Swift crash when unwrapping UILabel in UITableView with custom cell
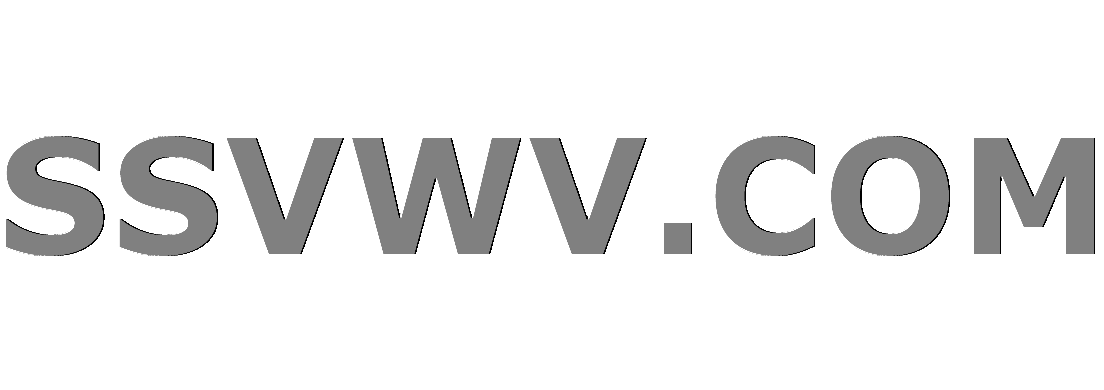
Multi tool use
I have a Table Bar where i have 3 separate MVC. The first MVC is a UITableView
where i can search for a location and it will display 10 day forecast. The second MVC also a UITableView
stores my favourite locations. I can tap on the location and it will bring me another UITableView
that displays the 10 day forecast just like the first tab bar. However i get an "Thread 1: Fatal error: Unexpectedly found nil while unwrapping an Optional value" I'm using a custom prototype cell just like the first tab bar view and it works great there.
The code for my first tab bar mvc looks like:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "WeatherDataCell", for: indexPath)
let weatherCell = timeSeries[indexPath.row]
if let wc = cell as? WeatherTableViewCell
wc.timeSeries = weatherCell
return cell
The custom prototype cell looks something like:
class WeatherTableViewCell: UITableViewCell {
@IBOutlet weak var WeatherImageView: UIImageView!
@IBOutlet weak var WeatherTimeLabel: UILabel!
@IBOutlet weak var WeatherTemperatureLabel: UILabel!
@IBOutlet weak var WeatherWindLabel: UILabel!
@IBOutlet weak var WeatherPrecipitationLabel: UILabel!
var timeSeries : TimeSeries?
didSet
updateUI()
func updateUI()
for parameters in timeSeries!.parameters
if parameters.name == "t"
let temperature = parameters.values[0]
WeatherTemperatureLabel.text? = "(temperature) °C" // <- works for the first MVC but crashes on the second MVC even though i can print out the temperature
The func in the seconds MVC basically looks the same as the first one so i don't understand why its crashing, it should have data.
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "DetailedWeatherCell", for: indexPath) as! WeatherTableViewCell
let weatherCell = timeSeries[indexPath.row]
let wc = cell
wc.timeSeries = weatherCell
return cell
added additional code for clarity (my FavoriteDetailedTableViewController class):
override func viewDidLoad()
super.viewDidLoad()
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
ios swift uitableview uilabel optional
add a comment |
I have a Table Bar where i have 3 separate MVC. The first MVC is a UITableView
where i can search for a location and it will display 10 day forecast. The second MVC also a UITableView
stores my favourite locations. I can tap on the location and it will bring me another UITableView
that displays the 10 day forecast just like the first tab bar. However i get an "Thread 1: Fatal error: Unexpectedly found nil while unwrapping an Optional value" I'm using a custom prototype cell just like the first tab bar view and it works great there.
The code for my first tab bar mvc looks like:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "WeatherDataCell", for: indexPath)
let weatherCell = timeSeries[indexPath.row]
if let wc = cell as? WeatherTableViewCell
wc.timeSeries = weatherCell
return cell
The custom prototype cell looks something like:
class WeatherTableViewCell: UITableViewCell {
@IBOutlet weak var WeatherImageView: UIImageView!
@IBOutlet weak var WeatherTimeLabel: UILabel!
@IBOutlet weak var WeatherTemperatureLabel: UILabel!
@IBOutlet weak var WeatherWindLabel: UILabel!
@IBOutlet weak var WeatherPrecipitationLabel: UILabel!
var timeSeries : TimeSeries?
didSet
updateUI()
func updateUI()
for parameters in timeSeries!.parameters
if parameters.name == "t"
let temperature = parameters.values[0]
WeatherTemperatureLabel.text? = "(temperature) °C" // <- works for the first MVC but crashes on the second MVC even though i can print out the temperature
The func in the seconds MVC basically looks the same as the first one so i don't understand why its crashing, it should have data.
