how to initialize final variable boolean array in method void
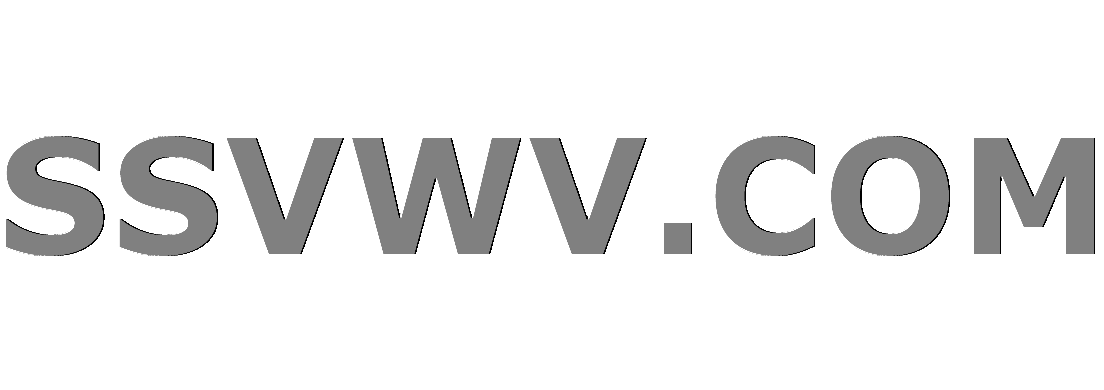
Multi tool use
my application will check whether the play protect settings are activated or not.
public static boolean checkGooglePlayProtectSettings(Context context)
final boolean enabled = new booleanfalse;
try
SafetyNet.getClient(context).isVerifyAppsEnabled().addOnCompleteListener(new OnCompleteListener<SafetyNetApi.VerifyAppsUserResponse>()
@Override
public void onComplete(@NonNull Task<SafetyNetApi.VerifyAppsUserResponse> task)
if(task.isSuccessful())
SafetyNetApi.VerifyAppsUserResponse result = task.getResult();
if(result != null)
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
else
Log.e("GoogleProtectPermission", "A general error occurred.");
);
catch (Exception e)
e.printStackTrace();
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
return false;
in this case I tried the final results to log, but always not active in the log results, how to get good results to check whether play prtoect is active or not?


add a comment |
my application will check whether the play protect settings are activated or not.
public static boolean checkGooglePlayProtectSettings(Context context)
final boolean enabled = new booleanfalse;
try
SafetyNet.getClient(context).isVerifyAppsEnabled().addOnCompleteListener(new OnCompleteListener<SafetyNetApi.VerifyAppsUserResponse>()
@Override
public void onComplete(@NonNull Task<SafetyNetApi.VerifyAppsUserResponse> task)
if(task.isSuccessful())
SafetyNetApi.VerifyAppsUserResponse result = task.getResult();
if(result != null)
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
else
Log.e("GoogleProtectPermission", "A general error occurred.");
);
catch (Exception e)
e.printStackTrace();
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
return false;
in this case I tried the final results to log, but always not active in the log results, how to get good results to check whether play prtoect is active or not?


add a comment |
my application will check whether the play protect settings are activated or not.
public static boolean checkGooglePlayProtectSettings(Context context)
final boolean enabled = new booleanfalse;
try
SafetyNet.getClient(context).isVerifyAppsEnabled().addOnCompleteListener(new OnCompleteListener<SafetyNetApi.VerifyAppsUserResponse>()
@Override
public void onComplete(@NonNull Task<SafetyNetApi.VerifyAppsUserResponse> task)
if(task.isSuccessful())
SafetyNetApi.VerifyAppsUserResponse result = task.getResult();
if(result != null)
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
else
Log.e("GoogleProtectPermission", "A general error occurred.");
);
catch (Exception e)
e.printStackTrace();
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
return false;
in this case I tried the final results to log, but always not active in the log results, how to get good results to check whether play prtoect is active or not?


my application will check whether the play protect settings are activated or not.
public static boolean checkGooglePlayProtectSettings(Context context)
final boolean enabled = new booleanfalse;
try
SafetyNet.getClient(context).isVerifyAppsEnabled().addOnCompleteListener(new OnCompleteListener<SafetyNetApi.VerifyAppsUserResponse>()
@Override
public void onComplete(@NonNull Task<SafetyNetApi.VerifyAppsUserResponse> task)
if(task.isSuccessful())
SafetyNetApi.VerifyAppsUserResponse result = task.getResult();
if(result != null)
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
else
Log.e("GoogleProtectPermission", "A general error occurred.");
);
catch (Exception e)
e.printStackTrace();
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
return false;
in this case I tried the final results to log, but always not active in the log results, how to get good results to check whether play prtoect is active or not?




