Django custom user model: IntegrityError: null value in column “is_superuser” violates not-null constraint
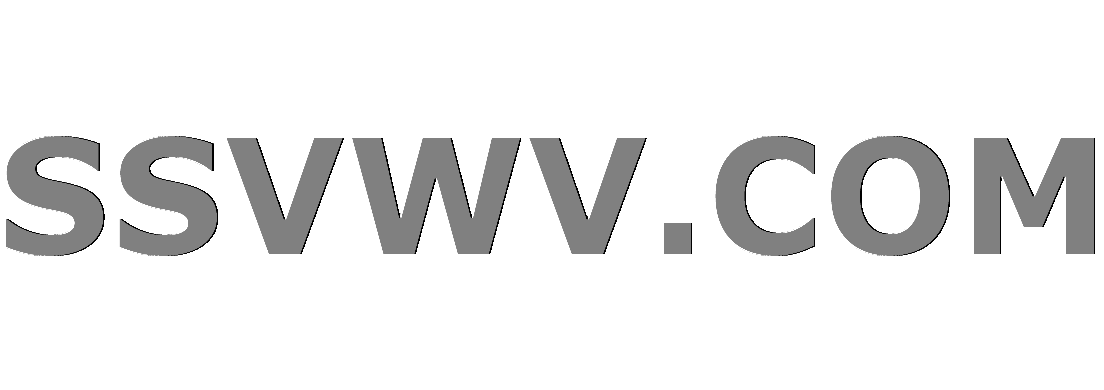
Multi tool use
I am trying to create a custom user model in Django and thereafter create RESTAPI as per django-rest-auth provides.
CustomUserModel and CustomUSerManager are defined as-
from django.contrib.auth.models import (
BaseUserManager, AbstractBaseUser
)
class UserManager(BaseUserManager):
use_in_migrations = True
def create_user(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password=None):
user = self.model(
email = self.normalize_email(email),
phone_no = phone_no,
password=password,
user_android_id = user_android_id,
user_fcm_token = user_fcm_token,
user_social_flag = user_social_flag,
user_fb_id = user_fb_id,
user_android_app_version = user_android_app_version,
name = name,
)
# user.is_staff = False
# user.is_superuser = True
user.set_password(password)
user.save(using=self._db)
return user
def create_staffuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_staff = True
user.is_admin = False
user.is_superuser = True
user.save(using=self._db)
return user
def create_superuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_admin = True
user.save(using=self._db)
return user
class User(AbstractBaseUser):
objects = UserManager()
name = models.CharField(max_length=100, blank=True, null=True)
email = models.EmailField(unique=True)
created_at = models.DateField(blank=True, null=True)
phone_no = models.BigIntegerField(blank=True, null=True)
user_android_id = models.CharField(max_length=255, blank=True, null=True)
user_fcm_token = models.CharField(max_length=255, blank=True, null=True)
user_social_flag = models.IntegerField(blank=True, null=True)
user_fb_id = models.CharField(max_length=255, blank=True, null=True)
user_android_app_version = models.CharField(max_length=25, blank=True, null=True)
USERNAME_FIELD = 'email'
def __str__(self):
return self.email
Custom View File:
from rest_auth.registration.views import RegisterView
from app.models import User
class CustomRegisterView(RegisterView):
queryset = User.objects.all()
In settings.py file I have set:
AUTH_USER_MODEL = 'app.User'
ACCOUNT_USER_MODEL_USERNAME_FIELD = None
ACCOUNT_AUTHENTICATION_METHOD = 'email'
ACCOUNT_EMAIL_VERIFICATION = 'none'
ACCOUNT_EMAIL_REQUIRED = True
ACCOUNT_UNIQUE_EMAIL = True
ACCOUNT_USERNAME_REQUIRED = False
ACCOUNT_USER_EMAIL_FIELD = 'email'
ACCOUNT_LOGOUT_ON_GET = True
REST_AUTH_SERIALIZERS =
"USER_DETAILS_SERIALIZER":
"app.serializers.CustomUserDetailsSerializer",
REST_AUTH_REGISTER_SERIALIZERS =
"REGISTER_SERIALIZER":
"app.serializers.CustomRegisterSerializer",
I have set the urls in url file.
re_path(r'^registration/$', views.CustomRegisterView.as_view())
When I run the /registration/ url, and fill all the details I get error:
django.db.utils.IntegrityError: null value in column "is_superuser" violates not-null constraint
DETAIL: Failing row contains (8, pbkdf2_sha256$120000$VpGItoUPHoJ9$yBQB2PqRDiqd4SQ2cDZX/wzaV3yFSf..., null, null, null, null, null, null, null, null, null, custom@user.com, null, null, null, null, null, null, null).
