dry-struct How to conditionally validate one attribute?
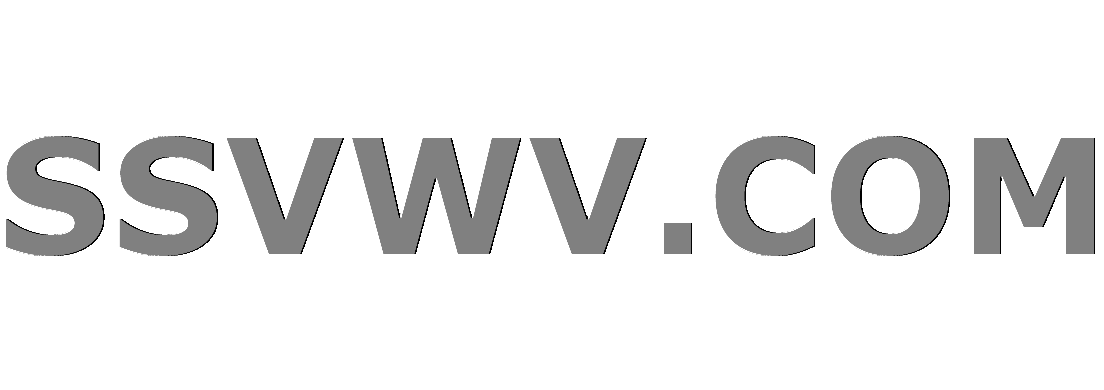
Multi tool use
I'm using dry-types and dry-struct and I would like to have a conditional validation.
for the class:
class Tax < Dry::Struct
attribute :tax_type, Types::String.constrained(min_size: 2, max_size: 3, included_in: %w[IVA IS NS])
attribute :tax_country_region, Types::String.constrained(max_size: 5)
attribute :tax_code, Types::String.constrained(max_size: 10)
attribute :description, Types::String.constrained(max_size: 255)
attribute :tax_percentage, Types::Integer
attribute :tax_ammount, Types::Integer.optional
end
I want to validate tax_ammount
as an Integer and mandatory if `tax_type == 'IS'.
ruby dry-rb dry-types dry-struct
add a comment |
I'm using dry-types and dry-struct and I would like to have a conditional validation.
for the class:
class Tax < Dry::Struct
attribute :tax_type, Types::String.constrained(min_size: 2, max_size: 3, included_in: %w[IVA IS NS])
attribute :tax_country_region, Types::String.constrained(max_size: 5)
attribute :tax_code, Types::String.constrained(max_size: 10)
attribute :description, Types::String.constrained(max_size: 255)
attribute :tax_percentage, Types::Integer
attribute :tax_ammount, Types::Integer.optional
end
I want to validate tax_ammount
as an Integer and mandatory if `tax_type == 'IS'.
ruby dry-rb dry-types dry-struct
add a comment |
I'm using dry-types and dry-struct and I would like to have a conditional validation.
for the class:
class Tax < Dry::Struct
attribute :tax_type, Types::String.constrained(min_size: 2, max_size: 3, included_in: %w[IVA IS NS])
attribute :tax_country_region, Types::String.constrained(max_size: 5)
attribute :tax_code, Types::String.constrained(max_size: 10)
attribute :description, Types::String.constrained(max_size: 255)
attribute :tax_percentage, Types::Integer
attribute :tax_ammount, Types::Integer.optional
end
I want to validate tax_ammount
as an Integer and mandatory if `tax_type == 'IS'.
ruby dry-rb dry-types dry-struct
I'm using dry-types and dry-struct and I would like to have a conditional validation.
for the class:
class Tax < Dry::Struct
attribute :tax_type, Types::String.constrained(min_size: 2, max_size: 3, included_in: %w[IVA IS NS])
attribute :tax_country_region, Types::String.constrained(max_size: 5)
attribute :tax_code, Types::String.constrained(max_size: 10)
attribute :description, Types::String.constrained(max_size: 255)
attribute :tax_percentage, Types::Integer
attribute :tax_ammount, Types::Integer.optional
end
I want to validate tax_ammount
as an Integer and mandatory if `tax_type == 'IS'.
ruby dry-rb dry-types dry-struct
ruby dry-rb dry-types dry-struct
asked Nov 12 '18 at 20:54


Paulo Fidalgo
15.9k66493
15.9k66493
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
dry-struct
is really for basic type assertion and coercion.
