React Native is ignoring state change
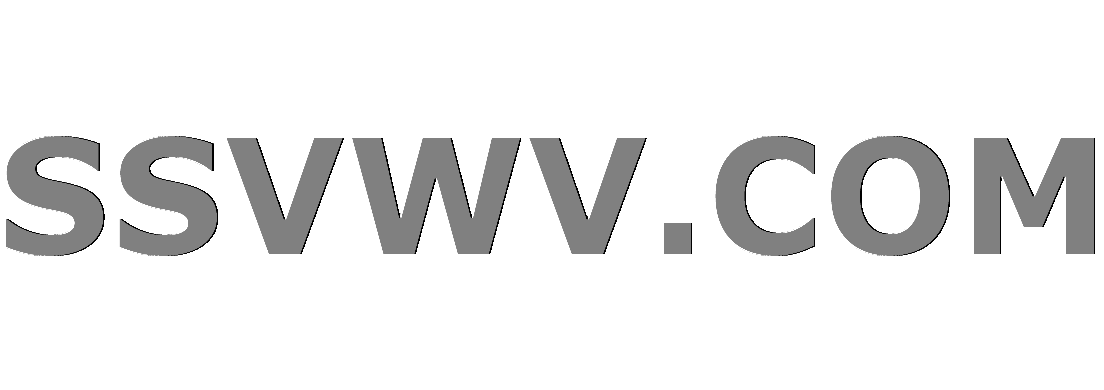
Multi tool use
up vote
0
down vote
favorite
Please explain why this isn't working. I am trying to replace one element with another on a button press in React, and, according to all documentation, what I am doing should work. However, React is entirely ignoring state changes and refusing to re-render an element. Here is my file:
import React, Component from 'react';
import View, Text, TextInput, TouchableOpacity from 'react-native';
import styles from '../styles';
import User from '../models/User';
export default class SignIn extends Component
constructor(props)
super(props);
this.state =
email: '',
password: '',
signIn: '',
errorMessage: null,
signUp: true
signIn()
var err = e => this.setState(errorMessage: e.message);
var next = u => this.props.navigation.push('Details');
User.login(this.state.email, this.state.password, err, next);
signUp()
var err = e => this.setState(errorMessage: e.message);
var next = _ => this.props.navigation.push('Details');
var user = new User(this.state.email, this.state.password);
user.save(err, next);
render()
return (
<View style=flex: 1, alignItems: 'center', justifyContent: 'center'>
<View style=flexDirection: 'row', width: 300, justifyContent: 'center'>
<TouchableOpacity onPress=_ => this.setState(signUp: true) style=[styles.navButton, styles.tabsButton]>
<Text style=[styles.buttonText, styles.tabs]>Sign Up</Text>
</TouchableOpacity>
<TouchableOpacity onPress=_ => this.setState(signUp: false) style=[styles.grey, styles.tabsButton]>
<Text style=[styles.greyText, styles.tabs]>Sign In</Text>
</TouchableOpacity>
</View>
this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
!this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
</View>
)
The Sign In button at the top should change the state and cause a Sign In form to appear and the Sign Up form to disappear, while the Sign Up button should do the opposite. If I console.log
the state after the change, it has changed, but nothing else happens. What is going on? All documentation shows that you can do this, but it doesn't work for me. I am running the app on an Android phone over Expo.
reactjs react-native react-state-management react-state
add a comment |
up vote
0
down vote
favorite
Please explain why this isn't working. I am trying to replace one element with another on a button press in React, and, according to all documentation, what I am doing should work. However, React is entirely ignoring state changes and refusing to re-render an element. Here is my file:
import React, Component from 'react';
import View, Text, TextInput, TouchableOpacity from 'react-native';
import styles from '../styles';
import User from '../models/User';
export default class SignIn extends Component
constructor(props)
super(props);
this.state =
email: '',
password: '',
signIn: '',
errorMessage: null,
signUp: true
signIn()
var err = e => this.setState(errorMessage: e.message);
var next = u => this.props.navigation.push('Details');
User.login(this.state.email, this.state.password, err, next);
signUp()
var err = e => this.setState(errorMessage: e.message);
var next = _ => this.props.navigation.push('Details');
var user = new User(this.state.email, this.state.password);
user.save(err, next);
render()
return (
<View style=flex: 1, alignItems: 'center', justifyContent: 'center'>
<View style=flexDirection: 'row', width: 300, justifyContent: 'center'>
<TouchableOpacity onPress=_ => this.setState(signUp: true) style=[styles.navButton, styles.tabsButton]>
<Text style=[styles.buttonText, styles.tabs]>Sign Up</Text>
</TouchableOpacity>
<TouchableOpacity onPress=_ => this.setState(signUp: false) style=[styles.grey, styles.tabsButton]>
<Text style=[styles.greyText, styles.tabs]>Sign In</Text>
</TouchableOpacity>
</View>
this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
!this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
</View>
)
The Sign In button at the top should change the state and cause a Sign In form to appear and the Sign Up form to disappear, while the Sign Up button should do the opposite. If I console.log
the state after the change, it has changed, but nothing else happens. What is going on? All documentation shows that you can do this, but it doesn't work for me. I am running the app on an Android phone over Expo.
reactjs react-native react-state-management react-state
I don't quite understand your code. Can you explain why regardless the value ofthis.state.signUp
you are rendering the same component?