What am I missing here?
UPDATE 1:
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
UPDATE 2:
CustomRegisterSerializer class:-
class CustomRegisterSerializer(RegisterSerializer):
email = serializers.EmailField()
password1 = serializers.CharField(write_only=True)
name = serializers.CharField()
phone_no = serializers.IntegerField()
user_android_id = serializers.CharField()
user_fcm_token = serializers.CharField(required=True)
user_social_flag = serializers.IntegerField()
user_fb_id = serializers.CharField(required=True)
user_android_app_version = serializers.CharField()
class Meta:
model = User
fields = ('email', 'name', 'phone_no', 'user_android_id', 'user_fcm_token',
'user_social_flag', 'user_fb_id', 'user_android_app_version')
def get_cleaned_data(self):
super(CustomRegisterSerializer, self).get_cleaned_data()
return
'password1': self.validated_data.get('password1', ''),
'email': self.validated_data.get('email', ''),
'phone_no': self.validated_data.get('phone_no'),
'name': self.validated_data.get('name'),
'user_android_id': self.validated_data.get('user_android_id'),
'user_fcm_token': self.validated_data.get('user_fcm_token'),
'user_social_flag': self.validated_data.get('user_social_flag'),
'user_fb_id': self.validated_data.get('user_fb_id'),
'user_android_app_version': self.validated_data.get('user_android_app_version'),
django django-models django-rest-framework django-rest-auth django-custom-user
add a comment |
I am trying to create a custom user model in Django and thereafter create RESTAPI as per django-rest-auth provides.
CustomUserModel and CustomUSerManager are defined as-
from django.contrib.auth.models import (
BaseUserManager, AbstractBaseUser
)
class UserManager(BaseUserManager):
use_in_migrations = True
def create_user(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password=None):
user = self.model(
email = self.normalize_email(email),
phone_no = phone_no,
password=password,
user_android_id = user_android_id,
user_fcm_token = user_fcm_token,
user_social_flag = user_social_flag,
user_fb_id = user_fb_id,
user_android_app_version = user_android_app_version,
name = name,
)
# user.is_staff = False
# user.is_superuser = True
user.set_password(password)
user.save(using=self._db)
return user
def create_staffuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_staff = True
user.is_admin = False
user.is_superuser = True
user.save(using=self._db)
return user
def create_superuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_admin = True
user.save(using=self._db)
return user
class User(AbstractBaseUser):
objects = UserManager()
name = models.CharField(max_length=100, blank=True, null=True)
email = models.EmailField(unique=True)
created_at = models.DateField(blank=True, null=True)
phone_no = models.BigIntegerField(blank=True, null=True)
user_android_id = models.CharField(max_length=255, blank=True, null=True)
user_fcm_token = models.CharField(max_length=255, blank=True, null=True)
user_social_flag = models.IntegerField(blank=True, null=True)
user_fb_id = models.CharField(max_length=255, blank=True, null=True)
user_android_app_version = models.CharField(max_length=25, blank=True, null=True)
USERNAME_FIELD = 'email'
def __str__(self):
return self.email
Custom View File:
from rest_auth.registration.views import RegisterView
from app.models import User
class CustomRegisterView(RegisterView):
queryset = User.objects.all()
In settings.py file I have set:
AUTH_USER_MODEL = 'app.User'
ACCOUNT_USER_MODEL_USERNAME_FIELD = None
ACCOUNT_AUTHENTICATION_METHOD = 'email'
ACCOUNT_EMAIL_VERIFICATION = 'none'
ACCOUNT_EMAIL_REQUIRED = True
ACCOUNT_UNIQUE_EMAIL = True
ACCOUNT_USERNAME_REQUIRED = False
ACCOUNT_USER_EMAIL_FIELD = 'email'
ACCOUNT_LOGOUT_ON_GET = True
REST_AUTH_SERIALIZERS =
"USER_DETAILS_SERIALIZER":
"app.serializers.CustomUserDetailsSerializer",
REST_AUTH_REGISTER_SERIALIZERS =
"REGISTER_SERIALIZER":
"app.serializers.CustomRegisterSerializer",
I have set the urls in url file.
