Plot after rounding dataframe column not working
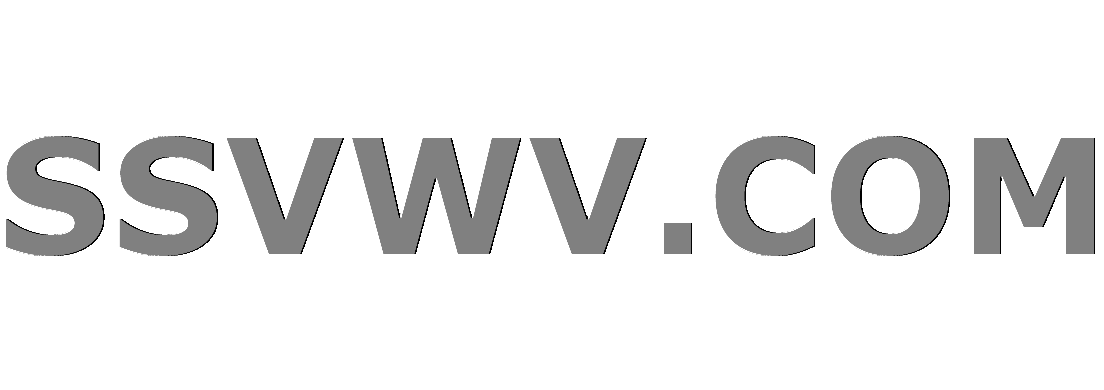
Multi tool use
up vote
1
down vote
favorite
I am trying to read the whitespace separated values, apply Savitzky-Golay filter to one of the columns, round the column to 6 decimal digits, plot the graph and export the data to the new file. Here is the working code where I commented out the line which makes graph window 'Not responding':
import pandas as pd
from datetime import datetime
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import savgol_filter
df = pd.read_csv('data.txt', delim_whitespace = True)
plt.plot(df.index, df.rotram, '-', lw=4)
#plt.plot(df.index, savgol_filter(df.rotram, 21, 3), 'r-', lw=2)
# smooth the 'rotram' column using Savitzky-Golay filter
df.rotram = savgol_filter(df.rotram, 21, 3)
# round to 6 decimal digits
#df.rotram = df.rotram.map(':.6f'.format) # <-- not responding when plotting
#df["rotram"] = df["rotram"].map(':,.6f'.format) # the same as above (not responding when plotting)
# When plot is removed then above rounding works well
plt.plot(df.index, df.rotram, 'r-', lw=2)
df.to_csv('filtered.txt', sep='t')
plt.show()
print "End"
The sample data looks like:
otklon rotram lakat rotnad
-6.240000 -3.317000 -34.445000 16.805000
-6.633000 -3.501000 -34.519000 17.192000
-5.099000 -2.742000 -34.456000 15.059000
-6.148000 -3.396000 -34.281000 17.277000
-4.797000 -3.032000 -34.851000 16.052000
-5.446000 -2.964000 -34.459000 15.677000
-6.341000 -3.490000 -34.934000 17.300000
-6.508000 -3.465000 -35.030000 16.722000
-6.513000 -3.505000 -35.018000 16.845000
-6.455000 -3.501000 -35.302000 16.896000
.
.
.
(more than 20000 lines)
The separator in the input file is space + TAB + space
.
If I uncomment the line df.rotram = df.rotram.map(':.6f'.format)
then program hangs (Not responding) with the empty graph although saved data is correct.
If I then remove the line plt.plot(df.index, df.rotram, 'r-', lw=2)
then the program ends normally.
