Columnar Transposition Cipher: How to encrypt
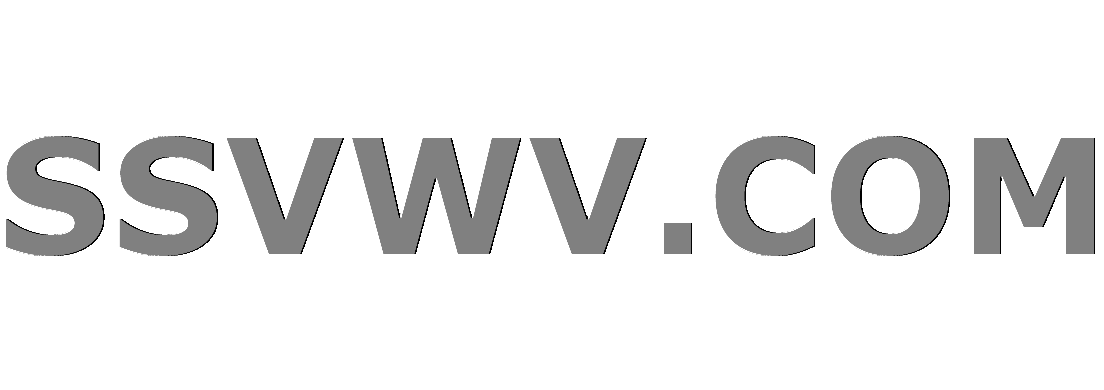
Multi tool use
Hi I am pretty new to Java and I am trying to figure out how to create a columnar transposition cipher. If you have created one can you show how it is coded. The cipher encrypts by finding printing out a column depending on the index of a key. For example if the key is TAPE then there would be 4 columns by how many ever rows depending on the length of a input string. Then the system would print out depending on which letter comes first in the alphabet, in this case the column A would be printed first then E and so on forth. I need to know how to print out these columns in the order of the key. I know how to create the columns for the input string. Any help would be greatly appreciated!!!
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
/// I am trying to sort the array and the give the index of the character in the sorted array to the same character of the unsorted array.
public String encrypt(String theString)
String s = theString;
int x = 0;
int y = 0;
for (int i = 0; (i * i) < s.length(); i++)
y = i;
x = i + 1;
// creates x arraylists
List<List<Character>> column = new ArrayList<>();
for (int i = 0; i < x; i++)
column.add(new ArrayList<>());
import java.util.Scanner;
public class TranspositionCipher
public static void main (String args)
Scanner sc = new Scanner(System.in);
System.out.println("Please print out a word for a key and a sentence for a string: ");
SortEncrypt s = new SortEncrypt(sc.nextLine());
EDIT:
I tried doing the sorting this way of the key:
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
{
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
String imp = tempArray.toString();
System.out.println(key);
System.out.println(tempArray);
for (int i = 0; i<imp.length();i ++)
char a_char = imp.charAt(i);
if (a_char == a_char)
int a = key.indexOf(a_char);
myList.add(a);
System.out.println(myList);
java
add a comment |
Hi I am pretty new to Java and I am trying to figure out how to create a columnar transposition cipher. If you have created one can you show how it is coded. The cipher encrypts by finding printing out a column depending on the index of a key. For example if the key is TAPE then there would be 4 columns by how many ever rows depending on the length of a input string. Then the system would print out depending on which letter comes first in the alphabet, in this case the column A would be printed first then E and so on forth. I need to know how to print out these columns in the order of the key. I know how to create the columns for the input string. Any help would be greatly appreciated!!!
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
/// I am trying to sort the array and the give the index of the character in the sorted array to the same character of the unsorted array.
public String encrypt(String theString)
String s = theString;
int x = 0;
int y = 0;
for (int i = 0; (i * i) < s.length(); i++)
y = i;
x = i + 1;
// creates x arraylists
List<List<Character>> column = new ArrayList<>();
for (int i = 0; i < x; i++)
column.add(new ArrayList<>());
import java.util.Scanner;
public class TranspositionCipher
public static void main (String args)
Scanner sc = new Scanner(System.in);
System.out.println("Please print out a word for a key and a sentence for a string: ");
SortEncrypt s = new SortEncrypt(sc.nextLine());
EDIT:
I tried doing the sorting this way of the key:
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
{
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
String imp = tempArray.toString();
System.out.println(key);
System.out.println(tempArray);
for (int i = 0; i<imp.length();i ++)
char a_char = imp.charAt(i);
if (a_char == a_char)
int a = key.indexOf(a_char);
myList.add(a);
System.out.println(myList);
java
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32
add a comment |
Hi I am pretty new to Java and I am trying to figure out how to create a columnar transposition cipher. If you have created one can you show how it is coded. The cipher encrypts by finding printing out a column depending on the index of a key. For example if the key is TAPE then there would be 4 columns by how many ever rows depending on the length of a input string. Then the system would print out depending on which letter comes first in the alphabet, in this case the column A would be printed first then E and so on forth. I need to know how to print out these columns in the order of the key. I know how to create the columns for the input string. Any help would be greatly appreciated!!!
