Fetching before navigation with multiple components using vue-router
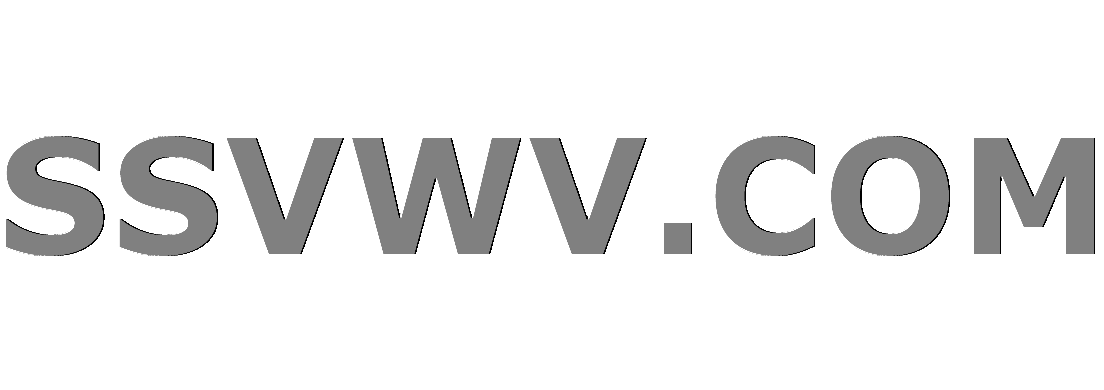
Multi tool use
Trying to figure out what is the best way to fetch some data before navigating to some routes that I have.
My routes are:
let routes = [
path: '/',
component: require('./views/Home.vue')
,
path: '/messages',
component: require('./views/Messages.vue'),
,
path: '/posts',
component: require('./views/Post.vue'),
,
path: '/login',
component: require('./views/Login.vue')
,
path: '/dashboard',
component: require('./views/Dashboard.vue'),
];
For /messages, /posts and /dashboard I want to fetch some data and while doing that show a spinner.
Vue-router docs suggest to use beforeRouteEnter, Fetching Before Navigation
beforeRouteEnter (to, from, next)
getPost(to.params.id, (err, post) =>
next(vm => vm.setData(err, post))
)
But my question is, must I implement this fetch logic in all my three view components?
Since it's the same data being fetched I don't want to repeat the logic in every component.
The beforeRouteEnter isn't called in my root component where I have this <router-view></router-view>
otherwise I feel that it would be the best place to have this logic.
I'm really new to vue and this one is a really hard nut to crack for me.
vue.js vue-component vue-router
add a comment |
Trying to figure out what is the best way to fetch some data before navigating to some routes that I have.
My routes are:
let routes = [
path: '/',
component: require('./views/Home.vue')
,
path: '/messages',
component: require('./views/Messages.vue'),
,
path: '/posts',
component: require('./views/Post.vue'),
,
path: '/login',
component: require('./views/Login.vue')
,
path: '/dashboard',
component: require('./views/Dashboard.vue'),
];
For /messages, /posts and /dashboard I want to fetch some data and while doing that show a spinner.
Vue-router docs suggest to use beforeRouteEnter, Fetching Before Navigation
beforeRouteEnter (to, from, next)
getPost(to.params.id, (err, post) =>
next(vm => vm.setData(err, post))
)
But my question is, must I implement this fetch logic in all my three view components?
Since it's the same data being fetched I don't want to repeat the logic in every component.
The beforeRouteEnter isn't called in my root component where I have this <router-view></router-view>
otherwise I feel that it would be the best place to have this logic.
I'm really new to vue and this one is a really hard nut to crack for me.
vue.js vue-component vue-router
add a comment |
Trying to figure out what is the best way to fetch some data before navigating to some routes that I have.
My routes are:
let routes = [
path: '/',
component: require('./views/Home.vue')
,
path: '/messages',
component: require('./views/Messages.vue'),
,
path: '/posts',
component: require('./views/Post.vue'),
,
path: '/login',
component: require('./views/Login.vue')
,
path: '/dashboard',
component: require('./views/Dashboard.vue'),
];
For /messages, /posts and /dashboard I want to fetch some data and while doing that show a spinner.
Vue-router docs suggest to use beforeRouteEnter, Fetching Before Navigation
beforeRouteEnter (to, from, next)
getPost(to.params.id, (err, post) =>
next(vm => vm.setData(err, post))
)
But my question is, must I implement this fetch logic in all my three view components?
