future_type = "Linear"
else:
raise NotImplementedError("Unknown future type; not quanto or inverse: %s" % instrument['symbol'])
if instrument['underlyingToSettleMultiplier'] is None:
multiplier = float(instrument['multiplier']) / float(instrument['quoteToSettleMultiplier'])
else:
multiplier = float(instrument['multiplier']) / float(instrument['underlyingToSettleMultiplier'])
portfolio[symbol] =
"currentQty": float(position['currentQty']),
"futureType": future_type,
"multiplier": multiplier,
"markPrice": float(instrument['markPrice']),
"spot": float(instrument['indicativeSettlePrice'])
return portfolio
qty = self.exchange.get_portfolio['currentQty']()
Does anybody know what I am doing wrong when I am calling the get_portfolio function because I keep getting this error message:
E1136:Value 'self.exchange.get_portfolio' is unsubscriptable
python algorithm bitmex
add a comment |
up vote
0
down vote
favorite
def get_portfolio(self):
contracts = settings.CONTRACTS
portfolio =
for symbol in contracts:
position = self.bitmex.position(symbol=symbol)
instrument = self.bitmex.instrument(symbol=symbol)
if instrument['isQuanto']:
future_type = "Quanto"
elif instrument['isInverse']:
future_type = "Inverse"
elif not instrument['isQuanto'] and not instrument['isInverse']:
future_type = "Linear"
else:
raise NotImplementedError("Unknown future type; not quanto or inverse: %s" % instrument['symbol'])
if instrument['underlyingToSettleMultiplier'] is None:
multiplier = float(instrument['multiplier']) / float(instrument['quoteToSettleMultiplier'])
else:
multiplier = float(instrument['multiplier']) / float(instrument['underlyingToSettleMultiplier'])
portfolio[symbol] =
"currentQty": float(position['currentQty']),
"futureType": future_type,
"multiplier": multiplier,
"markPrice": float(instrument['markPrice']),
"spot": float(instrument['indicativeSettlePrice'])
return portfolio
qty = self.exchange.get_portfolio['currentQty']()
Does anybody know what I am doing wrong when I am calling the get_portfolio function because I keep getting this error message:
E1136:Value 'self.exchange.get_portfolio' is unsubscriptable
python algorithm bitmex
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
def get_portfolio(self):
contracts = settings.CONTRACTS
portfolio =
for symbol in contracts:
position = self.bitmex.position(symbol=symbol)
instrument = self.bitmex.instrument(symbol=symbol)
if instrument['isQuanto']:
future_type = "Quanto"
elif instrument['isInverse']:
future_type = "Inverse"
elif not instrument['isQuanto'] and not instrument['isInverse']:
future_type = "Linear"
else:
raise NotImplementedError("Unknown future type; not quanto or inverse: %s" % instrument['symbol'])
if instrument['underlyingToSettleMultiplier'] is None:
multiplier = float(instrument['multiplier']) / float(instrument['quoteToSettleMultiplier'])
else:
multiplier = float(instrument['multiplier']) / float(instrument['underlyingToSettleMultiplier'])
portfolio[symbol] =
"currentQty": float(position['currentQty']),
"futureType": future_type,
"multiplier": multiplier,
"markPrice": float(instrument['markPrice']),
"spot": float(instrument['indicativeSettlePrice'])
return portfolio
qty = self.exchange.get_portfolio['currentQty']()
Does anybody know what I am doing wrong when I am calling the get_portfolio function because I keep getting this error message:
E1136:Value 'self.exchange.get_portfolio' is unsubscriptable
python algorithm bitmex
def get_portfolio(self):
contracts = settings.CONTRACTS
portfolio =
for symbol in contracts:
position = self.bitmex.position(symbol=symbol)
instrument = self.bitmex.instrument(symbol=symbol)
if instrument['isQuanto']:
future_type = "Quanto"
elif instrument['isInverse']:
future_type = "Inverse"
elif not instrument['isQuanto'] and not instrument['isInverse']:
future_type = "Linear"
else:
raise NotImplementedError("Unknown future type; not quanto or inverse: %s" % instrument['symbol'])
if instrument['underlyingToSettleMultiplier'] is None:
multiplier = float(instrument['multiplier']) / float(instrument['quoteToSettleMultiplier'])
else:
multiplier = float(instrument['multiplier']) / float(instrument['underlyingToSettleMultiplier'])
portfolio[symbol] =
"currentQty": float(position['currentQty']),
"futureType": future_type,
"multiplier": multiplier,
"markPrice": float(instrument['markPrice']),
"spot": float(instrument['indicativeSettlePrice'])
return portfolio
qty = self.exchange.get_portfolio['currentQty']()
Does anybody know what I am doing wrong when I am calling the get_portfolio function because I keep getting this error message:
E1136:Value 'self.exchange.get_portfolio' is unsubscriptable
python algorithm bitmex
python algorithm bitmex
edited Nov 10 at 16:48
shriek
2,01342651
2,01342651
asked Nov 10 at 16:07
Varun Reddy
1
1
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
You have a little mistake in the call:
self.exchange.get_portfolio
is a function, so you first have to call it and then you can reference the entries from the returned dict.
