angular-meteor app online and offline inconsistent image using gridFS
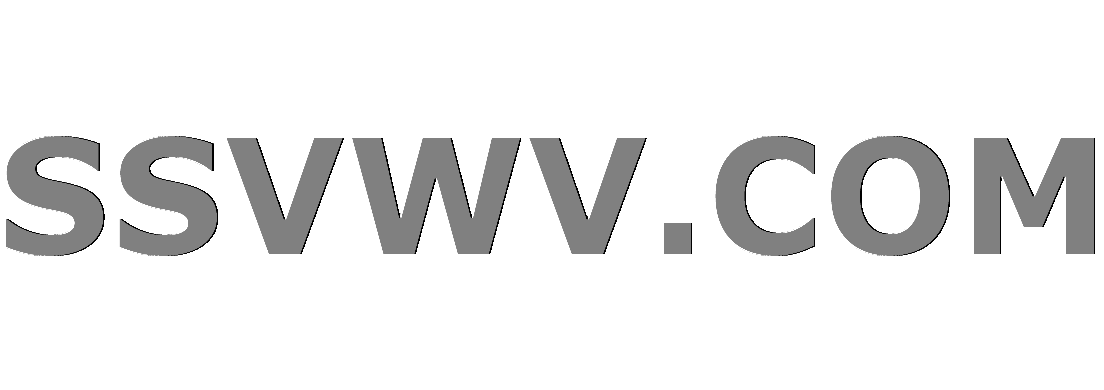
Multi tool use
up vote
0
down vote
favorite
I am working on Meteor/AngularJs app(meteor version 1.6 and angular version 1.6) where I have two collections, first collection is 'userWork' and second collection is 'images'. For images, I am using collectionFS package and gridFS storage adapter. I am passing userWork reference (i.e WOUID) into images collection as shown in below code. I need this to work for both offline and online images.
code of cfs.images.filerecord collection
"_id" : "",
"original" : ,
"WOUID" : "dfIFS",
"uploadedAt" : ISODate(""),
"copies" :
"original" : ,
"thumbnail" :
code of userWork collection
"_id" : ObjectId(""),
"woNum" : NumberInt(1234),
"createdOn" : ISODate(""),
"WOUID" : "dfIFS",
"userID" : ""
I am subscribing the usersWork and images using below code inside client/common/subscriptions/appSvc.js file.
Meteor.subscribe('UserWorkOrders',
onReady: function () ,
onError: function ()
);
Meteor.subscribe('images',
onReady: function () ,
onError: function ()
);
In server I am using below code to publish user work and images
server/publications/userwork.js
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
return userWork.find(criteria);
);
server/publications/images.js.
Meteor.publish('images', function ()
var criteria = ; //change criteria according to condition
return Images.find(criteria);
);
On client side to list the user work, I am getting list of documents from userWork collection and the images of respective work from images collection according to WOUID. I am calling a function to list the images of each user work. I am using below code to show image for each work in list.
client/modules/userwork/views/worklist.html
<ion-list>
<ion-item ng-repeat="ord in orders track by ord._id" >
<img ng-src="getImgSrc(ord)">
</ion-item>
</ion-list>
code of getImgSrc function is
client/modules/userwork/controllers/userWorkCtrl.js
$scope.getImgSrc = function(wo)
var fetchWOImages = Images.findOne(
"$and":[
WOUID:$exists:true
,
WOUID:wo.WOUID
]
);
return $rootScope.serverUrl+"/cfs/files/images/"+fetchWOImages.getFileRecord()._id+"?store=thumbnail";
;
I am getting inconsistent images sometimes while changing screens between work list, individual work & dashboard and sometimes no images are being shown, for which we have to wait for a long time.
I am thinking that the issue might be due to Images.find() query in getImgSrc function on client side (as given above).
I have tried different ways to fix this issue and below are my findings.
One solution is to use aggregate query but I am using mongodb 3.0 and don't want to upgrade mongodb version for some reason, due to which I can't use aggregate query on server to match images with userwork and return the merged result to client in 'UserWorkOrders' publication. Also aggregate query is not returning any cursor for publication.
Another solution is that I will merge the images with userWork documents in 'UserWorkOrders' publication as shown in below code but I am not getting any data on client side.
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
var wos = userWork.find(criteria);
wos.forEach(function(o,i)
var fetchWOImages = Images.find(
"$and":[
WOUID:$exists:true
,
WOUID:o.WOUID
]
).fetch();
o.imgs = fetchWOImages;
);
return wos;
);
So I am looking for something that will help me in getting images(below 1mb size) in much faster and easier way for my above problem.
I will be happy to work for any other alternative ways instead of using collectionFS for my images issue but it should be reactive so that it can work on both online and offline.
