object message sent with Netty can't be decoded correctly
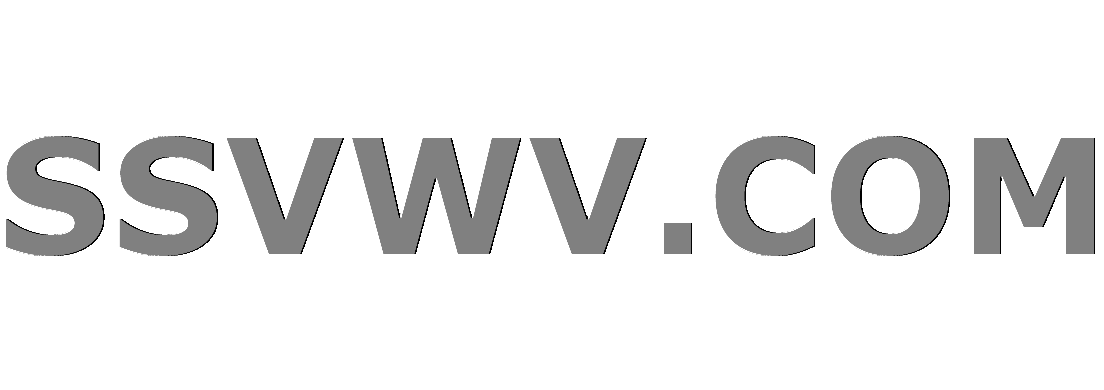
Multi tool use
I try to send object message PingMessage
with netty. Add ObjectEncoder
and ObjectDecoder
in channel pipeline. Client has sent packet successfully, but server can't decode the message. ObjectDecoder#decode() just returns null. Netty version is 4.1.28.Final.
PingMessage
public class PingMessage implements Serializable
private static final long serialVersionUID = 1L;
private int flag = 0xff3e;
private String ping = "ping";
public int getFlag()
return flag;
public void setFlag(int flag)
this.flag = flag;
public String getPing()
return ping;
public void setPing(String ping)
this.ping = ping;
client
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(PongMessage.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception
if (evt instanceof IdleStateEvent)
IdleStateEvent idleState = (IdleStateEvent)evt;
if (idleState.state() == IdleState.READER_IDLE)
else if (idleState.state() == IdleState.WRITER_IDLE)
try
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
catch (Exception e)
e.printStackTrace();
server
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(this.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg)
if (msg instanceof PingMessage)
logger.info("received ping");
return;
netty source code debug info
AbstractChannelHandlerContext#invokeChannelRead()
((ChannelInboundHandler) handler()).channelRead(this, msg);
the msg
variable is
UnpooledUnsafeDirectByteBuf(ridx: 0, widx: 61, cap: 1024)
ObjectDecoder#decode() just return null
super class of ObjectDecoder
is LengthFieldBasedFrameDecoder
. LengthFieldBasedFrameDecoder#decode() return null
// LengthFieldBasedFrameDecoder#decode()
int frameLengthInt = (int) frameLength;
if (in.readableBytes() < frameLengthInt)
return null; // get executed and return null
java netty
|
show 6 more comments
I try to send object message PingMessage
with netty. Add ObjectEncoder
and ObjectDecoder
in channel pipeline. Client has sent packet successfully, but server can't decode the message. ObjectDecoder#decode() just returns null. Netty version is 4.1.28.Final.
