Could not find acceptable representation for file download
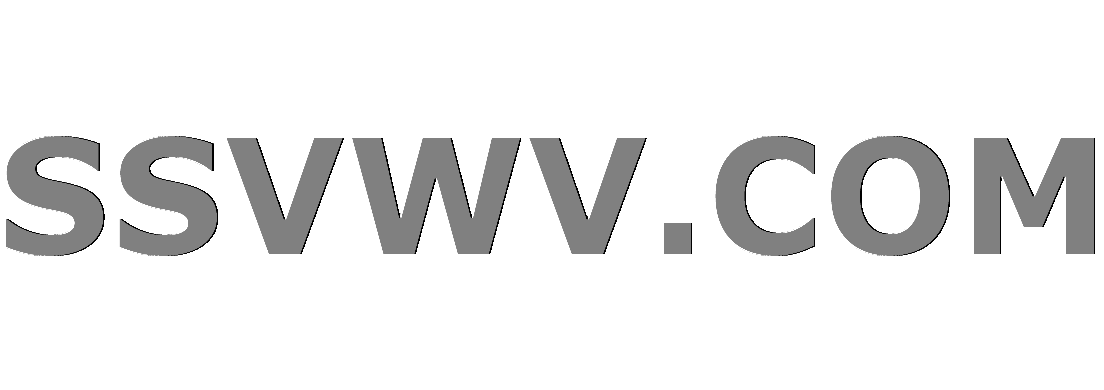
Multi tool use
up vote
2
down vote
favorite
I want to implement file download using this Angular 6 code:
Rest API:
private static final Logger LOG = LoggerFactory.getLogger(DownloadsController.class);
private static final String EXTERNAL_FILE_PATH = "/Users/test/Documents/blacklist_api.pdf";
@GetMapping("export")
public ResponseEntity<FileInputStream> export() throws IOException
File pdfFile = Paths.get(EXTERNAL_FILE_PATH).toFile();
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity.ok().headers(headers).contentLength(pdfFile.length())
.contentType(MediaType.parseMediaType("application/pdf"))
.body(new FileInputStream(pdfFile));
Service:
import Injectable from '@angular/core';
import HttpClient, HttpParams from "@angular/common/http";
import Observable from "rxjs/index";
import environment from "../../../environments/environment";
import HttpUtils from "../common/http-utils";
import map from 'rxjs/operators';
import Http, ResponseContentType from '@angular/http';
@Injectable(
providedIn: 'root'
)
export class DownloadService
constructor(private http: HttpClient)
downloadPDF(): any
return this.http.get(environment.api.urls.downloads.getPdf,
responseType: 'blob'
)
.pipe(
map((res: any) =>
return new Blob([res.blob()],
type: 'application/pdf'
)
)
);
Component:
import Component, OnInit from '@angular/core';
import DownloadService from "../service/download.service";
import ActivatedRoute, Router from "@angular/router";
import flatMap from "rxjs/internal/operators";
import of from "rxjs/index";
import map from 'rxjs/operators';
@Component(
selector: 'app-download',
templateUrl: './download.component.html',
styleUrls: ['./download.component.scss']
)
export class DownloadComponent implements OnInit
constructor(private downloadService: DownloadService,
private router: Router,
private route: ActivatedRoute)
ngOnInit()
export()
this.downloadService.downloadPDF().subscribe(res =>
const fileURL = URL.createObjectURL(res);
window.open(fileURL, '_blank');
);
The file is present in the directory but when I try to download it I get error:
18:35:25,032 WARN [org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver] (default task-2) Resolved [org.springframework.web.HttpMediaTypeNotAcceptableException: Could not find acceptable representation]
Do you know how I can fix this issue?
Do I need to add additional configuration in order to download the file via Angular web UI?