Since it's the same data being fetched I don't want to repeat the logic in every component.
The beforeRouteEnter isn't called in my root component where I have this <router-view></router-view>
otherwise I feel that it would be the best place to have this logic.
I'm really new to vue and this one is a really hard nut to crack for me.
vue.js vue-component vue-router
Trying to figure out what is the best way to fetch some data before navigating to some routes that I have.
My routes are:
let routes = [
path: '/',
component: require('./views/Home.vue')
,
path: '/messages',
component: require('./views/Messages.vue'),
,
path: '/posts',
component: require('./views/Post.vue'),
,
path: '/login',
component: require('./views/Login.vue')
,
path: '/dashboard',
component: require('./views/Dashboard.vue'),
];
For /messages, /posts and /dashboard I want to fetch some data and while doing that show a spinner.
Vue-router docs suggest to use beforeRouteEnter, Fetching Before Navigation
beforeRouteEnter (to, from, next)
getPost(to.params.id, (err, post) =>
next(vm => vm.setData(err, post))
)
But my question is, must I implement this fetch logic in all my three view components?
Since it's the same data being fetched I don't want to repeat the logic in every component.
The beforeRouteEnter isn't called in my root component where I have this <router-view></router-view>
otherwise I feel that it would be the best place to have this logic.
I'm really new to vue and this one is a really hard nut to crack for me.
vue.js vue-component vue-router
vue.js vue-component vue-router
asked Nov 14 '18 at 18:20
ToydorToydor
1,15241641
1,15241641
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Are you aware of vuex
? Since you need to share the same data on three different pages, which sounds like a job for vuex
store. Basically you can import the store
into your router, and set data in the store instead of on the component, and then you can conditionally fetch the data by checking the status of the data in the store and if the data already exists, don't fetch. Something like the following:
import store from './store'
beforeRouteEnter (to, from, next)
if (store.state.post === null)
getPost(to.params.id, (err, post) =>
store.dispatch('setPost', post)
next()
)
Assume your store
has a state called post
and action called setPost
.
const store = new Vuex.Store(
state: post: null ,
actions:
setPost ( commit , post)
// set the post state here
)
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you usebeforeRouteEnter
to conditionally fetch data. The second part sits separately in astore.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntaxthis.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check inbeforeEach
in router file?
– Toydor
Nov 14 '18 at 20:54
1
Yeah. That's what I meant to say. Essentially check the route and store state in router withbeforeEach
or if you don't mind set up the logic in each of the three pages then I guessbeforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component isbeforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306515%2ffetching-before-navigation-with-multiple-components-using-vue-router%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Are you aware of vuex
? Since you need to share the same data on three different pages, which sounds like a job for vuex
store. Basically you can import the store
into your router, and set data in the store instead of on the component, and then you can conditionally fetch the data by checking the status of the data in the store and if the data already exists, don't fetch. Something like the following:
import store from './store'
beforeRouteEnter (to, from, next)
if (store.state.post === null)
getPost(to.params.id, (err, post) =>
store.dispatch('setPost', post)
next()
)
Assume your store
has a state called post
and action called setPost
.
const store = new Vuex.Store(
state: post: null ,
actions:
setPost ( commit , post)
// set the post state here
)
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you usebeforeRouteEnter
to conditionally fetch data. The second part sits separately in astore.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntaxthis.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check inbeforeEach
in router file?
– Toydor
Nov 14 '18 at 20:54
1
Yeah. That's what I meant to say. Essentially check the route and store state in router withbeforeEach
or if you don't mind set up the logic in each of the three pages then I guessbeforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component isbeforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
add a comment |
Are you aware of vuex
? Since you need to share the same data on three different pages, which sounds like a job for vuex
store. Basically you can import the store
into your router, and set data in the store instead of on the component, and then you can conditionally fetch the data by checking the status of the data in the store and if the data already exists, don't fetch. Something like the following:
import store from './store'
beforeRouteEnter (to, from, next)
if (store.state.post === null)
getPost(to.params.id, (err, post) =>
store.dispatch('setPost', post)
next()
)
Assume your store
has a state called post
and action called setPost
.
const store = new Vuex.Store(
state: post: null ,
actions:
setPost ( commit , post)
// set the post state here
)
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you usebeforeRouteEnter
to conditionally fetch data. The second part sits separately in astore.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntaxthis.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check inbeforeEach
in router file?
