Giving array a bigger value doesn't increase its size?
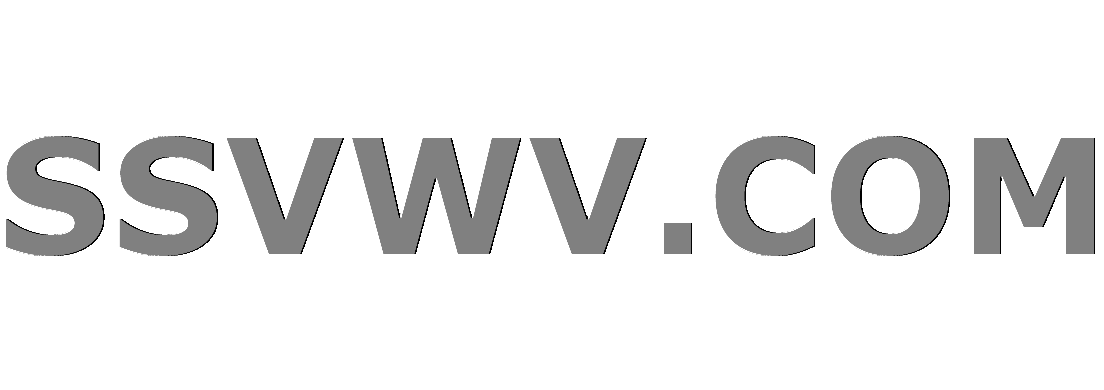
Multi tool use
Here's what I did:
#include <stdio.h>
#include <string.h>
int main()
char name = "longname";
printf("Name = %s n",name);
strcpy(name,"evenlongername");
printf("Name = %s n",name);
printf("size of the array is : %d",sizeof(name));
return 0;
It works, but how? I thought that once memory is assigned to an array in a program, it is not possible to change it. But, the output of this program is:
Name = longname
Name = evenlongername
size of the array is 9
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes (including the string terminator)?
c
add a comment |
Here's what I did:
#include <stdio.h>
#include <string.h>
int main()
char name = "longname";
printf("Name = %s n",name);
strcpy(name,"evenlongername");
printf("Name = %s n",name);
printf("size of the array is : %d",sizeof(name));
return 0;
It works, but how? I thought that once memory is assigned to an array in a program, it is not possible to change it. But, the output of this program is:
Name = longname
Name = evenlongername
size of the array is 9
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes (including the string terminator)?
c
3
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
1
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,sizeof()
is evaluated at compile time and doesn't change the result at runtime.
– Jonathan Leffler
Nov 14 '18 at 19:21
add a comment |
Here's what I did:
#include <stdio.h>
#include <string.h>
int main()
char name = "longname";
printf("Name = %s n",name);
strcpy(name,"evenlongername");
printf("Name = %s n",name);
printf("size of the array is : %d",sizeof(name));
return 0;
It works, but how? I thought that once memory is assigned to an array in a program, it is not possible to change it. But, the output of this program is:
Name = longname
Name = evenlongername
size of the array is 9
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes (including the string terminator)?
c
Here's what I did:
#include <stdio.h>
#include <string.h>
int main()
char name = "longname";
printf("Name = %s n",name);
strcpy(name,"evenlongername");
printf("Name = %s n",name);
printf("size of the array is : %d",sizeof(name));
return 0;
It works, but how? I thought that once memory is assigned to an array in a program, it is not possible to change it. But, the output of this program is:
Name = longname
Name = evenlongername
size of the array is 9
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes (including the string terminator)?
c
c
edited Nov 14 '18 at 19:26


Jonathan Leffler
568k916811031
568k916811031
asked Nov 14 '18 at 19:12
KrishKrish
37227
37227
3
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
1
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,sizeof()
is evaluated at compile time and doesn't change the result at runtime.
– Jonathan Leffler
Nov 14 '18 at 19:21
add a comment |
3
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
1
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,sizeof()
is evaluated at compile time and doesn't change the result at runtime.
– Jonathan Leffler
Nov 14 '18 at 19:21
3
3
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
1
1
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,
sizeof()
is evaluated at compile time and doesn't change the result at runtime.– Jonathan Leffler
Nov 14 '18 at 19:21
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,
sizeof()
is evaluated at compile time and doesn't change the result at runtime.– Jonathan Leffler
Nov 14 '18 at 19:21
add a comment |
3 Answers
3
active
oldest
votes
In this case, name
is allocated to fit "longname"
, which is 9 bytes. When you copy "evenlongername"
into it, you're writing outside of bounds of that array. It's undefined behavior to write outside of the bounds, this means it may or may not work. Some times, it'll work, other times you'll get seg fault, yet other times you'll get weird behavior.
add a comment |
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes(including the string terminator)?