If you want more complex validation then you probably want to implement dry-validation
as well (as recommended by dry-rb
)
See Validating data with dry-struct which states
Please don’t. Structs are meant to work with valid input, it cannot generate error messages good enough for displaying them for a user etc. Use dry-validation for validating incoming data and then pass its output to structs.
The conditional validation using dry-validation
would be something like
TaxValidation = Dry::Validation.Schema do
# Could be:
# required(:tax_type).filled(:str?,
# size?: 2..3,
# included_in?: %w(IVA IS NS))
# but since we are validating against a list of Strings I figured the rest was implied
required(:tax_type).filled(included_in?: %w(IVA IS NS))
optional(:tax_amount).maybe(:int?)
# rule name is of your choosing and will be used
# as the errors key (i just chose `tax_amount` for consistency)
rule(tax_amount:[:tax_type, :tax_amount]) do |tax_type, tax_amount|
tax_type.eql?('IS').then(tax_amount.filled?)
end
end
- This requires
tax_type
to be in the%w(IVA IS NS)
list; - Allows
tax_amount
to be optional but if it is filled in it must be anInteger
(int?
) and; - If
tax_type == 'IS'
(eql?('IS')
) thentax_amount
must be filled in (which means it must be anInteger
based on the rule above).
Obviously you can validate your other inputs as well but I left these out for the sake of brevity.
Examples:
TaxValidation.().success?
#=> false
TaxValidation.().errors
# => :tax_type=>["is missing"]
TaxValidation.(tax_type: 'NO').errors
#=> :tax_type=>["must be one of: IVA, IS, NS"]
TaxValidation.(tax_type: 'NS').errors
#=>
TaxValidation.(tax_type: 'IS').errors
#=> :tax_amount=>["must be filled"]
TaxValidation.(tax_type: 'IS',tax_amount:'NO').errors
#=> :tax_amount=>["must be an integer"]
TaxValidation.(tax_type: 'NS',tax_amount:12).errors
#=>
TaxValidation.(tax_type: 'NS',tax_amount:12).success?
#=> true
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53269946%2fdry-struct-how-to-conditionally-validate-one-attribute%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
dry-struct
is really for basic type assertion and coercion.
If you want more complex validation then you probably want to implement dry-validation
as well (as recommended by dry-rb
)
See Validating data with dry-struct which states
Please don’t. Structs are meant to work with valid input, it cannot generate error messages good enough for displaying them for a user etc. Use dry-validation for validating incoming data and then pass its output to structs.
The conditional validation using dry-validation
would be something like
TaxValidation = Dry::Validation.Schema do
# Could be:
# required(:tax_type).filled(:str?,
# size?: 2..3,
# included_in?: %w(IVA IS NS))
# but since we are validating against a list of Strings I figured the rest was implied
required(:tax_type).filled(included_in?: %w(IVA IS NS))
optional(:tax_amount).maybe(:int?)
# rule name is of your choosing and will be used
# as the errors key (i just chose `tax_amount` for consistency)
rule(tax_amount:[:tax_type, :tax_amount]) do |tax_type, tax_amount|
tax_type.eql?('IS').then(tax_amount.filled?)
end
end
- This requires
tax_type
to be in the%w(IVA IS NS)
list; - Allows
tax_amount
to be optional but if it is filled in it must be anInteger
(int?
) and; - If
tax_type == 'IS'
(eql?('IS')
) thentax_amount
must be filled in (which means it must be anInteger
based on the rule above).