re_path(r'^registration/$', views.CustomRegisterView.as_view())
When I run the /registration/ url, and fill all the details I get error:
django.db.utils.IntegrityError: null value in column "is_superuser" violates not-null constraint
DETAIL: Failing row contains (8, pbkdf2_sha256$120000$VpGItoUPHoJ9$yBQB2PqRDiqd4SQ2cDZX/wzaV3yFSf..., null, null, null, null, null, null, null, null, null, custom@user.com, null, null, null, null, null, null, null).
What am I missing here?
UPDATE 1:
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
UPDATE 2:
CustomRegisterSerializer class:-
class CustomRegisterSerializer(RegisterSerializer):
email = serializers.EmailField()
password1 = serializers.CharField(write_only=True)
name = serializers.CharField()
phone_no = serializers.IntegerField()
user_android_id = serializers.CharField()
user_fcm_token = serializers.CharField(required=True)
user_social_flag = serializers.IntegerField()
user_fb_id = serializers.CharField(required=True)
user_android_app_version = serializers.CharField()
class Meta:
model = User
fields = ('email', 'name', 'phone_no', 'user_android_id', 'user_fcm_token',
'user_social_flag', 'user_fb_id', 'user_android_app_version')
def get_cleaned_data(self):
super(CustomRegisterSerializer, self).get_cleaned_data()
return
'password1': self.validated_data.get('password1', ''),
'email': self.validated_data.get('email', ''),
'phone_no': self.validated_data.get('phone_no'),
'name': self.validated_data.get('name'),
'user_android_id': self.validated_data.get('user_android_id'),
'user_fcm_token': self.validated_data.get('user_fcm_token'),
'user_social_flag': self.validated_data.get('user_social_flag'),
'user_fb_id': self.validated_data.get('user_fb_id'),
'user_android_app_version': self.validated_data.get('user_android_app_version'),
django django-models django-rest-framework django-rest-auth django-custom-user
add a comment |
I am trying to create a custom user model in Django and thereafter create RESTAPI as per django-rest-auth provides.
CustomUserModel and CustomUSerManager are defined as-
from django.contrib.auth.models import (
BaseUserManager, AbstractBaseUser
)
class UserManager(BaseUserManager):
use_in_migrations = True
def create_user(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password=None):
user = self.model(
email = self.normalize_email(email),
phone_no = phone_no,
password=password,
user_android_id = user_android_id,
user_fcm_token = user_fcm_token,
user_social_flag = user_social_flag,
user_fb_id = user_fb_id,
user_android_app_version = user_android_app_version,
name = name,
)
# user.is_staff = False
# user.is_superuser = True
user.set_password(password)
user.save(using=self._db)
return user
def create_staffuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_staff = True
user.is_admin = False
user.is_superuser = True
user.save(using=self._db)
return user
def create_superuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_admin = True
user.save(using=self._db)
return user
class User(AbstractBaseUser):
objects = UserManager()
name = models.CharField(max_length=100, blank=True, null=True)
email = models.EmailField(unique=True)
created_at = models.DateField(blank=True, null=True)
phone_no = models.BigIntegerField(blank=True, null=True)
user_android_id = models.CharField(max_length=255, blank=True, null=True)
user_fcm_token = models.CharField(max_length=255, blank=True, null=True)
user_social_flag = models.IntegerField(blank=True, null=True)
user_fb_id = models.CharField(max_length=255, blank=True, null=True)
user_android_app_version = models.CharField(max_length=25, blank=True, null=True)
USERNAME_FIELD = 'email'
def __str__(self):
return self.email
Custom View File:
from rest_auth.registration.views import RegisterView
from app.models import User
class CustomRegisterView(RegisterView):
queryset = User.objects.all()
In settings.py file I have set:
AUTH_USER_MODEL = 'app.User'
ACCOUNT_USER_MODEL_USERNAME_FIELD = None
ACCOUNT_AUTHENTICATION_METHOD = 'email'
ACCOUNT_EMAIL_VERIFICATION = 'none'
ACCOUNT_EMAIL_REQUIRED = True
ACCOUNT_UNIQUE_EMAIL = True
ACCOUNT_USERNAME_REQUIRED = False
ACCOUNT_USER_EMAIL_FIELD = 'email'
ACCOUNT_LOGOUT_ON_GET = True
REST_AUTH_SERIALIZERS =
"USER_DETAILS_SERIALIZER":
"app.serializers.CustomUserDetailsSerializer",
REST_AUTH_REGISTER_SERIALIZERS =
"REGISTER_SERIALIZER":
"app.serializers.CustomRegisterSerializer",
I have set the urls in url file.
