How to call Cordova plugin using Angular without Ionic
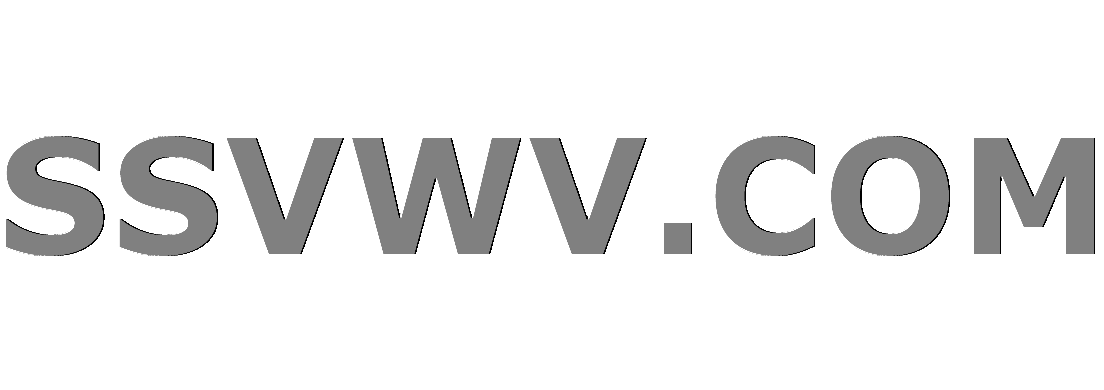
Multi tool use
I built a PWA in Angular 6 and was intending to use the Google Maps API for geolocation purposes. However, I soon realized that the geolocation services in a PWA only work when the user is interacting with the UI to deliberately request their location. My app is supposed to track the user's location in the background as they drive around, however. So when they lock the screen or go to a different application it should still track them. For the record, this is a private application and users are fully aware that they are being tracked. As a result, I converted the PWA to a hybrid app using Cordova. Everything works so far with what I had already (emulation is fine, etc), but I can't seem to figure out how to add the geolocation piece. I have installed this plugin, which appears to be installed and available. All of the examples I have seen use Ionic (which I don't need at this point) and are for the scenario where the user clicks a button to get their location, but I need it where the geolocation stuff is in a service and starts running in the background after they are logged in. I can't seem to find something that shows how to do this. Here's what I was thinking I should do:
(this isn't complete, I just pasted the example code from GitHub here and intend to fill it in with "stuff" when I know it's actually being called)
_services/geolocation.service.ts:
import Injectable from '@angular/core';
import Component, ViewChild from '@angular/core';
declare var cordova: any;
@Injectable()
export class GeolocationService
startBackgroundGeolocation()
console.log("Geolocation service called...");
cordova.plugins.BackgroundGeolocation.configure(
locationProvider: cordova.plugins.BackgroundGeolocation.ACTIVITY_PROVIDER,
desiredAccuracy: cordova.plugins.BackgroundGeolocation.HIGH_ACCURACY,
stationaryRadius: 50,
distanceFilter: 50,
notificationTitle: 'Background tracking',
notificationText: 'enabled',
debug: true,
interval: 10000,
fastestInterval: 5000,
activitiesInterval: 10000,
url: 'http://192.168.0.3:3000/location',
httpHeaders:
'X-FOO': 'bar'
,
// customize post properties
postTemplate:
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
);
cordova.plugins.BackgroundGeolocation.on('location', function(location)
// handle your locations here
// to perform long running operation on iOS
// you need to create background task
cordova.plugins.BackgroundGeolocation.startTask(function(taskKey)
// execute long running task
// eg. ajax post location
// IMPORTANT: task has to be ended by endTask
cordova.plugins.BackgroundGeolocation.endTask(taskKey);
);
);
cordova.plugins.BackgroundGeolocation.on('stationary', function(stationaryLocation)
// handle stationary locations here
);
cordova.plugins.BackgroundGeolocation.on('error', function(error)
console.log('[ERROR] cordova.plugins.BackgroundGeolocation error:', error.code, error.message);
);
cordova.plugins.BackgroundGeolocation.on('start', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been started');
);
cordova.plugins.BackgroundGeolocation.on('stop', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been stopped');
);
cordova.plugins.BackgroundGeolocation.on('authorization', function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation authorization status: ' + status);
if (status !== cordova.plugins.BackgroundGeolocation.AUTHORIZED)
// we need to set delay or otherwise alert may not be shown
setTimeout(function()
var showSettings = confirm('App requires location tracking permission. Would you like to open app settings?');
if (showSettings)
return cordova.plugins.BackgroundGeolocation.showAppSettings();
, 1000);
);
cordova.plugins.BackgroundGeolocation.on('background', function()
console.log('[INFO] App is in background');
// you can also reconfigure service (changes will be applied immediately)
cordova.plugins.BackgroundGeolocation.configure( debug: true );
);
cordova.plugins.BackgroundGeolocation.on('foreground', function()
console.log('[INFO] App is in foreground');
cordova.plugins.BackgroundGeolocation.configure( debug: false );
);
cordova.plugins.BackgroundGeolocation.on('abort_requested', function()
console.log('[INFO] Server responded with 285 Updates Not Required');
cordova.plugins.BackgroundGeolocation.stop();
// Here we can decide whether we want stop the updates or not.
