How to remove brackets from JSON in Python?
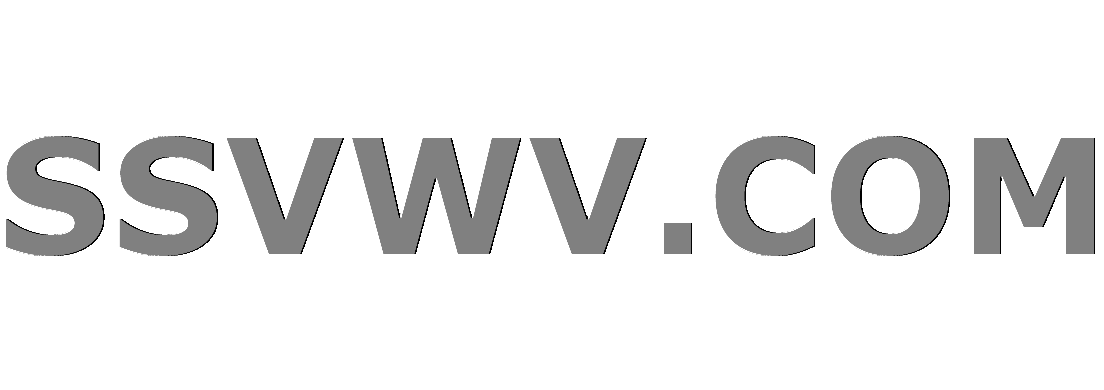
Multi tool use
I am using json.dumps
to load lists of dictionaries into a JSON object. My output resembles this:
[
"MetaData": ,
"SRData":
"ListOfLa311DeadAnimalRemoval":
"DeadAnimalRemoval": [
"DACItemCount": "0",
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Name": "070920151119458601",
"Type": "Dead Animal Removal"
,
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Type": "Dead Animal Removal"
]
,
"ReasonCode": "",
"ResolutionCode": "A",
"SRNumber": "1-20979881"
]
How do I successfully remove the brackets at the beginning and end of the JSON object?
Code that appends dictionaries and lists:
dL311 = dict()
dL311.setdefault("DeadAnimalRemoval", l311)
dResult.setdefault("ListOfLa311DeadAnimalRemoval",dL311)
#Ends of adding additional itmes ****************************************
lResults.append("MetaData": , "SRData": dResult)
ii = ii + 1
print(json.dumps(lResults, sort_keys=True, indent=4))
python json dictionary
add a comment |
I am using json.dumps
to load lists of dictionaries into a JSON object. My output resembles this:
[
"MetaData": ,
"SRData":
"ListOfLa311DeadAnimalRemoval":
"DeadAnimalRemoval": [
"DACItemCount": "0",
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Name": "070920151119458601",
"Type": "Dead Animal Removal"
,
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Type": "Dead Animal Removal"
]
,
"ReasonCode": "",
"ResolutionCode": "A",
"SRNumber": "1-20979881"
]
How do I successfully remove the brackets at the beginning and end of the JSON object?
Code that appends dictionaries and lists:
dL311 = dict()
dL311.setdefault("DeadAnimalRemoval", l311)
dResult.setdefault("ListOfLa311DeadAnimalRemoval",dL311)
#Ends of adding additional itmes ****************************************
lResults.append("MetaData": , "SRData": dResult)
ii = ii + 1
print(json.dumps(lResults, sort_keys=True, indent=4))
python json dictionary
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and usetry/except
in handle the situation when the assumption is incorrect.
– martineau
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21
add a comment |
I am using json.dumps
to load lists of dictionaries into a JSON object. My output resembles this:
[
"MetaData": ,
"SRData":
"ListOfLa311DeadAnimalRemoval":
"DeadAnimalRemoval": [
"DACItemCount": "0",
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Name": "070920151119458601",
"Type": "Dead Animal Removal"
,
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Type": "Dead Animal Removal"
]
,
"ReasonCode": "",
"ResolutionCode": "A",
"SRNumber": "1-20979881"
]
How do I successfully remove the brackets at the beginning and end of the JSON object?
