Double label while adding a datapoint in mschart
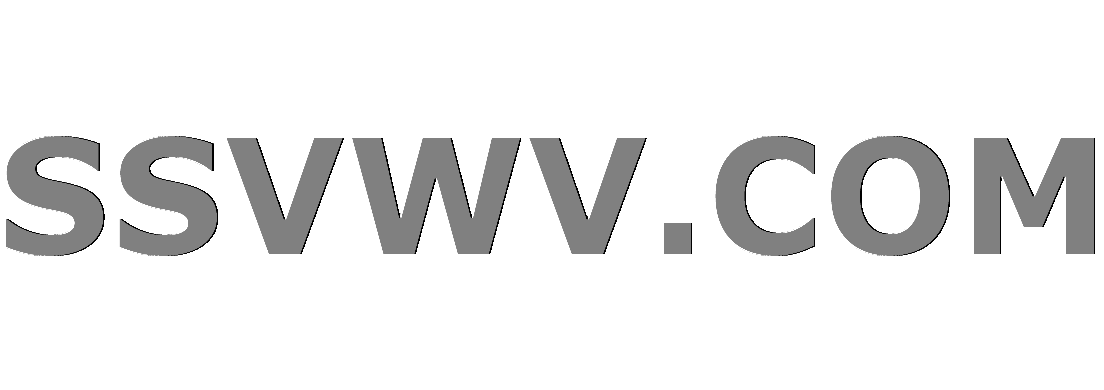
Multi tool use
I'm writing a code in C#.Net WinForms to add label on clicking some datapoints on chart.
Before clicking the chart, the graph looks like this.
Now I click a datapoint between 330-340. The chart shows selected datapoint with double label (338.61). As shown below:
Below is the debug output:
Here is the below code for chart_mouseclick
private void chart1_MouseClick(object sender, MouseEventArgs e)
if (e.Button == MouseButtons.Left)
double mouse_Xvalue = chart1.ChartAreas[0].AxisX.PixelPositionToValue(e.X);
double mouse_Yvalue = chart1.ChartAreas[0].AxisY.PixelPositionToValue(e.Y);
DataPoint Prev_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue >= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.First()).First();
DataPoint Next_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue <= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.Last()).Last();
DataPoint Add_DataPoint = Math.Abs(Prev_DataPoint.XValue - mouse_Xvalue) < Math.Abs(Next_DataPoint.XValue - mouse_Xvalue) ? Prev_DataPoint : Next_DataPoint;
int add_data_point_index = chart1.Series[0].Points.IndexOf(Add_DataPoint);
DataPoint max = Add_DataPoint;
for (int i = add_data_point_index - 10; i <= add_data_point_index + 10; i++)
DataPoint dp = chart1.Series[0].Points[i];
if (dp.YValues[0] > max.YValues[0])
add_data_point_index = i;
max = dp;
chart1.Series[1].Points.Add(max);
chart1.Series[1].Sort(PointSortOrder.Ascending, "X");
for (int i = 0; i < chart1.Series[1].Points.Count; i++)
chart1.Series[1].Points[i].Label =
Math.Round(chart1.Series[1].Points[i].XValue, 2).ToString();
What is wrong with the code ?
c# .net charts label mschart
|
show 13 more comments
I'm writing a code in C#.Net WinForms to add label on clicking some datapoints on chart.
Before clicking the chart, the graph looks like this.
Now I click a datapoint between 330-340. The chart shows selected datapoint with double label (338.61). As shown below:
Below is the debug output:
Here is the below code for chart_mouseclick
private void chart1_MouseClick(object sender, MouseEventArgs e)
if (e.Button == MouseButtons.Left)
double mouse_Xvalue = chart1.ChartAreas[0].AxisX.PixelPositionToValue(e.X);
double mouse_Yvalue = chart1.ChartAreas[0].AxisY.PixelPositionToValue(e.Y);
DataPoint Prev_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue >= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.First()).First();
DataPoint Next_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue <= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.Last()).Last();
DataPoint Add_DataPoint = Math.Abs(Prev_DataPoint.XValue - mouse_Xvalue) < Math.Abs(Next_DataPoint.XValue - mouse_Xvalue) ? Prev_DataPoint : Next_DataPoint;
int add_data_point_index = chart1.Series[0].Points.IndexOf(Add_DataPoint);
DataPoint max = Add_DataPoint;
for (int i = add_data_point_index - 10; i <= add_data_point_index + 10; i++)
DataPoint dp = chart1.Series[0].Points[i];
if (dp.YValues[0] > max.YValues[0])
add_data_point_index = i;
max = dp;
chart1.Series[1].Points.Add(max);
chart1.Series[1].Sort(PointSortOrder.Ascending, "X");
for (int i = 0; i < chart1.Series[1].Points.Count; i++)
chart1.Series[1].Points[i].Label =
Math.Round(chart1.Series[1].Points[i].XValue, 2).ToString();
What is wrong with the code ?