Obviously you can validate your other inputs as well but I left these out for the sake of brevity.
Examples:
TaxValidation.().success?
#=> false
TaxValidation.().errors
# => :tax_type=>["is missing"]
TaxValidation.(tax_type: 'NO').errors
#=> :tax_type=>["must be one of: IVA, IS, NS"]
TaxValidation.(tax_type: 'NS').errors
#=>
TaxValidation.(tax_type: 'IS').errors
#=> :tax_amount=>["must be filled"]
TaxValidation.(tax_type: 'IS',tax_amount:'NO').errors
#=> :tax_amount=>["must be an integer"]
TaxValidation.(tax_type: 'NS',tax_amount:12).errors
#=>
TaxValidation.(tax_type: 'NS',tax_amount:12).success?
#=> true
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
add a comment |
dry-struct
is really for basic type assertion and coercion.
If you want more complex validation then you probably want to implement dry-validation
as well (as recommended by dry-rb
)
See Validating data with dry-struct which states
Please don’t. Structs are meant to work with valid input, it cannot generate error messages good enough for displaying them for a user etc. Use dry-validation for validating incoming data and then pass its output to structs.
The conditional validation using dry-validation
would be something like
TaxValidation = Dry::Validation.Schema do
# Could be:
# required(:tax_type).filled(:str?,
# size?: 2..3,
# included_in?: %w(IVA IS NS))
# but since we are validating against a list of Strings I figured the rest was implied
required(:tax_type).filled(included_in?: %w(IVA IS NS))
optional(:tax_amount).maybe(:int?)
# rule name is of your choosing and will be used
# as the errors key (i just chose `tax_amount` for consistency)
rule(tax_amount:[:tax_type, :tax_amount]) do |tax_type, tax_amount|
tax_type.eql?('IS').then(tax_amount.filled?)
end
end
- This requires
tax_type
to be in the%w(IVA IS NS)
list; - Allows
tax_amount
to be optional but if it is filled in it must be anInteger
(int?
) and; - If
tax_type == 'IS'
(eql?('IS')
) thentax_amount
must be filled in (which means it must be anInteger
based on the rule above).
Obviously you can validate your other inputs as well but I left these out for the sake of brevity.
Examples:
TaxValidation.().success?
#=> false
TaxValidation.().errors
# => :tax_type=>["is missing"]
TaxValidation.(tax_type: 'NO').errors
#=> :tax_type=>["must be one of: IVA, IS, NS"]
TaxValidation.(tax_type: 'NS').errors
#=>
TaxValidation.(tax_type: 'IS').errors
#=> :tax_amount=>["must be filled"]
TaxValidation.(tax_type: 'IS',tax_amount:'NO').errors
#=> :tax_amount=>["must be an integer"]
TaxValidation.(tax_type: 'NS',tax_amount:12).errors
#=>
TaxValidation.(tax_type: 'NS',tax_amount:12).success?
#=> true
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
add a comment |
dry-struct
is really for basic type assertion and coercion.
If you want more complex validation then you probably want to implement dry-validation
as well (as recommended by dry-rb
)
See Validating data with dry-struct which states
Please don’t. Structs are meant to work with valid input, it cannot generate error messages good enough for displaying them for a user etc. Use dry-validation for validating incoming data and then pass its output to structs.
The conditional validation using dry-validation
would be something like
TaxValidation = Dry::Validation.Schema do
# Could be:
# required(:tax_type).filled(:str?,
# size?: 2..3,
# included_in?: %w(IVA IS NS))
# but since we are validating against a list of Strings I figured the rest was implied
required(:tax_type).filled(included_in?: %w(IVA IS NS))
optional(:tax_amount).maybe(:int?)