Oh I just saw, that you also have to insert your symbol
before:
qty = self.exchange.get_portfolio()[<YOUR_SYMBOL>]['currentQty']
If you don't know the symbols, you can use the keys
function which lists all the keys of your dict:
port = self.exchange.get_portfolio()
port_keys = port.keys()
qty = port[port_keys[<SOME KEY NUMBER>]]['currentQty']
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is akeys
function?
– user8408080
Nov 10 at 16:32
|
show 2 more comments
up vote
0
down vote
You should do it as follows:
qty = self.exchange.get_portfolio()
qty = qty[qty.keys()[0]]['currentQty']
or in a single row:
qty = self.exchange.get_portfolio()[self.exchange.get_portfolio().keys()[0]]['currentQty']
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You have a little mistake in the call:
self.exchange.get_portfolio
is a function, so you first have to call it and then you can reference the entries from the returned dict.
Oh I just saw, that you also have to insert your symbol
before:
qty = self.exchange.get_portfolio()[<YOUR_SYMBOL>]['currentQty']
If you don't know the symbols, you can use the keys
function which lists all the keys of your dict:
port = self.exchange.get_portfolio()
port_keys = port.keys()
qty = port[port_keys[<SOME KEY NUMBER>]]['currentQty']
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is akeys
function?
– user8408080
Nov 10 at 16:32
|
show 2 more comments
up vote
1
down vote
You have a little mistake in the call:
self.exchange.get_portfolio
is a function, so you first have to call it and then you can reference the entries from the returned dict.
Oh I just saw, that you also have to insert your symbol
before:
qty = self.exchange.get_portfolio()[<YOUR_SYMBOL>]['currentQty']
If you don't know the symbols, you can use the keys
function which lists all the keys of your dict:
port = self.exchange.get_portfolio()
port_keys = port.keys()
qty = port[port_keys[<SOME KEY NUMBER>]]['currentQty']
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is akeys
function?
– user8408080
Nov 10 at 16:32
|
show 2 more comments
up vote
1
down vote
up vote
1
down vote
You have a little mistake in the call:
self.exchange.get_portfolio
is a function, so you first have to call it and then you can reference the entries from the returned dict.
Oh I just saw, that you also have to insert your symbol
before:
qty = self.exchange.get_portfolio()[<YOUR_SYMBOL>]['currentQty']
If you don't know the symbols, you can use the keys
function which lists all the keys of your dict:
port = self.exchange.get_portfolio()
port_keys = port.keys()
qty = port[port_keys[<SOME KEY NUMBER>]]['currentQty']
You have a little mistake in the call:
self.exchange.get_portfolio
is a function, so you first have to call it and then you can reference the entries from the returned dict.
Oh I just saw, that you also have to insert your symbol
before:
qty = self.exchange.get_portfolio()[<YOUR_SYMBOL>]['currentQty']
If you don't know the symbols, you can use the keys
function which lists all the keys of your dict:
port = self.exchange.get_portfolio()
port_keys = port.keys()
qty = port[port_keys[<SOME KEY NUMBER>]]['currentQty']
edited Nov 10 at 16:36
answered Nov 10 at 16:11
user8408080
75228
75228
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is akeys
function?
– user8408080
Nov 10 at 16:32
|
show 2 more comments
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is akeys
function?
– user8408080
Nov 10 at 16:32
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
thank you!!, your help was much appreciated
– Varun Reddy
Nov 10 at 16:16
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
Don't forget to accept the answer, so others know it's solved ;)
– user8408080
Nov 10 at 16:18
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy you tested it and worked?
– Geeocode
Nov 10 at 16:19
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
@VarunReddy user8408080 He may not know the symbol's value, see my working answer below
– Geeocode
Nov 10 at 16:30
You think I should also point out, that there is a
keys
function?– user8408080
Nov 10 at 16:32
You think I should also point out, that there is a
keys
function?– user8408080
Nov 10 at 16:32
|
show 2 more comments
up vote
0
down vote
You should do it as follows:
qty = self.exchange.get_portfolio()
qty = qty[qty.keys()[0]]['currentQty']
or in a single row:
qty = self.exchange.get_portfolio()[self.exchange.get_portfolio().keys()[0]]['currentQty']
add a comment |
up vote
0
down vote
You should do it as follows:
qty = self.exchange.get_portfolio()
qty = qty[qty.keys()[0]]['currentQty']
or in a single row:
qty = self.exchange.get_portfolio()[self.exchange.get_portfolio().keys()[0]]['currentQty']
add a comment |
up vote
0
down vote
up vote
0
down vote
You should do it as follows:
qty = self.exchange.get_portfolio()
qty = qty[qty.keys()[0]]['currentQty']
or in a single row:
qty = self.exchange.get_portfolio()[self.exchange.get_portfolio().keys()[0]]['currentQty']
You should do it as follows:
qty = self.exchange.get_portfolio()
qty = qty[qty.keys()[0]]['currentQty']
or in a single row:
qty = self.exchange.get_portfolio()[self.exchange.get_portfolio().keys()[0]]['currentQty']
answered Nov 10 at 16:25
Geeocode
1,7701718
1,7701718
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240803%2fpython-e1136value-self-exchange-get-portfolio-is-unsubscriptable%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QPehTQ5dS1fw