You are using a dangerous function (see Bugs), strcpy
, which blindly copies source string to destination buffer without knowing about its size; in your case of copying 15 bytes into a buffer with size 9 bytes, essentially you have overflown. Your program may work fine if the memory access is valid and it doesn't overwrite something important.
Because C is a lower-level programming language, a C char
is "barebone" mapping of memory, and not a "smart" container like C++ std::vector
which automatically manages its size for you as you dynamically add and remove elements. If you are still not clear about the philosophy of C in this, I'd recommend you read *YOU* are full of bullshit
. Very classic and rewarding.
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.
– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; ifstrcpy
is really that safe to use then there isnt a reason to addstrncpy
?
– Cyker
Nov 14 '18 at 19:36
2
strncpy()
is not a "safe" version ofstrcpy()
. Each has its place for different tasks.
– chux
Nov 14 '18 at 19:40
@chuxstrncpy()
is a "safer" version ofstrcpy()
, in the sense thatstrncpy()
is overflow-safe butstrcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;
– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promotingstrncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promotestrncpy()
as safer has 152 up and 0 down votes.
– chux
Nov 14 '18 at 19:52
|
show 1 more comment
Using sizeof
on a char array will return the size of the buffer, not the length of the null-terminated string in the buffer. If you use strcpy
to try and overflow the array, and it just happens to work (it's still undefined behavior), sizeof
is still going to report the size used at declaration. That never changes.
If what you're interested in is observing how the length of a string changes with different assignments:
- Use an adequate buffer to store every string you're going to test.
- Use the function
strlen
in<string.h>
which will give you the actual length of the string, and not the length of your buffer, which, once declared, is constant.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307241%2fgiving-array-a-bigger-value-doesnt-increase-its-size%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
In this case, name
is allocated to fit "longname"
, which is 9 bytes. When you copy "evenlongername"
into it, you're writing outside of bounds of that array. It's undefined behavior to write outside of the bounds, this means it may or may not work. Some times, it'll work, other times you'll get seg fault, yet other times you'll get weird behavior.
add a comment |
In this case, name
is allocated to fit "longname"
, which is 9 bytes. When you copy "evenlongername"
into it, you're writing outside of bounds of that array. It's undefined behavior to write outside of the bounds, this means it may or may not work. Some times, it'll work, other times you'll get seg fault, yet other times you'll get weird behavior.
add a comment |
In this case, name
is allocated to fit "longname"
, which is 9 bytes. When you copy "evenlongername"
into it, you're writing outside of bounds of that array. It's undefined behavior to write outside of the bounds, this means it may or may not work. Some times, it'll work, other times you'll get seg fault, yet other times you'll get weird behavior.
In this case, name
is allocated to fit "longname"
, which is 9 bytes. When you copy "evenlongername"
into it, you're writing outside of bounds of that array. It's undefined behavior to write outside of the bounds, this means it may or may not work. Some times, it'll work, other times you'll get seg fault, yet other times you'll get weird behavior.
edited Nov 14 '18 at 19:24
answered Nov 14 '18 at 19:16


ODYN-KonODYN-Kon
2,3852825
2,3852825
add a comment |
add a comment |
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes(including the string terminator)?
You are using a dangerous function (see Bugs), strcpy
, which blindly copies source string to destination buffer without knowing about its size; in your case of copying 15 bytes into a buffer with size 9 bytes, essentially you have overflown. Your program may work fine if the memory access is valid and it doesn't overwrite something important.
Because C is a lower-level programming language, a C char
is "barebone" mapping of memory, and not a "smart" container like C++ std::vector
which automatically manages its size for you as you dynamically add and remove elements. If you are still not clear about the philosophy of C in this, I'd recommend you read *YOU* are full of bullshit
. Very classic and rewarding.
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.
– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; ifstrcpy
is really that safe to use then there isnt a reason to addstrncpy
?
– Cyker
Nov 14 '18 at 19:36
2
strncpy()
is not a "safe" version ofstrcpy()
. Each has its place for different tasks.