re_path(r'^registration/$', views.CustomRegisterView.as_view())
When I run the /registration/ url, and fill all the details I get error:
django.db.utils.IntegrityError: null value in column "is_superuser" violates not-null constraint
DETAIL: Failing row contains (8, pbkdf2_sha256$120000$VpGItoUPHoJ9$yBQB2PqRDiqd4SQ2cDZX/wzaV3yFSf..., null, null, null, null, null, null, null, null, null, custom@user.com, null, null, null, null, null, null, null).
What am I missing here?
UPDATE 1:
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
UPDATE 2:
CustomRegisterSerializer class:-
class CustomRegisterSerializer(RegisterSerializer):
email = serializers.EmailField()
password1 = serializers.CharField(write_only=True)
name = serializers.CharField()
phone_no = serializers.IntegerField()
user_android_id = serializers.CharField()
user_fcm_token = serializers.CharField(required=True)
user_social_flag = serializers.IntegerField()
user_fb_id = serializers.CharField(required=True)
user_android_app_version = serializers.CharField()
class Meta:
model = User
fields = ('email', 'name', 'phone_no', 'user_android_id', 'user_fcm_token',
'user_social_flag', 'user_fb_id', 'user_android_app_version')
def get_cleaned_data(self):
super(CustomRegisterSerializer, self).get_cleaned_data()
return
'password1': self.validated_data.get('password1', ''),
'email': self.validated_data.get('email', ''),
'phone_no': self.validated_data.get('phone_no'),
'name': self.validated_data.get('name'),
'user_android_id': self.validated_data.get('user_android_id'),
'user_fcm_token': self.validated_data.get('user_fcm_token'),
'user_social_flag': self.validated_data.get('user_social_flag'),
'user_fb_id': self.validated_data.get('user_fb_id'),
'user_android_app_version': self.validated_data.get('user_android_app_version'),
django django-models django-rest-framework django-rest-auth django-custom-user
I am trying to create a custom user model in Django and thereafter create RESTAPI as per django-rest-auth provides.
