How to create a Real-time graph or Histogram based on Python OpenCV?
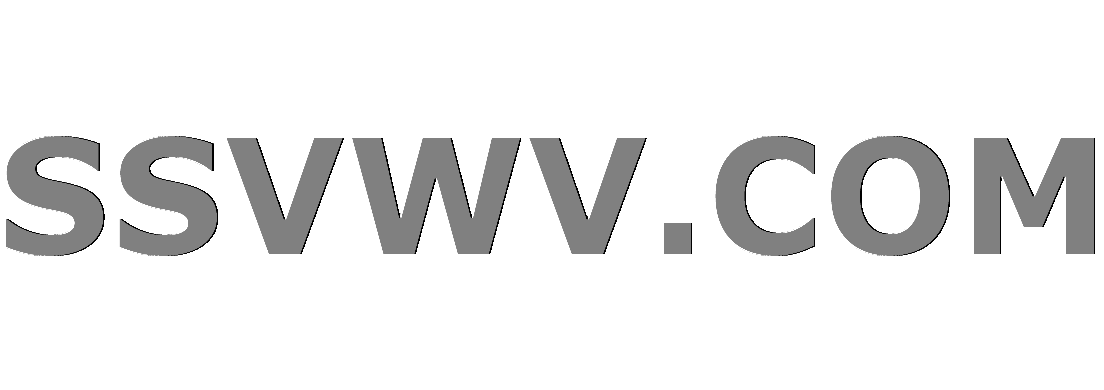
Multi tool use
My color detection code detects some colors while also providing the percentage value of the detected color. However I would like to create a more visual based output like a histogram or graph so I would like any advice in how to create one. I've searched in some forums but most are for still images.
My code is below:
#importing modules
import cv2
import numpy as np
import time
import matplotlib.pyplot as plt
#capturing video through webcam
cap=cv2.VideoCapture(0)
while(1):
_, img = cap.read()
#converting frame(img i.e BGR) to HSV (hue-saturation-value)
hsv=cv2.cvtColor(img,cv2.COLOR_BGR2HSV)
#definig the range of red color
red_lower=np.array([166,84,141],np.uint8)
red_upper=np.array([186,255,255],np.uint8)
#definig the range of dark red color
dred_lower=np.array([0,180,80],np.uint8)
dred_upper=np.array([6,255,141],np.uint8)
#defining the range of pink color
pink_lower=np.array([137,17,130],np.uint8)
pink_upper=np.array([150,165,255],np.uint8)
#defining the Range of cream color
cream_lower=np.array([0,5,168],np.uint8)
cream_upper=np.array([33,113,255],np.uint8)
#defining the Range of Blue color
blue_lower=np.array([97,100,80],np.uint8)
blue_upper=np.array([117,255,180],np.uint8)
#defining the Range of light Blue color
lblue_lower=np.array([100,56.1,142.8],np.uint8)
lblue_upper=np.array([100,70,155.5],np.uint8)
#defining the Range of cyan color
cyan_lower=np.array([90,200,200],np.uint8)
cyan_upper=np.array([90,255,255],np.uint8)
#defining the Range of purple color
purple_lower=np.array([128,40,125],np.uint8)
purple_upper=np.array([138,255,255],np.uint8)
#defining the Range of yellow color
yellow_lower=np.array([24,45,110],np.uint8)
yellow_upper=np.array([30,255,255],np.uint8)
#defining the Range of green color
green_lower=np.array([45,59,119],np.uint8)
green_upper=np.array([68,255,255],np.uint8)
#defining the Range of White color
White_lower=np.array([0,0,200],np.uint8)
White_upper=np.array([180,255,255],np.uint8)
#defining the range of grey color
grey_lower=np.array([106,5,168],np.uint8)
grey_upper=np.array([120,75,255],np.uint8)
#defining the Range of orange color
orange_lower=np.array([15,30,60],np.uint8)
orange_upper=np.array([15,255,255],np.uint8)
#defining the range of offwhite color
offwhite_lower=np.array([0,0,168],np.uint8)
offwhite_upper=np.array([0,0,210],np.uint8)
#defining the Range of black color
Black_lower=np.array([0,0,0],np.uint8)
Black_upper=np.array([180,255,40],np.uint8)
#defining the Range of brown color
Brown_lower=np.array([128,100,150],np.uint8)
Brown_upper=np.array([160,150,255],np.uint8)
#defining the range of beige color
beige_lower=np.array([10,50,180],np.uint8)
beige_upper=np.array([100,255,255],np.uint8)
#finding the range of the colors in the image
red=cv2.inRange(hsv, red_lower, red_upper)
pink=cv2.inRange(hsv,pink_lower,pink_upper)
cream=cv2.inRange(hsv,cream_lower,cream_upper)
dred=cv2.inRange(hsv, dred_lower, dred_upper)
blue=cv2.inRange(hsv,blue_lower,blue_upper)
lblue=cv2.inRange(hsv,lblue_lower,lblue_upper)
cyan=cv2.inRange(hsv,cyan_lower,cyan_upper)
yellow=cv2.inRange(hsv,yellow_lower,yellow_upper)
green=cv2.inRange(hsv,green_lower,green_upper)
purple=cv2.inRange(hsv,purple_lower,purple_upper)
orange=cv2.inRange(hsv,orange_lower,orange_upper)
White=cv2.inRange(hsv,White_lower,White_upper)
offwhite=cv2.inRange(hsv,offwhite_lower,offwhite_upper)
Black=cv2.inRange(hsv,Black_lower,Black_upper)
grey=cv2.inRange(hsv,grey_lower,grey_upper)
brown=cv2.inRange(hsv,Brown_lower,Brown_upper)
beige=cv2.inRange(hsv,beige_lower,beige_upper)
#Morphological transformation, Dilation
kernal = np.ones((5 ,5), "uint8")
red=cv2.dilate(red, kernal)
res=cv2.bitwise_and(img, img, mask = red)
pink=cv2.dilate(pink,kernal)
res1=cv2.bitwise_and(img, img, mask = pink)
cream=cv2.dilate(cream,kernal)
res1=cv2.bitwise_and(img, img, mask = cream)
dred=cv2.dilate(dred, kernal)
res=cv2.bitwise_and(img, img, mask = dred)
blue=cv2.dilate(blue,kernal)
res1=cv2.bitwise_and(img, img, mask = blue)
lblue=cv2.dilate(lblue,kernal)
res1=cv2.bitwise_and(img, img, mask = lblue)
cyan=cv2.dilate(cyan,kernal)
res1=cv2.bitwise_and(img, img, mask = cyan)
yellow=cv2.dilate(yellow,kernal)
res2=cv2.bitwise_and(img, img, mask = yellow)
purple=cv2.dilate(purple, kernal)
res2=cv2.bitwise_and(img, img, mask = purple)
green=cv2.dilate(green,kernal)
res2=cv2.bitwise_and(img, img, mask = green)
orange=cv2.dilate(orange,kernal)
res2=cv2.bitwise_and(img, img, mask = orange)
White=cv2.dilate(White,kernal)
res2=cv2.bitwise_and(img, img, mask = White)
offwhite=cv2.dilate(offwhite, kernal)
res2=cv2.bitwise_and(img, img, mask = offwhite)
Black=cv2.dilate(Black,kernal)
res2=cv2.bitwise_and(img, img, mask = Black)
grey=cv2.dilate(grey, kernal)
res2=cv2.bitwise_and(img, img, mask = grey)
brown=cv2.dilate(brown,kernal)
res2=cv2.bitwise_and(img, img, mask = brown)
beige=cv2.dilate(beige, kernal)
res2=cv2.bitwise_and(img, img, mask = beige)
#Tracking the Red Color
(_,contours,hierarchy)=cv2.findContours(red,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,255),2)
cv2.putText(img,"RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,255))
#Tracking the dark Red Color
(_,contours,hierarchy)=cv2.findContours(dred,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,139),2)
cv2.putText(img,"Dark RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,139))
#Tracking the pink Color
(_,contours,hierarchy)=cv2.findContours(pink,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,255),2)
cv2.putText(img,"Pink color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,255))
#Tracking the cream Color
(_,contours,hierarchy)=cv2.findContours(cream,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(150,255,255),2)
cv2.putText(img,"cream color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (150,255,255))
#Tracking the light Blue Color
(_,contours,hierarchy)=cv2.findContours(lblue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(180,0,0),2)
cv2.putText(img,"light blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (180,0,0))
#Tracking the Blue Color
(_,contours,hierarchy)=cv2.findContours(blue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
cv2.putText(img,"Blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0))
#Tracking the cyan Color
(_,contours,hierarchy)=cv2.findContours(cyan,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,0),2)
cv2.putText(img,"cyan color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,255,0))
#Tracking the yellow Color