asked Nov 15 '18 at 4:20
Robby RamadhanRobby Ramadhan
1
1
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The isVerifyAppsEnabled()
method is asynchronous, it calls the OnCompleteListener
's onComplete
method to notify when the result is ready.
It's likely that this block is executed before the OnCompleteListener
is fired, so the enabled[0]
value remains the same at this point.
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
Since there's no synchronous method to get the result, you'll probably have to change your approach someway to take account for this asynchronicity and perform dependent operations when the callback results are ready or (highly uncrecommended) perform a blocking wait until the Task
returned by isVerifyAppsEnabled
is completed.
Also, disregarding the question, in your current code there seems to be little point in using boolean array instead of just a boolean for this, and this
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
can be simplified to this enabled[0] = result.isVerifyAppsEnabled()
add a comment |
You could do like this:
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
...
if(sb.toString().equals("good"))
Log.d("GoogleProtectPermission", "Is enabled");
if(sb.toString().equals("bad"))
Log.d("GoogleProtectPermission", "Is disabled");
Edited:
Yes, as comment points, My answer is wrong. so there are two ways:
1 directly output log info
if(result.isVerifyAppsEnabled())
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
2 using interface callback
public interface Callback //add an interface
void onResponse(String message);
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
callback.onResponse(sb.toString()); //add this line
and subscribe the interface in some place.
That won't work due to asynchronous nature of theisVerifyAppsEnabled()
method. And usingString
s andStringBuilder
s instead ofboolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.
– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312392%2fhow-to-initialize-final-variable-boolean-array-in-method-void%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The isVerifyAppsEnabled()
method is asynchronous, it calls the OnCompleteListener
's onComplete
method to notify when the result is ready.
It's likely that this block is executed before the OnCompleteListener
is fired, so the enabled[0]
value remains the same at this point.
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
Since there's no synchronous method to get the result, you'll probably have to change your approach someway to take account for this asynchronicity and perform dependent operations when the callback results are ready or (highly uncrecommended) perform a blocking wait until the Task
returned by isVerifyAppsEnabled
is completed.
Also, disregarding the question, in your current code there seems to be little point in using boolean array instead of just a boolean for this, and this
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
can be simplified to this enabled[0] = result.isVerifyAppsEnabled()
add a comment |
The isVerifyAppsEnabled()
method is asynchronous, it calls the OnCompleteListener
's onComplete
method to notify when the result is ready.
It's likely that this block is executed before the OnCompleteListener
is fired, so the enabled[0]
value remains the same at this point.
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
Since there's no synchronous method to get the result, you'll probably have to change your approach someway to take account for this asynchronicity and perform dependent operations when the callback results are ready or (highly uncrecommended) perform a blocking wait until the Task
returned by isVerifyAppsEnabled
is completed.
Also, disregarding the question, in your current code there seems to be little point in using boolean array instead of just a boolean for this, and this
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
can be simplified to this enabled[0] = result.isVerifyAppsEnabled()
add a comment |
The isVerifyAppsEnabled()
method is asynchronous, it calls the OnCompleteListener
's onComplete
method to notify when the result is ready.
It's likely that this block is executed before the OnCompleteListener
is fired, so the enabled[0]
value remains the same at this point.
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
Since there's no synchronous method to get the result, you'll probably have to change your approach someway to take account for this asynchronicity and perform dependent operations when the callback results are ready or (highly uncrecommended) perform a blocking wait until the Task
returned by isVerifyAppsEnabled
is completed.
Also, disregarding the question, in your current code there seems to be little point in using boolean array instead of just a boolean for this, and this
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
can be simplified to this enabled[0] = result.isVerifyAppsEnabled()
The isVerifyAppsEnabled()
method is asynchronous, it calls the OnCompleteListener
's onComplete
method to notify when the result is ready.
It's likely that this block is executed before the OnCompleteListener
is fired, so the enabled[0]
value remains the same at this point.
if (enabled[0])
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
Since there's no synchronous method to get the result, you'll probably have to change your approach someway to take account for this asynchronicity and perform dependent operations when the callback results are ready or (highly uncrecommended) perform a blocking wait until the Task
returned by isVerifyAppsEnabled
is completed.
Also, disregarding the question, in your current code there seems to be little point in using boolean array instead of just a boolean for this, and this
if(result.isVerifyAppsEnabled())
enabled[0] = true;
else
enabled[0] = false;
can be simplified to this enabled[0] = result.isVerifyAppsEnabled()
answered Nov 15 '18 at 5:17