Thanks for your efforts and help.
mongodb meteor gridfs angular-meteor collectionfs
add a comment |
up vote
0
down vote
favorite
I am working on Meteor/AngularJs app(meteor version 1.6 and angular version 1.6) where I have two collections, first collection is 'userWork' and second collection is 'images'. For images, I am using collectionFS package and gridFS storage adapter. I am passing userWork reference (i.e WOUID) into images collection as shown in below code. I need this to work for both offline and online images.
code of cfs.images.filerecord collection
"_id" : "",
"original" : ,
"WOUID" : "dfIFS",
"uploadedAt" : ISODate(""),
"copies" :
"original" : ,
"thumbnail" :
code of userWork collection
"_id" : ObjectId(""),
"woNum" : NumberInt(1234),
"createdOn" : ISODate(""),
"WOUID" : "dfIFS",
"userID" : ""
I am subscribing the usersWork and images using below code inside client/common/subscriptions/appSvc.js file.
Meteor.subscribe('UserWorkOrders',
onReady: function () ,
onError: function ()
);
Meteor.subscribe('images',
onReady: function () ,
onError: function ()
);
In server I am using below code to publish user work and images
server/publications/userwork.js
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
return userWork.find(criteria);
);
server/publications/images.js.
Meteor.publish('images', function ()
var criteria = ; //change criteria according to condition
return Images.find(criteria);
);
On client side to list the user work, I am getting list of documents from userWork collection and the images of respective work from images collection according to WOUID. I am calling a function to list the images of each user work. I am using below code to show image for each work in list.
client/modules/userwork/views/worklist.html
<ion-list>
<ion-item ng-repeat="ord in orders track by ord._id" >
<img ng-src="getImgSrc(ord)">
</ion-item>
</ion-list>
code of getImgSrc function is
client/modules/userwork/controllers/userWorkCtrl.js
$scope.getImgSrc = function(wo)
var fetchWOImages = Images.findOne(
"$and":[
WOUID:$exists:true
,
WOUID:wo.WOUID
]
);
return $rootScope.serverUrl+"/cfs/files/images/"+fetchWOImages.getFileRecord()._id+"?store=thumbnail";
;
I am getting inconsistent images sometimes while changing screens between work list, individual work & dashboard and sometimes no images are being shown, for which we have to wait for a long time.
I am thinking that the issue might be due to Images.find() query in getImgSrc function on client side (as given above).
I have tried different ways to fix this issue and below are my findings.
One solution is to use aggregate query but I am using mongodb 3.0 and don't want to upgrade mongodb version for some reason, due to which I can't use aggregate query on server to match images with userwork and return the merged result to client in 'UserWorkOrders' publication. Also aggregate query is not returning any cursor for publication.
Another solution is that I will merge the images with userWork documents in 'UserWorkOrders' publication as shown in below code but I am not getting any data on client side.
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
var wos = userWork.find(criteria);
wos.forEach(function(o,i)
var fetchWOImages = Images.find(
"$and":[
WOUID:$exists:true
,
WOUID:o.WOUID
]
).fetch();
o.imgs = fetchWOImages;
);
return wos;
);
So I am looking for something that will help me in getting images(below 1mb size) in much faster and easier way for my above problem.
I will be happy to work for any other alternative ways instead of using collectionFS for my images issue but it should be reactive so that it can work on both online and offline.
Thanks for your efforts and help.
mongodb meteor gridfs angular-meteor collectionfs
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am working on Meteor/AngularJs app(meteor version 1.6 and angular version 1.6) where I have two collections, first collection is 'userWork' and second collection is 'images'. For images, I am using collectionFS package and gridFS storage adapter. I am passing userWork reference (i.e WOUID) into images collection as shown in below code. I need this to work for both offline and online images.
code of cfs.images.filerecord collection
"_id" : "",
"original" : ,
"WOUID" : "dfIFS",
"uploadedAt" : ISODate(""),
"copies" :
"original" : ,
"thumbnail" :
code of userWork collection
"_id" : ObjectId(""),
"woNum" : NumberInt(1234),
"createdOn" : ISODate(""),
"WOUID" : "dfIFS",
"userID" : ""
I am subscribing the usersWork and images using below code inside client/common/subscriptions/appSvc.js file.
Meteor.subscribe('UserWorkOrders',
onReady: function () ,
onError: function ()
);
Meteor.subscribe('images',
onReady: function () ,
onError: function ()
);
In server I am using below code to publish user work and images
server/publications/userwork.js
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
return userWork.find(criteria);
);
server/publications/images.js.
Meteor.publish('images', function ()
var criteria = ; //change criteria according to condition
return Images.find(criteria);
);
On client side to list the user work, I am getting list of documents from userWork collection and the images of respective work from images collection according to WOUID. I am calling a function to list the images of each user work. I am using below code to show image for each work in list.
client/modules/userwork/views/worklist.html
<ion-list>
<ion-item ng-repeat="ord in orders track by ord._id" >
<img ng-src="getImgSrc(ord)">
</ion-item>
</ion-list>
code of getImgSrc function is
client/modules/userwork/controllers/userWorkCtrl.js
$scope.getImgSrc = function(wo)
var fetchWOImages = Images.findOne(
"$and":[
WOUID:$exists:true
,
WOUID:wo.WOUID
]
);
return $rootScope.serverUrl+"/cfs/files/images/"+fetchWOImages.getFileRecord()._id+"?store=thumbnail";
;
I am getting inconsistent images sometimes while changing screens between work list, individual work & dashboard and sometimes no images are being shown, for which we have to wait for a long time.