(_,contours,hierarchy)=cv2.findContours(yellow,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,217),2)
cv2.putText(img,"yellow color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,217))
#Tracking the purple Color
(_,contours,hierarchy)=cv2.findContours(purple,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,0,128),2)
cv2.putText(img,"Purple color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,0,128))
#Tracking the green Color
(_,contours,hierarchy)=cv2.findContours(green,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,0),2)
cv2.putText(img,"green color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,0))
#Tracking the orange Color
(_,contours,hierarchy)=cv2.findContours(orange,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,140,255),2)
cv2.putText(img,"orange color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,140,255))
#Tracking the White Color
(_,contours,hierarchy)=cv2.findContours(White,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,255),2)
cv2.putText(img,"White color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (255,255,255))
#Tracking the offwhite Color
(_,contours,hierarchy)=cv2.findContours(offwhite,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,128,128),2)
cv2.putText(img,"Offwhite color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,128,128))
#Tracking the black Color
(_,contours,hierarchy)=cv2.findContours(Black,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,0),2)
cv2.putText(img,"black color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,0,0))
#Tracking the grey Color
(_,contours,hierarchy)=cv2.findContours(grey,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(220,220,220),2)
cv2.putText(img,"Grey color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (220,220,220))
#Tracking the brown Color
(_,contours,hierarchy)=cv2.findContours(brown,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(33,67,101),2)
cv2.putText(img,"brown color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (33,67,101))
#Tracking the beige Color
(_,contours,hierarchy)=cv2.findContours(beige,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(126,169,230),2)
cv2.putText(img,"Beige color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (126,169,236))
#Show red percentage
red_per = red
Total_image = (blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Red = np.mean((red_per/Total_image)*100)
print('Percentage of red color',Per_Red)
#Show blue percentage
blue_per = blue
Total_image2 = (blue+red+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Blue = np.mean((blue_per/Total_image2)*100)
print('Percentage blue',Per_Blue)
#Show green percentage
green_per = green
Total_image3 = (green+blue+yellow+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Green = np.mean((green_per/Total_image3)*100)
print('Percentage green',Per_Green)
#Show yellow percentage
yellow_per = yellow
Total_image4 = (yellow+blue+red+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Yellow = np.mean((yellow_per/Total_image4)*100)
print('Percentage yellow',Per_Yellow)
#Show dark red percentage
dred_per = dred
Total_image = (dred+blue+yellow+green+red+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_dR = np.mean((dred_per/Total_image)*100)
print('Percentage of dark red color',Per_dR)
#Show Cream percentage
Cream_per = cream
Total_image2 = (cream+blue+yellow+green+dred+White+Black+offwhite+red+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Cream = np.mean((Cream_per/Total_image2)*100)
print('Percentage of cream color',Per_Cream)
#Show lblue percentage
lblue_per = lblue
Total_image3 = (lblue+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+red+beige+brown+grey+orange+cyan)
Per_lBlue = np.mean((lblue_per/Total_image3)*100)
print('Percentage of light blue color',Per_lBlue)
#Show White percentage
White_per = White
Total_image4 = (White+blue+yellow+green+dred+red+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_White = np.mean((White_per/Total_image4)*100)
print('Percentage of white color',Per_White)
#Show orange percentage
orange_per = orange
Total_image = (orange+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+red+cyan)
Per_Orange = np.mean((orange_per/Total_image)*100)
print('Percentage of orange color',Per_Orange)
#Show purple percentage
purple_per = purple
Total_image2 = (purple+blue+yellow+green+dred+White+Black+offwhite+cream+pink+red+lblue+beige+brown+grey+orange+cyan)
Per_Purple = np.mean((purple_per/Total_image2)*100)
print('Percentage of purple color',Per_Purple)
#Show pink percentage
pink_per = pink
Total_image3 = (pink+blue+yellow+green+dred+White+Black+offwhite+cream+red+purple+lblue+beige+brown+grey+orange+cyan)
Per_Pink = np.mean((pink_per/Total_image3)*100)
print('Percentage of pink color',Per_Pink)
#Show cyan percentage
cyan_per = cyan
Total_image4 = (cyan+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+red)
Per_Cyan = np.mean((cyan_per/Total_image4)*100)
print('Percentage of cyan color',Per_Cyan)
#Show grey percentage
grey_per = grey
Total_image = (grey+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+red+orange+cyan)
Per_Grey = np.mean((grey_per/Total_image)*100)
print('Percentage of grey color',Per_Grey)
#Show black percentage
black_per = Black
Total_image1 = (Black+blue+yellow+green+dred+White+red+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Black = np.mean((black_per/Total_image1)*100)
print('Percentage of black color',Per_Black)
#Show beige percentage
beige_per = beige
Total_image2 = (beige+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+red+brown+grey+orange+cyan)
Per_Beige = np.mean((beige_per/Total_image2)*100)
print('Percentage of beige',Per_Beige)
#Show brown percentage
brown_per = brown
Total_image3 = (brown+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+red+grey+orange+cyan)
Per_Brown = np.mean((brown_per/Total_image3)*100)
print('Percentage of brown color',Per_Brown)
#Show offwhite percentage
offwhite_per = offwhite
Total_image4 = (offwhite+blue+yellow+green+dred+White+Black+red+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Offwhite = np.mean((offwhite_per/Total_image4)*100)
print('Percentage of offwhite',Per_Offwhite)
cv2.imshow("Color Tracking",img)
if cv2.waitKey(10) & 0xFF == ord('q'):
cap.release()
cv2.destroyAllWindows()
break
What I'm trying to achieve to the code to present a histogram where it shows the y-axis (0-100)% and x-axis(the colors that will be detected) and shows how the data is changing when more colors are being detected and how the values fluctuates as more and more colors are being detected.
