Providing validation on model class level in sqlalchemy
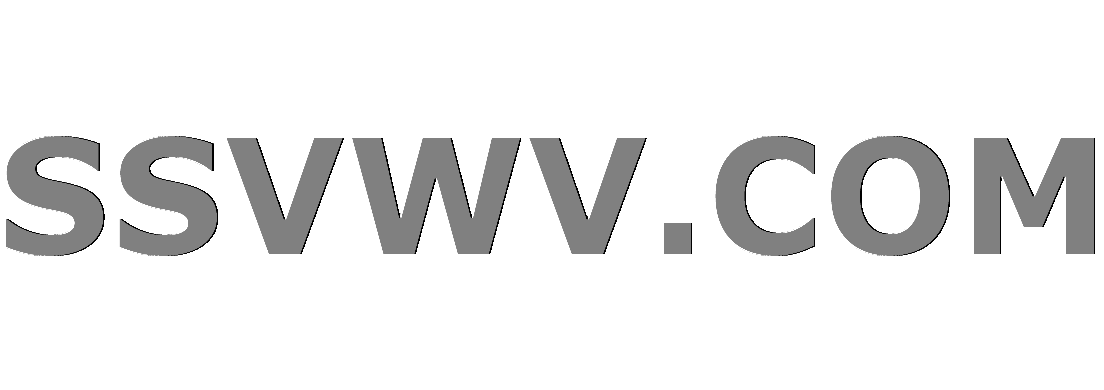
Multi tool use
I am trying to insert data to my postgres table after reading a xlsx file.
I need to validate the xlsx sheet data before I insert it into my table.
I am working on pandas dataframe df = pd.read_excel('/Users/ankitg3-mac/Downloads/medical_plans/%s' % filename)
I am using sqlalchemy as my ORM tool.
My model class:
class MedicalPlan(Base):
__tablename__ = "medical_plans"
id = Column(Integer, nullable=False , primary_key=True)
issuer_id = Column(Integer, ForeignKey('issuers.id'), nullable=False)
service_area_id = Column(Integer)
name = Column(String)
on_exchange = Column(Boolean)
off_exchange = Column(Boolean)
starting_percentage_fpl = Column(REAL, nullable=False , default=0)
ending_percentage_fpl = Column(REAL, nullable=False, default=0)
metal_level_name = Column(String)
network_type = Column(String)
type = Column(String)
is_age_29_plan = Column(Boolean)
original_medicare = Column(Boolean)
default_bhp = Column(Boolean, default=False)
sort_rank_override = Column(Integer)
recommended = Column(Boolean, default=False)
comparable_individual_plan_id_trash = Column(Integer)
group_or_individual_plan_type = Column(String)
hios_plan_identifier = Column(String)
I am doing a bulk insert using a list of dictionaries.
conn.execute(MedicalPlan.__table__.insert(), medicalPlan_dict)
My medicalPlan_dict looks like below:
[u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800001', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 484, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99806CAAUSJ-TMP1', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800002', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity']
I need to validate the data before I insert it into my table.
I read about sqlalchemy validations, and tried something like below, assuming that it will take care of the validations at the model level.
@validates('hios_plan_identifier')
def validate_hios_plan_identifier(self, key, hios_plan_identifier):
assert '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
return hios_plan_identifier
I need to put few validations on each variable. Only the rows which passes should be inserted.
I am not sure how to work on validations at model level. How can I achieve this.
python postgresql pandas sqlalchemy
|
show 12 more comments
I am trying to insert data to my postgres table after reading a xlsx file.
I need to validate the xlsx sheet data before I insert it into my table.
I am working on pandas dataframe df = pd.read_excel('/Users/ankitg3-mac/Downloads/medical_plans/%s' % filename)
I am using sqlalchemy as my ORM tool.
My model class:
class MedicalPlan(Base):
__tablename__ = "medical_plans"
id = Column(Integer, nullable=False , primary_key=True)
issuer_id = Column(Integer, ForeignKey('issuers.id'), nullable=False)
service_area_id = Column(Integer)
name = Column(String)
on_exchange = Column(Boolean)
off_exchange = Column(Boolean)
starting_percentage_fpl = Column(REAL, nullable=False , default=0)
ending_percentage_fpl = Column(REAL, nullable=False, default=0)
metal_level_name = Column(String)
network_type = Column(String)
type = Column(String)
is_age_29_plan = Column(Boolean)
original_medicare = Column(Boolean)
default_bhp = Column(Boolean, default=False)
sort_rank_override = Column(Integer)
recommended = Column(Boolean, default=False)
comparable_individual_plan_id_trash = Column(Integer)
group_or_individual_plan_type = Column(String)
hios_plan_identifier = Column(String)
I am doing a bulk insert using a list of dictionaries.
conn.execute(MedicalPlan.__table__.insert(), medicalPlan_dict)
My medicalPlan_dict looks like below:
[u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800001', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 484, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99806CAAUSJ-TMP1', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800002', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity']
I need to validate the data before I insert it into my table.