– chux
Nov 14 '18 at 19:40
@chuxstrncpy()
is a "safer" version ofstrcpy()
, in the sense thatstrncpy()
is overflow-safe butstrcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;
– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promotingstrncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promotestrncpy()
as safer has 152 up and 0 down votes.
– chux
Nov 14 '18 at 19:52
|
show 1 more comment
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes(including the string terminator)?
You are using a dangerous function (see Bugs), strcpy
, which blindly copies source string to destination buffer without knowing about its size; in your case of copying 15 bytes into a buffer with size 9 bytes, essentially you have overflown. Your program may work fine if the memory access is valid and it doesn't overwrite something important.
Because C is a lower-level programming language, a C char
is "barebone" mapping of memory, and not a "smart" container like C++ std::vector
which automatically manages its size for you as you dynamically add and remove elements. If you are still not clear about the philosophy of C in this, I'd recommend you read *YOU* are full of bullshit
. Very classic and rewarding.
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.
– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; ifstrcpy
is really that safe to use then there isnt a reason to addstrncpy
?
– Cyker
Nov 14 '18 at 19:36
2
strncpy()
is not a "safe" version ofstrcpy()
. Each has its place for different tasks.
– chux
Nov 14 '18 at 19:40
@chuxstrncpy()
is a "safer" version ofstrcpy()
, in the sense thatstrncpy()
is overflow-safe butstrcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;
– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promotingstrncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promotestrncpy()
as safer has 152 up and 0 down votes.
– chux
Nov 14 '18 at 19:52
|
show 1 more comment
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes(including the string terminator)?
You are using a dangerous function (see Bugs), strcpy
, which blindly copies source string to destination buffer without knowing about its size; in your case of copying 15 bytes into a buffer with size 9 bytes, essentially you have overflown. Your program may work fine if the memory access is valid and it doesn't overwrite something important.
Because C is a lower-level programming language, a C char
is "barebone" mapping of memory, and not a "smart" container like C++ std::vector
which automatically manages its size for you as you dynamically add and remove elements. If you are still not clear about the philosophy of C in this, I'd recommend you read *YOU* are full of bullshit
. Very classic and rewarding.
So the compiler affirms that the size of the array is still 9. How is it able to store the word 'evenlongername' which has a size of 15 bytes(including the string terminator)?
You are using a dangerous function (see Bugs), strcpy
, which blindly copies source string to destination buffer without knowing about its size; in your case of copying 15 bytes into a buffer with size 9 bytes, essentially you have overflown. Your program may work fine if the memory access is valid and it doesn't overwrite something important.
Because C is a lower-level programming language, a C char
is "barebone" mapping of memory, and not a "smart" container like C++ std::vector
which automatically manages its size for you as you dynamically add and remove elements. If you are still not clear about the philosophy of C in this, I'd recommend you read *YOU* are full of bullshit
. Very classic and rewarding.
edited Nov 14 '18 at 19:48
answered Nov 14 '18 at 19:21
CykerCyker
2,98853345
2,98853345
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.
– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; ifstrcpy
is really that safe to use then there isnt a reason to addstrncpy
?
– Cyker
Nov 14 '18 at 19:36
2
strncpy()
is not a "safe" version ofstrcpy()
. Each has its place for different tasks.
– chux
Nov 14 '18 at 19:40
@chuxstrncpy()
is a "safer" version ofstrcpy()
, in the sense thatstrncpy()
is overflow-safe butstrcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;
– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promotingstrncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promotestrncpy()
as safer has 152 up and 0 down votes.
– chux
Nov 14 '18 at 19:52
|
show 1 more comment
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.
– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; ifstrcpy
is really that safe to use then there isnt a reason to addstrncpy
?
– Cyker
Nov 14 '18 at 19:36
2
strncpy()
is not a "safe" version ofstrcpy()
. Each has its place for different tasks.
– chux
Nov 14 '18 at 19:40
@chuxstrncpy()
is a "safer" version ofstrcpy()
, in the sense thatstrncpy()
is overflow-safe butstrcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;
– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promotingstrncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promotestrncpy()
as safer has 152 up and 0 down votes.
