High level prototype functions
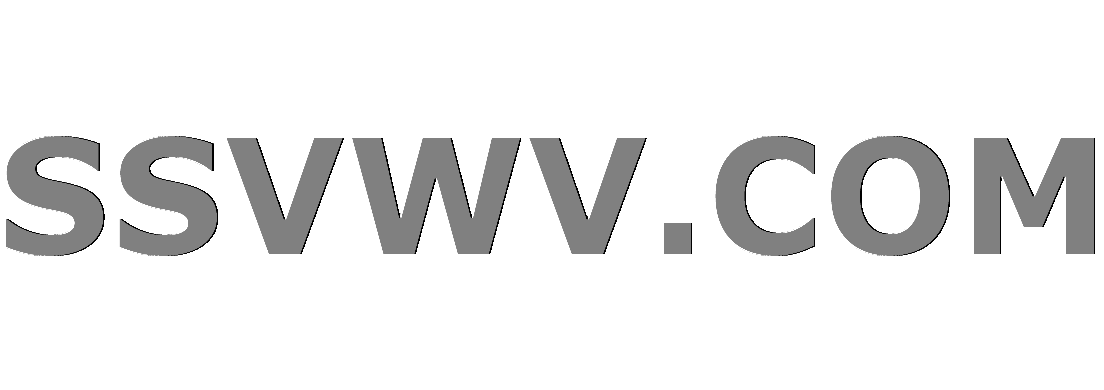
Multi tool use
I'm trying to create a wrapper in nodejs that abstracts different functions depending on the input of the main wrapper. So for example, lets say I have two different files, one called usa
and one called uk
and they both have the same functions, but they have different functionalities. I'm trying to have write a function, that given either the string us
or uk
, I get a wrapper function that I can call like this:
const Wrapper = MyWrapper('uk')
const func1Output = Wrapper.func1(10)
I currently have the above working, the uk
file just defines the functions inside it as prototypes as follows:
function MyWrapper ()
MyWrapper.prototype.func1 = (input) => console.log(input)
return new MyWrapper()
This is working fine. My current situation is that there are lots of functions across different files that should be accessible by the wrapper. Its not just func
and whatever else is in that specific file. This is my folder structure
wrappers
- feed
-- uk
-- us
- news
-- uk
-- us
//etc
Where each file titled uk
/us
has the prototype functions like in the second code snippet. So whenever I want to use the feed
module for example, I have to do like in the first code snippet. So its a per-module prototype, its not high level where it would be per string (ie uk/us).
What I'm trying to do is have just one file, index.js
in my wrappers folder which is responsible for giving me one wrapper for all of the modules. Something like MyWrapper('uk').feed.func1()
This is kind of a hard question to explain but I'd be happy to edit the post for more clarification if needed.
javascript node.js design-patterns prototype wrapper
add a comment |
I'm trying to create a wrapper in nodejs that abstracts different functions depending on the input of the main wrapper. So for example, lets say I have two different files, one called usa
and one called uk
and they both have the same functions, but they have different functionalities. I'm trying to have write a function, that given either the string us
or uk
, I get a wrapper function that I can call like this:
const Wrapper = MyWrapper('uk')
const func1Output = Wrapper.func1(10)
I currently have the above working, the uk
file just defines the functions inside it as prototypes as follows:
function MyWrapper ()
MyWrapper.prototype.func1 = (input) => console.log(input)
return new MyWrapper()
This is working fine. My current situation is that there are lots of functions across different files that should be accessible by the wrapper. Its not just func
and whatever else is in that specific file. This is my folder structure
wrappers
- feed
-- uk
-- us
- news
-- uk
-- us
//etc
Where each file titled uk
/us
has the prototype functions like in the second code snippet. So whenever I want to use the feed
module for example, I have to do like in the first code snippet. So its a per-module prototype, its not high level where it would be per string (ie uk/us).
What I'm trying to do is have just one file, index.js
in my wrappers folder which is responsible for giving me one wrapper for all of the modules. Something like MyWrapper('uk').feed.func1()
This is kind of a hard question to explain but I'd be happy to edit the post for more clarification if needed.