Thank you in advance!
python opencv colors histogram live-streaming
add a comment |
My color detection code detects some colors while also providing the percentage value of the detected color. However I would like to create a more visual based output like a histogram or graph so I would like any advice in how to create one. I've searched in some forums but most are for still images.
My code is below:
#importing modules
import cv2
import numpy as np
import time
import matplotlib.pyplot as plt
#capturing video through webcam
cap=cv2.VideoCapture(0)
while(1):
_, img = cap.read()
#converting frame(img i.e BGR) to HSV (hue-saturation-value)
hsv=cv2.cvtColor(img,cv2.COLOR_BGR2HSV)
#definig the range of red color
red_lower=np.array([166,84,141],np.uint8)
red_upper=np.array([186,255,255],np.uint8)
#definig the range of dark red color
dred_lower=np.array([0,180,80],np.uint8)
dred_upper=np.array([6,255,141],np.uint8)
#defining the range of pink color
pink_lower=np.array([137,17,130],np.uint8)
pink_upper=np.array([150,165,255],np.uint8)
#defining the Range of cream color
cream_lower=np.array([0,5,168],np.uint8)
cream_upper=np.array([33,113,255],np.uint8)
#defining the Range of Blue color
blue_lower=np.array([97,100,80],np.uint8)
blue_upper=np.array([117,255,180],np.uint8)
#defining the Range of light Blue color
lblue_lower=np.array([100,56.1,142.8],np.uint8)
lblue_upper=np.array([100,70,155.5],np.uint8)
#defining the Range of cyan color
cyan_lower=np.array([90,200,200],np.uint8)
cyan_upper=np.array([90,255,255],np.uint8)
#defining the Range of purple color
purple_lower=np.array([128,40,125],np.uint8)
purple_upper=np.array([138,255,255],np.uint8)
#defining the Range of yellow color
yellow_lower=np.array([24,45,110],np.uint8)
yellow_upper=np.array([30,255,255],np.uint8)
#defining the Range of green color
green_lower=np.array([45,59,119],np.uint8)
green_upper=np.array([68,255,255],np.uint8)
#defining the Range of White color
White_lower=np.array([0,0,200],np.uint8)
White_upper=np.array([180,255,255],np.uint8)
#defining the range of grey color
grey_lower=np.array([106,5,168],np.uint8)
grey_upper=np.array([120,75,255],np.uint8)
#defining the Range of orange color
orange_lower=np.array([15,30,60],np.uint8)
orange_upper=np.array([15,255,255],np.uint8)
#defining the range of offwhite color
offwhite_lower=np.array([0,0,168],np.uint8)
offwhite_upper=np.array([0,0,210],np.uint8)
#defining the Range of black color
Black_lower=np.array([0,0,0],np.uint8)
Black_upper=np.array([180,255,40],np.uint8)
#defining the Range of brown color
Brown_lower=np.array([128,100,150],np.uint8)
Brown_upper=np.array([160,150,255],np.uint8)
#defining the range of beige color
beige_lower=np.array([10,50,180],np.uint8)
beige_upper=np.array([100,255,255],np.uint8)
#finding the range of the colors in the image
red=cv2.inRange(hsv, red_lower, red_upper)
pink=cv2.inRange(hsv,pink_lower,pink_upper)
cream=cv2.inRange(hsv,cream_lower,cream_upper)
dred=cv2.inRange(hsv, dred_lower, dred_upper)
blue=cv2.inRange(hsv,blue_lower,blue_upper)
lblue=cv2.inRange(hsv,lblue_lower,lblue_upper)
cyan=cv2.inRange(hsv,cyan_lower,cyan_upper)
yellow=cv2.inRange(hsv,yellow_lower,yellow_upper)
green=cv2.inRange(hsv,green_lower,green_upper)
purple=cv2.inRange(hsv,purple_lower,purple_upper)
orange=cv2.inRange(hsv,orange_lower,orange_upper)
White=cv2.inRange(hsv,White_lower,White_upper)
offwhite=cv2.inRange(hsv,offwhite_lower,offwhite_upper)
Black=cv2.inRange(hsv,Black_lower,Black_upper)
grey=cv2.inRange(hsv,grey_lower,grey_upper)
brown=cv2.inRange(hsv,Brown_lower,Brown_upper)
beige=cv2.inRange(hsv,beige_lower,beige_upper)
#Morphological transformation, Dilation
kernal = np.ones((5 ,5), "uint8")
red=cv2.dilate(red, kernal)
res=cv2.bitwise_and(img, img, mask = red)
pink=cv2.dilate(pink,kernal)
res1=cv2.bitwise_and(img, img, mask = pink)
cream=cv2.dilate(cream,kernal)
res1=cv2.bitwise_and(img, img, mask = cream)
dred=cv2.dilate(dred, kernal)
res=cv2.bitwise_and(img, img, mask = dred)
blue=cv2.dilate(blue,kernal)
res1=cv2.bitwise_and(img, img, mask = blue)
lblue=cv2.dilate(lblue,kernal)
res1=cv2.bitwise_and(img, img, mask = lblue)
cyan=cv2.dilate(cyan,kernal)
res1=cv2.bitwise_and(img, img, mask = cyan)
yellow=cv2.dilate(yellow,kernal)
res2=cv2.bitwise_and(img, img, mask = yellow)
purple=cv2.dilate(purple, kernal)
res2=cv2.bitwise_and(img, img, mask = purple)
green=cv2.dilate(green,kernal)
res2=cv2.bitwise_and(img, img, mask = green)
orange=cv2.dilate(orange,kernal)
res2=cv2.bitwise_and(img, img, mask = orange)
White=cv2.dilate(White,kernal)
res2=cv2.bitwise_and(img, img, mask = White)
offwhite=cv2.dilate(offwhite, kernal)
res2=cv2.bitwise_and(img, img, mask = offwhite)
Black=cv2.dilate(Black,kernal)
res2=cv2.bitwise_and(img, img, mask = Black)
grey=cv2.dilate(grey, kernal)
res2=cv2.bitwise_and(img, img, mask = grey)
brown=cv2.dilate(brown,kernal)
res2=cv2.bitwise_and(img, img, mask = brown)
beige=cv2.dilate(beige, kernal)
res2=cv2.bitwise_and(img, img, mask = beige)
#Tracking the Red Color
(_,contours,hierarchy)=cv2.findContours(red,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,255),2)
cv2.putText(img,"RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,255))
#Tracking the dark Red Color
(_,contours,hierarchy)=cv2.findContours(dred,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,139),2)
cv2.putText(img,"Dark RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,139))
#Tracking the pink Color
(_,contours,hierarchy)=cv2.findContours(pink,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,255),2)
cv2.putText(img,"Pink color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,255))
#Tracking the cream Color
(_,contours,hierarchy)=cv2.findContours(cream,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(150,255,255),2)
cv2.putText(img,"cream color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (150,255,255))
#Tracking the light Blue Color
(_,contours,hierarchy)=cv2.findContours(lblue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(180,0,0),2)
cv2.putText(img,"light blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (180,0,0))
#Tracking the Blue Color
(_,contours,hierarchy)=cv2.findContours(blue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
cv2.putText(img,"Blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0))
#Tracking the cyan Color
(_,contours,hierarchy)=cv2.findContours(cyan,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,0),2)
cv2.putText(img,"cyan color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,255,0))
#Tracking the yellow Color