// If you've configured the server to return 285, then it means the server does not require further update.
// So the normal thing to do here would be to `cordova.plugins.BackgroundGeolocation.stop()`.
// But you might be counting on it to receive location updates in the UI, so you could just reconfigure and set `url` to null.
);
cordova.plugins.BackgroundGeolocation.on('http_authorization', () =>
console.log('[INFO] App needs to authorize the http requests');
);
cordova.plugins.BackgroundGeolocation.checkStatus(function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation service is running', status.isRunning);
console.log('[INFO] cordova.plugins.BackgroundGeolocation services enabled', status.locationServicesEnabled);
console.log('[INFO] cordova.plugins.BackgroundGeolocation auth status: ' + status.authorization);
// you don't need to check status before start (this is just the example)
if (!status.isRunning)
cordova.plugins.BackgroundGeolocation.start(); //triggers start on start event
);
// you can also just start without checking for status
// cordova.plugins.BackgroundGeolocation.start();
// Don't forget to remove listeners at some point!
// cordova.plugins.BackgroundGeolocation.events.forEach(function(event)
// return cordova.plugins.BackgroundGeolocation.removeAllListeners(event);
// );
Then from app.component:
import Component, OnInit from '@angular/core';
import BrowserAnimationsModule from '@angular/platform-browser/animations';
import
transition,
trigger,
query,
style,
animate,
group,
animateChild
from '@angular/animations';
import GeolocationService from './_services/geolocation.service';
declare const device;
@Component(
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('routeAnimation', [
transition('* => *', [
query(
':enter',
[style( opacity: 0 )],
optional: true
),
query(
':leave',
[style( opacity: 1 ), animate('0.3s', style( opacity: 0 ))],
optional: true
),
query(
':enter',
[style( opacity: 0 ), animate('0.3s', style( opacity: 1 ))],
optional: true
)
])
])
]
)
export class AppComponent implements OnInit
title = 'HLD Phlebotomist App';
constructor(private geolocationService: GeolocationService)
ngOnInit()
document.addEventListener("deviceready", function()
this.geolocationService.startBackgroundGeolocation();
alert(device.platform);
, false);
However, when I run this in an android emulator, I get "Uncaught TypeError: Cannot read property 'startBackgroundGeolocation' of undefined". No idea why. Can someone help me understand the structure here? I think my problem is that I don't totally understand how to "call" the Cordova plugin.