Mikhail OlshanskiMikhail Olshanski
44849
44849
add a comment |
add a comment |
You could do like this:
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
...
if(sb.toString().equals("good"))
Log.d("GoogleProtectPermission", "Is enabled");
if(sb.toString().equals("bad"))
Log.d("GoogleProtectPermission", "Is disabled");
Edited:
Yes, as comment points, My answer is wrong. so there are two ways:
1 directly output log info
if(result.isVerifyAppsEnabled())
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
2 using interface callback
public interface Callback //add an interface
void onResponse(String message);
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
callback.onResponse(sb.toString()); //add this line
and subscribe the interface in some place.
That won't work due to asynchronous nature of theisVerifyAppsEnabled()
method. And usingString
s andStringBuilder
s instead ofboolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.
– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
add a comment |
You could do like this:
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
...
if(sb.toString().equals("good"))
Log.d("GoogleProtectPermission", "Is enabled");
if(sb.toString().equals("bad"))
Log.d("GoogleProtectPermission", "Is disabled");
Edited:
Yes, as comment points, My answer is wrong. so there are two ways:
1 directly output log info
if(result.isVerifyAppsEnabled())
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
2 using interface callback
public interface Callback //add an interface
void onResponse(String message);
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
callback.onResponse(sb.toString()); //add this line
and subscribe the interface in some place.
That won't work due to asynchronous nature of theisVerifyAppsEnabled()
method. And usingString
s andStringBuilder
s instead ofboolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.
– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
add a comment |
You could do like this:
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
...
if(sb.toString().equals("good"))
Log.d("GoogleProtectPermission", "Is enabled");
if(sb.toString().equals("bad"))
Log.d("GoogleProtectPermission", "Is disabled");
Edited:
Yes, as comment points, My answer is wrong. so there are two ways:
1 directly output log info
if(result.isVerifyAppsEnabled())
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
2 using interface callback
public interface Callback //add an interface
void onResponse(String message);
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
callback.onResponse(sb.toString()); //add this line
and subscribe the interface in some place.
You could do like this:
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
...
if(sb.toString().equals("good"))
Log.d("GoogleProtectPermission", "Is enabled");
if(sb.toString().equals("bad"))
Log.d("GoogleProtectPermission", "Is disabled");
Edited:
Yes, as comment points, My answer is wrong. so there are two ways:
1 directly output log info
if(result.isVerifyAppsEnabled())
Log.d("GoogleProtectPermission", "Is enabled");
else
Log.d("GoogleProtectPermission", "Is disabled");
2 using interface callback
public interface Callback //add an interface
void onResponse(String message);
final StringBuilder sb = new StringBuilder();
...
if(result.isVerifyAppsEnabled())
sb.append("good");
else
sb.append("bad");
callback.onResponse(sb.toString()); //add this line
and subscribe the interface in some place.
edited Nov 15 '18 at 5:38
answered Nov 15 '18 at 5:17
navylovernavylover
3,51531119
3,51531119
That won't work due to asynchronous nature of theisVerifyAppsEnabled()
method. And usingString
s andStringBuilder
s instead ofboolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.
– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
add a comment |
That won't work due to asynchronous nature of theisVerifyAppsEnabled()
method. And usingString
s andStringBuilder
s instead ofboolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.
– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
That won't work due to asynchronous nature of the
isVerifyAppsEnabled()
method. And using String
s and StringBuilder
s instead of boolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.– Mikhail Olshanski
Nov 15 '18 at 5:30
That won't work due to asynchronous nature of the
isVerifyAppsEnabled()
method. And using String
s and StringBuilder
s instead of boolean
values is a bad practice, it creates a lot of unnecessary objects, performs a lot of unneeded operations, lacks type safety and compile checks and it shouldn't be done at all. There is, in fact a term for this - Stringly Typed.– Mikhail Olshanski
Nov 15 '18 at 5:30
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
@MikhailOlshanski yes, you are right, I modify answer, thank you!
– navylover
Nov 15 '18 at 5:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312392%2fhow-to-initialize-final-variable-boolean-array-in-method-void%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W LNmZ18X7vjVLu 4JpJzL Ax6ur,Sgl qOAcz,ol