c# .net charts label mschart
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
@AccessDenied There is no duplicate points. I have checked theSeries.Point.Count
before and after adding a datapoint.Series.Point.Count
increases by one only, after a click.
– user2587
Nov 13 '18 at 5:14
1
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48
|
show 13 more comments
I'm writing a code in C#.Net WinForms to add label on clicking some datapoints on chart.
Before clicking the chart, the graph looks like this.
Now I click a datapoint between 330-340. The chart shows selected datapoint with double label (338.61). As shown below:
Below is the debug output:
Here is the below code for chart_mouseclick
private void chart1_MouseClick(object sender, MouseEventArgs e)
if (e.Button == MouseButtons.Left)
double mouse_Xvalue = chart1.ChartAreas[0].AxisX.PixelPositionToValue(e.X);
double mouse_Yvalue = chart1.ChartAreas[0].AxisY.PixelPositionToValue(e.Y);
DataPoint Prev_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue >= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.First()).First();
DataPoint Next_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue <= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.Last()).Last();
DataPoint Add_DataPoint = Math.Abs(Prev_DataPoint.XValue - mouse_Xvalue) < Math.Abs(Next_DataPoint.XValue - mouse_Xvalue) ? Prev_DataPoint : Next_DataPoint;
int add_data_point_index = chart1.Series[0].Points.IndexOf(Add_DataPoint);
DataPoint max = Add_DataPoint;
for (int i = add_data_point_index - 10; i <= add_data_point_index + 10; i++)
DataPoint dp = chart1.Series[0].Points[i];
if (dp.YValues[0] > max.YValues[0])
add_data_point_index = i;
max = dp;
chart1.Series[1].Points.Add(max);
chart1.Series[1].Sort(PointSortOrder.Ascending, "X");
for (int i = 0; i < chart1.Series[1].Points.Count; i++)
chart1.Series[1].Points[i].Label =
Math.Round(chart1.Series[1].Points[i].XValue, 2).ToString();
What is wrong with the code ?
c# .net charts label mschart
I'm writing a code in C#.Net WinForms to add label on clicking some datapoints on chart.
Before clicking the chart, the graph looks like this.
Now I click a datapoint between 330-340. The chart shows selected datapoint with double label (338.61). As shown below:
Below is the debug output:
Here is the below code for chart_mouseclick
private void chart1_MouseClick(object sender, MouseEventArgs e)
if (e.Button == MouseButtons.Left)
double mouse_Xvalue = chart1.ChartAreas[0].AxisX.PixelPositionToValue(e.X);
double mouse_Yvalue = chart1.ChartAreas[0].AxisY.PixelPositionToValue(e.Y);
DataPoint Prev_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue >= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.First()).First();
DataPoint Next_DataPoint = chart1.Series[0].Points.Select(x => x)
.Where(x => x.XValue <= mouse_Xvalue)
.DefaultIfEmpty(chart1.Series[0].Points.Last()).Last();
DataPoint Add_DataPoint = Math.Abs(Prev_DataPoint.XValue - mouse_Xvalue) < Math.Abs(Next_DataPoint.XValue - mouse_Xvalue) ? Prev_DataPoint : Next_DataPoint;
int add_data_point_index = chart1.Series[0].Points.IndexOf(Add_DataPoint);
DataPoint max = Add_DataPoint;
for (int i = add_data_point_index - 10; i <= add_data_point_index + 10; i++)
DataPoint dp = chart1.Series[0].Points[i];
if (dp.YValues[0] > max.YValues[0])
add_data_point_index = i;
max = dp;
chart1.Series[1].Points.Add(max);
chart1.Series[1].Sort(PointSortOrder.Ascending, "X");
for (int i = 0; i < chart1.Series[1].Points.Count; i++)
chart1.Series[1].Points[i].Label =
Math.Round(chart1.Series[1].Points[i].XValue, 2).ToString();
What is wrong with the code ?
c# .net charts label mschart
c# .net charts label mschart
edited Nov 21 '18 at 9:05
user2587
asked Nov 13 '18 at 4:35
user2587user2587
818
818
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
@AccessDenied There is no duplicate points. I have checked theSeries.Point.Count
before and after adding a datapoint.Series.Point.Count
increases by one only, after a click.