add a comment |
I built a PWA in Angular 6 and was intending to use the Google Maps API for geolocation purposes. However, I soon realized that the geolocation services in a PWA only work when the user is interacting with the UI to deliberately request their location. My app is supposed to track the user's location in the background as they drive around, however. So when they lock the screen or go to a different application it should still track them. For the record, this is a private application and users are fully aware that they are being tracked. As a result, I converted the PWA to a hybrid app using Cordova. Everything works so far with what I had already (emulation is fine, etc), but I can't seem to figure out how to add the geolocation piece. I have installed this plugin, which appears to be installed and available. All of the examples I have seen use Ionic (which I don't need at this point) and are for the scenario where the user clicks a button to get their location, but I need it where the geolocation stuff is in a service and starts running in the background after they are logged in. I can't seem to find something that shows how to do this. Here's what I was thinking I should do:
(this isn't complete, I just pasted the example code from GitHub here and intend to fill it in with "stuff" when I know it's actually being called)
_services/geolocation.service.ts:
import Injectable from '@angular/core';
import Component, ViewChild from '@angular/core';
declare var cordova: any;
@Injectable()
export class GeolocationService
startBackgroundGeolocation()
console.log("Geolocation service called...");
cordova.plugins.BackgroundGeolocation.configure(
locationProvider: cordova.plugins.BackgroundGeolocation.ACTIVITY_PROVIDER,
desiredAccuracy: cordova.plugins.BackgroundGeolocation.HIGH_ACCURACY,
stationaryRadius: 50,
distanceFilter: 50,
notificationTitle: 'Background tracking',
notificationText: 'enabled',
debug: true,
interval: 10000,
fastestInterval: 5000,
activitiesInterval: 10000,
url: 'http://192.168.0.3:3000/location',
httpHeaders:
'X-FOO': 'bar'
,
// customize post properties
postTemplate:
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
);
cordova.plugins.BackgroundGeolocation.on('location', function(location)
// handle your locations here
// to perform long running operation on iOS
// you need to create background task
cordova.plugins.BackgroundGeolocation.startTask(function(taskKey)
// execute long running task
// eg. ajax post location
// IMPORTANT: task has to be ended by endTask
cordova.plugins.BackgroundGeolocation.endTask(taskKey);
);
);
cordova.plugins.BackgroundGeolocation.on('stationary', function(stationaryLocation)
// handle stationary locations here
);
cordova.plugins.BackgroundGeolocation.on('error', function(error)
console.log('[ERROR] cordova.plugins.BackgroundGeolocation error:', error.code, error.message);
);
cordova.plugins.BackgroundGeolocation.on('start', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been started');
);
cordova.plugins.BackgroundGeolocation.on('stop', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been stopped');
);
cordova.plugins.BackgroundGeolocation.on('authorization', function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation authorization status: ' + status);
if (status !== cordova.plugins.BackgroundGeolocation.AUTHORIZED)
// we need to set delay or otherwise alert may not be shown
setTimeout(function()
var showSettings = confirm('App requires location tracking permission. Would you like to open app settings?');
if (showSettings)
return cordova.plugins.BackgroundGeolocation.showAppSettings();
, 1000);
);
cordova.plugins.BackgroundGeolocation.on('background', function()
console.log('[INFO] App is in background');
// you can also reconfigure service (changes will be applied immediately)
cordova.plugins.BackgroundGeolocation.configure( debug: true );
);
cordova.plugins.BackgroundGeolocation.on('foreground', function()
console.log('[INFO] App is in foreground');
cordova.plugins.BackgroundGeolocation.configure( debug: false );
);
cordova.plugins.BackgroundGeolocation.on('abort_requested', function()
console.log('[INFO] Server responded with 285 Updates Not Required');
cordova.plugins.BackgroundGeolocation.stop();
// Here we can decide whether we want stop the updates or not.
// If you've configured the server to return 285, then it means the server does not require further update.
// So the normal thing to do here would be to `cordova.plugins.BackgroundGeolocation.stop()`.
// But you might be counting on it to receive location updates in the UI, so you could just reconfigure and set `url` to null.
);
cordova.plugins.BackgroundGeolocation.on('http_authorization', () =>
console.log('[INFO] App needs to authorize the http requests');
);
cordova.plugins.BackgroundGeolocation.checkStatus(function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation service is running', status.isRunning);
console.log('[INFO] cordova.plugins.BackgroundGeolocation services enabled', status.locationServicesEnabled);
console.log('[INFO] cordova.plugins.BackgroundGeolocation auth status: ' + status.authorization);
// you don't need to check status before start (this is just the example)
if (!status.isRunning)
cordova.plugins.BackgroundGeolocation.start(); //triggers start on start event
);
// you can also just start without checking for status
// cordova.plugins.BackgroundGeolocation.start();
// Don't forget to remove listeners at some point!