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "DetailedWeatherCell", for: indexPath) as! WeatherTableViewCell
let weatherCell = timeSeries[indexPath.row]
let wc = cell
wc.timeSeries = weatherCell
return cell
added additional code for clarity (my FavoriteDetailedTableViewController class):
override func viewDidLoad()
super.viewDidLoad()
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
ios swift uitableview uilabel optional
You're force unwrapping this:for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's atimeseries
that's expected, but isn't there. You should use anif let
orguard let
for unwrappingtimeseries
– Adrian
Nov 12 '18 at 21:24
Check ifWeatherTemperatureLabel
is linked in storyboard or not
– Satish
Nov 12 '18 at 21:34
add a comment |
I have a Table Bar where i have 3 separate MVC. The first MVC is a UITableView
where i can search for a location and it will display 10 day forecast. The second MVC also a UITableView
stores my favourite locations. I can tap on the location and it will bring me another UITableView
that displays the 10 day forecast just like the first tab bar. However i get an "Thread 1: Fatal error: Unexpectedly found nil while unwrapping an Optional value" I'm using a custom prototype cell just like the first tab bar view and it works great there.
The code for my first tab bar mvc looks like:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "WeatherDataCell", for: indexPath)
let weatherCell = timeSeries[indexPath.row]
if let wc = cell as? WeatherTableViewCell
wc.timeSeries = weatherCell
return cell
The custom prototype cell looks something like:
class WeatherTableViewCell: UITableViewCell {
@IBOutlet weak var WeatherImageView: UIImageView!
@IBOutlet weak var WeatherTimeLabel: UILabel!
@IBOutlet weak var WeatherTemperatureLabel: UILabel!
@IBOutlet weak var WeatherWindLabel: UILabel!
@IBOutlet weak var WeatherPrecipitationLabel: UILabel!
var timeSeries : TimeSeries?
didSet
updateUI()
func updateUI()
for parameters in timeSeries!.parameters
if parameters.name == "t"
let temperature = parameters.values[0]
WeatherTemperatureLabel.text? = "(temperature) °C" // <- works for the first MVC but crashes on the second MVC even though i can print out the temperature
The func in the seconds MVC basically looks the same as the first one so i don't understand why its crashing, it should have data.
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "DetailedWeatherCell", for: indexPath) as! WeatherTableViewCell
let weatherCell = timeSeries[indexPath.row]
let wc = cell
wc.timeSeries = weatherCell
return cell
added additional code for clarity (my FavoriteDetailedTableViewController class):
override func viewDidLoad()
super.viewDidLoad()
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
ios swift uitableview uilabel optional
I have a Table Bar where i have 3 separate MVC. The first MVC is a UITableView
where i can search for a location and it will display 10 day forecast. The second MVC also a UITableView
stores my favourite locations. I can tap on the location and it will bring me another UITableView
that displays the 10 day forecast just like the first tab bar. However i get an "Thread 1: Fatal error: Unexpectedly found nil while unwrapping an Optional value" I'm using a custom prototype cell just like the first tab bar view and it works great there.
The code for my first tab bar mvc looks like:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "WeatherDataCell", for: indexPath)
let weatherCell = timeSeries[indexPath.row]
if let wc = cell as? WeatherTableViewCell
wc.timeSeries = weatherCell
return cell
The custom prototype cell looks something like:
class WeatherTableViewCell: UITableViewCell {
@IBOutlet weak var WeatherImageView: UIImageView!
@IBOutlet weak var WeatherTimeLabel: UILabel!
@IBOutlet weak var WeatherTemperatureLabel: UILabel!
@IBOutlet weak var WeatherWindLabel: UILabel!
@IBOutlet weak var WeatherPrecipitationLabel: UILabel!
var timeSeries : TimeSeries?
didSet
updateUI()
func updateUI()
for parameters in timeSeries!.parameters
if parameters.name == "t"
let temperature = parameters.values[0]
WeatherTemperatureLabel.text? = "(temperature) °C" // <- works for the first MVC but crashes on the second MVC even though i can print out the temperature
The func in the seconds MVC basically looks the same as the first one so i don't understand why its crashing, it should have data.
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
let cell = tableView.dequeueReusableCell(withIdentifier: "DetailedWeatherCell", for: indexPath) as! WeatherTableViewCell
let weatherCell = timeSeries[indexPath.row]
let wc = cell
wc.timeSeries = weatherCell
return cell
added additional code for clarity (my FavoriteDetailedTableViewController class):
override func viewDidLoad()
super.viewDidLoad()
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
ios swift uitableview uilabel optional
ios swift uitableview uilabel optional
edited Nov 12 '18 at 21:21
asked Nov 12 '18 at 21:09
xxFlashxx
1168
1168
You're force unwrapping this:for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's atimeseries
that's expected, but isn't there. You should use anif let
orguard let
for unwrappingtimeseries
– Adrian
Nov 12 '18 at 21:24
Check ifWeatherTemperatureLabel
is linked in storyboard or not
– Satish
Nov 12 '18 at 21:34
add a comment |
You're force unwrapping this:for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's atimeseries
that's expected, but isn't there. You should use anif let
orguard let
for unwrappingtimeseries
– Adrian
Nov 12 '18 at 21:24
Check ifWeatherTemperatureLabel
is linked in storyboard or not
– Satish
Nov 12 '18 at 21:34
You're force unwrapping this:
for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's a timeseries
that's expected, but isn't there. You should use an if let
or guard let
for unwrapping timeseries
– Adrian
Nov 12 '18 at 21:24
You're force unwrapping this:
for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's a timeseries
that's expected, but isn't there. You should use an if let
or guard let
for unwrapping timeseries
– Adrian
Nov 12 '18 at 21:24
Check if
WeatherTemperatureLabel
is linked in storyboard or not– Satish
Nov 12 '18 at 21:34
Check if
WeatherTemperatureLabel
is linked in storyboard or not– Satish
Nov 12 '18 at 21:34
add a comment |
2 Answers
2
active
oldest
votes
I think you forgot to change identifier of prototype cell in FavoritedDetailedTableViewController. In this TableViewController change it to
and it should work.