(_,contours,hierarchy)=cv2.findContours(yellow,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,217),2)
cv2.putText(img,"yellow color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,217))
#Tracking the purple Color
(_,contours,hierarchy)=cv2.findContours(purple,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,0,128),2)
cv2.putText(img,"Purple color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,0,128))
#Tracking the green Color
(_,contours,hierarchy)=cv2.findContours(green,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,0),2)
cv2.putText(img,"green color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,0))
#Tracking the orange Color
(_,contours,hierarchy)=cv2.findContours(orange,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,140,255),2)
cv2.putText(img,"orange color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,140,255))
#Tracking the White Color
(_,contours,hierarchy)=cv2.findContours(White,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,255),2)
cv2.putText(img,"White color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (255,255,255))
#Tracking the offwhite Color
(_,contours,hierarchy)=cv2.findContours(offwhite,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,128,128),2)
cv2.putText(img,"Offwhite color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,128,128))
#Tracking the black Color
(_,contours,hierarchy)=cv2.findContours(Black,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,0),2)
cv2.putText(img,"black color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,0,0))
#Tracking the grey Color
(_,contours,hierarchy)=cv2.findContours(grey,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(220,220,220),2)
cv2.putText(img,"Grey color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (220,220,220))
#Tracking the brown Color
(_,contours,hierarchy)=cv2.findContours(brown,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(33,67,101),2)
cv2.putText(img,"brown color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (33,67,101))
#Tracking the beige Color
(_,contours,hierarchy)=cv2.findContours(beige,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(126,169,230),2)
cv2.putText(img,"Beige color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (126,169,236))
#Show red percentage
red_per = red
Total_image = (blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Red = np.mean((red_per/Total_image)*100)
print('Percentage of red color',Per_Red)
#Show blue percentage
blue_per = blue
Total_image2 = (blue+red+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Blue = np.mean((blue_per/Total_image2)*100)
print('Percentage blue',Per_Blue)
#Show green percentage
green_per = green
Total_image3 = (green+blue+yellow+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Green = np.mean((green_per/Total_image3)*100)
print('Percentage green',Per_Green)
#Show yellow percentage
yellow_per = yellow
Total_image4 = (yellow+blue+red+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Yellow = np.mean((yellow_per/Total_image4)*100)
print('Percentage yellow',Per_Yellow)
#Show dark red percentage
dred_per = dred
Total_image = (dred+blue+yellow+green+red+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_dR = np.mean((dred_per/Total_image)*100)
print('Percentage of dark red color',Per_dR)
#Show Cream percentage
Cream_per = cream
Total_image2 = (cream+blue+yellow+green+dred+White+Black+offwhite+red+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Cream = np.mean((Cream_per/Total_image2)*100)
print('Percentage of cream color',Per_Cream)
#Show lblue percentage
lblue_per = lblue
Total_image3 = (lblue+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+red+beige+brown+grey+orange+cyan)
Per_lBlue = np.mean((lblue_per/Total_image3)*100)
print('Percentage of light blue color',Per_lBlue)
#Show White percentage
White_per = White
Total_image4 = (White+blue+yellow+green+dred+red+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_White = np.mean((White_per/Total_image4)*100)
print('Percentage of white color',Per_White)
#Show orange percentage
orange_per = orange
Total_image = (orange+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+red+cyan)
Per_Orange = np.mean((orange_per/Total_image)*100)
print('Percentage of orange color',Per_Orange)
#Show purple percentage
purple_per = purple
Total_image2 = (purple+blue+yellow+green+dred+White+Black+offwhite+cream+pink+red+lblue+beige+brown+grey+orange+cyan)
Per_Purple = np.mean((purple_per/Total_image2)*100)
print('Percentage of purple color',Per_Purple)
#Show pink percentage
pink_per = pink
Total_image3 = (pink+blue+yellow+green+dred+White+Black+offwhite+cream+red+purple+lblue+beige+brown+grey+orange+cyan)
Per_Pink = np.mean((pink_per/Total_image3)*100)
print('Percentage of pink color',Per_Pink)
#Show cyan percentage
cyan_per = cyan
Total_image4 = (cyan+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+red)
Per_Cyan = np.mean((cyan_per/Total_image4)*100)
print('Percentage of cyan color',Per_Cyan)
#Show grey percentage
grey_per = grey
Total_image = (grey+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+red+orange+cyan)
Per_Grey = np.mean((grey_per/Total_image)*100)
print('Percentage of grey color',Per_Grey)
#Show black percentage
black_per = Black
Total_image1 = (Black+blue+yellow+green+dred+White+red+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Black = np.mean((black_per/Total_image1)*100)
print('Percentage of black color',Per_Black)
#Show beige percentage
beige_per = beige
Total_image2 = (beige+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+red+brown+grey+orange+cyan)
Per_Beige = np.mean((beige_per/Total_image2)*100)
print('Percentage of beige',Per_Beige)
#Show brown percentage
brown_per = brown
Total_image3 = (brown+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+red+grey+orange+cyan)
Per_Brown = np.mean((brown_per/Total_image3)*100)
print('Percentage of brown color',Per_Brown)
#Show offwhite percentage
offwhite_per = offwhite
Total_image4 = (offwhite+blue+yellow+green+dred+White+Black+red+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Offwhite = np.mean((offwhite_per/Total_image4)*100)
print('Percentage of offwhite',Per_Offwhite)
cv2.imshow("Color Tracking",img)
if cv2.waitKey(10) & 0xFF == ord('q'):
cap.release()
cv2.destroyAllWindows()
break
What I'm trying to achieve to the code to present a histogram where it shows the y-axis (0-100)% and x-axis(the colors that will be detected) and shows how the data is changing when more colors are being detected and how the values fluctuates as more and more colors are being detected.