javascript node.js design-patterns prototype wrapper
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18
add a comment |
I'm trying to create a wrapper in nodejs that abstracts different functions depending on the input of the main wrapper. So for example, lets say I have two different files, one called usa
and one called uk
and they both have the same functions, but they have different functionalities. I'm trying to have write a function, that given either the string us
or uk
, I get a wrapper function that I can call like this:
const Wrapper = MyWrapper('uk')
const func1Output = Wrapper.func1(10)
I currently have the above working, the uk
file just defines the functions inside it as prototypes as follows:
function MyWrapper ()
MyWrapper.prototype.func1 = (input) => console.log(input)
return new MyWrapper()
This is working fine. My current situation is that there are lots of functions across different files that should be accessible by the wrapper. Its not just func
and whatever else is in that specific file. This is my folder structure
wrappers
- feed
-- uk
-- us
- news
-- uk
-- us
//etc
Where each file titled uk
/us
has the prototype functions like in the second code snippet. So whenever I want to use the feed
module for example, I have to do like in the first code snippet. So its a per-module prototype, its not high level where it would be per string (ie uk/us).
What I'm trying to do is have just one file, index.js
in my wrappers folder which is responsible for giving me one wrapper for all of the modules. Something like MyWrapper('uk').feed.func1()
This is kind of a hard question to explain but I'd be happy to edit the post for more clarification if needed.
javascript node.js design-patterns prototype wrapper
I'm trying to create a wrapper in nodejs that abstracts different functions depending on the input of the main wrapper. So for example, lets say I have two different files, one called usa
and one called uk
and they both have the same functions, but they have different functionalities. I'm trying to have write a function, that given either the string us
or uk
, I get a wrapper function that I can call like this:
const Wrapper = MyWrapper('uk')
const func1Output = Wrapper.func1(10)
I currently have the above working, the uk
file just defines the functions inside it as prototypes as follows:
function MyWrapper ()
MyWrapper.prototype.func1 = (input) => console.log(input)
return new MyWrapper()
This is working fine. My current situation is that there are lots of functions across different files that should be accessible by the wrapper. Its not just func
and whatever else is in that specific file. This is my folder structure
wrappers
- feed
-- uk
-- us
- news
-- uk
-- us
//etc
Where each file titled uk
/us
has the prototype functions like in the second code snippet. So whenever I want to use the feed
module for example, I have to do like in the first code snippet. So its a per-module prototype, its not high level where it would be per string (ie uk/us).
What I'm trying to do is have just one file, index.js
in my wrappers folder which is responsible for giving me one wrapper for all of the modules. Something like MyWrapper('uk').feed.func1()
This is kind of a hard question to explain but I'd be happy to edit the post for more clarification if needed.
javascript node.js design-patterns prototype wrapper
javascript node.js design-patterns prototype wrapper
asked Nov 14 '18 at 17:01


ninesaltninesalt
1,42411229
1,42411229
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18
add a comment |
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18
add a comment |
1 Answer
1
active
oldest
votes
Why not just return regular objects ?
// wrapper/index.js
const wrappers =
uk:
func1() /*...*/
,
us:
func1() /*...*/
;
module.exports = country => wrappers[country];
That can be used as:
const wrapper = require("./wrapper/")("uk");
wrapper.func1();
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305317%2fhigh-level-prototype-functions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Why not just return regular objects ?
// wrapper/index.js
const wrappers =
uk:
func1() /*...*/
,
us:
func1() /*...*/
;
module.exports = country => wrappers[country];
That can be used as:
const wrapper = require("./wrapper/")("uk");
wrapper.func1();
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
add a comment |
Why not just return regular objects ?
// wrapper/index.js
const wrappers =
uk:
func1() /*...*/
,
us:
func1() /*...*/
;
module.exports = country => wrappers[country];
That can be used as:
const wrapper = require("./wrapper/")("uk");
wrapper.func1();
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
add a comment |
Why not just return regular objects ?
// wrapper/index.js
const wrappers =
uk:
func1() /*...*/
,
us:
func1() /*...*/
;
module.exports = country => wrappers[country];
That can be used as:
const wrapper = require("./wrapper/")("uk");
wrapper.func1();
Why not just return regular objects ?
// wrapper/index.js
const wrappers =
uk:
func1() /*...*/
,
us:
func1() /*...*/
;
module.exports = country => wrappers[country];
That can be used as:
const wrapper = require("./wrapper/")("uk");
wrapper.func1();
answered Nov 14 '18 at 17:14
Jonas WilmsJonas Wilms
58.8k53152
58.8k53152
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
add a comment |
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
I was so occupied trying to think of a complicated solution, I didn't even consider the simplest one.
– ninesalt
Nov 14 '18 at 17:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305317%2fhigh-level-prototype-functions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9bZ,oyjDv1EF74OeRe8rO,W,Z
You may find proxies helpful for these situations.Take a look at MDN: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Mehmet Baker
Nov 14 '18 at 17:18