How come in this code to find prime numbers, is_prime(9) returns True?
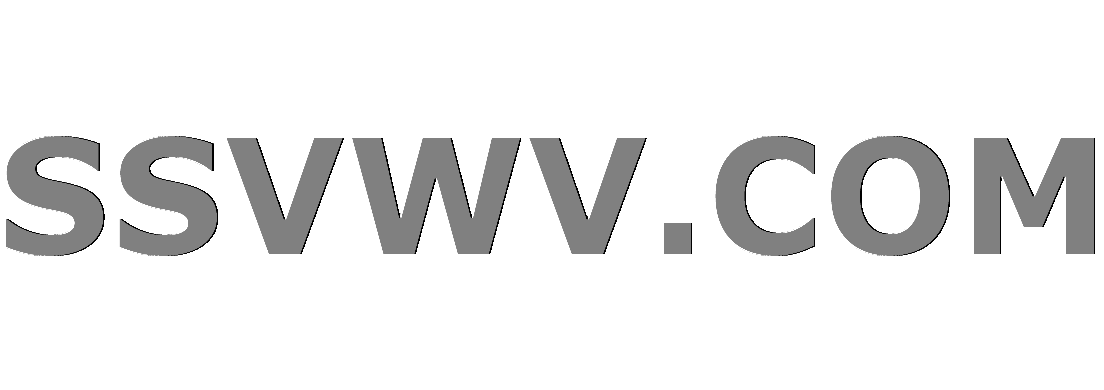
Multi tool use
up vote
1
down vote
favorite
def is_prime(x):
if x < 2:
return False
else:
for n in range(2, x):
if x % n == 0:
return False
else:
return True
print is_prime(9)
returns True
instead of False
.
I don't quite understand.
The range (2,9)
includes this list: 2,3,4,5,6,7,8
and 9 % 3 == 0
,
So how come I do not get False
as the answer of that function?
python primes
add a comment |
up vote
1
down vote
favorite
def is_prime(x):
if x < 2:
return False
else:
for n in range(2, x):
if x % n == 0:
return False
else:
return True
print is_prime(9)
returns True
instead of False
.
I don't quite understand.
The range (2,9)
includes this list: 2,3,4,5,6,7,8
and 9 % 3 == 0
,
So how come I do not get False
as the answer of that function?
python primes
3
Theelse: return True
should have one level of indent less (yes, you read that right). Also you should run the loop fromrange(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
def is_prime(x):
if x < 2:
return False
else:
for n in range(2, x):
if x % n == 0:
return False
else:
return True
print is_prime(9)
returns True
instead of False
.
I don't quite understand.
The range (2,9)
includes this list: 2,3,4,5,6,7,8
and 9 % 3 == 0
,
So how come I do not get False
as the answer of that function?
python primes
def is_prime(x):
if x < 2:
return False
else:
for n in range(2, x):
if x % n == 0:
return False
else:
return True
print is_prime(9)
returns True
instead of False
.
I don't quite understand.
The range (2,9)
includes this list: 2,3,4,5,6,7,8
and 9 % 3 == 0
,
So how come I do not get False
as the answer of that function?
python primes
python primes
edited Nov 11 at 1:59


martineau
64.7k887172
64.7k887172
asked Nov 11 at 1:08
FNM
82
82
3
Theelse: return True
should have one level of indent less (yes, you read that right). Also you should run the loop fromrange(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11
add a comment |
3
Theelse: return True
should have one level of indent less (yes, you read that right). Also you should run the loop fromrange(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11
3
3
The
else: return True
should have one level of indent less (yes, you read that right). Also you should run the loop from range(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
The
else: return True
should have one level of indent less (yes, you read that right). Also you should run the loop from range(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
This is because you don't actually loop, as you return True during the first cycle (9 % 2 == 0 is False).
Something like this should solve the problem:
def is_prime(x):
if x < 2:
return False
for n in range(2, x):
if x % n == 0:
return False
return True
add a comment |
up vote
1
down vote
You can simplify the logic a good amount by keeping your original loop and not exiting early. You can add your first conditional to your final return:
def is_prime(x):
for n in range(2, x):
if x % n == 0:
return False
return x > 2
BTW, the Sieve of Erastothenes is a pretty cool method of solving this problem in a much better run time complexity. Here's a link to a brief explanation:
https://math.stackexchange.com/questions/58799/why-in-sieve-of-erastothenes-of-n-number-you-need-to-check-and-cross-out-numbe
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
This is because you don't actually loop, as you return True during the first cycle (9 % 2 == 0 is False).