– user2587
Nov 13 '18 at 5:14
1
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48
|
show 13 more comments
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
@AccessDenied There is no duplicate points. I have checked theSeries.Point.Count
before and after adding a datapoint.Series.Point.Count
increases by one only, after a click.
– user2587
Nov 13 '18 at 5:14
1
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
@AccessDenied There is no duplicate points. I have checked the
Series.Point.Count
before and after adding a datapoint. Series.Point.Count
increases by one only, after a click.– user2587
Nov 13 '18 at 5:14
@AccessDenied There is no duplicate points. I have checked the
Series.Point.Count
before and after adding a datapoint. Series.Point.Count
increases by one only, after a click.– user2587
Nov 13 '18 at 5:14
1
1
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48
|
show 13 more comments
1 Answer
1
active
oldest
votes
This is actually a quite interesting observation..
Let's look at the second version, which works fine for you:
chart1.Series[1].Points.AddXY(max.XValue, max.YValue[0])
or in short:
series1.Points.AddXY(x,y);
This is the normal way to add DataPoints
: A new point is created with the two (or more) values and all other properties taken from the Series
defaults.
The 'other' properties include colors, markers and label data, including IsValueShownAsLabel
.
No surprises here.
Now for the original version:
chart1.Series[1].Points.Add(max);
or in short
series1.Points.Add(dp);
where max
(or dp
) are DataPoints
in the first series (series0
).
This behaves surprisingly different..:
One could imagine that the DataPoint
gets moved (like Controls
would be) but it isn't. Or cloned like strings would be.
Instead only a new reference is created and added to series1.Points
. This has several surprising consequences..:
Both references point to the same datapoint object; so suddenly there is a point in series1 with properties taken from series0, including
IsValueShownAsLabel
!As both series now contain a datapoint with the same values/coordinates the chart is smart enough to display their labels slightly apart to avoid overlapping. This is the effect you see.
If you now remove the datapoint from its original series0, series1 will still contain a reference to it with color, label etc as it was in series0..
So there indeed is no way to make the original version work since two references to the same point will always make the chart display the label either twice or not at all.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273891%2fdouble-label-while-adding-a-datapoint-in-mschart%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is actually a quite interesting observation..
Let's look at the second version, which works fine for you:
chart1.Series[1].Points.AddXY(max.XValue, max.YValue[0])
or in short:
series1.Points.AddXY(x,y);
This is the normal way to add DataPoints
: A new point is created with the two (or more) values and all other properties taken from the Series
defaults.
The 'other' properties include colors, markers and label data, including IsValueShownAsLabel
.
No surprises here.
Now for the original version:
chart1.Series[1].Points.Add(max);
or in short
series1.Points.Add(dp);
where max
(or dp
) are DataPoints
in the first series (series0
).
This behaves surprisingly different..:
One could imagine that the DataPoint
gets moved (like Controls
would be) but it isn't. Or cloned like strings would be.
Instead only a new reference is created and added to series1.Points
. This has several surprising consequences..:
Both references point to the same datapoint object; so suddenly there is a point in series1 with properties taken from series0, including
IsValueShownAsLabel
!As both series now contain a datapoint with the same values/coordinates the chart is smart enough to display their labels slightly apart to avoid overlapping. This is the effect you see.
If you now remove the datapoint from its original series0, series1 will still contain a reference to it with color, label etc as it was in series0..
So there indeed is no way to make the original version work since two references to the same point will always make the chart display the label either twice or not at all.
add a comment |
This is actually a quite interesting observation..
Let's look at the second version, which works fine for you:
chart1.Series[1].Points.AddXY(max.XValue, max.YValue[0])
or in short:
series1.Points.AddXY(x,y);
This is the normal way to add DataPoints
: A new point is created with the two (or more) values and all other properties taken from the Series
defaults.
The 'other' properties include colors, markers and label data, including IsValueShownAsLabel
.
No surprises here.
Now for the original version:
chart1.Series[1].Points.Add(max);
or in short
series1.Points.Add(dp);
where max
(or dp
) are DataPoints
in the first series (series0
).
