handle multiple checkboxes and complex data structure (reactjs)
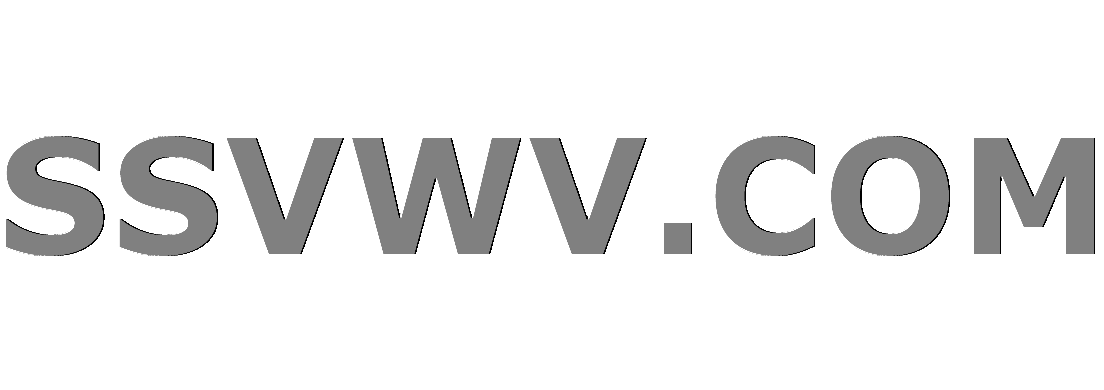
Multi tool use
up vote
2
down vote
favorite
Since I am pretty new to React and also not a huge expert on JS especially ES6, I wonder how to make my code (that works) prettier and refactor it.
I want to handle multiple checkboxes in a form and store the current value in the state object. Since the checkboxes are grouped I want a structure as answeredTasks like that:
"isLoaded":true,
"answeredTasks":
"1":[
"green",
"blue",
"red"
],
"5":[
"car",
"cat",
"house"
]
Here is my onChange method on a checkbox:
checkBoxOnChange = e =>
let currentAnswers = this.state.answeredTasks;
let element = e.currentTarget;
// if it is the first answer checked
if (currentAnswers[element.name] === undefined)
currentAnswers[element.name] = ;
// if uncheck
if (!element.checked)
const index = currentAnswers[element.name].indexOf(element.value);
currentAnswers[element.name].splice(index, 1);
else
currentAnswers[element.name].push(element.value);
this.setState( answeredTasks: currentAnswers );
;
Can you tell me how to improve my method, especially with ES6?
I mostly struggled with creating the data structure.
Thanks.
javascript arrays reactjs object ecmascript-6
add a comment |
up vote
2
down vote
favorite
Since I am pretty new to React and also not a huge expert on JS especially ES6, I wonder how to make my code (that works) prettier and refactor it.
I want to handle multiple checkboxes in a form and store the current value in the state object. Since the checkboxes are grouped I want a structure as answeredTasks like that:
"isLoaded":true,
"answeredTasks":
"1":[
"green",
"blue",
"red"
],
"5":[
"car",
"cat",
"house"
]
Here is my onChange method on a checkbox:
checkBoxOnChange = e =>
let currentAnswers = this.state.answeredTasks;
let element = e.currentTarget;
// if it is the first answer checked
if (currentAnswers[element.name] === undefined)
currentAnswers[element.name] = ;
// if uncheck
if (!element.checked)
const index = currentAnswers[element.name].indexOf(element.value);
currentAnswers[element.name].splice(index, 1);
else
currentAnswers[element.name].push(element.value);
this.setState( answeredTasks: currentAnswers );
;
Can you tell me how to improve my method, especially with ES6?
I mostly struggled with creating the data structure.
Thanks.
javascript arrays reactjs object ecmascript-6
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Since I am pretty new to React and also not a huge expert on JS especially ES6, I wonder how to make my code (that works) prettier and refactor it.
I want to handle multiple checkboxes in a form and store the current value in the state object. Since the checkboxes are grouped I want a structure as answeredTasks like that:
"isLoaded":true,
"answeredTasks":
"1":[
"green",
"blue",
"red"
],
"5":[
"car",
"cat",
"house"
]
Here is my onChange method on a checkbox:
checkBoxOnChange = e =>
let currentAnswers = this.state.answeredTasks;
let element = e.currentTarget;
// if it is the first answer checked
if (currentAnswers[element.name] === undefined)
currentAnswers[element.name] = ;
// if uncheck
if (!element.checked)
const index = currentAnswers[element.name].indexOf(element.value);
currentAnswers[element.name].splice(index, 1);
else
currentAnswers[element.name].push(element.value);
this.setState( answeredTasks: currentAnswers );
;
Can you tell me how to improve my method, especially with ES6?