Something like this should solve the problem:
def is_prime(x):
if x < 2:
return False
for n in range(2, x):
if x % n == 0:
return False
return True
add a comment |
up vote
0
down vote
accepted
This is because you don't actually loop, as you return True during the first cycle (9 % 2 == 0 is False).
Something like this should solve the problem:
def is_prime(x):
if x < 2:
return False
for n in range(2, x):
if x % n == 0:
return False
return True
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
This is because you don't actually loop, as you return True during the first cycle (9 % 2 == 0 is False).
Something like this should solve the problem:
def is_prime(x):
if x < 2:
return False
for n in range(2, x):
if x % n == 0:
return False
return True
This is because you don't actually loop, as you return True during the first cycle (9 % 2 == 0 is False).
Something like this should solve the problem:
def is_prime(x):
if x < 2:
return False
for n in range(2, x):
if x % n == 0:
return False
return True
answered Nov 11 at 1:11


mistiru
40012
40012
add a comment |
add a comment |
up vote
1
down vote
You can simplify the logic a good amount by keeping your original loop and not exiting early. You can add your first conditional to your final return:
def is_prime(x):
for n in range(2, x):
if x % n == 0:
return False
return x > 2
BTW, the Sieve of Erastothenes is a pretty cool method of solving this problem in a much better run time complexity. Here's a link to a brief explanation:
https://math.stackexchange.com/questions/58799/why-in-sieve-of-erastothenes-of-n-number-you-need-to-check-and-cross-out-numbe
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
add a comment |
up vote
1
down vote
You can simplify the logic a good amount by keeping your original loop and not exiting early. You can add your first conditional to your final return:
def is_prime(x):
for n in range(2, x):
if x % n == 0:
return False
return x > 2
BTW, the Sieve of Erastothenes is a pretty cool method of solving this problem in a much better run time complexity. Here's a link to a brief explanation:
https://math.stackexchange.com/questions/58799/why-in-sieve-of-erastothenes-of-n-number-you-need-to-check-and-cross-out-numbe
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
add a comment |
up vote
1
down vote
up vote
1
down vote
You can simplify the logic a good amount by keeping your original loop and not exiting early. You can add your first conditional to your final return:
def is_prime(x):
for n in range(2, x):
if x % n == 0:
return False
return x > 2
BTW, the Sieve of Erastothenes is a pretty cool method of solving this problem in a much better run time complexity. Here's a link to a brief explanation:
https://math.stackexchange.com/questions/58799/why-in-sieve-of-erastothenes-of-n-number-you-need-to-check-and-cross-out-numbe
You can simplify the logic a good amount by keeping your original loop and not exiting early. You can add your first conditional to your final return:
def is_prime(x):
for n in range(2, x):
if x % n == 0:
return False
return x > 2
BTW, the Sieve of Erastothenes is a pretty cool method of solving this problem in a much better run time complexity. Here's a link to a brief explanation:
https://math.stackexchange.com/questions/58799/why-in-sieve-of-erastothenes-of-n-number-you-need-to-check-and-cross-out-numbe
edited Nov 11 at 1:26
answered Nov 11 at 1:15
LeKhan9
911111
911111
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
add a comment |
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
With this code, negative numbers, 0 and 1 will be considered as prime whereas they aren't
– mistiru
Nov 11 at 1:18
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
Great point, I made the terrible assumption the caller would take that into consideration. Added a fix.
– LeKhan9
Nov 11 at 1:26
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244958%2fhow-come-in-this-code-to-find-prime-numbers-is-prime9-returns-true%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IupmB0URt5RfCPH8v p NVLvyA4QP9MuE
3
The
else: return True
should have one level of indent less (yes, you read that right). Also you should run the loop fromrange(2, int(x ** .5))
– coldspeed
Nov 11 at 1:09
Because your loop only executes once, for 9 % 2.
– Tieson T.
Nov 11 at 1:11