– Toydor
Nov 14 '18 at 20:54
1
Yeah. That's what I meant to say. Essentially check the route and store state in router withbeforeEach
or if you don't mind set up the logic in each of the three pages then I guessbeforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component isbeforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
add a comment |
Are you aware of vuex
? Since you need to share the same data on three different pages, which sounds like a job for vuex
store. Basically you can import the store
into your router, and set data in the store instead of on the component, and then you can conditionally fetch the data by checking the status of the data in the store and if the data already exists, don't fetch. Something like the following:
import store from './store'
beforeRouteEnter (to, from, next)
if (store.state.post === null)
getPost(to.params.id, (err, post) =>
store.dispatch('setPost', post)
next()
)
Assume your store
has a state called post
and action called setPost
.
const store = new Vuex.Store(
state: post: null ,
actions:
setPost ( commit , post)
// set the post state here
)
Are you aware of vuex
? Since you need to share the same data on three different pages, which sounds like a job for vuex
store. Basically you can import the store
into your router, and set data in the store instead of on the component, and then you can conditionally fetch the data by checking the status of the data in the store and if the data already exists, don't fetch. Something like the following:
import store from './store'
beforeRouteEnter (to, from, next)
if (store.state.post === null)
getPost(to.params.id, (err, post) =>
store.dispatch('setPost', post)
next()
)
Assume your store
has a state called post
and action called setPost
.
const store = new Vuex.Store(
state: post: null ,
actions:
setPost ( commit , post)
// set the post state here
)
edited Nov 14 '18 at 20:28
answered Nov 14 '18 at 18:34


PsidomPsidom
126k1290134
126k1290134
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you usebeforeRouteEnter
to conditionally fetch data. The second part sits separately in astore.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntaxthis.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check inbeforeEach
in router file?
– Toydor
Nov 14 '18 at 20:54
1
Yeah. That's what I meant to say. Essentially check the route and store state in router withbeforeEach
or if you don't mind set up the logic in each of the three pages then I guessbeforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component isbeforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
add a comment |
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you usebeforeRouteEnter
to conditionally fetch data. The second part sits separately in astore.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntaxthis.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check inbeforeEach
in router file?
– Toydor
Nov 14 '18 at 20:54
1
Yeah. That's what I meant to say. Essentially check the route and store state in router withbeforeEach
or if you don't mind set up the logic in each of the three pages then I guessbeforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component isbeforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
Vuex sounds really nice, I'll check it out tnx! But I still need to have this code on every component to check if I have fetched the data to the store?
– Toydor
Nov 14 '18 at 20:20
You don't need this to be on the components. The first part sits in the router file where you use
beforeRouteEnter
to conditionally fetch data. The second part sits separately in a store.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntax this.$store
– Psidom
Nov 14 '18 at 20:26
You don't need this to be on the components. The first part sits in the router file where you use
beforeRouteEnter
to conditionally fetch data. The second part sits separately in a store.js
which acts as a centralized data store for your application, and can be accessed from all components and pages, via the syntax this.$store
– Psidom
Nov 14 '18 at 20:26
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check in
beforeEach
in router file?– Toydor
Nov 14 '18 at 20:54
beforeRouteEnter seems only to be available in component router.vuejs.org/guide/advanced/… . Or you mean I put the check in
beforeEach
in router file?– Toydor
Nov 14 '18 at 20:54
1
1
Yeah. That's what I meant to say. Essentially check the route and store state in router with
beforeEach
or if you don't mind set up the logic in each of the three pages then I guess beforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component is beforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
Yeah. That's what I meant to say. Essentially check the route and store state in router with
beforeEach
or if you don't mind set up the logic in each of the three pages then I guess beforeRouteEnter
is also doable especially if you refactor all the logic into the store action, then all you need to do in the component is beforeRouteEnter (to, from, next) this.$store.dispatch('getPost', to.params.id)
– Psidom
Nov 14 '18 at 20:59
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306515%2ffetching-before-navigation-with-multiple-components-using-vue-router%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EZ1L,9Pi,vc,vL0hZ LS3oiR0pMJc,k8K25CtH51mickNADU74df0wD7I,dkkzJ,t1