to break the tree in even number of nodes
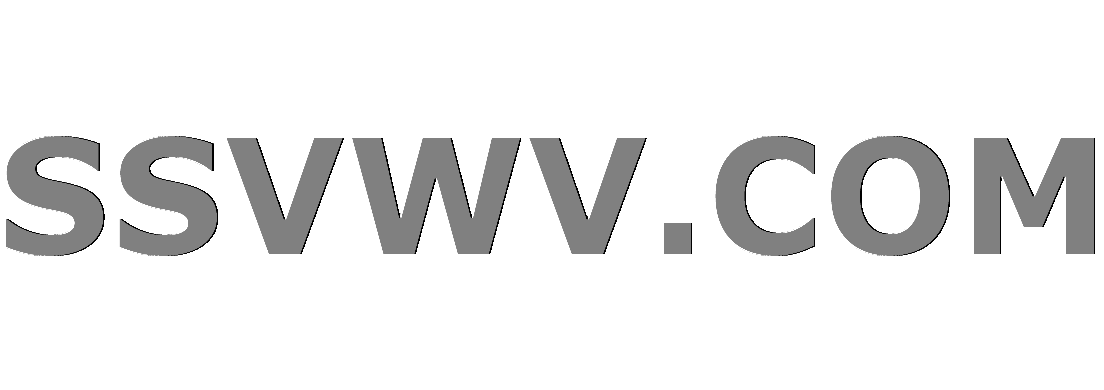
Multi tool use
You are given a tree (a simple connected graph with no cycles).
Find the maximum number of edges you can remove from the tree to get a forest such that each connected component of the forest contains an even number of nodes.
https://www.hackerrank.com/challenges/even-tree/problem
In the above link the test cases are given. For sampple input 1, I am getting 0 as output instead of expected value 2.
#include<stdio.h>
#include<stdlib.h>
int ans = 0;
int v, e;
int visited[201];
int gph[201][201];
int dfs(int i)
int num_nodes;
int num_vertex = 0;
visited[i] = 1;
for (int j = 1; j <= v; j++)
if (visited[i] == 0 && gph[i][j] == 1)
num_nodes = dfs(j);
if (num_nodes % 2 == 0)
ans++;
else
num_vertex += num_nodes;
return num_vertex + 1;
int main()
scanf("%d %d", &v, &e); // vertices and edges
int u, v;
for (int i = 0; i < e; i++)
scanf("%d %d", &u, &v); //edges of undirected graph
gph[u][v] = 1;
gph[v][u] = 1;
dfs(1);
printf("%d", ans);
Test case:
10 9
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
Expected output: 2
Actual output: 0
c algorithm graph-theory dfs
add a comment |
You are given a tree (a simple connected graph with no cycles).
Find the maximum number of edges you can remove from the tree to get a forest such that each connected component of the forest contains an even number of nodes.
https://www.hackerrank.com/challenges/even-tree/problem
In the above link the test cases are given. For sampple input 1, I am getting 0 as output instead of expected value 2.
#include<stdio.h>
#include<stdlib.h>
int ans = 0;
int v, e;
int visited[201];
int gph[201][201];
int dfs(int i)
int num_nodes;
int num_vertex = 0;
visited[i] = 1;
for (int j = 1; j <= v; j++)
if (visited[i] == 0 && gph[i][j] == 1)
num_nodes = dfs(j);
if (num_nodes % 2 == 0)
ans++;
else
num_vertex += num_nodes;
return num_vertex + 1;
int main()
scanf("%d %d", &v, &e); // vertices and edges
int u, v;
for (int i = 0; i < e; i++)
scanf("%d %d", &u, &v); //edges of undirected graph
gph[u][v] = 1;
gph[v][u] = 1;
dfs(1);
printf("%d", ans);
Test case:
10 9
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
Expected output: 2
Actual output: 0
c algorithm graph-theory dfs
1
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
Not done, replace thescanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.