// cordova.plugins.BackgroundGeolocation.events.forEach(function(event)
// return cordova.plugins.BackgroundGeolocation.removeAllListeners(event);
// );
Then from app.component:
import Component, OnInit from '@angular/core';
import BrowserAnimationsModule from '@angular/platform-browser/animations';
import
transition,
trigger,
query,
style,
animate,
group,
animateChild
from '@angular/animations';
import GeolocationService from './_services/geolocation.service';
declare const device;
@Component(
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('routeAnimation', [
transition('* => *', [
query(
':enter',
[style( opacity: 0 )],
optional: true
),
query(
':leave',
[style( opacity: 1 ), animate('0.3s', style( opacity: 0 ))],
optional: true
),
query(
':enter',
[style( opacity: 0 ), animate('0.3s', style( opacity: 1 ))],
optional: true
)
])
])
]
)
export class AppComponent implements OnInit
title = 'HLD Phlebotomist App';
constructor(private geolocationService: GeolocationService)
ngOnInit()
document.addEventListener("deviceready", function()
this.geolocationService.startBackgroundGeolocation();
alert(device.platform);
, false);
However, when I run this in an android emulator, I get "Uncaught TypeError: Cannot read property 'startBackgroundGeolocation' of undefined". No idea why. Can someone help me understand the structure here? I think my problem is that I don't totally understand how to "call" the Cordova plugin.


add a comment |
I built a PWA in Angular 6 and was intending to use the Google Maps API for geolocation purposes. However, I soon realized that the geolocation services in a PWA only work when the user is interacting with the UI to deliberately request their location. My app is supposed to track the user's location in the background as they drive around, however. So when they lock the screen or go to a different application it should still track them. For the record, this is a private application and users are fully aware that they are being tracked. As a result, I converted the PWA to a hybrid app using Cordova. Everything works so far with what I had already (emulation is fine, etc), but I can't seem to figure out how to add the geolocation piece. I have installed this plugin, which appears to be installed and available. All of the examples I have seen use Ionic (which I don't need at this point) and are for the scenario where the user clicks a button to get their location, but I need it where the geolocation stuff is in a service and starts running in the background after they are logged in. I can't seem to find something that shows how to do this. Here's what I was thinking I should do:
(this isn't complete, I just pasted the example code from GitHub here and intend to fill it in with "stuff" when I know it's actually being called)
_services/geolocation.service.ts:
import Injectable from '@angular/core';
import Component, ViewChild from '@angular/core';
declare var cordova: any;
@Injectable()
export class GeolocationService
startBackgroundGeolocation()
console.log("Geolocation service called...");
cordova.plugins.BackgroundGeolocation.configure(
locationProvider: cordova.plugins.BackgroundGeolocation.ACTIVITY_PROVIDER,
desiredAccuracy: cordova.plugins.BackgroundGeolocation.HIGH_ACCURACY,
stationaryRadius: 50,
distanceFilter: 50,
notificationTitle: 'Background tracking',
notificationText: 'enabled',
debug: true,
interval: 10000,
fastestInterval: 5000,
activitiesInterval: 10000,
url: 'http://192.168.0.3:3000/location',
httpHeaders:
'X-FOO': 'bar'
,
// customize post properties
postTemplate:
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
);
cordova.plugins.BackgroundGeolocation.on('location', function(location)
// handle your locations here
// to perform long running operation on iOS
// you need to create background task
cordova.plugins.BackgroundGeolocation.startTask(function(taskKey)
// execute long running task
// eg. ajax post location
// IMPORTANT: task has to be ended by endTask
cordova.plugins.BackgroundGeolocation.endTask(taskKey);
);
);
cordova.plugins.BackgroundGeolocation.on('stationary', function(stationaryLocation)
// handle stationary locations here
);
cordova.plugins.BackgroundGeolocation.on('error', function(error)
console.log('[ERROR] cordova.plugins.BackgroundGeolocation error:', error.code, error.message);
);
cordova.plugins.BackgroundGeolocation.on('start', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been started');
);
cordova.plugins.BackgroundGeolocation.on('stop', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been stopped');
);
cordova.plugins.BackgroundGeolocation.on('authorization', function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation authorization status: ' + status);
if (status !== cordova.plugins.BackgroundGeolocation.AUTHORIZED)
// we need to set delay or otherwise alert may not be shown
setTimeout(function()
var showSettings = confirm('App requires location tracking permission. Would you like to open app settings?');
if (showSettings)
return cordova.plugins.BackgroundGeolocation.showAppSettings();
, 1000);
);
cordova.plugins.BackgroundGeolocation.on('background', function()
console.log('[INFO] App is in background');
// you can also reconfigure service (changes will be applied immediately)
cordova.plugins.BackgroundGeolocation.configure( debug: true );
);
cordova.plugins.BackgroundGeolocation.on('foreground', function()
console.log('[INFO] App is in foreground');
cordova.plugins.BackgroundGeolocation.configure( debug: false );
);
cordova.plugins.BackgroundGeolocation.on('abort_requested', function()
console.log('[INFO] Server responded with 285 Updates Not Required');
cordova.plugins.BackgroundGeolocation.stop();
// Here we can decide whether we want stop the updates or not.