Thank you in advance!
python opencv colors histogram live-streaming
add a comment |
My color detection code detects some colors while also providing the percentage value of the detected color. However I would like to create a more visual based output like a histogram or graph so I would like any advice in how to create one. I've searched in some forums but most are for still images.
My code is below:
#importing modules
import cv2
import numpy as np
import time
import matplotlib.pyplot as plt
#capturing video through webcam
cap=cv2.VideoCapture(0)
while(1):
_, img = cap.read()
#converting frame(img i.e BGR) to HSV (hue-saturation-value)
hsv=cv2.cvtColor(img,cv2.COLOR_BGR2HSV)
#definig the range of red color
red_lower=np.array([166,84,141],np.uint8)
red_upper=np.array([186,255,255],np.uint8)
#definig the range of dark red color
dred_lower=np.array([0,180,80],np.uint8)
dred_upper=np.array([6,255,141],np.uint8)
#defining the range of pink color
pink_lower=np.array([137,17,130],np.uint8)
pink_upper=np.array([150,165,255],np.uint8)
#defining the Range of cream color
cream_lower=np.array([0,5,168],np.uint8)
cream_upper=np.array([33,113,255],np.uint8)
#defining the Range of Blue color
blue_lower=np.array([97,100,80],np.uint8)
blue_upper=np.array([117,255,180],np.uint8)
#defining the Range of light Blue color
lblue_lower=np.array([100,56.1,142.8],np.uint8)
lblue_upper=np.array([100,70,155.5],np.uint8)
#defining the Range of cyan color
cyan_lower=np.array([90,200,200],np.uint8)
cyan_upper=np.array([90,255,255],np.uint8)
#defining the Range of purple color
purple_lower=np.array([128,40,125],np.uint8)
purple_upper=np.array([138,255,255],np.uint8)
#defining the Range of yellow color
yellow_lower=np.array([24,45,110],np.uint8)
yellow_upper=np.array([30,255,255],np.uint8)
#defining the Range of green color
green_lower=np.array([45,59,119],np.uint8)
green_upper=np.array([68,255,255],np.uint8)
#defining the Range of White color
White_lower=np.array([0,0,200],np.uint8)
White_upper=np.array([180,255,255],np.uint8)
#defining the range of grey color
grey_lower=np.array([106,5,168],np.uint8)
grey_upper=np.array([120,75,255],np.uint8)
#defining the Range of orange color
orange_lower=np.array([15,30,60],np.uint8)
orange_upper=np.array([15,255,255],np.uint8)
#defining the range of offwhite color
offwhite_lower=np.array([0,0,168],np.uint8)
offwhite_upper=np.array([0,0,210],np.uint8)
#defining the Range of black color
Black_lower=np.array([0,0,0],np.uint8)
Black_upper=np.array([180,255,40],np.uint8)
#defining the Range of brown color
Brown_lower=np.array([128,100,150],np.uint8)
Brown_upper=np.array([160,150,255],np.uint8)
#defining the range of beige color
beige_lower=np.array([10,50,180],np.uint8)
beige_upper=np.array([100,255,255],np.uint8)
#finding the range of the colors in the image
red=cv2.inRange(hsv, red_lower, red_upper)
pink=cv2.inRange(hsv,pink_lower,pink_upper)
cream=cv2.inRange(hsv,cream_lower,cream_upper)
dred=cv2.inRange(hsv, dred_lower, dred_upper)
blue=cv2.inRange(hsv,blue_lower,blue_upper)
lblue=cv2.inRange(hsv,lblue_lower,lblue_upper)
cyan=cv2.inRange(hsv,cyan_lower,cyan_upper)
yellow=cv2.inRange(hsv,yellow_lower,yellow_upper)
green=cv2.inRange(hsv,green_lower,green_upper)
purple=cv2.inRange(hsv,purple_lower,purple_upper)
orange=cv2.inRange(hsv,orange_lower,orange_upper)
White=cv2.inRange(hsv,White_lower,White_upper)
offwhite=cv2.inRange(hsv,offwhite_lower,offwhite_upper)
Black=cv2.inRange(hsv,Black_lower,Black_upper)
grey=cv2.inRange(hsv,grey_lower,grey_upper)
brown=cv2.inRange(hsv,Brown_lower,Brown_upper)
beige=cv2.inRange(hsv,beige_lower,beige_upper)
#Morphological transformation, Dilation
kernal = np.ones((5 ,5), "uint8")
red=cv2.dilate(red, kernal)
res=cv2.bitwise_and(img, img, mask = red)
pink=cv2.dilate(pink,kernal)
res1=cv2.bitwise_and(img, img, mask = pink)
cream=cv2.dilate(cream,kernal)
res1=cv2.bitwise_and(img, img, mask = cream)
dred=cv2.dilate(dred, kernal)
res=cv2.bitwise_and(img, img, mask = dred)
blue=cv2.dilate(blue,kernal)
res1=cv2.bitwise_and(img, img, mask = blue)
lblue=cv2.dilate(lblue,kernal)
res1=cv2.bitwise_and(img, img, mask = lblue)
cyan=cv2.dilate(cyan,kernal)
res1=cv2.bitwise_and(img, img, mask = cyan)
yellow=cv2.dilate(yellow,kernal)
res2=cv2.bitwise_and(img, img, mask = yellow)
purple=cv2.dilate(purple, kernal)
res2=cv2.bitwise_and(img, img, mask = purple)
green=cv2.dilate(green,kernal)
res2=cv2.bitwise_and(img, img, mask = green)
orange=cv2.dilate(orange,kernal)
res2=cv2.bitwise_and(img, img, mask = orange)
White=cv2.dilate(White,kernal)
res2=cv2.bitwise_and(img, img, mask = White)
offwhite=cv2.dilate(offwhite, kernal)
res2=cv2.bitwise_and(img, img, mask = offwhite)
Black=cv2.dilate(Black,kernal)
res2=cv2.bitwise_and(img, img, mask = Black)
grey=cv2.dilate(grey, kernal)
res2=cv2.bitwise_and(img, img, mask = grey)
brown=cv2.dilate(brown,kernal)
res2=cv2.bitwise_and(img, img, mask = brown)
beige=cv2.dilate(beige, kernal)
res2=cv2.bitwise_and(img, img, mask = beige)
#Tracking the Red Color
(_,contours,hierarchy)=cv2.findContours(red,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,255),2)
cv2.putText(img,"RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,255))
#Tracking the dark Red Color
(_,contours,hierarchy)=cv2.findContours(dred,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,139),2)
cv2.putText(img,"Dark RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,139))
#Tracking the pink Color
(_,contours,hierarchy)=cv2.findContours(pink,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,255),2)
cv2.putText(img,"Pink color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,255))
#Tracking the cream Color
(_,contours,hierarchy)=cv2.findContours(cream,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(150,255,255),2)
cv2.putText(img,"cream color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (150,255,255))
#Tracking the light Blue Color
(_,contours,hierarchy)=cv2.findContours(lblue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(180,0,0),2)
cv2.putText(img,"light blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (180,0,0))
#Tracking the Blue Color
(_,contours,hierarchy)=cv2.findContours(blue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
cv2.putText(img,"Blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0))
#Tracking the cyan Color
(_,contours,hierarchy)=cv2.findContours(cyan,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,0),2)
cv2.putText(img,"cyan color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,255,0))
#Tracking the yellow Color
(_,contours,hierarchy)=cv2.findContours(yellow,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,217),2)
cv2.putText(img,"yellow color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,217))