– Ulrich Eckhardt
Nov 14 '18 at 19:50
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26
add a comment |
You are given a tree (a simple connected graph with no cycles).
Find the maximum number of edges you can remove from the tree to get a forest such that each connected component of the forest contains an even number of nodes.
https://www.hackerrank.com/challenges/even-tree/problem
In the above link the test cases are given. For sampple input 1, I am getting 0 as output instead of expected value 2.
#include<stdio.h>
#include<stdlib.h>
int ans = 0;
int v, e;
int visited[201];
int gph[201][201];
int dfs(int i)
int num_nodes;
int num_vertex = 0;
visited[i] = 1;
for (int j = 1; j <= v; j++)
if (visited[i] == 0 && gph[i][j] == 1)
num_nodes = dfs(j);
if (num_nodes % 2 == 0)
ans++;
else
num_vertex += num_nodes;
return num_vertex + 1;
int main()
scanf("%d %d", &v, &e); // vertices and edges
int u, v;
for (int i = 0; i < e; i++)
scanf("%d %d", &u, &v); //edges of undirected graph
gph[u][v] = 1;
gph[v][u] = 1;
dfs(1);
printf("%d", ans);
Test case:
10 9
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
Expected output: 2
Actual output: 0
c algorithm graph-theory dfs
You are given a tree (a simple connected graph with no cycles).
Find the maximum number of edges you can remove from the tree to get a forest such that each connected component of the forest contains an even number of nodes.
https://www.hackerrank.com/challenges/even-tree/problem
In the above link the test cases are given. For sampple input 1, I am getting 0 as output instead of expected value 2.
#include<stdio.h>
#include<stdlib.h>
int ans = 0;
int v, e;
int visited[201];
int gph[201][201];
int dfs(int i)
int num_nodes;
int num_vertex = 0;
visited[i] = 1;
for (int j = 1; j <= v; j++)
if (visited[i] == 0 && gph[i][j] == 1)
num_nodes = dfs(j);
if (num_nodes % 2 == 0)
ans++;
else
num_vertex += num_nodes;
return num_vertex + 1;
int main()
scanf("%d %d", &v, &e); // vertices and edges
int u, v;
for (int i = 0; i < e; i++)
scanf("%d %d", &u, &v); //edges of undirected graph
gph[u][v] = 1;
gph[v][u] = 1;
dfs(1);
printf("%d", ans);
Test case:
10 9
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
Expected output: 2
Actual output: 0
c algorithm graph-theory dfs
c algorithm graph-theory dfs
edited Nov 15 '18 at 10:35


JohnKoch
402317
402317
asked Nov 14 '18 at 19:14
user10628441
1
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
Not done, replace thescanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.
– Ulrich Eckhardt
Nov 14 '18 at 19:50
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26
add a comment |
1
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
Not done, replace thescanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.
– Ulrich Eckhardt
Nov 14 '18 at 19:50
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26
1
1
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
Not done, replace the
scanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.– Ulrich Eckhardt
Nov 14 '18 at 19:50
Not done, replace the
scanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.– Ulrich Eckhardt
Nov 14 '18 at 19:50
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26
add a comment |
2 Answers
2
active
oldest
votes
There is a typo. The condition expression in if
clause
if (visited[i] == 0 && gph[i][j] == 1)
should be
if (visited[j] == 0 && gph[i][j] == 1)
BTW, the constraints in hackrank question is 2 <= n <= 100
, so you definitely don't need a fixed array of size 201.
add a comment |
// ans is total number of edges removed and al is adjacency list of the tree.
int dfs(int node)
visit[node]=true;
int num_vertex=0;
for(int i=0;i<al[node].size();i++)
if(!visit[al[node][i]])
int num_nodes=dfs(al[node][i]);
if(num_nodes%2==0)
ans++;
else
num_vertex+=num_nodes;
return num_vertex+1;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307277%2fto-break-the-tree-in-even-number-of-nodes%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a typo. The condition expression in if
clause
if (visited[i] == 0 && gph[i][j] == 1)
should be
if (visited[j] == 0 && gph[i][j] == 1)
BTW, the constraints in hackrank question is 2 <= n <= 100
, so you definitely don't need a fixed array of size 201.