I mostly struggled with creating the data structure.
Thanks.
javascript arrays reactjs object ecmascript-6
Since I am pretty new to React and also not a huge expert on JS especially ES6, I wonder how to make my code (that works) prettier and refactor it.
I want to handle multiple checkboxes in a form and store the current value in the state object. Since the checkboxes are grouped I want a structure as answeredTasks like that:
"isLoaded":true,
"answeredTasks":
"1":[
"green",
"blue",
"red"
],
"5":[
"car",
"cat",
"house"
]
Here is my onChange method on a checkbox:
checkBoxOnChange = e =>
let currentAnswers = this.state.answeredTasks;
let element = e.currentTarget;
// if it is the first answer checked
if (currentAnswers[element.name] === undefined)
currentAnswers[element.name] = ;
// if uncheck
if (!element.checked)
const index = currentAnswers[element.name].indexOf(element.value);
currentAnswers[element.name].splice(index, 1);
else
currentAnswers[element.name].push(element.value);
this.setState( answeredTasks: currentAnswers );
;
Can you tell me how to improve my method, especially with ES6?
I mostly struggled with creating the data structure.
Thanks.
javascript arrays reactjs object ecmascript-6
javascript arrays reactjs object ecmascript-6
asked Nov 10 at 19:41
André
111
111
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59
add a comment |
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
I'm not the first but here is my version with a test
static changeAnswers = (target: name, value, checked) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
checkBoxOnChange = e =>
const answeredTasks = this.state
this.setState(answeredTasks: myComponent.changeAnswers(e))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
add a comment |
up vote
0
down vote
I preferred I could see your whole code.
But if I wanted to suggest you any change:
In general, avoid using "let" in your code and instead use "const". This way, you avoid mutating data which is the industry best practice, and React documentation highly recommends that.
Avoid using splice/push/pop methods which mutate data, and instead use array functions such as filter/map/some/every etc... The methods I mentioned do not mutate data and instead return a referencially different object.
Here is the change you should make:
checkBoxOnChange = e =>
const currentAnswers = this.state.answeredTasks;
const element = e.target;
const newAnswers = !element.checked ?
(currentAnswers[element.name] ;
Please note that the ...currentAnswers, newAnswers inside setState() function is merging the currentAnswers with the new changes in newAnswers. i.e. newAnswers will overwrite the specific object in the outer array.
This is the Functional way of doing things.
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I'm not the first but here is my version with a test
static changeAnswers = (target: name, value, checked) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
checkBoxOnChange = e =>
const answeredTasks = this.state
this.setState(answeredTasks: myComponent.changeAnswers(e))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
add a comment |
up vote
0
down vote
I'm not the first but here is my version with a test
static changeAnswers = (target: name, value, checked) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
checkBoxOnChange = e =>
const answeredTasks = this.state
this.setState(answeredTasks: myComponent.changeAnswers(e))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
add a comment |
up vote
0
down vote
up vote
0
down vote
I'm not the first but here is my version with a test
static changeAnswers = (target: name, value, checked) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
checkBoxOnChange = e =>
const answeredTasks = this.state
this.setState(answeredTasks: myComponent.changeAnswers(e))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
I'm not the first but here is my version with a test
static changeAnswers = (target: name, value, checked) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
checkBoxOnChange = e =>
const answeredTasks = this.state
this.setState(answeredTasks: myComponent.changeAnswers(e))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
const answeredTasks = "1": ["green", "blue", "red"]
const elements = [
name: "1", value: "green", checked: false ,
name: "1", value: "black", checked: true ,
name: "2", value: "lol", checked: true ,
name: "2", value: "lol", checked: false
]
const click = ( name, value, checked ) =>
checked
? ...answeredTasks, ... [name]: [...(answeredTasks[name]
:
...answeredTasks,
... [name]: (answeredTasks[name]
elements.map(element => console.log(click(element)))
edited Nov 10 at 22:41
answered Nov 10 at 21:58
victor zadorozhnyy
251412
251412
add a comment |
add a comment |
up vote
0
down vote
I preferred I could see your whole code.
But if I wanted to suggest you any change:
In general, avoid using "let" in your code and instead use "const". This way, you avoid mutating data which is the industry best practice, and React documentation highly recommends that.