– Isaac
Nov 12 at 1:29
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Please explain why this isn't working. I am trying to replace one element with another on a button press in React, and, according to all documentation, what I am doing should work. However, React is entirely ignoring state changes and refusing to re-render an element. Here is my file:
import React, Component from 'react';
import View, Text, TextInput, TouchableOpacity from 'react-native';
import styles from '../styles';
import User from '../models/User';
export default class SignIn extends Component
constructor(props)
super(props);
this.state =
email: '',
password: '',
signIn: '',
errorMessage: null,
signUp: true
signIn()
var err = e => this.setState(errorMessage: e.message);
var next = u => this.props.navigation.push('Details');
User.login(this.state.email, this.state.password, err, next);
signUp()
var err = e => this.setState(errorMessage: e.message);
var next = _ => this.props.navigation.push('Details');
var user = new User(this.state.email, this.state.password);
user.save(err, next);
render()
return (
<View style=flex: 1, alignItems: 'center', justifyContent: 'center'>
<View style=flexDirection: 'row', width: 300, justifyContent: 'center'>
<TouchableOpacity onPress=_ => this.setState(signUp: true) style=[styles.navButton, styles.tabsButton]>
<Text style=[styles.buttonText, styles.tabs]>Sign Up</Text>
</TouchableOpacity>
<TouchableOpacity onPress=_ => this.setState(signUp: false) style=[styles.grey, styles.tabsButton]>
<Text style=[styles.greyText, styles.tabs]>Sign In</Text>
</TouchableOpacity>
</View>
this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
!this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
</View>
)
The Sign In button at the top should change the state and cause a Sign In form to appear and the Sign Up form to disappear, while the Sign Up button should do the opposite. If I console.log
the state after the change, it has changed, but nothing else happens. What is going on? All documentation shows that you can do this, but it doesn't work for me. I am running the app on an Android phone over Expo.
reactjs react-native react-state-management react-state
Please explain why this isn't working. I am trying to replace one element with another on a button press in React, and, according to all documentation, what I am doing should work. However, React is entirely ignoring state changes and refusing to re-render an element. Here is my file:
import React, Component from 'react';
import View, Text, TextInput, TouchableOpacity from 'react-native';
import styles from '../styles';
import User from '../models/User';
export default class SignIn extends Component
constructor(props)
super(props);
this.state =
email: '',
password: '',
signIn: '',
errorMessage: null,
signUp: true
signIn()
var err = e => this.setState(errorMessage: e.message);
var next = u => this.props.navigation.push('Details');
User.login(this.state.email, this.state.password, err, next);
signUp()
var err = e => this.setState(errorMessage: e.message);
var next = _ => this.props.navigation.push('Details');
var user = new User(this.state.email, this.state.password);
user.save(err, next);
render()
return (
<View style=flex: 1, alignItems: 'center', justifyContent: 'center'>
<View style=flexDirection: 'row', width: 300, justifyContent: 'center'>
<TouchableOpacity onPress=_ => this.setState(signUp: true) style=[styles.navButton, styles.tabsButton]>
<Text style=[styles.buttonText, styles.tabs]>Sign Up</Text>
</TouchableOpacity>
<TouchableOpacity onPress=_ => this.setState(signUp: false) style=[styles.grey, styles.tabsButton]>
<Text style=[styles.greyText, styles.tabs]>Sign In</Text>
</TouchableOpacity>
</View>
this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
!this.state.signUp && <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
</View>
)
The Sign In button at the top should change the state and cause a Sign In form to appear and the Sign Up form to disappear, while the Sign Up button should do the opposite. If I console.log
the state after the change, it has changed, but nothing else happens. What is going on? All documentation shows that you can do this, but it doesn't work for me. I am running the app on an Android phone over Expo.
reactjs react-native react-state-management react-state
reactjs react-native react-state-management react-state
asked Nov 11 at 15:16


Isaac Krementsov
14816
14816
I don't quite understand your code. Can you explain why regardless the value ofthis.state.signUp
you are rendering the same component?
– Isaac
Nov 12 at 1:29
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46
add a comment |
I don't quite understand your code. Can you explain why regardless the value ofthis.state.signUp
you are rendering the same component?
– Isaac
Nov 12 at 1:29
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46
I don't quite understand your code. Can you explain why regardless the value of
this.state.signUp
you are rendering the same component?– Isaac
Nov 12 at 1:29
I don't quite understand your code. Can you explain why regardless the value of
this.state.signUp
you are rendering the same component?– Isaac
Nov 12 at 1:29
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46
add a comment |
1 Answer
1
active
oldest
votes
up vote
-1
down vote
accepted
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
If this wouldn't work, maybe styles?
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
accepted
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
If this wouldn't work, maybe styles?
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
add a comment |
up vote
-1
down vote
accepted
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
If this wouldn't work, maybe styles?
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
add a comment |
up vote
-1
down vote
accepted
up vote
-1
down vote
accepted
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
If this wouldn't work, maybe styles?
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
If this wouldn't work, maybe styles?
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
this.state.signUp
? <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=styles.navButton>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
: <View>
<Text style=color: 'red'>this.state.errorMessage</Text>
<TextInput
placeholder='Email'
style=styles.input
value=this.state.email
onChangeText=(email) => this.setState(email)
/>
<TextInput
placeholder='Password'
style=styles.input
secureTextEntry=true
onChangeText=(password) => this.setState(password)
/>
<TouchableOpacity style=[styles.navButton]>
<Text style=styles.buttonText>Sign Up</Text>
</TouchableOpacity>
</View>
answered Nov 12 at 1:17


Ziyo
21819
21819
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
add a comment |
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
@IsaacKrementsov: How can this be answer? It doesnt make sense
– Isaac
Nov 13 at 13:57
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
Did you try and did it work?
– Ziyo
Nov 13 at 16:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250129%2freact-native-is-ignoring-state-change%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wtmTrOsfpnVpgaTcodP
I don't quite understand your code. Can you explain why regardless the value of
this.state.signUp
you are rendering the same component?– Isaac
Nov 12 at 1:29
Sorry, I figured out the issue but I can't yet close the question
– Isaac Krementsov
Nov 13 at 13:46