// If you've configured the server to return 285, then it means the server does not require further update.
// So the normal thing to do here would be to `cordova.plugins.BackgroundGeolocation.stop()`.
// But you might be counting on it to receive location updates in the UI, so you could just reconfigure and set `url` to null.
);
cordova.plugins.BackgroundGeolocation.on('http_authorization', () =>
console.log('[INFO] App needs to authorize the http requests');
);
cordova.plugins.BackgroundGeolocation.checkStatus(function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation service is running', status.isRunning);
console.log('[INFO] cordova.plugins.BackgroundGeolocation services enabled', status.locationServicesEnabled);
console.log('[INFO] cordova.plugins.BackgroundGeolocation auth status: ' + status.authorization);
// you don't need to check status before start (this is just the example)
if (!status.isRunning)
cordova.plugins.BackgroundGeolocation.start(); //triggers start on start event
);
// you can also just start without checking for status
// cordova.plugins.BackgroundGeolocation.start();
// Don't forget to remove listeners at some point!
// cordova.plugins.BackgroundGeolocation.events.forEach(function(event)
// return cordova.plugins.BackgroundGeolocation.removeAllListeners(event);
// );
Then from app.component:
import Component, OnInit from '@angular/core';
import BrowserAnimationsModule from '@angular/platform-browser/animations';
import
transition,
trigger,
query,
style,
animate,
group,
animateChild
from '@angular/animations';
import GeolocationService from './_services/geolocation.service';
declare const device;
@Component(
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('routeAnimation', [
transition('* => *', [
query(
':enter',
[style( opacity: 0 )],
optional: true
),
query(
':leave',
[style( opacity: 1 ), animate('0.3s', style( opacity: 0 ))],
optional: true
),
query(
':enter',
[style( opacity: 0 ), animate('0.3s', style( opacity: 1 ))],
optional: true
)
])
])
]
)
export class AppComponent implements OnInit
title = 'HLD Phlebotomist App';
constructor(private geolocationService: GeolocationService)
ngOnInit()
document.addEventListener("deviceready", function()
this.geolocationService.startBackgroundGeolocation();
alert(device.platform);
, false);
However, when I run this in an android emulator, I get "Uncaught TypeError: Cannot read property 'startBackgroundGeolocation' of undefined". No idea why. Can someone help me understand the structure here? I think my problem is that I don't totally understand how to "call" the Cordova plugin.