Although saving the data to file after rounding works well, plotting doesn't :-/
python pandas matplotlib formatting
add a comment |
up vote
1
down vote
favorite
I am trying to read the whitespace separated values, apply Savitzky-Golay filter to one of the columns, round the column to 6 decimal digits, plot the graph and export the data to the new file. Here is the working code where I commented out the line which makes graph window 'Not responding':
import pandas as pd
from datetime import datetime
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import savgol_filter
df = pd.read_csv('data.txt', delim_whitespace = True)
plt.plot(df.index, df.rotram, '-', lw=4)
#plt.plot(df.index, savgol_filter(df.rotram, 21, 3), 'r-', lw=2)
# smooth the 'rotram' column using Savitzky-Golay filter
df.rotram = savgol_filter(df.rotram, 21, 3)
# round to 6 decimal digits
#df.rotram = df.rotram.map(':.6f'.format) # <-- not responding when plotting
#df["rotram"] = df["rotram"].map(':,.6f'.format) # the same as above (not responding when plotting)
# When plot is removed then above rounding works well
plt.plot(df.index, df.rotram, 'r-', lw=2)
df.to_csv('filtered.txt', sep='t')
plt.show()
print "End"
The sample data looks like:
otklon rotram lakat rotnad
-6.240000 -3.317000 -34.445000 16.805000
-6.633000 -3.501000 -34.519000 17.192000
-5.099000 -2.742000 -34.456000 15.059000
-6.148000 -3.396000 -34.281000 17.277000
-4.797000 -3.032000 -34.851000 16.052000
-5.446000 -2.964000 -34.459000 15.677000
-6.341000 -3.490000 -34.934000 17.300000
-6.508000 -3.465000 -35.030000 16.722000
-6.513000 -3.505000 -35.018000 16.845000
-6.455000 -3.501000 -35.302000 16.896000
.
.
.
(more than 20000 lines)
The separator in the input file is space + TAB + space
.
If I uncomment the line df.rotram = df.rotram.map(':.6f'.format)
then program hangs (Not responding) with the empty graph although saved data is correct.
If I then remove the line plt.plot(df.index, df.rotram, 'r-', lw=2)
then the program ends normally.
Although saving the data to file after rounding works well, plotting doesn't :-/
python pandas matplotlib formatting
1
Better would be to usedf.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
@coldspeed: You are right, when usinground()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.
– Chupo_cro
Nov 10 at 23:26
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to read the whitespace separated values, apply Savitzky-Golay filter to one of the columns, round the column to 6 decimal digits, plot the graph and export the data to the new file. Here is the working code where I commented out the line which makes graph window 'Not responding':
import pandas as pd
from datetime import datetime
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import savgol_filter
df = pd.read_csv('data.txt', delim_whitespace = True)
plt.plot(df.index, df.rotram, '-', lw=4)
#plt.plot(df.index, savgol_filter(df.rotram, 21, 3), 'r-', lw=2)
# smooth the 'rotram' column using Savitzky-Golay filter
df.rotram = savgol_filter(df.rotram, 21, 3)
# round to 6 decimal digits
#df.rotram = df.rotram.map(':.6f'.format) # <-- not responding when plotting
#df["rotram"] = df["rotram"].map(':,.6f'.format) # the same as above (not responding when plotting)
# When plot is removed then above rounding works well
plt.plot(df.index, df.rotram, 'r-', lw=2)
df.to_csv('filtered.txt', sep='t')
plt.show()
print "End"
The sample data looks like:
otklon rotram lakat rotnad
-6.240000 -3.317000 -34.445000 16.805000
-6.633000 -3.501000 -34.519000 17.192000
-5.099000 -2.742000 -34.456000 15.059000
-6.148000 -3.396000 -34.281000 17.277000
-4.797000 -3.032000 -34.851000 16.052000
-5.446000 -2.964000 -34.459000 15.677000
-6.341000 -3.490000 -34.934000 17.300000
-6.508000 -3.465000 -35.030000 16.722000
-6.513000 -3.505000 -35.018000 16.845000
-6.455000 -3.501000 -35.302000 16.896000
.
.
.
(more than 20000 lines)
The separator in the input file is space + TAB + space
.
If I uncomment the line df.rotram = df.rotram.map(':.6f'.format)
then program hangs (Not responding) with the empty graph although saved data is correct.
If I then remove the line plt.plot(df.index, df.rotram, 'r-', lw=2)
then the program ends normally.