#Tracking the purple Color
(_,contours,hierarchy)=cv2.findContours(purple,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,0,128),2)
cv2.putText(img,"Purple color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,0,128))
#Tracking the green Color
(_,contours,hierarchy)=cv2.findContours(green,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,0),2)
cv2.putText(img,"green color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,0))
#Tracking the orange Color
(_,contours,hierarchy)=cv2.findContours(orange,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,140,255),2)
cv2.putText(img,"orange color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,140,255))
#Tracking the White Color
(_,contours,hierarchy)=cv2.findContours(White,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,255),2)
cv2.putText(img,"White color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (255,255,255))
#Tracking the offwhite Color
(_,contours,hierarchy)=cv2.findContours(offwhite,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,128,128),2)
cv2.putText(img,"Offwhite color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,128,128))
#Tracking the black Color
(_,contours,hierarchy)=cv2.findContours(Black,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,0),2)
cv2.putText(img,"black color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,0,0))
#Tracking the grey Color
(_,contours,hierarchy)=cv2.findContours(grey,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(220,220,220),2)
cv2.putText(img,"Grey color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (220,220,220))
#Tracking the brown Color
(_,contours,hierarchy)=cv2.findContours(brown,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(33,67,101),2)
cv2.putText(img,"brown color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (33,67,101))
#Tracking the beige Color
(_,contours,hierarchy)=cv2.findContours(beige,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(126,169,230),2)
cv2.putText(img,"Beige color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (126,169,236))
#Show red percentage
red_per = red
Total_image = (blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Red = np.mean((red_per/Total_image)*100)
print('Percentage of red color',Per_Red)
#Show blue percentage
blue_per = blue
Total_image2 = (blue+red+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Blue = np.mean((blue_per/Total_image2)*100)
print('Percentage blue',Per_Blue)
#Show green percentage
green_per = green
Total_image3 = (green+blue+yellow+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Green = np.mean((green_per/Total_image3)*100)
print('Percentage green',Per_Green)
#Show yellow percentage
yellow_per = yellow
Total_image4 = (yellow+blue+red+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Yellow = np.mean((yellow_per/Total_image4)*100)
print('Percentage yellow',Per_Yellow)
#Show dark red percentage
dred_per = dred
Total_image = (dred+blue+yellow+green+red+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_dR = np.mean((dred_per/Total_image)*100)
print('Percentage of dark red color',Per_dR)
#Show Cream percentage
Cream_per = cream
Total_image2 = (cream+blue+yellow+green+dred+White+Black+offwhite+red+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Cream = np.mean((Cream_per/Total_image2)*100)
print('Percentage of cream color',Per_Cream)
#Show lblue percentage
lblue_per = lblue
Total_image3 = (lblue+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+red+beige+brown+grey+orange+cyan)
Per_lBlue = np.mean((lblue_per/Total_image3)*100)
print('Percentage of light blue color',Per_lBlue)
#Show White percentage
White_per = White
Total_image4 = (White+blue+yellow+green+dred+red+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_White = np.mean((White_per/Total_image4)*100)
print('Percentage of white color',Per_White)
#Show orange percentage
orange_per = orange
Total_image = (orange+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+red+cyan)
Per_Orange = np.mean((orange_per/Total_image)*100)
print('Percentage of orange color',Per_Orange)
#Show purple percentage
purple_per = purple
Total_image2 = (purple+blue+yellow+green+dred+White+Black+offwhite+cream+pink+red+lblue+beige+brown+grey+orange+cyan)
Per_Purple = np.mean((purple_per/Total_image2)*100)
print('Percentage of purple color',Per_Purple)
#Show pink percentage
pink_per = pink
Total_image3 = (pink+blue+yellow+green+dred+White+Black+offwhite+cream+red+purple+lblue+beige+brown+grey+orange+cyan)
Per_Pink = np.mean((pink_per/Total_image3)*100)
print('Percentage of pink color',Per_Pink)
#Show cyan percentage
cyan_per = cyan
Total_image4 = (cyan+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+red)
Per_Cyan = np.mean((cyan_per/Total_image4)*100)
print('Percentage of cyan color',Per_Cyan)
#Show grey percentage
grey_per = grey
Total_image = (grey+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+red+orange+cyan)
Per_Grey = np.mean((grey_per/Total_image)*100)
print('Percentage of grey color',Per_Grey)
#Show black percentage
black_per = Black
Total_image1 = (Black+blue+yellow+green+dred+White+red+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Black = np.mean((black_per/Total_image1)*100)
print('Percentage of black color',Per_Black)
#Show beige percentage
beige_per = beige
Total_image2 = (beige+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+red+brown+grey+orange+cyan)
Per_Beige = np.mean((beige_per/Total_image2)*100)
print('Percentage of beige',Per_Beige)
#Show brown percentage
brown_per = brown
Total_image3 = (brown+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+red+grey+orange+cyan)
Per_Brown = np.mean((brown_per/Total_image3)*100)
print('Percentage of brown color',Per_Brown)
#Show offwhite percentage
offwhite_per = offwhite
Total_image4 = (offwhite+blue+yellow+green+dred+White+Black+red+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Offwhite = np.mean((offwhite_per/Total_image4)*100)
print('Percentage of offwhite',Per_Offwhite)
cv2.imshow("Color Tracking",img)
if cv2.waitKey(10) & 0xFF == ord('q'):
cap.release()
cv2.destroyAllWindows()
break
What I'm trying to achieve to the code to present a histogram where it shows the y-axis (0-100)% and x-axis(the colors that will be detected) and shows how the data is changing when more colors are being detected and how the values fluctuates as more and more colors are being detected.
Thank you in advance!
python opencv colors histogram live-streaming
My color detection code detects some colors while also providing the percentage value of the detected color. However I would like to create a more visual based output like a histogram or graph so I would like any advice in how to create one. I've searched in some forums but most are for still images.