– chux
Nov 14 '18 at 19:52
2
2
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.– chux
Nov 14 '18 at 19:33
strcpy()
is not dangerous. It is an optimized function for a particular task. If a coder needs to use "safe" functions even without understanding them, C is not the a language to use.– chux
Nov 14 '18 at 19:33
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; if
strcpy
is really that safe to use then there isnt a reason to add strncpy
?– Cyker
Nov 14 '18 at 19:36
@chux well, that depends on how much an expert you are; i think most of the time it doesnt hurt calculating and adding the destination buffer size; but you are free to omit that if you are confident; if
strcpy
is really that safe to use then there isnt a reason to add strncpy
?– Cyker
Nov 14 '18 at 19:36
2
2
strncpy()
is not a "safe" version of strcpy()
. Each has its place for different tasks.– chux
Nov 14 '18 at 19:40
strncpy()
is not a "safe" version of strcpy()
. Each has its place for different tasks.– chux
Nov 14 '18 at 19:40
@chux
strncpy()
is a "safer" version of strcpy()
, in the sense that strncpy()
is overflow-safe but strcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;– Cyker
Nov 14 '18 at 19:47
@chux
strncpy()
is a "safer" version of strcpy()
, in the sense that strncpy()
is overflow-safe but strcpy()
isnt; but you are still free to use either if you know that would work correctly in your program;– Cyker
Nov 14 '18 at 19:47
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promoting
strncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promote strncpy()
as safer has 152 up and 0 down votes.– chux
Nov 14 '18 at 19:52
This is not only my view, Why should you use strncpy instead of strcpy?. The accepted answer promoting
strncpy()
, 109 Up and 26 down votes. The higher rated answer which does not promote strncpy()
as safer has 152 up and 0 down votes.– chux
Nov 14 '18 at 19:52
|
show 1 more comment
Using sizeof
on a char array will return the size of the buffer, not the length of the null-terminated string in the buffer. If you use strcpy
to try and overflow the array, and it just happens to work (it's still undefined behavior), sizeof
is still going to report the size used at declaration. That never changes.
If what you're interested in is observing how the length of a string changes with different assignments:
- Use an adequate buffer to store every string you're going to test.
- Use the function
strlen
in<string.h>
which will give you the actual length of the string, and not the length of your buffer, which, once declared, is constant.
add a comment |
Using sizeof
on a char array will return the size of the buffer, not the length of the null-terminated string in the buffer. If you use strcpy
to try and overflow the array, and it just happens to work (it's still undefined behavior), sizeof
is still going to report the size used at declaration. That never changes.
If what you're interested in is observing how the length of a string changes with different assignments:
- Use an adequate buffer to store every string you're going to test.
- Use the function
strlen
in<string.h>
which will give you the actual length of the string, and not the length of your buffer, which, once declared, is constant.
add a comment |
Using sizeof
on a char array will return the size of the buffer, not the length of the null-terminated string in the buffer. If you use strcpy
to try and overflow the array, and it just happens to work (it's still undefined behavior), sizeof
is still going to report the size used at declaration. That never changes.
If what you're interested in is observing how the length of a string changes with different assignments:
- Use an adequate buffer to store every string you're going to test.
- Use the function
strlen
in<string.h>
which will give you the actual length of the string, and not the length of your buffer, which, once declared, is constant.
Using sizeof
on a char array will return the size of the buffer, not the length of the null-terminated string in the buffer. If you use strcpy
to try and overflow the array, and it just happens to work (it's still undefined behavior), sizeof
is still going to report the size used at declaration. That never changes.
If what you're interested in is observing how the length of a string changes with different assignments:
- Use an adequate buffer to store every string you're going to test.
- Use the function
strlen
in<string.h>
which will give you the actual length of the string, and not the length of your buffer, which, once declared, is constant.
edited Nov 14 '18 at 19:25
answered Nov 14 '18 at 19:21


Govind ParmarGovind Parmar
11.6k53361
11.6k53361
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307241%2fgiving-array-a-bigger-value-doesnt-increase-its-size%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bA6oLY6M,I3ZyUJII81Ss99bfIfwVI00IE,wApSVsXKfR 3WkLmkCy 3N8wysBmqTa7zIJZ,qTLsZu8 U,iBh1A30
3
Undefined behavior includes working perfectly fine.
– Neil Edelman
Nov 14 '18 at 19:15
1
Accidents will happen; it is an accident that it works. It was not guaranteed to work; it was not guaranteed to fail. You have undefined behaviour, and any response is legitimate. Also, in this context,
sizeof()
is evaluated at compile time and doesn't change the result at runtime.– Jonathan Leffler
Nov 14 '18 at 19:21