I am thinking that the issue might be due to Images.find() query in getImgSrc function on client side (as given above).
I have tried different ways to fix this issue and below are my findings.
One solution is to use aggregate query but I am using mongodb 3.0 and don't want to upgrade mongodb version for some reason, due to which I can't use aggregate query on server to match images with userwork and return the merged result to client in 'UserWorkOrders' publication. Also aggregate query is not returning any cursor for publication.
Another solution is that I will merge the images with userWork documents in 'UserWorkOrders' publication as shown in below code but I am not getting any data on client side.
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
var wos = userWork.find(criteria);
wos.forEach(function(o,i)
var fetchWOImages = Images.find(
"$and":[
WOUID:$exists:true
,
WOUID:o.WOUID
]
).fetch();
o.imgs = fetchWOImages;
);
return wos;
);
So I am looking for something that will help me in getting images(below 1mb size) in much faster and easier way for my above problem.
I will be happy to work for any other alternative ways instead of using collectionFS for my images issue but it should be reactive so that it can work on both online and offline.
Thanks for your efforts and help.
mongodb meteor gridfs angular-meteor collectionfs
I am working on Meteor/AngularJs app(meteor version 1.6 and angular version 1.6) where I have two collections, first collection is 'userWork' and second collection is 'images'. For images, I am using collectionFS package and gridFS storage adapter. I am passing userWork reference (i.e WOUID) into images collection as shown in below code. I need this to work for both offline and online images.
code of cfs.images.filerecord collection
"_id" : "",
"original" : ,
"WOUID" : "dfIFS",
"uploadedAt" : ISODate(""),
"copies" :
"original" : ,
"thumbnail" :
code of userWork collection
"_id" : ObjectId(""),
"woNum" : NumberInt(1234),
"createdOn" : ISODate(""),
"WOUID" : "dfIFS",
"userID" : ""
I am subscribing the usersWork and images using below code inside client/common/subscriptions/appSvc.js file.
Meteor.subscribe('UserWorkOrders',
onReady: function () ,
onError: function ()
);
Meteor.subscribe('images',
onReady: function () ,
onError: function ()
);
In server I am using below code to publish user work and images
server/publications/userwork.js
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
return userWork.find(criteria);
);
server/publications/images.js.
Meteor.publish('images', function ()
var criteria = ; //change criteria according to condition
return Images.find(criteria);
);
On client side to list the user work, I am getting list of documents from userWork collection and the images of respective work from images collection according to WOUID. I am calling a function to list the images of each user work. I am using below code to show image for each work in list.
client/modules/userwork/views/worklist.html
<ion-list>
<ion-item ng-repeat="ord in orders track by ord._id" >
<img ng-src="getImgSrc(ord)">
</ion-item>
</ion-list>
code of getImgSrc function is
client/modules/userwork/controllers/userWorkCtrl.js
$scope.getImgSrc = function(wo)
var fetchWOImages = Images.findOne(
"$and":[
WOUID:$exists:true
,
WOUID:wo.WOUID
]
);
return $rootScope.serverUrl+"/cfs/files/images/"+fetchWOImages.getFileRecord()._id+"?store=thumbnail";
;
I am getting inconsistent images sometimes while changing screens between work list, individual work & dashboard and sometimes no images are being shown, for which we have to wait for a long time.
I am thinking that the issue might be due to Images.find() query in getImgSrc function on client side (as given above).
I have tried different ways to fix this issue and below are my findings.
One solution is to use aggregate query but I am using mongodb 3.0 and don't want to upgrade mongodb version for some reason, due to which I can't use aggregate query on server to match images with userwork and return the merged result to client in 'UserWorkOrders' publication. Also aggregate query is not returning any cursor for publication.
Another solution is that I will merge the images with userWork documents in 'UserWorkOrders' publication as shown in below code but I am not getting any data on client side.
Meteor.publish("UserWorkOrders", function()
var criteria = ; //change criteria according to condition
var wos = userWork.find(criteria);
wos.forEach(function(o,i)
var fetchWOImages = Images.find(
"$and":[
WOUID:$exists:true
,
WOUID:o.WOUID
]
).fetch();
o.imgs = fetchWOImages;
);
return wos;
);
So I am looking for something that will help me in getting images(below 1mb size) in much faster and easier way for my above problem.
I will be happy to work for any other alternative ways instead of using collectionFS for my images issue but it should be reactive so that it can work on both online and offline.
Thanks for your efforts and help.
mongodb meteor gridfs angular-meteor collectionfs
mongodb meteor gridfs angular-meteor collectionfs
asked Nov 10 at 10:43
saurabh
841314
841314
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238145%2fangular-meteor-app-online-and-offline-inconsistent-image-using-gridfs%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
A1sO2j,GZj66nBYVzYH,F9YWQ,3J0f2WyzH9Gv toXYq2H0N2klCX F,z h