I read about sqlalchemy validations, and tried something like below, assuming that it will take care of the validations at the model level.
@validates('hios_plan_identifier')
def validate_hios_plan_identifier(self, key, hios_plan_identifier):
assert '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
return hios_plan_identifier
I need to put few validations on each variable. Only the rows which passes should be inserted.
I am not sure how to work on validations at model level. How can I achieve this.
python postgresql pandas sqlalchemy
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
This line:'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
.'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value ofhios_plan_identifier
.
– SuperShoot
Nov 13 '18 at 10:37
isre.search()
what you are looking for?
– SuperShoot
Nov 13 '18 at 10:44
1
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51
|
show 12 more comments
I am trying to insert data to my postgres table after reading a xlsx file.
I need to validate the xlsx sheet data before I insert it into my table.
I am working on pandas dataframe df = pd.read_excel('/Users/ankitg3-mac/Downloads/medical_plans/%s' % filename)
I am using sqlalchemy as my ORM tool.
My model class:
class MedicalPlan(Base):
__tablename__ = "medical_plans"
id = Column(Integer, nullable=False , primary_key=True)
issuer_id = Column(Integer, ForeignKey('issuers.id'), nullable=False)
service_area_id = Column(Integer)
name = Column(String)
on_exchange = Column(Boolean)
off_exchange = Column(Boolean)
starting_percentage_fpl = Column(REAL, nullable=False , default=0)
ending_percentage_fpl = Column(REAL, nullable=False, default=0)
metal_level_name = Column(String)
network_type = Column(String)
type = Column(String)
is_age_29_plan = Column(Boolean)
original_medicare = Column(Boolean)
default_bhp = Column(Boolean, default=False)
sort_rank_override = Column(Integer)
recommended = Column(Boolean, default=False)
comparable_individual_plan_id_trash = Column(Integer)
group_or_individual_plan_type = Column(String)
hios_plan_identifier = Column(String)
I am doing a bulk insert using a list of dictionaries.
conn.execute(MedicalPlan.__table__.insert(), medicalPlan_dict)
My medicalPlan_dict looks like below:
[u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800001', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 484, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99806CAAUSJ-TMP1', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800002', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity']
I need to validate the data before I insert it into my table.
I read about sqlalchemy validations, and tried something like below, assuming that it will take care of the validations at the model level.
@validates('hios_plan_identifier')
def validate_hios_plan_identifier(self, key, hios_plan_identifier):
assert '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
return hios_plan_identifier
I need to put few validations on each variable. Only the rows which passes should be inserted.
I am not sure how to work on validations at model level. How can I achieve this.
python postgresql pandas sqlalchemy
I am trying to insert data to my postgres table after reading a xlsx file.
I need to validate the xlsx sheet data before I insert it into my table.
I am working on pandas dataframe df = pd.read_excel('/Users/ankitg3-mac/Downloads/medical_plans/%s' % filename)
I am using sqlalchemy as my ORM tool.
My model class:
class MedicalPlan(Base):
__tablename__ = "medical_plans"
id = Column(Integer, nullable=False , primary_key=True)
issuer_id = Column(Integer, ForeignKey('issuers.id'), nullable=False)
service_area_id = Column(Integer)
name = Column(String)
on_exchange = Column(Boolean)
off_exchange = Column(Boolean)
starting_percentage_fpl = Column(REAL, nullable=False , default=0)
ending_percentage_fpl = Column(REAL, nullable=False, default=0)
metal_level_name = Column(String)
network_type = Column(String)
type = Column(String)
is_age_29_plan = Column(Boolean)
original_medicare = Column(Boolean)
default_bhp = Column(Boolean, default=False)
sort_rank_override = Column(Integer)
recommended = Column(Boolean, default=False)
comparable_individual_plan_id_trash = Column(Integer)
group_or_individual_plan_type = Column(String)
hios_plan_identifier = Column(String)
I am doing a bulk insert using a list of dictionaries.
conn.execute(MedicalPlan.__table__.insert(), medicalPlan_dict)
My medicalPlan_dict looks like below:
[u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800001', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 484, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99806CAAUSJ-TMP1', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity', u'default_bhp': False, u'price_period': u'Monthly', u'plan_description': '', u'sbc_download_url': '', u'price_note': '', u'starting_percentage_fpl': 0, u'is_uhc_plan': False, 'issuer_id': 440, u'part_b_deductible': '', u'promotional_label': '', u'metal_level_name': u'Silver', u'network_url': '', u'group_or_individual_plan_type': u'Group', u'treatment_cost_calculator_url': '', u'hios_plan_identifier': u'99844RI1800002', u'original_medicare': False, u'part_d_prescription_coverage': '', u'recommended': False, u'off_exchange': True, u'is_age_29_plan': False, u'type': u'MetalPlan', u'ending_percentage_fpl': 0, u'plan_detail_footer': '', u'formulary_url': '', u'plan_detail_items': '', u'highlight_6': '', u'highlight_4': '', u'highlight_5': '', u'hsa_eligible': False, u'highlight_3': u'PCP 20% coinsurance', u'highlight_1': u'Silver', u'name': u'WI 80 INDEMNITY 18 OPTION 1 SILVER RX $10/45/90/25%', u'network_description': '', u'plan_detail_header': '', 'service_area_id': 1, u'data_sourced_from': u'uhc', u'plan_year': 2018, u'highlight_2': u'Indemnity', u'on_exchange': False, u'network_type': u'Indemnity']
I need to validate the data before I insert it into my table.