This behaves surprisingly different..:
One could imagine that the DataPoint
gets moved (like Controls
would be) but it isn't. Or cloned like strings would be.
Instead only a new reference is created and added to series1.Points
. This has several surprising consequences..:
Both references point to the same datapoint object; so suddenly there is a point in series1 with properties taken from series0, including
IsValueShownAsLabel
!As both series now contain a datapoint with the same values/coordinates the chart is smart enough to display their labels slightly apart to avoid overlapping. This is the effect you see.
If you now remove the datapoint from its original series0, series1 will still contain a reference to it with color, label etc as it was in series0..
So there indeed is no way to make the original version work since two references to the same point will always make the chart display the label either twice or not at all.
add a comment |
This is actually a quite interesting observation..
Let's look at the second version, which works fine for you:
chart1.Series[1].Points.AddXY(max.XValue, max.YValue[0])
or in short:
series1.Points.AddXY(x,y);
This is the normal way to add DataPoints
: A new point is created with the two (or more) values and all other properties taken from the Series
defaults.
The 'other' properties include colors, markers and label data, including IsValueShownAsLabel
.
No surprises here.
Now for the original version:
chart1.Series[1].Points.Add(max);
or in short
series1.Points.Add(dp);
where max
(or dp
) are DataPoints
in the first series (series0
).
This behaves surprisingly different..:
One could imagine that the DataPoint
gets moved (like Controls
would be) but it isn't. Or cloned like strings would be.
Instead only a new reference is created and added to series1.Points
. This has several surprising consequences..:
Both references point to the same datapoint object; so suddenly there is a point in series1 with properties taken from series0, including
IsValueShownAsLabel
!As both series now contain a datapoint with the same values/coordinates the chart is smart enough to display their labels slightly apart to avoid overlapping. This is the effect you see.
If you now remove the datapoint from its original series0, series1 will still contain a reference to it with color, label etc as it was in series0..
So there indeed is no way to make the original version work since two references to the same point will always make the chart display the label either twice or not at all.
This is actually a quite interesting observation..
Let's look at the second version, which works fine for you:
chart1.Series[1].Points.AddXY(max.XValue, max.YValue[0])
or in short:
series1.Points.AddXY(x,y);
This is the normal way to add DataPoints
: A new point is created with the two (or more) values and all other properties taken from the Series
defaults.
The 'other' properties include colors, markers and label data, including IsValueShownAsLabel
.
No surprises here.
Now for the original version:
chart1.Series[1].Points.Add(max);
or in short
series1.Points.Add(dp);
where max
(or dp
) are DataPoints
in the first series (series0
).
This behaves surprisingly different..:
One could imagine that the DataPoint
gets moved (like Controls
would be) but it isn't. Or cloned like strings would be.
Instead only a new reference is created and added to series1.Points
. This has several surprising consequences..:
Both references point to the same datapoint object; so suddenly there is a point in series1 with properties taken from series0, including
IsValueShownAsLabel
!As both series now contain a datapoint with the same values/coordinates the chart is smart enough to display their labels slightly apart to avoid overlapping. This is the effect you see.
If you now remove the datapoint from its original series0, series1 will still contain a reference to it with color, label etc as it was in series0..
So there indeed is no way to make the original version work since two references to the same point will always make the chart display the label either twice or not at all.
edited Nov 26 '18 at 11:44
answered Nov 26 '18 at 10:53


TaWTaW
41k62762
41k62762
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273891%2fdouble-label-while-adding-a-datapoint-in-mschart%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lkffO,rKLG1TujnvPii JU m,goGcwj8TtA3WFJtJAq
Have you tried debugging your code?
– Access Denied
Nov 13 '18 at 4:47
Yes. @AccessDenied
– user2587
Nov 13 '18 at 5:01
Do you have duplicate points in your array?
– Access Denied
Nov 13 '18 at 5:07
@AccessDenied There is no duplicate points. I have checked the
Series.Point.Count
before and after adding a datapoint.Series.Point.Count
increases by one only, after a click.– user2587
Nov 13 '18 at 5:14
1
One usually will not need to Refresh a chart. - What is your question ? Don't you add a new DataPoint? Why is it suprising that it comes with a Label?? Also: Instead of Sorting the Points you can also Insert at the correct position.
– TaW
Nov 13 '18 at 11:48