I use spring-boot-starter-parent version 2.1.0.RELEASE
spring angular spring-boot spring-data-jpa angular6
add a comment |
up vote
2
down vote
favorite
I want to implement file download using this Angular 6 code:
Rest API:
private static final Logger LOG = LoggerFactory.getLogger(DownloadsController.class);
private static final String EXTERNAL_FILE_PATH = "/Users/test/Documents/blacklist_api.pdf";
@GetMapping("export")
public ResponseEntity<FileInputStream> export() throws IOException
File pdfFile = Paths.get(EXTERNAL_FILE_PATH).toFile();
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity.ok().headers(headers).contentLength(pdfFile.length())
.contentType(MediaType.parseMediaType("application/pdf"))
.body(new FileInputStream(pdfFile));
Service:
import Injectable from '@angular/core';
import HttpClient, HttpParams from "@angular/common/http";
import Observable from "rxjs/index";
import environment from "../../../environments/environment";
import HttpUtils from "../common/http-utils";
import map from 'rxjs/operators';
import Http, ResponseContentType from '@angular/http';
@Injectable(
providedIn: 'root'
)
export class DownloadService
constructor(private http: HttpClient)
downloadPDF(): any
return this.http.get(environment.api.urls.downloads.getPdf,
responseType: 'blob'
)
.pipe(
map((res: any) =>
return new Blob([res.blob()],
type: 'application/pdf'
)
)
);
Component:
import Component, OnInit from '@angular/core';
import DownloadService from "../service/download.service";
import ActivatedRoute, Router from "@angular/router";
import flatMap from "rxjs/internal/operators";
import of from "rxjs/index";
import map from 'rxjs/operators';
@Component(
selector: 'app-download',
templateUrl: './download.component.html',
styleUrls: ['./download.component.scss']
)
export class DownloadComponent implements OnInit
constructor(private downloadService: DownloadService,
private router: Router,
private route: ActivatedRoute)
ngOnInit()
export()
this.downloadService.downloadPDF().subscribe(res =>
const fileURL = URL.createObjectURL(res);
window.open(fileURL, '_blank');
);
The file is present in the directory but when I try to download it I get error:
18:35:25,032 WARN [org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver] (default task-2) Resolved [org.springframework.web.HttpMediaTypeNotAcceptableException: Could not find acceptable representation]
Do you know how I can fix this issue?
Do I need to add additional configuration in order to download the file via Angular web UI?
I use spring-boot-starter-parent version 2.1.0.RELEASE
spring angular spring-boot spring-data-jpa angular6
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
1
UseFile Saver
npmjs.com/package/ngx-filesaver
– Sunil Singh
Nov 10 at 16:45
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
I updated the code.
– Peter Penzov
Nov 10 at 16:50
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I want to implement file download using this Angular 6 code:
Rest API:
private static final Logger LOG = LoggerFactory.getLogger(DownloadsController.class);
private static final String EXTERNAL_FILE_PATH = "/Users/test/Documents/blacklist_api.pdf";
@GetMapping("export")
public ResponseEntity<FileInputStream> export() throws IOException
File pdfFile = Paths.get(EXTERNAL_FILE_PATH).toFile();
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity.ok().headers(headers).contentLength(pdfFile.length())
.contentType(MediaType.parseMediaType("application/pdf"))
.body(new FileInputStream(pdfFile));
Service:
import Injectable from '@angular/core';
import HttpClient, HttpParams from "@angular/common/http";
import Observable from "rxjs/index";
import environment from "../../../environments/environment";
import HttpUtils from "../common/http-utils";
import map from 'rxjs/operators';
import Http, ResponseContentType from '@angular/http';
@Injectable(
providedIn: 'root'
)
export class DownloadService
constructor(private http: HttpClient)
downloadPDF(): any
return this.http.get(environment.api.urls.downloads.getPdf,
responseType: 'blob'
)
.pipe(
map((res: any) =>
return new Blob([res.blob()],
type: 'application/pdf'
)
)
);
Component:
import Component, OnInit from '@angular/core';
import DownloadService from "../service/download.service";
import ActivatedRoute, Router from "@angular/router";
import flatMap from "rxjs/internal/operators";
import of from "rxjs/index";
import map from 'rxjs/operators';
@Component(
selector: 'app-download',
templateUrl: './download.component.html',
styleUrls: ['./download.component.scss']
)
export class DownloadComponent implements OnInit
constructor(private downloadService: DownloadService,
private router: Router,
private route: ActivatedRoute)
ngOnInit()
export()
this.downloadService.downloadPDF().subscribe(res =>
const fileURL = URL.createObjectURL(res);
window.open(fileURL, '_blank');
);
The file is present in the directory but when I try to download it I get error:
18:35:25,032 WARN [org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver] (default task-2) Resolved [org.springframework.web.HttpMediaTypeNotAcceptableException: Could not find acceptable representation]
Do you know how I can fix this issue?