Although saving the data to file after rounding works well, plotting doesn't :-/
python pandas matplotlib formatting
I am trying to read the whitespace separated values, apply Savitzky-Golay filter to one of the columns, round the column to 6 decimal digits, plot the graph and export the data to the new file. Here is the working code where I commented out the line which makes graph window 'Not responding':
import pandas as pd
from datetime import datetime
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import savgol_filter
df = pd.read_csv('data.txt', delim_whitespace = True)
plt.plot(df.index, df.rotram, '-', lw=4)
#plt.plot(df.index, savgol_filter(df.rotram, 21, 3), 'r-', lw=2)
# smooth the 'rotram' column using Savitzky-Golay filter
df.rotram = savgol_filter(df.rotram, 21, 3)
# round to 6 decimal digits
#df.rotram = df.rotram.map(':.6f'.format) # <-- not responding when plotting
#df["rotram"] = df["rotram"].map(':,.6f'.format) # the same as above (not responding when plotting)
# When plot is removed then above rounding works well
plt.plot(df.index, df.rotram, 'r-', lw=2)
df.to_csv('filtered.txt', sep='t')
plt.show()
print "End"
The sample data looks like:
otklon rotram lakat rotnad
-6.240000 -3.317000 -34.445000 16.805000
-6.633000 -3.501000 -34.519000 17.192000
-5.099000 -2.742000 -34.456000 15.059000
-6.148000 -3.396000 -34.281000 17.277000
-4.797000 -3.032000 -34.851000 16.052000
-5.446000 -2.964000 -34.459000 15.677000
-6.341000 -3.490000 -34.934000 17.300000
-6.508000 -3.465000 -35.030000 16.722000
-6.513000 -3.505000 -35.018000 16.845000
-6.455000 -3.501000 -35.302000 16.896000
.
.
.
(more than 20000 lines)
The separator in the input file is space + TAB + space
.
If I uncomment the line df.rotram = df.rotram.map(':.6f'.format)
then program hangs (Not responding) with the empty graph although saved data is correct.
If I then remove the line plt.plot(df.index, df.rotram, 'r-', lw=2)
then the program ends normally.
Although saving the data to file after rounding works well, plotting doesn't :-/
python pandas matplotlib formatting
python pandas matplotlib formatting
asked Nov 10 at 23:19
Chupo_cro
350213
350213
1
Better would be to usedf.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
@coldspeed: You are right, when usinground()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.
– Chupo_cro
Nov 10 at 23:26
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40
add a comment |
1
Better would be to usedf.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
@coldspeed: You are right, when usinground()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.
– Chupo_cro
Nov 10 at 23:26
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40
1
1
Better would be to use
df.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
Better would be to use
df.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
@coldspeed: You are right, when using
round()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.– Chupo_cro
Nov 10 at 23:26
@coldspeed: You are right, when using
round()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.– Chupo_cro
Nov 10 at 23:26
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
If you want to round your column to the Nth decimal place, use pd.Series.round
or np.around
:
df.rotram = df.rotram.round(decimals=6)
# Or,
# df.rotram = np.around(df.rotram, decimals=6)
However, I would still like to know why the above code doesn't work as
expected.
When you call map
, you convert your numeric column to a string. Pandas will plot this data without making any assumptions. For your sample data, the plot looks hideous:
Versus, the latter case using round
:
The plots are completely different (in the former case, each string is sorted lexicographically and given its own tick on the y-axis).
Are you saying the program didn't freeze when you tried to usemap
? BTW, when I accidentally uncommented themap
code after insertinground()
below themap
, the error was:Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/
– Chupo_cro
Nov 10 at 23:50
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
If you want to round your column to the Nth decimal place, use pd.Series.round
or np.around
:
df.rotram = df.rotram.round(decimals=6)
# Or,
# df.rotram = np.around(df.rotram, decimals=6)
However, I would still like to know why the above code doesn't work as
expected.
When you call map
, you convert your numeric column to a string. Pandas will plot this data without making any assumptions. For your sample data, the plot looks hideous:
Versus, the latter case using round
:
The plots are completely different (in the former case, each string is sorted lexicographically and given its own tick on the y-axis).