I built a PWA in Angular 6 and was intending to use the Google Maps API for geolocation purposes. However, I soon realized that the geolocation services in a PWA only work when the user is interacting with the UI to deliberately request their location. My app is supposed to track the user's location in the background as they drive around, however. So when they lock the screen or go to a different application it should still track them. For the record, this is a private application and users are fully aware that they are being tracked. As a result, I converted the PWA to a hybrid app using Cordova. Everything works so far with what I had already (emulation is fine, etc), but I can't seem to figure out how to add the geolocation piece. I have installed this plugin, which appears to be installed and available. All of the examples I have seen use Ionic (which I don't need at this point) and are for the scenario where the user clicks a button to get their location, but I need it where the geolocation stuff is in a service and starts running in the background after they are logged in. I can't seem to find something that shows how to do this. Here's what I was thinking I should do:
(this isn't complete, I just pasted the example code from GitHub here and intend to fill it in with "stuff" when I know it's actually being called)
_services/geolocation.service.ts:
import Injectable from '@angular/core';
import Component, ViewChild from '@angular/core';
declare var cordova: any;
@Injectable()
export class GeolocationService
startBackgroundGeolocation()
console.log("Geolocation service called...");
cordova.plugins.BackgroundGeolocation.configure(
locationProvider: cordova.plugins.BackgroundGeolocation.ACTIVITY_PROVIDER,
desiredAccuracy: cordova.plugins.BackgroundGeolocation.HIGH_ACCURACY,
stationaryRadius: 50,
distanceFilter: 50,
notificationTitle: 'Background tracking',
notificationText: 'enabled',
debug: true,
interval: 10000,
fastestInterval: 5000,
activitiesInterval: 10000,
url: 'http://192.168.0.3:3000/location',
httpHeaders:
'X-FOO': 'bar'
,
// customize post properties
postTemplate:
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
);
cordova.plugins.BackgroundGeolocation.on('location', function(location)
// handle your locations here
// to perform long running operation on iOS
// you need to create background task
cordova.plugins.BackgroundGeolocation.startTask(function(taskKey)
// execute long running task
// eg. ajax post location
// IMPORTANT: task has to be ended by endTask
cordova.plugins.BackgroundGeolocation.endTask(taskKey);
);
);
cordova.plugins.BackgroundGeolocation.on('stationary', function(stationaryLocation)
// handle stationary locations here
);
cordova.plugins.BackgroundGeolocation.on('error', function(error)
console.log('[ERROR] cordova.plugins.BackgroundGeolocation error:', error.code, error.message);
);
cordova.plugins.BackgroundGeolocation.on('start', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been started');
);
cordova.plugins.BackgroundGeolocation.on('stop', function()
console.log('[INFO] cordova.plugins.BackgroundGeolocation service has been stopped');
);
cordova.plugins.BackgroundGeolocation.on('authorization', function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation authorization status: ' + status);
if (status !== cordova.plugins.BackgroundGeolocation.AUTHORIZED)
// we need to set delay or otherwise alert may not be shown
setTimeout(function()
var showSettings = confirm('App requires location tracking permission. Would you like to open app settings?');
if (showSettings)
return cordova.plugins.BackgroundGeolocation.showAppSettings();
, 1000);
);
cordova.plugins.BackgroundGeolocation.on('background', function()
console.log('[INFO] App is in background');
// you can also reconfigure service (changes will be applied immediately)
cordova.plugins.BackgroundGeolocation.configure( debug: true );
);
cordova.plugins.BackgroundGeolocation.on('foreground', function()
console.log('[INFO] App is in foreground');
cordova.plugins.BackgroundGeolocation.configure( debug: false );
);
cordova.plugins.BackgroundGeolocation.on('abort_requested', function()
console.log('[INFO] Server responded with 285 Updates Not Required');
cordova.plugins.BackgroundGeolocation.stop();
// Here we can decide whether we want stop the updates or not.
// If you've configured the server to return 285, then it means the server does not require further update.
// So the normal thing to do here would be to `cordova.plugins.BackgroundGeolocation.stop()`.
// But you might be counting on it to receive location updates in the UI, so you could just reconfigure and set `url` to null.
);
cordova.plugins.BackgroundGeolocation.on('http_authorization', () =>
console.log('[INFO] App needs to authorize the http requests');
);
cordova.plugins.BackgroundGeolocation.checkStatus(function(status)
console.log('[INFO] cordova.plugins.BackgroundGeolocation service is running', status.isRunning);
console.log('[INFO] cordova.plugins.BackgroundGeolocation services enabled', status.locationServicesEnabled);
console.log('[INFO] cordova.plugins.BackgroundGeolocation auth status: ' + status.authorization);
// you don't need to check status before start (this is just the example)
if (!status.isRunning)
cordova.plugins.BackgroundGeolocation.start(); //triggers start on start event
);
// you can also just start without checking for status
// cordova.plugins.BackgroundGeolocation.start();
// Don't forget to remove listeners at some point!