Do I need to add additional configuration in order to download the file via Angular web UI?
I use spring-boot-starter-parent version 2.1.0.RELEASE
spring angular spring-boot spring-data-jpa angular6
I want to implement file download using this Angular 6 code:
Rest API:
private static final Logger LOG = LoggerFactory.getLogger(DownloadsController.class);
private static final String EXTERNAL_FILE_PATH = "/Users/test/Documents/blacklist_api.pdf";
@GetMapping("export")
public ResponseEntity<FileInputStream> export() throws IOException
File pdfFile = Paths.get(EXTERNAL_FILE_PATH).toFile();
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity.ok().headers(headers).contentLength(pdfFile.length())
.contentType(MediaType.parseMediaType("application/pdf"))
.body(new FileInputStream(pdfFile));
Service:
import Injectable from '@angular/core';
import HttpClient, HttpParams from "@angular/common/http";
import Observable from "rxjs/index";
import environment from "../../../environments/environment";
import HttpUtils from "../common/http-utils";
import map from 'rxjs/operators';
import Http, ResponseContentType from '@angular/http';
@Injectable(
providedIn: 'root'
)
export class DownloadService
constructor(private http: HttpClient)
downloadPDF(): any
return this.http.get(environment.api.urls.downloads.getPdf,
responseType: 'blob'
)
.pipe(
map((res: any) =>
return new Blob([res.blob()],
type: 'application/pdf'
)
)
);
Component:
import Component, OnInit from '@angular/core';
import DownloadService from "../service/download.service";
import ActivatedRoute, Router from "@angular/router";
import flatMap from "rxjs/internal/operators";
import of from "rxjs/index";
import map from 'rxjs/operators';
@Component(
selector: 'app-download',
templateUrl: './download.component.html',
styleUrls: ['./download.component.scss']
)
export class DownloadComponent implements OnInit
constructor(private downloadService: DownloadService,
private router: Router,
private route: ActivatedRoute)
ngOnInit()
export()
this.downloadService.downloadPDF().subscribe(res =>
const fileURL = URL.createObjectURL(res);
window.open(fileURL, '_blank');
);
The file is present in the directory but when I try to download it I get error:
18:35:25,032 WARN [org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver] (default task-2) Resolved [org.springframework.web.HttpMediaTypeNotAcceptableException: Could not find acceptable representation]
Do you know how I can fix this issue?
Do I need to add additional configuration in order to download the file via Angular web UI?
I use spring-boot-starter-parent version 2.1.0.RELEASE
spring angular spring-boot spring-data-jpa angular6
spring angular spring-boot spring-data-jpa angular6
edited Nov 10 at 16:50
asked Nov 10 at 16:38
Peter Penzov
1656177371
1656177371
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
1
UseFile Saver
npmjs.com/package/ngx-filesaver
– Sunil Singh
Nov 10 at 16:45
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
I updated the code.
– Peter Penzov
Nov 10 at 16:50
add a comment |
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
1
UseFile Saver
npmjs.com/package/ngx-filesaver
– Sunil Singh
Nov 10 at 16:45
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
I updated the code.
– Peter Penzov
Nov 10 at 16:50
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
1
1
Use
File Saver
npmjs.com/package/ngx-filesaver– Sunil Singh
Nov 10 at 16:45
Use
File Saver
npmjs.com/package/ngx-filesaver– Sunil Singh
Nov 10 at 16:45
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
I updated the code.
– Peter Penzov
Nov 10 at 16:50
I updated the code.
– Peter Penzov
Nov 10 at 16:50
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241079%2fcould-not-find-acceptable-representation-for-file-download%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P6f2 K 8viZV2W7ZS6NYgUf3dhf PS
from the logs we .. the path to the file is not correct try to check it
– Mohamed Ali RACHID
Nov 10 at 16:42
1
Use
File Saver
npmjs.com/package/ngx-filesaver– Sunil Singh
Nov 10 at 16:45
Can you paste working example so I can vote it, please?
– Peter Penzov
Nov 10 at 16:47
I updated the code.
– Peter Penzov
Nov 10 at 16:50