Avoid using splice/push/pop methods which mutate data, and instead use array functions such as filter/map/some/every etc... The methods I mentioned do not mutate data and instead return a referencially different object.
Here is the change you should make:
checkBoxOnChange = e =>
const currentAnswers = this.state.answeredTasks;
const element = e.target;
const newAnswers = !element.checked ?
(currentAnswers[element.name] ;
Please note that the ...currentAnswers, newAnswers inside setState() function is merging the currentAnswers with the new changes in newAnswers. i.e. newAnswers will overwrite the specific object in the outer array.
This is the Functional way of doing things.
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
add a comment |
up vote
0
down vote
I preferred I could see your whole code.
But if I wanted to suggest you any change:
In general, avoid using "let" in your code and instead use "const". This way, you avoid mutating data which is the industry best practice, and React documentation highly recommends that.
Avoid using splice/push/pop methods which mutate data, and instead use array functions such as filter/map/some/every etc... The methods I mentioned do not mutate data and instead return a referencially different object.
Here is the change you should make:
checkBoxOnChange = e =>
const currentAnswers = this.state.answeredTasks;
const element = e.target;
const newAnswers = !element.checked ?
(currentAnswers[element.name] ;
Please note that the ...currentAnswers, newAnswers inside setState() function is merging the currentAnswers with the new changes in newAnswers. i.e. newAnswers will overwrite the specific object in the outer array.
This is the Functional way of doing things.
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
add a comment |
up vote
0
down vote
up vote
0
down vote
I preferred I could see your whole code.
But if I wanted to suggest you any change:
In general, avoid using "let" in your code and instead use "const". This way, you avoid mutating data which is the industry best practice, and React documentation highly recommends that.
Avoid using splice/push/pop methods which mutate data, and instead use array functions such as filter/map/some/every etc... The methods I mentioned do not mutate data and instead return a referencially different object.
Here is the change you should make:
checkBoxOnChange = e =>
const currentAnswers = this.state.answeredTasks;
const element = e.target;
const newAnswers = !element.checked ?
(currentAnswers[element.name] ;
Please note that the ...currentAnswers, newAnswers inside setState() function is merging the currentAnswers with the new changes in newAnswers. i.e. newAnswers will overwrite the specific object in the outer array.
This is the Functional way of doing things.
I preferred I could see your whole code.
But if I wanted to suggest you any change:
In general, avoid using "let" in your code and instead use "const". This way, you avoid mutating data which is the industry best practice, and React documentation highly recommends that.
Avoid using splice/push/pop methods which mutate data, and instead use array functions such as filter/map/some/every etc... The methods I mentioned do not mutate data and instead return a referencially different object.
Here is the change you should make:
checkBoxOnChange = e =>
const currentAnswers = this.state.answeredTasks;
const element = e.target;
const newAnswers = !element.checked ?
(currentAnswers[element.name] ;
Please note that the ...currentAnswers, newAnswers inside setState() function is merging the currentAnswers with the new changes in newAnswers. i.e. newAnswers will overwrite the specific object in the outer array.
This is the Functional way of doing things.
edited Nov 12 at 2:11


Pang
6,8031563101
6,8031563101
answered Nov 10 at 21:15
xeiton
2216
2216
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
add a comment |
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
"let" and instead use "const" - it is not important in the case of objects. When you're "mutating" an array or object you're not re-assigning or re-declaring the constant, it's already declared and assigned, you're just adding to the "list" that the constant points to.
– victor zadorozhnyy
Nov 10 at 22:04
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Victor, you are a bit confused. Mutation is NOT re-declaring a new object, and that is exactly why it is bad !! ... Functional programming doesn't like side effects, and doesn't like making the changes to the referentially same object. Immutability means you should referencially create a new object with every new change, which is the industry best practice and ReactJs is highly recommending it.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
Modifying the object/array with methods like splice/pop/push are mutating the object (changing the same object), where as using map/filter/some/every are NOT. They will referentially return a new object/array which is the "pure" way of doing things, and thus recommended by ReactJs.
– xeiton
Nov 11 at 22:13
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242743%2fhandle-multiple-checkboxes-and-complex-data-structure-reactjs%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fk9hGb 3S4Hw3I5,z96ulQL0R5O,7pBE5yLO5icAKvfw5,teuSb5ZX,Cs79CxH0ALlhI cks1s,Zy19eT47lyPBoZsG7h
Can you please provide the code to your whole component code? Also, as an advice, avoid using "let" in your code and instead use "const" to avoid mutating data. Try learning functional programming paradigm which is the best industry practice.
– xeiton
Nov 10 at 20:59