PingMessage
public class PingMessage implements Serializable
private static final long serialVersionUID = 1L;
private int flag = 0xff3e;
private String ping = "ping";
public int getFlag()
return flag;
public void setFlag(int flag)
this.flag = flag;
public String getPing()
return ping;
public void setPing(String ping)
this.ping = ping;
client
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(PongMessage.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception
if (evt instanceof IdleStateEvent)
IdleStateEvent idleState = (IdleStateEvent)evt;
if (idleState.state() == IdleState.READER_IDLE)
else if (idleState.state() == IdleState.WRITER_IDLE)
try
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
catch (Exception e)
e.printStackTrace();
server
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(this.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg)
if (msg instanceof PingMessage)
logger.info("received ping");
return;
netty source code debug info
AbstractChannelHandlerContext#invokeChannelRead()
((ChannelInboundHandler) handler()).channelRead(this, msg);
the msg
variable is
UnpooledUnsafeDirectByteBuf(ridx: 0, widx: 61, cap: 1024)
ObjectDecoder#decode() just return null
super class of ObjectDecoder
is LengthFieldBasedFrameDecoder
. LengthFieldBasedFrameDecoder#decode() return null
// LengthFieldBasedFrameDecoder#decode()
int frameLengthInt = (int) frameLength;
if (in.readableBytes() < frameLengthInt)
return null; // get executed and return null
java netty
We will need more infos but just let me say thatChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never callsync*
orawait*
in the EventLoop
– Norman Maurer
Nov 15 '18 at 6:46
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I addsync()
for debug purpose to assure that messages written without exception.
– Hel
Nov 15 '18 at 6:56
1. You didn't add aLengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.
– Ingrim4
Nov 15 '18 at 13:17
1
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29
|
show 6 more comments
I try to send object message PingMessage
with netty. Add ObjectEncoder
and ObjectDecoder
in channel pipeline. Client has sent packet successfully, but server can't decode the message. ObjectDecoder#decode() just returns null. Netty version is 4.1.28.Final.
PingMessage
public class PingMessage implements Serializable
private static final long serialVersionUID = 1L;
private int flag = 0xff3e;
private String ping = "ping";
public int getFlag()
return flag;
public void setFlag(int flag)
this.flag = flag;
public String getPing()
return ping;
public void setPing(String ping)
this.ping = ping;
client
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(PongMessage.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception
if (evt instanceof IdleStateEvent)
IdleStateEvent idleState = (IdleStateEvent)evt;
if (idleState.state() == IdleState.READER_IDLE)
else if (idleState.state() == IdleState.WRITER_IDLE)
try
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
catch (Exception e)
e.printStackTrace();
server
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(this.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg)
if (msg instanceof PingMessage)
logger.info("received ping");
return;
netty source code debug info
AbstractChannelHandlerContext#invokeChannelRead()
((ChannelInboundHandler) handler()).channelRead(this, msg);
the msg
variable is
UnpooledUnsafeDirectByteBuf(ridx: 0, widx: 61, cap: 1024)
ObjectDecoder#decode() just return null
super class of ObjectDecoder
is LengthFieldBasedFrameDecoder
. LengthFieldBasedFrameDecoder#decode() return null
// LengthFieldBasedFrameDecoder#decode()
int frameLengthInt = (int) frameLength;
if (in.readableBytes() < frameLengthInt)
return null; // get executed and return null
java netty
I try to send object message PingMessage
with netty. Add ObjectEncoder
and ObjectDecoder
in channel pipeline. Client has sent packet successfully, but server can't decode the message. ObjectDecoder#decode() just returns null. Netty version is 4.1.28.Final.