CustomUserModel and CustomUSerManager are defined as-
from django.contrib.auth.models import (
BaseUserManager, AbstractBaseUser
)
class UserManager(BaseUserManager):
use_in_migrations = True
def create_user(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password=None):
user = self.model(
email = self.normalize_email(email),
phone_no = phone_no,
password=password,
user_android_id = user_android_id,
user_fcm_token = user_fcm_token,
user_social_flag = user_social_flag,
user_fb_id = user_fb_id,
user_android_app_version = user_android_app_version,
name = name,
)
# user.is_staff = False
# user.is_superuser = True
user.set_password(password)
user.save(using=self._db)
return user
def create_staffuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_staff = True
user.is_admin = False
user.is_superuser = True
user.save(using=self._db)
return user
def create_superuser(self, email, name, phone_no, user_android_id, user_fcm_token,
user_social_flag, user_fb_id, user_android_app_version, password):
user = self.create_user(
email,
password=password,
phone_no=phone_no,
user_android_id=user_android_id,
user_fcm_token=user_fcm_token,
user_social_flag=user_social_flag,
user_fb_id=user_fb_id,
user_android_app_version=user_android_app_version,
name=name,
)
user.is_admin = True
user.save(using=self._db)
return user
class User(AbstractBaseUser):
objects = UserManager()
name = models.CharField(max_length=100, blank=True, null=True)
email = models.EmailField(unique=True)
created_at = models.DateField(blank=True, null=True)
phone_no = models.BigIntegerField(blank=True, null=True)
user_android_id = models.CharField(max_length=255, blank=True, null=True)
user_fcm_token = models.CharField(max_length=255, blank=True, null=True)
user_social_flag = models.IntegerField(blank=True, null=True)
user_fb_id = models.CharField(max_length=255, blank=True, null=True)
user_android_app_version = models.CharField(max_length=25, blank=True, null=True)
USERNAME_FIELD = 'email'
def __str__(self):
return self.email
Custom View File:
from rest_auth.registration.views import RegisterView
from app.models import User
class CustomRegisterView(RegisterView):
queryset = User.objects.all()
In settings.py file I have set:
AUTH_USER_MODEL = 'app.User'
ACCOUNT_USER_MODEL_USERNAME_FIELD = None
ACCOUNT_AUTHENTICATION_METHOD = 'email'
ACCOUNT_EMAIL_VERIFICATION = 'none'
ACCOUNT_EMAIL_REQUIRED = True
ACCOUNT_UNIQUE_EMAIL = True
ACCOUNT_USERNAME_REQUIRED = False
ACCOUNT_USER_EMAIL_FIELD = 'email'
ACCOUNT_LOGOUT_ON_GET = True
REST_AUTH_SERIALIZERS =
"USER_DETAILS_SERIALIZER":
"app.serializers.CustomUserDetailsSerializer",
REST_AUTH_REGISTER_SERIALIZERS =
"REGISTER_SERIALIZER":
"app.serializers.CustomRegisterSerializer",
I have set the urls in url file.
re_path(r'^registration/$', views.CustomRegisterView.as_view())
When I run the /registration/ url, and fill all the details I get error:
django.db.utils.IntegrityError: null value in column "is_superuser" violates not-null constraint
DETAIL: Failing row contains (8, pbkdf2_sha256$120000$VpGItoUPHoJ9$yBQB2PqRDiqd4SQ2cDZX/wzaV3yFSf..., null, null, null, null, null, null, null, null, null, custom@user.com, null, null, null, null, null, null, null).
What am I missing here?
UPDATE 1:
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
UPDATE 2:
CustomRegisterSerializer class:-
class CustomRegisterSerializer(RegisterSerializer):
email = serializers.EmailField()
password1 = serializers.CharField(write_only=True)
name = serializers.CharField()
phone_no = serializers.IntegerField()
user_android_id = serializers.CharField()
user_fcm_token = serializers.CharField(required=True)
user_social_flag = serializers.IntegerField()
user_fb_id = serializers.CharField(required=True)
user_android_app_version = serializers.CharField()
class Meta:
model = User
fields = ('email', 'name', 'phone_no', 'user_android_id', 'user_fcm_token',
'user_social_flag', 'user_fb_id', 'user_android_app_version')
def get_cleaned_data(self):
super(CustomRegisterSerializer, self).get_cleaned_data()
return
'password1': self.validated_data.get('password1', ''),
'email': self.validated_data.get('email', ''),
'phone_no': self.validated_data.get('phone_no'),
'name': self.validated_data.get('name'),
'user_android_id': self.validated_data.get('user_android_id'),
'user_fcm_token': self.validated_data.get('user_fcm_token'),
'user_social_flag': self.validated_data.get('user_social_flag'),
'user_fb_id': self.validated_data.get('user_fb_id'),
'user_android_app_version': self.validated_data.get('user_android_app_version'),
django django-models django-rest-framework django-rest-auth django-custom-user
django django-models django-rest-framework django-rest-auth django-custom-user
edited Nov 15 '18 at 7:17
Reema Parakh
asked Nov 14 '18 at 12:47


Reema ParakhReema Parakh
522522
522522
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In create_user
you've commented is_staff
and is_superuser
. Uncomment and set them to False
.