Second you maybe forgot to set your cell class here
Also delete this line from viewDidLoad
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
|
show 5 more comments
- 2 Viewcontrollers can not reuse the same prototype cell which had been created by one of two viewcontrollers
- WeatherTableViewCell can't have 2 layouts in mvc1, mvc2
=> You have to create your customer cell in xib file and register a cell for UITableViewCell reuse.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270147%2fswift-crash-when-unwrapping-uilabel-in-uitableview-with-custom-cell%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you forgot to change identifier of prototype cell in FavoritedDetailedTableViewController. In this TableViewController change it to
and it should work.
Second you maybe forgot to set your cell class here
Also delete this line from viewDidLoad
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
|
show 5 more comments
I think you forgot to change identifier of prototype cell in FavoritedDetailedTableViewController. In this TableViewController change it to
and it should work.
Second you maybe forgot to set your cell class here
Also delete this line from viewDidLoad
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
|
show 5 more comments
I think you forgot to change identifier of prototype cell in FavoritedDetailedTableViewController. In this TableViewController change it to
and it should work.
Second you maybe forgot to set your cell class here
Also delete this line from viewDidLoad
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
I think you forgot to change identifier of prototype cell in FavoritedDetailedTableViewController. In this TableViewController change it to
and it should work.
Second you maybe forgot to set your cell class here
Also delete this line from viewDidLoad
self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
edited Nov 12 '18 at 22:21
answered Nov 12 '18 at 21:17


Robert Dresler
4,3741526
4,3741526
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
|
show 5 more comments
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
Did u mean self.tableView.register(WeatherTableViewCell.self, forCellReuseIdentifier: "DetailedWeatherCell")
– xxFlashxx
Nov 12 '18 at 21:18
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
If the cells are designed in IB as prototype cells you must not register the cells.
– vadian
Nov 12 '18 at 21:22
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@xxFlashxx check edited answer
– Robert Dresler
Nov 12 '18 at 21:26
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@Robert Dresler I did as you said but i got another crash now: "Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'unable to dequeue a cell with identifier WeatherDataCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'"
– xxFlashxx
Nov 12 '18 at 21:29
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
@xxFlashxx ok, let code as it was and try just change reuse identifier in storyboard and delete this one line where you register cell as I described in edited answer
– Robert Dresler
Nov 12 '18 at 21:32
|
show 5 more comments
- 2 Viewcontrollers can not reuse the same prototype cell which had been created by one of two viewcontrollers
- WeatherTableViewCell can't have 2 layouts in mvc1, mvc2
=> You have to create your customer cell in xib file and register a cell for UITableViewCell reuse.
add a comment |
- 2 Viewcontrollers can not reuse the same prototype cell which had been created by one of two viewcontrollers
- WeatherTableViewCell can't have 2 layouts in mvc1, mvc2
=> You have to create your customer cell in xib file and register a cell for UITableViewCell reuse.
add a comment |
- 2 Viewcontrollers can not reuse the same prototype cell which had been created by one of two viewcontrollers
- WeatherTableViewCell can't have 2 layouts in mvc1, mvc2
=> You have to create your customer cell in xib file and register a cell for UITableViewCell reuse.
- 2 Viewcontrollers can not reuse the same prototype cell which had been created by one of two viewcontrollers
- WeatherTableViewCell can't have 2 layouts in mvc1, mvc2
=> You have to create your customer cell in xib file and register a cell for UITableViewCell reuse.
answered Nov 13 '18 at 3:27
Nguyen Hoan
1,139616
1,139616
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270147%2fswift-crash-when-unwrapping-uilabel-in-uitableview-with-custom-cell%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
U,XyrriGcN,9d4 YW7Hgk
You're force unwrapping this:
for parameters in timeSeries!.parameters
. There's probably a nil value in there that blows up when there's atimeseries
that's expected, but isn't there. You should use anif let
orguard let
for unwrappingtimeseries
– Adrian
Nov 12 '18 at 21:24
Check if
WeatherTemperatureLabel
is linked in storyboard or not– Satish
Nov 12 '18 at 21:34