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
/// I am trying to sort the array and the give the index of the character in the sorted array to the same character of the unsorted array.
public String encrypt(String theString)
String s = theString;
int x = 0;
int y = 0;
for (int i = 0; (i * i) < s.length(); i++)
y = i;
x = i + 1;
// creates x arraylists
List<List<Character>> column = new ArrayList<>();
for (int i = 0; i < x; i++)
column.add(new ArrayList<>());
import java.util.Scanner;
public class TranspositionCipher
public static void main (String args)
Scanner sc = new Scanner(System.in);
System.out.println("Please print out a word for a key and a sentence for a string: ");
SortEncrypt s = new SortEncrypt(sc.nextLine());
EDIT:
I tried doing the sorting this way of the key:
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
{
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
String imp = tempArray.toString();
System.out.println(key);
System.out.println(tempArray);
for (int i = 0; i<imp.length();i ++)
char a_char = imp.charAt(i);
if (a_char == a_char)
int a = key.indexOf(a_char);
myList.add(a);
System.out.println(myList);
java
Hi I am pretty new to Java and I am trying to figure out how to create a columnar transposition cipher. If you have created one can you show how it is coded. The cipher encrypts by finding printing out a column depending on the index of a key. For example if the key is TAPE then there would be 4 columns by how many ever rows depending on the length of a input string. Then the system would print out depending on which letter comes first in the alphabet, in this case the column A would be printed first then E and so on forth. I need to know how to print out these columns in the order of the key. I know how to create the columns for the input string. Any help would be greatly appreciated!!!
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
/// I am trying to sort the array and the give the index of the character in the sorted array to the same character of the unsorted array.
public String encrypt(String theString)
String s = theString;
int x = 0;
int y = 0;
for (int i = 0; (i * i) < s.length(); i++)
y = i;
x = i + 1;
// creates x arraylists
List<List<Character>> column = new ArrayList<>();
for (int i = 0; i < x; i++)
column.add(new ArrayList<>());
import java.util.Scanner;
public class TranspositionCipher
public static void main (String args)
Scanner sc = new Scanner(System.in);
System.out.println("Please print out a word for a key and a sentence for a string: ");
SortEncrypt s = new SortEncrypt(sc.nextLine());
EDIT:
I tried doing the sorting this way of the key:
import java.util.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Collections;
import java.io.PrintWriter;
public class SortEncrypt
{
public static String key;
public static char sortKey;
public SortEncrypt(String theKey)
key = theKey;
List<Integer> myList = new ArrayList<Integer>();
char tempArray = key.toCharArray();
Arrays.sort(tempArray);
String imp = tempArray.toString();
System.out.println(key);
System.out.println(tempArray);
for (int i = 0; i<imp.length();i ++)
char a_char = imp.charAt(i);
if (a_char == a_char)
int a = key.indexOf(a_char);
myList.add(a);
System.out.println(myList);
java
java
edited Nov 13 '18 at 9:55
Rishab Vivek
asked Nov 13 '18 at 9:16


Rishab VivekRishab Vivek
112
112
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32
add a comment |
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277541%2fcolumnar-transposition-cipher-how-to-encrypt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277541%2fcolumnar-transposition-cipher-how-to-encrypt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yxs,o5gHy3V,Ai1ca,j,ZSo S3AJrp7zozQuU iQQYTnbt
You should show what you have tried so far, preferably through some Java source code, where the problem is.
– amn
Nov 13 '18 at 9:18
Welcome to Stack Overflow! Please take the tour, look around, and read through the Help Center, in particular How do I ask a good question? If you run into a specific problem, research it thoroughly, search thoroughly here, and if you're still stuck post your code and a description of the problem. Also, remember to include Minimum, Complete, Verifiable Example. People will be glad to help
– Andreas
Nov 13 '18 at 9:18
Sorry @amn I am kinda new to both Java and StackOverflow i updated the post but my code is still not done to completion
– Rishab Vivek
Nov 13 '18 at 9:32