// cordova.plugins.BackgroundGeolocation.events.forEach(function(event)
// return cordova.plugins.BackgroundGeolocation.removeAllListeners(event);
// );
Then from app.component:
import Component, OnInit from '@angular/core';
import BrowserAnimationsModule from '@angular/platform-browser/animations';
import
transition,
trigger,
query,
style,
animate,
group,
animateChild
from '@angular/animations';
import GeolocationService from './_services/geolocation.service';
declare const device;
@Component(
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('routeAnimation', [
transition('* => *', [
query(
':enter',
[style( opacity: 0 )],
optional: true
),
query(
':leave',
[style( opacity: 1 ), animate('0.3s', style( opacity: 0 ))],
optional: true
),
query(
':enter',
[style( opacity: 0 ), animate('0.3s', style( opacity: 1 ))],
optional: true
)
])
])
]
)
export class AppComponent implements OnInit
title = 'HLD Phlebotomist App';
constructor(private geolocationService: GeolocationService)
ngOnInit()
document.addEventListener("deviceready", function()
this.geolocationService.startBackgroundGeolocation();
alert(device.platform);
, false);
However, when I run this in an android emulator, I get "Uncaught TypeError: Cannot read property 'startBackgroundGeolocation' of undefined". No idea why. Can someone help me understand the structure here? I think my problem is that I don't totally understand how to "call" the Cordova plugin.




asked Nov 14 '18 at 23:20
NellaGnuteNellaGnute
437
437
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The problem here was that the Cordova CLI was pulling version 2.x of the plugin, but the documentation was for 3.x. In 2.x, the plugin file was backgroundGeolocation.js, whereas 3.x changes it to BackgroundGeolocation.js, and adds a BackgroundGeolocation.d.ts file to support TypeScript implementations. Being that it's case-sensitive, it was being called by the wrong name and failing. Adding @latest to my cordova plugin add <plugin-name>
command pulled down the correct version and it started working.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310236%2fhow-to-call-cordova-plugin-using-angular-without-ionic%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem here was that the Cordova CLI was pulling version 2.x of the plugin, but the documentation was for 3.x. In 2.x, the plugin file was backgroundGeolocation.js, whereas 3.x changes it to BackgroundGeolocation.js, and adds a BackgroundGeolocation.d.ts file to support TypeScript implementations. Being that it's case-sensitive, it was being called by the wrong name and failing. Adding @latest to my cordova plugin add <plugin-name>
command pulled down the correct version and it started working.
add a comment |
The problem here was that the Cordova CLI was pulling version 2.x of the plugin, but the documentation was for 3.x. In 2.x, the plugin file was backgroundGeolocation.js, whereas 3.x changes it to BackgroundGeolocation.js, and adds a BackgroundGeolocation.d.ts file to support TypeScript implementations. Being that it's case-sensitive, it was being called by the wrong name and failing. Adding @latest to my cordova plugin add <plugin-name>
command pulled down the correct version and it started working.
add a comment |
The problem here was that the Cordova CLI was pulling version 2.x of the plugin, but the documentation was for 3.x. In 2.x, the plugin file was backgroundGeolocation.js, whereas 3.x changes it to BackgroundGeolocation.js, and adds a BackgroundGeolocation.d.ts file to support TypeScript implementations. Being that it's case-sensitive, it was being called by the wrong name and failing. Adding @latest to my cordova plugin add <plugin-name>
command pulled down the correct version and it started working.
The problem here was that the Cordova CLI was pulling version 2.x of the plugin, but the documentation was for 3.x. In 2.x, the plugin file was backgroundGeolocation.js, whereas 3.x changes it to BackgroundGeolocation.js, and adds a BackgroundGeolocation.d.ts file to support TypeScript implementations. Being that it's case-sensitive, it was being called by the wrong name and failing. Adding @latest to my cordova plugin add <plugin-name>
command pulled down the correct version and it started working.
edited Nov 19 '18 at 16:57


E_net4
12.3k63670
12.3k63670
answered Nov 17 '18 at 14:56
NellaGnuteNellaGnute
437
437
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310236%2fhow-to-call-cordova-plugin-using-angular-without-ionic%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wOffhGSSI1ekMAz86PrSU6,wax ce3eelNB08aFzR8d8yu 09ijaRhG1KG8wXg0AxHx,A4zb