Are you saying the program didn't freeze when you tried to usemap
? BTW, when I accidentally uncommented themap
code after insertinground()
below themap
, the error was:Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/
– Chupo_cro
Nov 10 at 23:50
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
add a comment |
up vote
1
down vote
accepted
If you want to round your column to the Nth decimal place, use pd.Series.round
or np.around
:
df.rotram = df.rotram.round(decimals=6)
# Or,
# df.rotram = np.around(df.rotram, decimals=6)
However, I would still like to know why the above code doesn't work as
expected.
When you call map
, you convert your numeric column to a string. Pandas will plot this data without making any assumptions. For your sample data, the plot looks hideous:
Versus, the latter case using round
:
The plots are completely different (in the former case, each string is sorted lexicographically and given its own tick on the y-axis).
Are you saying the program didn't freeze when you tried to usemap
? BTW, when I accidentally uncommented themap
code after insertinground()
below themap
, the error was:Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/
– Chupo_cro
Nov 10 at 23:50
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
If you want to round your column to the Nth decimal place, use pd.Series.round
or np.around
:
df.rotram = df.rotram.round(decimals=6)
# Or,
# df.rotram = np.around(df.rotram, decimals=6)
However, I would still like to know why the above code doesn't work as
expected.
When you call map
, you convert your numeric column to a string. Pandas will plot this data without making any assumptions. For your sample data, the plot looks hideous:
Versus, the latter case using round
:
The plots are completely different (in the former case, each string is sorted lexicographically and given its own tick on the y-axis).
If you want to round your column to the Nth decimal place, use pd.Series.round
or np.around
:
df.rotram = df.rotram.round(decimals=6)
# Or,
# df.rotram = np.around(df.rotram, decimals=6)
However, I would still like to know why the above code doesn't work as
expected.
When you call map
, you convert your numeric column to a string. Pandas will plot this data without making any assumptions. For your sample data, the plot looks hideous:
Versus, the latter case using round
:
The plots are completely different (in the former case, each string is sorted lexicographically and given its own tick on the y-axis).
answered Nov 10 at 23:40


coldspeed
111k17101169
111k17101169
Are you saying the program didn't freeze when you tried to usemap
? BTW, when I accidentally uncommented themap
code after insertinground()
below themap
, the error was:Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/
– Chupo_cro
Nov 10 at 23:50
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
add a comment |
Are you saying the program didn't freeze when you tried to usemap
? BTW, when I accidentally uncommented themap
code after insertinground()
below themap
, the error was:Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/
– Chupo_cro
Nov 10 at 23:50
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
Are you saying the program didn't freeze when you tried to use
map
? BTW, when I accidentally uncommented the map
code after inserting round()
below the map
, the error was: Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/– Chupo_cro
Nov 10 at 23:50
Are you saying the program didn't freeze when you tried to use
map
? BTW, when I accidentally uncommented the map
code after inserting round()
below the map
, the error was: Type error: can't multiply sequence by non-int of type 'float'
, from what it seems as there aren't any strings :-/– Chupo_cro
Nov 10 at 23:50
1
1
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
@Chupo_cro I understand what that error is saying, so what you're trying to conclude is not right. str.format will produce a string (and strings can be multiplied by integers - the result is the string replicated N times .... the issue arises when you multiply a string and a float—that operation isn't defined). Anyway, it does not hang for me because I have 10 data points while you have 20,000, and making a plot with 10 ticks is easy (while wrong) while making a plot with 20,000 ticks isn't.
– coldspeed
Nov 10 at 23:53
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244369%2fplot-after-rounding-dataframe-column-not-working%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dmGG bZo
1
Better would be to use
df.rotram = df.rotram.round(decimals=6)
– coldspeed
Nov 10 at 23:21
@coldspeed: You are right, when using
round()
everything works well, thank you! However, I would still like to know why the above code doesn't work as expected.– Chupo_cro
Nov 10 at 23:26
I assume it does not understand how to plot strings that look like numbers.
– coldspeed
Nov 10 at 23:35
It's strange there isn't an error but the program just hangs :-/
– Chupo_cro
Nov 10 at 23:40