I read about sqlalchemy validations, and tried something like below, assuming that it will take care of the validations at the model level.
@validates('hios_plan_identifier')
def validate_hios_plan_identifier(self, key, hios_plan_identifier):
assert '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
return hios_plan_identifier
I need to put few validations on each variable. Only the rows which passes should be inserted.
I am not sure how to work on validations at model level. How can I achieve this.
python postgresql pandas sqlalchemy
python postgresql pandas sqlalchemy
edited Nov 13 '18 at 10:31
user1896796
asked Nov 13 '18 at 9:39
user1896796user1896796
129217
129217
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
This line:'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
.'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value ofhios_plan_identifier
.
– SuperShoot
Nov 13 '18 at 10:37
isre.search()
what you are looking for?
– SuperShoot
Nov 13 '18 at 10:44
1
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51
|
show 12 more comments
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
This line:'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
.'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value ofhios_plan_identifier
.
– SuperShoot
Nov 13 '18 at 10:37
isre.search()
what you are looking for?
– SuperShoot
Nov 13 '18 at 10:44
1
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
This line:
'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
. '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value of hios_plan_identifier
.– SuperShoot
Nov 13 '18 at 10:37
This line:
'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
. '/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value of hios_plan_identifier
.– SuperShoot
Nov 13 '18 at 10:37
is
re.search()
what you are looking for?– SuperShoot
Nov 13 '18 at 10:44
is
re.search()
what you are looking for?– SuperShoot
Nov 13 '18 at 10:44
1
1
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51
|
show 12 more comments
1 Answer
1
active
oldest
votes
Two options:
- Add a
CheckConstraint
on the column and add your regex there, see
https://www.postgresql.org/docs/9.3/functions-matching.html . That works in whatever way you throw the data into the database. - Use event based validation as demonstrated here , create a list of objects you want to insert and use
session.add_all()
for batch insert.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277979%2fproviding-validation-on-model-class-level-in-sqlalchemy%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Two options:
- Add a
CheckConstraint
on the column and add your regex there, see
https://www.postgresql.org/docs/9.3/functions-matching.html . That works in whatever way you throw the data into the database. - Use event based validation as demonstrated here , create a list of objects you want to insert and use
session.add_all()
for batch insert.
add a comment |
Two options:
- Add a
CheckConstraint
on the column and add your regex there, see
https://www.postgresql.org/docs/9.3/functions-matching.html . That works in whatever way you throw the data into the database. - Use event based validation as demonstrated here , create a list of objects you want to insert and use
session.add_all()
for batch insert.
add a comment |
Two options:
- Add a
CheckConstraint
on the column and add your regex there, see
https://www.postgresql.org/docs/9.3/functions-matching.html . That works in whatever way you throw the data into the database. - Use event based validation as demonstrated here , create a list of objects you want to insert and use
session.add_all()
for batch insert.
Two options:
- Add a
CheckConstraint
on the column and add your regex there, see
https://www.postgresql.org/docs/9.3/functions-matching.html . That works in whatever way you throw the data into the database. - Use event based validation as demonstrated here , create a list of objects you want to insert and use
session.add_all()
for batch insert.
answered Nov 22 '18 at 12:16


MichaelMichael
3,7542554
3,7542554
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277979%2fproviding-validation-on-model-class-level-in-sqlalchemy%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ptUx2U
Though it is somewhat obvious, you should provide a bit more information as to how is it not working. Questions without a clear problem statement are not useful in general.
– Ilja Everilä
Nov 13 '18 at 9:58
updated the whole question . Hope you get where I am stuck
– user1896796
Nov 13 '18 at 10:33
This line:
'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,' in hios_plan_identifier
.'/A(d5[A-Z]2[a-zA-Z0-9]3,7-TMP|d5[A-Z]2d3,7(-?d2)*)z/,'
is not a regex, it's just a normal string. That assert will not evaluate to True unless that whole string is in the value ofhios_plan_identifier
.– SuperShoot
Nov 13 '18 at 10:37
is
re.search()
what you are looking for?– SuperShoot
Nov 13 '18 at 10:44
1
I wonder if the ORM level validations even apply to bulk inserts.
– Ilja Everilä
Nov 13 '18 at 10:51