# rule name is of your choosing and will be used
# as the errors key (i just chose `tax_amount` for consistency)
rule(tax_amount:[:tax_type, :tax_amount]) do |tax_type, tax_amount|
tax_type.eql?('IS').then(tax_amount.filled?)
end
end
- This requires
tax_type
to be in the%w(IVA IS NS)
list; - Allows
tax_amount
to be optional but if it is filled in it must be anInteger
(int?
) and; - If
tax_type == 'IS'
(eql?('IS')
) thentax_amount
must be filled in (which means it must be anInteger
based on the rule above).
Obviously you can validate your other inputs as well but I left these out for the sake of brevity.
Examples:
TaxValidation.().success?
#=> false
TaxValidation.().errors
# => :tax_type=>["is missing"]
TaxValidation.(tax_type: 'NO').errors
#=> :tax_type=>["must be one of: IVA, IS, NS"]
TaxValidation.(tax_type: 'NS').errors
#=>
TaxValidation.(tax_type: 'IS').errors
#=> :tax_amount=>["must be filled"]
TaxValidation.(tax_type: 'IS',tax_amount:'NO').errors
#=> :tax_amount=>["must be an integer"]
TaxValidation.(tax_type: 'NS',tax_amount:12).errors
#=>
TaxValidation.(tax_type: 'NS',tax_amount:12).success?
#=> true
dry-struct
is really for basic type assertion and coercion.
If you want more complex validation then you probably want to implement dry-validation
as well (as recommended by dry-rb
)
See Validating data with dry-struct which states
Please don’t. Structs are meant to work with valid input, it cannot generate error messages good enough for displaying them for a user etc. Use dry-validation for validating incoming data and then pass its output to structs.
The conditional validation using dry-validation
would be something like
TaxValidation = Dry::Validation.Schema do
# Could be:
# required(:tax_type).filled(:str?,
# size?: 2..3,
# included_in?: %w(IVA IS NS))
# but since we are validating against a list of Strings I figured the rest was implied
required(:tax_type).filled(included_in?: %w(IVA IS NS))
optional(:tax_amount).maybe(:int?)
# rule name is of your choosing and will be used
# as the errors key (i just chose `tax_amount` for consistency)
rule(tax_amount:[:tax_type, :tax_amount]) do |tax_type, tax_amount|
tax_type.eql?('IS').then(tax_amount.filled?)
end
end
- This requires
tax_type
to be in the%w(IVA IS NS)
list; - Allows
tax_amount
to be optional but if it is filled in it must be anInteger
(int?
) and; - If
tax_type == 'IS'
(eql?('IS')
) thentax_amount
must be filled in (which means it must be anInteger
based on the rule above).
Obviously you can validate your other inputs as well but I left these out for the sake of brevity.
Examples:
TaxValidation.().success?
#=> false
TaxValidation.().errors
# => :tax_type=>["is missing"]
TaxValidation.(tax_type: 'NO').errors
#=> :tax_type=>["must be one of: IVA, IS, NS"]
TaxValidation.(tax_type: 'NS').errors
#=>
TaxValidation.(tax_type: 'IS').errors
#=> :tax_amount=>["must be filled"]
TaxValidation.(tax_type: 'IS',tax_amount:'NO').errors
#=> :tax_amount=>["must be an integer"]
TaxValidation.(tax_type: 'NS',tax_amount:12).errors
#=>
TaxValidation.(tax_type: 'NS',tax_amount:12).success?
#=> true
edited Nov 12 '18 at 23:02
answered Nov 12 '18 at 21:14
engineersmnky
13.2k12138
13.2k12138
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
add a comment |
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
Even knowing that dry-struct is not meant to use as validator, for the most cases I have (data mapping between the database and XML) is fit's perfectly, as it's simple and efective. For more complex cases, your sugestion works fine, thank you.
– Paulo Fidalgo
Nov 13 '18 at 15:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53269946%2fdry-struct-how-to-conditionally-validate-one-attribute%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9GY8KsfSiMc9gwOsSB R0eoY t ct p,gh,u9OuTJ