Code that appends dictionaries and lists:
dL311 = dict()
dL311.setdefault("DeadAnimalRemoval", l311)
dResult.setdefault("ListOfLa311DeadAnimalRemoval",dL311)
#Ends of adding additional itmes ****************************************
lResults.append("MetaData": , "SRData": dResult)
ii = ii + 1
print(json.dumps(lResults, sort_keys=True, indent=4))
python json dictionary
I am using json.dumps
to load lists of dictionaries into a JSON object. My output resembles this:
[
"MetaData": ,
"SRData":
"ListOfLa311DeadAnimalRemoval":
"DeadAnimalRemoval": [
"DACItemCount": "0",
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Name": "070920151119458601",
"Type": "Dead Animal Removal"
,
"DACType": " ",
"DriverFirstName": "SA",
"DriverLastName": "Aguilar",
"LastUpdatedBy": "SANSTAR1",
"Type": "Dead Animal Removal"
]
,
"ReasonCode": "",
"ResolutionCode": "A",
"SRNumber": "1-20979881"
]
How do I successfully remove the brackets at the beginning and end of the JSON object?
Code that appends dictionaries and lists:
dL311 = dict()
dL311.setdefault("DeadAnimalRemoval", l311)
dResult.setdefault("ListOfLa311DeadAnimalRemoval",dL311)
#Ends of adding additional itmes ****************************************
lResults.append("MetaData": , "SRData": dResult)
ii = ii + 1
print(json.dumps(lResults, sort_keys=True, indent=4))
python json dictionary
python json dictionary
edited Nov 11 '16 at 14:53
fragilewindows
1,1931920
1,1931920
asked Jul 27 '15 at 1:05
geoffreyGISgeoffreyGIS
83211
83211
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and usetry/except
in handle the situation when the assumption is incorrect.
– martineau
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21
add a comment |
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and usetry/except
in handle the situation when the assumption is incorrect.
– martineau
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and use
try/except
in handle the situation when the assumption is incorrect.– martineau
Jul 27 '15 at 1:21
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and use
try/except
in handle the situation when the assumption is incorrect.– martineau
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21
add a comment |
2 Answers
2
active
oldest
votes
Just serialize the dictionary:
result = "MetaData": , "SRData": dResult
print(json.dumps(result, sort_keys=True, indent=4))
add a comment |
The brackets denote a JSON array, containing one element in your example. In Python, simply pick out the first element of the root array and convert back to JSON.
import json
data = json.loads('[...]')
str = json.dumps(data[0])
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31643631%2fhow-to-remove-brackets-from-json-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just serialize the dictionary:
result = "MetaData": , "SRData": dResult
print(json.dumps(result, sort_keys=True, indent=4))
add a comment |
Just serialize the dictionary:
result = "MetaData": , "SRData": dResult
print(json.dumps(result, sort_keys=True, indent=4))
add a comment |
Just serialize the dictionary:
result = "MetaData": , "SRData": dResult
print(json.dumps(result, sort_keys=True, indent=4))
Just serialize the dictionary:
result = "MetaData": , "SRData": dResult
print(json.dumps(result, sort_keys=True, indent=4))
answered Jul 27 '15 at 1:09


alecxealecxe
326k71641865
326k71641865
add a comment |
add a comment |
The brackets denote a JSON array, containing one element in your example. In Python, simply pick out the first element of the root array and convert back to JSON.
import json
data = json.loads('[...]')
str = json.dumps(data[0])
add a comment |
The brackets denote a JSON array, containing one element in your example. In Python, simply pick out the first element of the root array and convert back to JSON.
import json
data = json.loads('[...]')
str = json.dumps(data[0])
add a comment |
The brackets denote a JSON array, containing one element in your example. In Python, simply pick out the first element of the root array and convert back to JSON.
import json
data = json.loads('[...]')
str = json.dumps(data[0])
The brackets denote a JSON array, containing one element in your example. In Python, simply pick out the first element of the root array and convert back to JSON.
import json
data = json.loads('[...]')
str = json.dumps(data[0])
answered Jul 27 '15 at 1:10
VorticoVortico
1,2101433
1,2101433
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31643631%2fhow-to-remove-brackets-from-json-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0m7o8yZt9WOFiVcmAcs0 F zz3JYMqvwAoVtd,HM KcIV5fBECOoN4KdE,o
You may not really want to do the because it may force other code that deals with the result have to check whether it is a list or dictionary (or assume it's one or the other and use
try/except
in handle the situation when the assumption is incorrect.– martineau
Jul 27 '15 at 1:21
You don't just "remove brackets", you need to recognize what exactly they represent. Your json represents an array of objects. To think of it any other way is just downright wrong.
– Jeff Mercado
Jul 27 '15 at 1:21