add a comment |
There is a typo. The condition expression in if
clause
if (visited[i] == 0 && gph[i][j] == 1)
should be
if (visited[j] == 0 && gph[i][j] == 1)
BTW, the constraints in hackrank question is 2 <= n <= 100
, so you definitely don't need a fixed array of size 201.
add a comment |
There is a typo. The condition expression in if
clause
if (visited[i] == 0 && gph[i][j] == 1)
should be
if (visited[j] == 0 && gph[i][j] == 1)
BTW, the constraints in hackrank question is 2 <= n <= 100
, so you definitely don't need a fixed array of size 201.
There is a typo. The condition expression in if
clause
if (visited[i] == 0 && gph[i][j] == 1)
should be
if (visited[j] == 0 && gph[i][j] == 1)
BTW, the constraints in hackrank question is 2 <= n <= 100
, so you definitely don't need a fixed array of size 201.
answered Nov 15 '18 at 10:08


JohnKochJohnKoch
402317
402317
add a comment |
add a comment |
// ans is total number of edges removed and al is adjacency list of the tree.
int dfs(int node)
visit[node]=true;
int num_vertex=0;
for(int i=0;i<al[node].size();i++)
if(!visit[al[node][i]])
int num_nodes=dfs(al[node][i]);
if(num_nodes%2==0)
ans++;
else
num_vertex+=num_nodes;
return num_vertex+1;
add a comment |
// ans is total number of edges removed and al is adjacency list of the tree.
int dfs(int node)
visit[node]=true;
int num_vertex=0;
for(int i=0;i<al[node].size();i++)
if(!visit[al[node][i]])
int num_nodes=dfs(al[node][i]);
if(num_nodes%2==0)
ans++;
else
num_vertex+=num_nodes;
return num_vertex+1;
add a comment |
// ans is total number of edges removed and al is adjacency list of the tree.
int dfs(int node)
visit[node]=true;
int num_vertex=0;
for(int i=0;i<al[node].size();i++)
if(!visit[al[node][i]])
int num_nodes=dfs(al[node][i]);
if(num_nodes%2==0)
ans++;
else
num_vertex+=num_nodes;
return num_vertex+1;
// ans is total number of edges removed and al is adjacency list of the tree.
int dfs(int node)
visit[node]=true;
int num_vertex=0;
for(int i=0;i<al[node].size();i++)
if(!visit[al[node][i]])
int num_nodes=dfs(al[node][i]);
if(num_nodes%2==0)
ans++;
else
num_vertex+=num_nodes;
return num_vertex+1;
answered Nov 17 '18 at 4:09
user9728599
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307277%2fto-break-the-tree-in-even-number-of-nodes%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kyz0SLn31CjAzaXGQkvp8eUp w,hk0wjNB1 T,ci076Ykrs98H,Cbw,CM1G
1
Please adhere to the posting guidelines: supply the problem test case in your posting. The link you gave does not permit general access; don't expect people to create an account specifically to diagnose your problem. Just hard-code the example into your code -- reading input shouldn't be part of the problem, so it shouldn't be in this posting.
– Prune
Nov 14 '18 at 19:25
done boss @Prune
– user10628441
Nov 14 '18 at 19:32
Not done, replace the
scanf()
calls. The goal should be to provide a Minimal, Complete, and Verifiable example. BTW: Do some research why using global variables is a bad idea, that may well cause your problems. Also, learn how to use a debugger to step through code.– Ulrich Eckhardt
Nov 14 '18 at 19:50
When your program is producing incorrect output, the correct thing to do is to use a debugger and trace through its execution. You may also wish to work through it on paper. You could add statements to display intermediate values during execution to test or debunk assumptions you're making. The time to post a question on Stack Overflow is after you have done all the above.
– paddy
Nov 14 '18 at 22:26