EDIT:
About the fields not getting into database - is_staff
and is_superuser
are not defined in your user model and are also not defined in the AbstractBaseUser
. Which is strange, because in this case you shouldn't have gotten that exception in the first place.
Are you just now starting your project or have you ran migrations before swapping the user model?
If you've started with the default user model from Django, you'll need some more work to do. Hopefully you don't have any real users yet and you could do it quick.
Take a careful look at the topic in Django docs.
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300608%2fdjango-custom-user-model-integrityerror-null-value-in-column-is-superuser-vi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In create_user
you've commented is_staff
and is_superuser
. Uncomment and set them to False
.
EDIT:
About the fields not getting into database - is_staff
and is_superuser
are not defined in your user model and are also not defined in the AbstractBaseUser
. Which is strange, because in this case you shouldn't have gotten that exception in the first place.
Are you just now starting your project or have you ran migrations before swapping the user model?
If you've started with the default user model from Django, you'll need some more work to do. Hopefully you don't have any real users yet and you could do it quick.
Take a careful look at the topic in Django docs.
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
|
show 1 more comment
In create_user
you've commented is_staff
and is_superuser
. Uncomment and set them to False
.
EDIT:
About the fields not getting into database - is_staff
and is_superuser
are not defined in your user model and are also not defined in the AbstractBaseUser
. Which is strange, because in this case you shouldn't have gotten that exception in the first place.
Are you just now starting your project or have you ran migrations before swapping the user model?
If you've started with the default user model from Django, you'll need some more work to do. Hopefully you don't have any real users yet and you could do it quick.
Take a careful look at the topic in Django docs.
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
|
show 1 more comment
In create_user
you've commented is_staff
and is_superuser
. Uncomment and set them to False
.
EDIT:
About the fields not getting into database - is_staff
and is_superuser
are not defined in your user model and are also not defined in the AbstractBaseUser
. Which is strange, because in this case you shouldn't have gotten that exception in the first place.
Are you just now starting your project or have you ran migrations before swapping the user model?
If you've started with the default user model from Django, you'll need some more work to do. Hopefully you don't have any real users yet and you could do it quick.
Take a careful look at the topic in Django docs.
In create_user
you've commented is_staff
and is_superuser
. Uncomment and set them to False
.
EDIT:
About the fields not getting into database - is_staff
and is_superuser
are not defined in your user model and are also not defined in the AbstractBaseUser
. Which is strange, because in this case you shouldn't have gotten that exception in the first place.
Are you just now starting your project or have you ran migrations before swapping the user model?
If you've started with the default user model from Django, you'll need some more work to do. Hopefully you don't have any real users yet and you could do it quick.
Take a careful look at the topic in Django docs.
edited Nov 15 '18 at 8:05
answered Nov 14 '18 at 12:59


4140tm4140tm
1,136714
1,136714
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
|
show 1 more comment
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
Great. +1. It helped. Thanks.
– Reema Parakh
Nov 14 '18 at 13:11
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
One more thing, Can I customize RegisterAPIView and LoginAPIView response, so that the same is used for android and ios.
– Reema Parakh
Nov 15 '18 at 4:07
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
While checking the database records, I found that only email, password, and last_login fields are only inserted and rest all are null. What could be reason?
– Reema Parakh
Nov 15 '18 at 4:22
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
Okay, I have updated the model, and now custom fields got saved. Another Point here is: When we register using API, it returns the a key value. But I want to customize the response with additional fields such as primary key value and a result_flag which will be either 0 or 1. How can we achieve this?
– Reema Parakh
Nov 15 '18 at 8:13
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
I think this is for separate question.
– 4140tm
Nov 15 '18 at 8:21
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300608%2fdjango-custom-user-model-integrityerror-null-value-in-column-is-superuser-vi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DEw8iG 5s,6k Ss88i2KClgjUnze7 fc2RxaNN,r4Int,ttEQy zwSkYEJXYgQO0VMtJY x