PingMessage
public class PingMessage implements Serializable
private static final long serialVersionUID = 1L;
private int flag = 0xff3e;
private String ping = "ping";
public int getFlag()
return flag;
public void setFlag(int flag)
this.flag = flag;
public String getPing()
return ping;
public void setPing(String ping)
this.ping = ping;
client
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(PongMessage.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception
if (evt instanceof IdleStateEvent)
IdleStateEvent idleState = (IdleStateEvent)evt;
if (idleState.state() == IdleState.READER_IDLE)
else if (idleState.state() == IdleState.WRITER_IDLE)
try
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
catch (Exception e)
e.printStackTrace();
server
public class HeartbeatInitializer extends ChannelInitializer<Channel>
protected void initChannel(Channel ch) throws Exception
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.weakCachingResolver(this.class.getClassLoader())));
pipeline.addLast(new IdleStateHandler(BusinessConstant.READER_IDLE_SECONDS, BusinessConstant.WRITER_IDLE_SECONDS, 0));
pipeline.addLast(new HeartbeatServerHandler());
pipeline.addLast(new ClientHandler());
public class HeartbeatServerHandler extends ChannelDuplexHandler
private static Logger logger = LoggerFactory.getLogger(HeartbeatServerHandler.class);
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg)
if (msg instanceof PingMessage)
logger.info("received ping");
return;
netty source code debug info
AbstractChannelHandlerContext#invokeChannelRead()
((ChannelInboundHandler) handler()).channelRead(this, msg);
the msg
variable is
UnpooledUnsafeDirectByteBuf(ridx: 0, widx: 61, cap: 1024)
ObjectDecoder#decode() just return null
super class of ObjectDecoder
is LengthFieldBasedFrameDecoder
. LengthFieldBasedFrameDecoder#decode() return null
// LengthFieldBasedFrameDecoder#decode()
int frameLengthInt = (int) frameLength;
if (in.readableBytes() < frameLengthInt)
return null; // get executed and return null
java netty
java netty
edited Nov 15 '18 at 15:16
Hel
asked Nov 15 '18 at 1:24
HelHel
10419
10419
We will need more infos but just let me say thatChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never callsync*
orawait*
in the EventLoop
– Norman Maurer
Nov 15 '18 at 6:46
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I addsync()
for debug purpose to assure that messages written without exception.
– Hel
Nov 15 '18 at 6:56
1. You didn't add aLengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.
– Ingrim4
Nov 15 '18 at 13:17
1
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29
|
show 6 more comments
We will need more infos but just let me say thatChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never callsync*
orawait*
in the EventLoop
– Norman Maurer
Nov 15 '18 at 6:46
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I addsync()
for debug purpose to assure that messages written without exception.
– Hel
Nov 15 '18 at 6:56
1. You didn't add aLengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.
– Ingrim4
Nov 15 '18 at 13:17
1
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29
We will need more infos but just let me say that
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never call sync*
or await*
in the EventLoop– Norman Maurer
Nov 15 '18 at 6:46
We will need more infos but just let me say that
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never call sync*
or await*
in the EventLoop– Norman Maurer
Nov 15 '18 at 6:46
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I add
sync()
for debug purpose to assure that messages written without exception.– Hel
Nov 15 '18 at 6:56
@NormanMaurer I add
sync()
for debug purpose to assure that messages written without exception.– Hel
Nov 15 '18 at 6:56
1. You didn't add a
LengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.– Ingrim4
Nov 15 '18 at 13:17
1. You didn't add a
LengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.– Ingrim4
Nov 15 '18 at 13:17
1
1
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29
|
show 6 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311147%2fobject-message-sent-with-netty-cant-be-decoded-correctly%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311147%2fobject-message-sent-with-netty-cant-be-decoded-correctly%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hWd,hlrOYRchjeCdm1G97F4U,n1HvxoFZjGDbb38EXDCWVvP0mQAx4SHlCTb2YCmOHC4ySFEnDm
We will need more infos but just let me say that
ChannelFuture future = ctx.writeAndFlush(new PingMessage()).sync();
will not work (and should even produce an exception) as you block the EventLoop. You should never callsync*
orawait*
in the EventLoop– Norman Maurer
Nov 15 '18 at 6:46
@NormanMaurer I've debugged the client code and no exception found. I use wireshark to capture packets and find "ping" packet which is 61 bytes. But the server just can't decode bytes to PingMessage. How can I provide more infomation? I don't post code about PongMessage(from server to client), which can be decoded well by client. Does Netty support both directions message processing?
– Hel
Nov 15 '18 at 6:49
@NormanMaurer I add
sync()
for debug purpose to assure that messages written without exception.– Hel
Nov 15 '18 at 6:56
1. You didn't add a
LengthFieldBasedFrameDecoder
in your ChannelInitializer but mentioned it in your question. Why? 2. I tried your code and for me everything woks fine. Maybe you imported something wrong since all your classes have the same name.– Ingrim4
Nov 15 '18 at 13:17
1
Are you sure you have the same version of the serializable class on both class-path. ?
– Norman Maurer
Nov 16 '18 at 16:29