My code is below:
#importing modules
import cv2
import numpy as np
import time
import matplotlib.pyplot as plt
#capturing video through webcam
cap=cv2.VideoCapture(0)
while(1):
_, img = cap.read()
#converting frame(img i.e BGR) to HSV (hue-saturation-value)
hsv=cv2.cvtColor(img,cv2.COLOR_BGR2HSV)
#definig the range of red color
red_lower=np.array([166,84,141],np.uint8)
red_upper=np.array([186,255,255],np.uint8)
#definig the range of dark red color
dred_lower=np.array([0,180,80],np.uint8)
dred_upper=np.array([6,255,141],np.uint8)
#defining the range of pink color
pink_lower=np.array([137,17,130],np.uint8)
pink_upper=np.array([150,165,255],np.uint8)
#defining the Range of cream color
cream_lower=np.array([0,5,168],np.uint8)
cream_upper=np.array([33,113,255],np.uint8)
#defining the Range of Blue color
blue_lower=np.array([97,100,80],np.uint8)
blue_upper=np.array([117,255,180],np.uint8)
#defining the Range of light Blue color
lblue_lower=np.array([100,56.1,142.8],np.uint8)
lblue_upper=np.array([100,70,155.5],np.uint8)
#defining the Range of cyan color
cyan_lower=np.array([90,200,200],np.uint8)
cyan_upper=np.array([90,255,255],np.uint8)
#defining the Range of purple color
purple_lower=np.array([128,40,125],np.uint8)
purple_upper=np.array([138,255,255],np.uint8)
#defining the Range of yellow color
yellow_lower=np.array([24,45,110],np.uint8)
yellow_upper=np.array([30,255,255],np.uint8)
#defining the Range of green color
green_lower=np.array([45,59,119],np.uint8)
green_upper=np.array([68,255,255],np.uint8)
#defining the Range of White color
White_lower=np.array([0,0,200],np.uint8)
White_upper=np.array([180,255,255],np.uint8)
#defining the range of grey color
grey_lower=np.array([106,5,168],np.uint8)
grey_upper=np.array([120,75,255],np.uint8)
#defining the Range of orange color
orange_lower=np.array([15,30,60],np.uint8)
orange_upper=np.array([15,255,255],np.uint8)
#defining the range of offwhite color
offwhite_lower=np.array([0,0,168],np.uint8)
offwhite_upper=np.array([0,0,210],np.uint8)
#defining the Range of black color
Black_lower=np.array([0,0,0],np.uint8)
Black_upper=np.array([180,255,40],np.uint8)
#defining the Range of brown color
Brown_lower=np.array([128,100,150],np.uint8)
Brown_upper=np.array([160,150,255],np.uint8)
#defining the range of beige color
beige_lower=np.array([10,50,180],np.uint8)
beige_upper=np.array([100,255,255],np.uint8)
#finding the range of the colors in the image
red=cv2.inRange(hsv, red_lower, red_upper)
pink=cv2.inRange(hsv,pink_lower,pink_upper)
cream=cv2.inRange(hsv,cream_lower,cream_upper)
dred=cv2.inRange(hsv, dred_lower, dred_upper)
blue=cv2.inRange(hsv,blue_lower,blue_upper)
lblue=cv2.inRange(hsv,lblue_lower,lblue_upper)
cyan=cv2.inRange(hsv,cyan_lower,cyan_upper)
yellow=cv2.inRange(hsv,yellow_lower,yellow_upper)
green=cv2.inRange(hsv,green_lower,green_upper)
purple=cv2.inRange(hsv,purple_lower,purple_upper)
orange=cv2.inRange(hsv,orange_lower,orange_upper)
White=cv2.inRange(hsv,White_lower,White_upper)
offwhite=cv2.inRange(hsv,offwhite_lower,offwhite_upper)
Black=cv2.inRange(hsv,Black_lower,Black_upper)
grey=cv2.inRange(hsv,grey_lower,grey_upper)
brown=cv2.inRange(hsv,Brown_lower,Brown_upper)
beige=cv2.inRange(hsv,beige_lower,beige_upper)
#Morphological transformation, Dilation
kernal = np.ones((5 ,5), "uint8")
red=cv2.dilate(red, kernal)
res=cv2.bitwise_and(img, img, mask = red)
pink=cv2.dilate(pink,kernal)
res1=cv2.bitwise_and(img, img, mask = pink)
cream=cv2.dilate(cream,kernal)
res1=cv2.bitwise_and(img, img, mask = cream)
dred=cv2.dilate(dred, kernal)
res=cv2.bitwise_and(img, img, mask = dred)
blue=cv2.dilate(blue,kernal)
res1=cv2.bitwise_and(img, img, mask = blue)
lblue=cv2.dilate(lblue,kernal)
res1=cv2.bitwise_and(img, img, mask = lblue)
cyan=cv2.dilate(cyan,kernal)
res1=cv2.bitwise_and(img, img, mask = cyan)
yellow=cv2.dilate(yellow,kernal)
res2=cv2.bitwise_and(img, img, mask = yellow)
purple=cv2.dilate(purple, kernal)
res2=cv2.bitwise_and(img, img, mask = purple)
green=cv2.dilate(green,kernal)
res2=cv2.bitwise_and(img, img, mask = green)
orange=cv2.dilate(orange,kernal)
res2=cv2.bitwise_and(img, img, mask = orange)
White=cv2.dilate(White,kernal)
res2=cv2.bitwise_and(img, img, mask = White)
offwhite=cv2.dilate(offwhite, kernal)
res2=cv2.bitwise_and(img, img, mask = offwhite)
Black=cv2.dilate(Black,kernal)
res2=cv2.bitwise_and(img, img, mask = Black)
grey=cv2.dilate(grey, kernal)
res2=cv2.bitwise_and(img, img, mask = grey)
brown=cv2.dilate(brown,kernal)
res2=cv2.bitwise_and(img, img, mask = brown)
beige=cv2.dilate(beige, kernal)
res2=cv2.bitwise_and(img, img, mask = beige)
#Tracking the Red Color
(_,contours,hierarchy)=cv2.findContours(red,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,255),2)
cv2.putText(img,"RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,255))
#Tracking the dark Red Color
(_,contours,hierarchy)=cv2.findContours(dred,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,139),2)
cv2.putText(img,"Dark RED color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0,0,139))
#Tracking the pink Color
(_,contours,hierarchy)=cv2.findContours(pink,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,255),2)
cv2.putText(img,"Pink color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,255))
#Tracking the cream Color
(_,contours,hierarchy)=cv2.findContours(cream,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(150,255,255),2)
cv2.putText(img,"cream color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (150,255,255))
#Tracking the light Blue Color
(_,contours,hierarchy)=cv2.findContours(lblue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(180,0,0),2)
cv2.putText(img,"light blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (180,0,0))
#Tracking the Blue Color
(_,contours,hierarchy)=cv2.findContours(blue,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
cv2.putText(img,"Blue color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0))
#Tracking the cyan Color
(_,contours,hierarchy)=cv2.findContours(cyan,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,0),2)
cv2.putText(img,"cyan color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,255,0))
#Tracking the yellow Color
(_,contours,hierarchy)=cv2.findContours(yellow,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,217),2)
cv2.putText(img,"yellow color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,217))
#Tracking the purple Color
(_,contours,hierarchy)=cv2.findContours(purple,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,0,128),2)
cv2.putText(img,"Purple color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,0,128))
#Tracking the green Color
(_,contours,hierarchy)=cv2.findContours(green,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,0),2)
cv2.putText(img,"green color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,255,0))
#Tracking the orange Color
(_,contours,hierarchy)=cv2.findContours(orange,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,140,255),2)
cv2.putText(img,"orange color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,140,255))
#Tracking the White Color
(_,contours,hierarchy)=cv2.findContours(White,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(255,255,255),2)
cv2.putText(img,"White color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (255,255,255))
#Tracking the offwhite Color
(_,contours,hierarchy)=cv2.findContours(offwhite,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(128,128,128),2)
cv2.putText(img,"Offwhite color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (128,128,128))
#Tracking the black Color
(_,contours,hierarchy)=cv2.findContours(Black,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(0,0,0),2)
cv2.putText(img,"black color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (0,0,0))
#Tracking the grey Color
(_,contours,hierarchy)=cv2.findContours(grey,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(220,220,220),2)
cv2.putText(img,"Grey color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (220,220,220))
#Tracking the brown Color
(_,contours,hierarchy)=cv2.findContours(brown,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(33,67,101),2)
cv2.putText(img,"brown color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 1.0, (33,67,101))
#Tracking the beige Color
(_,contours,hierarchy)=cv2.findContours(beige,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for pic, contour in enumerate(contours):
area = cv2.contourArea(contour)
if(area>=300):
x,y,w,h = cv2.boundingRect(contour)
img = cv2.rectangle(img,(x,y),(x+w,y+h),(126,169,230),2)
cv2.putText(img,"Beige color",(x,y),cv2.FONT_HERSHEY_SIMPLEX, 0.7, (126,169,236))
#Show red percentage
red_per = red
Total_image = (blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Red = np.mean((red_per/Total_image)*100)
print('Percentage of red color',Per_Red)
#Show blue percentage
blue_per = blue
Total_image2 = (blue+red+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Blue = np.mean((blue_per/Total_image2)*100)
print('Percentage blue',Per_Blue)
#Show green percentage
green_per = green
Total_image3 = (green+blue+yellow+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan+red)
Per_Green = np.mean((green_per/Total_image3)*100)
print('Percentage green',Per_Green)
#Show yellow percentage
yellow_per = yellow
Total_image4 = (yellow+blue+red+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Yellow = np.mean((yellow_per/Total_image4)*100)
print('Percentage yellow',Per_Yellow)
#Show dark red percentage
dred_per = dred
Total_image = (dred+blue+yellow+green+red+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_dR = np.mean((dred_per/Total_image)*100)
print('Percentage of dark red color',Per_dR)
#Show Cream percentage
Cream_per = cream
Total_image2 = (cream+blue+yellow+green+dred+White+Black+offwhite+red+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Cream = np.mean((Cream_per/Total_image2)*100)
print('Percentage of cream color',Per_Cream)
#Show lblue percentage
lblue_per = lblue
Total_image3 = (lblue+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+red+beige+brown+grey+orange+cyan)
Per_lBlue = np.mean((lblue_per/Total_image3)*100)
print('Percentage of light blue color',Per_lBlue)
#Show White percentage
White_per = White
Total_image4 = (White+blue+yellow+green+dred+red+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_White = np.mean((White_per/Total_image4)*100)
print('Percentage of white color',Per_White)
#Show orange percentage
orange_per = orange
Total_image = (orange+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+red+cyan)
Per_Orange = np.mean((orange_per/Total_image)*100)
print('Percentage of orange color',Per_Orange)
#Show purple percentage
purple_per = purple
Total_image2 = (purple+blue+yellow+green+dred+White+Black+offwhite+cream+pink+red+lblue+beige+brown+grey+orange+cyan)
Per_Purple = np.mean((purple_per/Total_image2)*100)
print('Percentage of purple color',Per_Purple)
#Show pink percentage
pink_per = pink
Total_image3 = (pink+blue+yellow+green+dred+White+Black+offwhite+cream+red+purple+lblue+beige+brown+grey+orange+cyan)
Per_Pink = np.mean((pink_per/Total_image3)*100)
print('Percentage of pink color',Per_Pink)
#Show cyan percentage
cyan_per = cyan
Total_image4 = (cyan+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+red)
Per_Cyan = np.mean((cyan_per/Total_image4)*100)
print('Percentage of cyan color',Per_Cyan)
#Show grey percentage
grey_per = grey
Total_image = (grey+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+brown+red+orange+cyan)
Per_Grey = np.mean((grey_per/Total_image)*100)
print('Percentage of grey color',Per_Grey)
#Show black percentage
black_per = Black
Total_image1 = (Black+blue+yellow+green+dred+White+red+offwhite+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Black = np.mean((black_per/Total_image1)*100)
print('Percentage of black color',Per_Black)
#Show beige percentage
beige_per = beige
Total_image2 = (beige+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+red+brown+grey+orange+cyan)
Per_Beige = np.mean((beige_per/Total_image2)*100)
print('Percentage of beige',Per_Beige)
#Show brown percentage
brown_per = brown
Total_image3 = (brown+blue+yellow+green+dred+White+Black+offwhite+cream+pink+purple+lblue+beige+red+grey+orange+cyan)
Per_Brown = np.mean((brown_per/Total_image3)*100)
print('Percentage of brown color',Per_Brown)
#Show offwhite percentage
offwhite_per = offwhite
Total_image4 = (offwhite+blue+yellow+green+dred+White+Black+red+cream+pink+purple+lblue+beige+brown+grey+orange+cyan)
Per_Offwhite = np.mean((offwhite_per/Total_image4)*100)
print('Percentage of offwhite',Per_Offwhite)
cv2.imshow("Color Tracking",img)
if cv2.waitKey(10) & 0xFF == ord('q'):
cap.release()
cv2.destroyAllWindows()
break
What I'm trying to achieve to the code to present a histogram where it shows the y-axis (0-100)% and x-axis(the colors that will be detected) and shows how the data is changing when more colors are being detected and how the values fluctuates as more and more colors are being detected.
Thank you in advance!
python opencv colors histogram live-streaming
python opencv colors histogram live-streaming
asked Nov 15 '18 at 5:07
Rayyan BataisRayyan Batais
12
12
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312778%2fhow-to-create-a-real-time-graph-or-histogram-based-on-python-opencv%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312778%2fhow-to-create-a-real-time-graph-or-histogram-based-on-python-opencv%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JU,wm2 qFx16MXXAlF9mbLQm,hwvHmk6nMNyXjG9uVnngHbjOYbAYtkv4bL